Auto expanding archives in PowerShell allows you to automatically extract and expand the contents of a compressed file upon access, enhancing your file management efficiency. Here's a simple code snippet to achieve this:
Expand-Archive -Path 'C:\Path\To\Your\Archive.zip' -DestinationPath 'C:\Path\To\Your\Destination' -Force
Understanding Archives in PowerShell
What is an Archive?
An archive is a collection of one or more files that have been combined and compressed into a single file for easier storage and transfer. Archives are essential for organizing data, providing backups, and facilitating file-sharing processes. In many IT contexts, archives serve as a way to manage data retention and retrieval, ultimately helping to optimize storage space.
Types of Archives
PowerShell provides support for various archive formats, with ZIP being the most commonly used. Other formats, such as TAR or GZ, can also be handled using PowerShell, either natively or through additional modules. Understanding these formats equips you with the necessary tools to manage your archived data effectively.
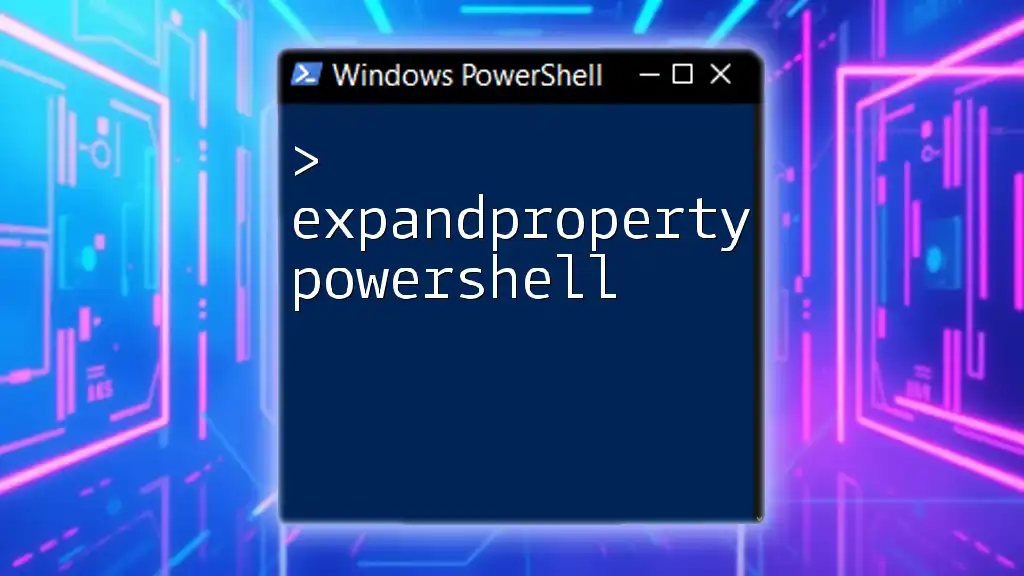
The Need for Auto Expanding Archives
Why Auto Expand?
The implementation of auto expanding archives significantly enhances efficiency by automating the extraction process. This allows you to avoid the tedious and time-consuming task of manual extraction. Saving time translates directly to increased productivity, particularly in environments where frequent updates and backups are necessary.
Use Cases for Auto Expanding Archives
Several scenarios exemplify the utility of auto expanding archives:
- Backup and Recovery: Automatically extracting archived backups when new files are created streamlines the recovery process.
- Real-Time Data Processing Tasks: For data pipelines, auto expanding archives can help maintain up-to-date datasets continuously.
- Automated Deployment Scenarios: In CI/CD (Continuous Integration/Continuous Deployment) pipelines, using auto expanding archives can simplify the deployment of application updates.
