You can set a printer as the default printer in PowerShell using the following command:
Set-Printer -Name "YourPrinterName" -IsDefault $true
Just replace `"YourPrinterName"` with the name of the printer you wish to set as default.
Understanding the Default Printer Concept
What is a Default Printer?
A default printer is the printer that your computer automatically uses when you send a document for printing. This is particularly significant in environments where multiple printers are available. Using a default printer can enhance efficiency by eliminating the need to select a printer each time.
Why Use PowerShell to Set a Default Printer?
PowerShell is an incredibly powerful tool that allows for automation and scripting, which can be especially beneficial in corporate environments. Here are a few reasons why using PowerShell to set a default printer is preferable:
- Automation: Streamline repetitive tasks across multiple machines.
- Configurability: Create scripts that can adapt the printer settings based on conditions or user profiles.
- Remote Management: Manage printers in remote environments without needing to log in physically.