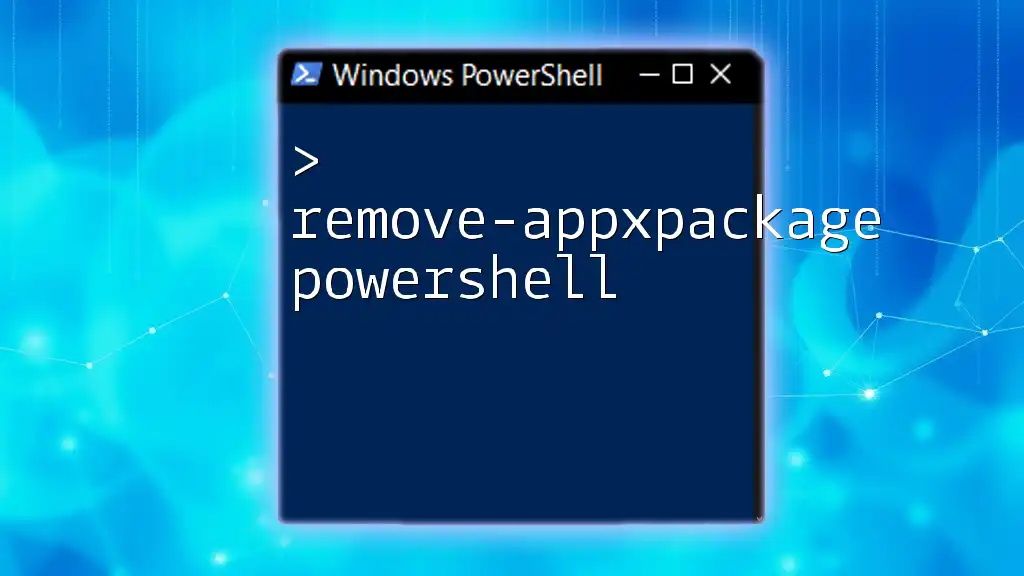
Setting Up Your Environment
Required PowerShell Version
Before diving into creating auto expanding archives, ensure you have an appropriate version of PowerShell. PowerShell 5.1 or later is recommended, as it natively supports the management of archives.
Installation of Necessary Modules
To effectively work with archives in PowerShell, you may need the `Microsoft.PowerShell.Archive` module. This module includes cmdlets that simplify operations related to archiving.
You can install this module using the following command:
Install-Module -Name Microsoft.PowerShell.Archive -Force
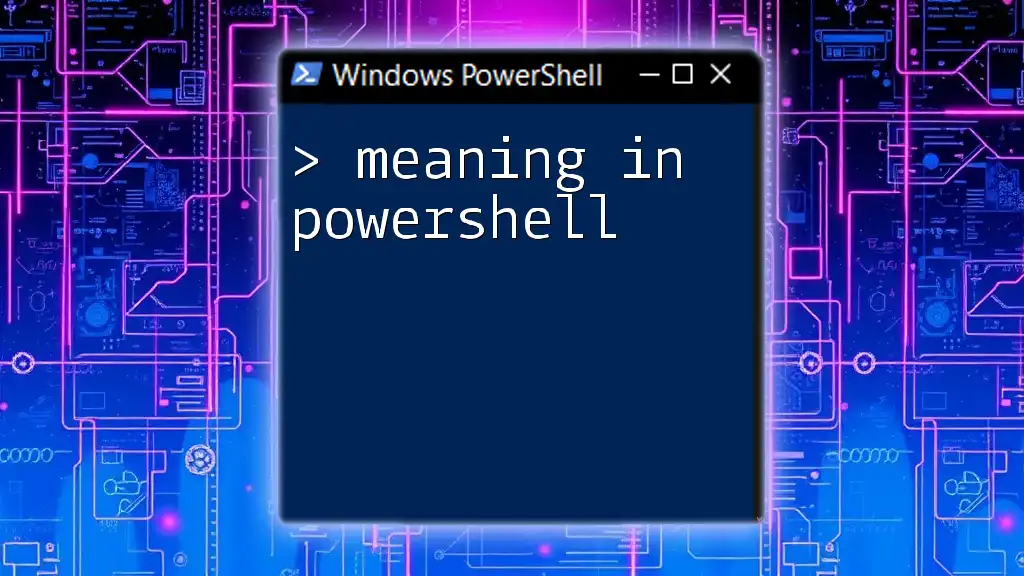
Basic Archive Commands in PowerShell
Creating a ZIP Archive
The `Compress-Archive` cmdlet is the primary tool for creating ZIP archives in PowerShell. This cmdlet allows you to specify a path to the files or directories you want to compress. Here’s a simple example:
Compress-Archive -Path "C:\source\*" -DestinationPath "C:\backup\archive.zip"
This command compresses all files in the `C:\source` directory into a single archive named `archive.zip` located in the `C:\backup` directory.
Extracting a ZIP Archive
To extract files from a ZIP archive, use the `Expand-Archive` cmdlet. This cmdlet takes the path to your archive and the intended destination for the extracted files. Here’s how it works in practice:
Expand-Archive -Path "C:\backup\archive.zip" -DestinationPath "C:\restore\"
This command extracts the contents of `archive.zip` into the `C:\restore` directory, making the files accessible immediately.
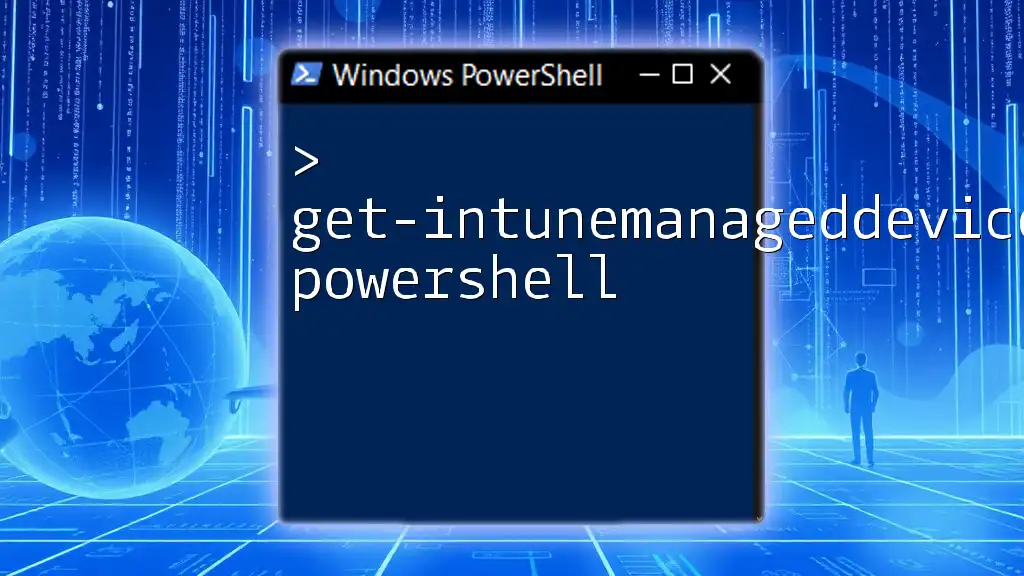
Implementing Auto Expanding Archives
The Concept of Auto Expansion
In the context of PowerShell, auto expansion refers to the automatic extraction of files from an archive upon certain triggers, such as the arrival of a new ZIP file in a designated directory. This automated process can significantly reduce the manual overhead involved in file management tasks.
Using PowerShell to Monitor Directory Changes
Introduction to FileSystemWatcher
The `FileSystemWatcher` class is an invaluable tool for tracking changes to directory contents. This feature gives you the capability to monitor changes, such as the creation of new archive files, enabling real-time responses to these events.
Example of a PowerShell Script Using FileSystemWatcher
To set up file monitoring and execute an auto expansion when a new ZIP file is created, use the following PowerShell script:
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = 'C:\backup'
$watcher.Filter = '*.zip'
$watcher.EnableRaisingEvents = $true
Register-ObjectEvent $watcher 'Created' -Action {
Expand-Archive -Path $Event.SourceEventArgs.FullPath -DestinationPath 'C:\restore\'
}
In this script:
- A watcher is initialized to monitor the `C:\backup` directory for any new files with a `.zip` extension.
- Upon detecting a newly created ZIP file, it automatically executes the extraction using `Expand-Archive`.
Scheduling Automatic Extraction with Task Scheduler
For scenarios where you want to implement auto expansion at specific intervals, the Windows Task Scheduler is an excellent solution. Here’s how to create a scheduled task that triggers a PowerShell script to expand archives regularly:
- Open Task Scheduler and create a new task.
- Set your task's trigger to occur based on a defined schedule (e.g., daily, weekly).
- Under the "Actions" tab, select "Start a program" and input the path to your PowerShell script that includes the extraction commands.
- Configure conditions and settings as needed, ensuring that the task runs even if you’re not logged in.
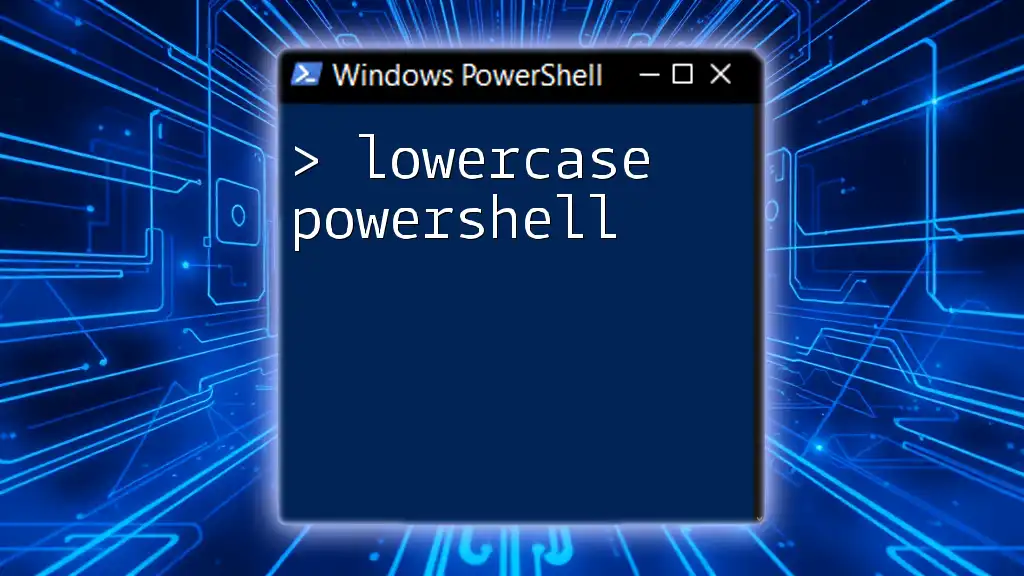
Common Issues and Troubleshooting
Error Handling in PowerShell Scripts
When developing scripts, it is crucial to incorporate error handling to gracefully manage unexpected scenarios. Use the `Try-Catch` block to capture errors efficiently and provide feedback.
For example:
try {
Expand-Archive -Path "C:\backup\archive.zip" -DestinationPath "C:\restore\"
} catch {
Write-Host "Error: $($_.Exception.Message)"
}
This block attempts to extract the archive and catches any errors, outputting a message if something goes wrong.
Resolving Extraction Errors
Users may encounter various issues while extracting archives, including:
- File path issues: Ensure the paths specified are correct and accessible.
- Corrupted ZIP files: If an archive is corrupted, extraction will fail. Always validate the integrity of your archives.
By understanding these common problems and their solutions, you can manage your archives more effectively.
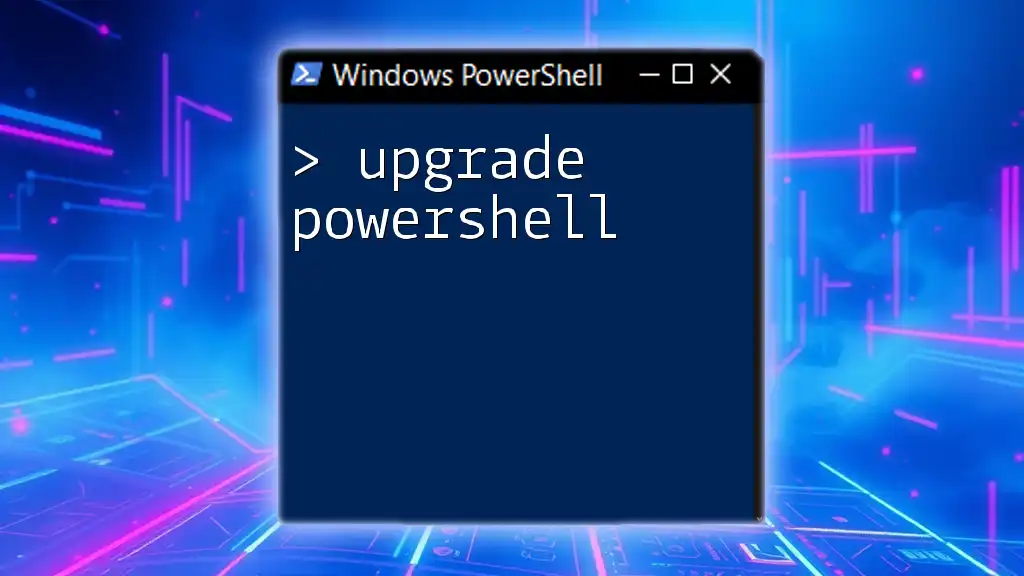
Best Practices for Using Auto Expanding Archives
Regular Maintenance
Keeping your scripts and monitoring processes updated is crucial. Regularly review and refine your auto expanding archive setup to ensure it meets your current needs. Consider implementing automated logging to monitor the extraction process for better troubleshooting.
Security Considerations
Always prioritize security when dealing with archives, especially those from untrusted sources. To prevent potential risks, employ practices such as:
- Scanning archive files for malware before extraction.
- Configuring user permissions carefully to control access to the extracted files.
- Using secure paths for storing sensitive data.
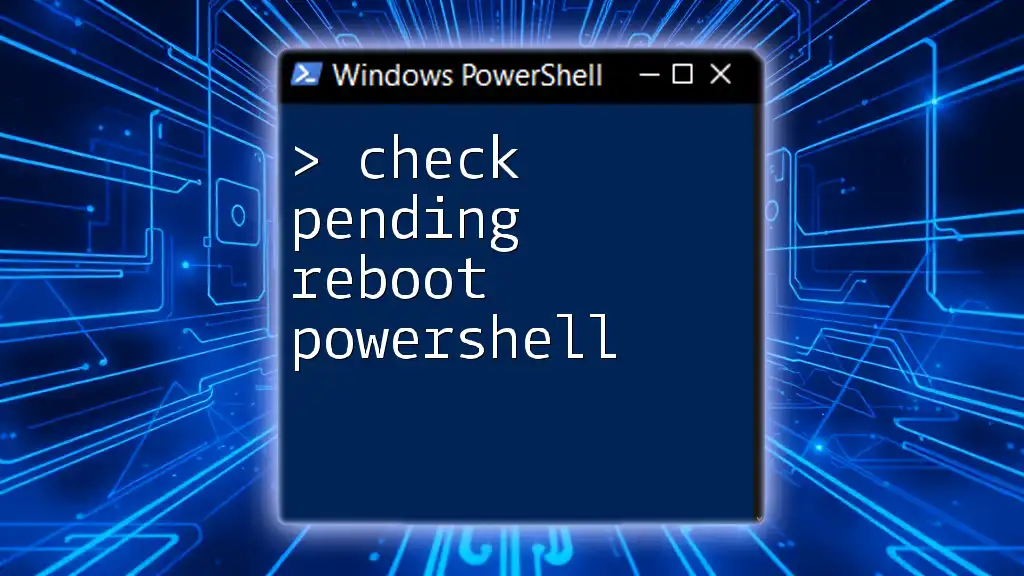
Conclusion
Auto expanding archives in PowerShell represent an outstanding approach to streamline file management tasks. By automating the extraction process, you can significantly enhance productivity and efficiency within your workflows. Implementing the commands, examples, and best practices outlined in this guide will not only help you manage your archives but also inspire you to explore further applications of PowerShell in your daily tasks. For more insights and resources, be sure to stay connected and leverage the power of PowerShell to its fullest!
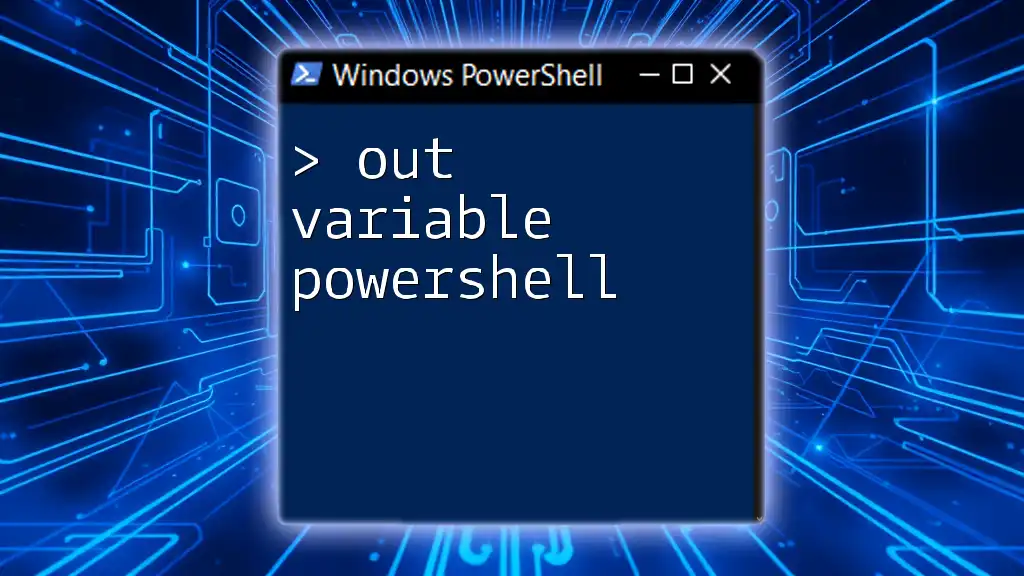
Additional Resources
- [Official PowerShell Documentation on Archiving](https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.archive/)
- Recommended blogs and video tutorials for advanced PowerShell users.
With this comprehensive guide, you are now well-equipped to dive into the world of auto expanding archives in PowerShell.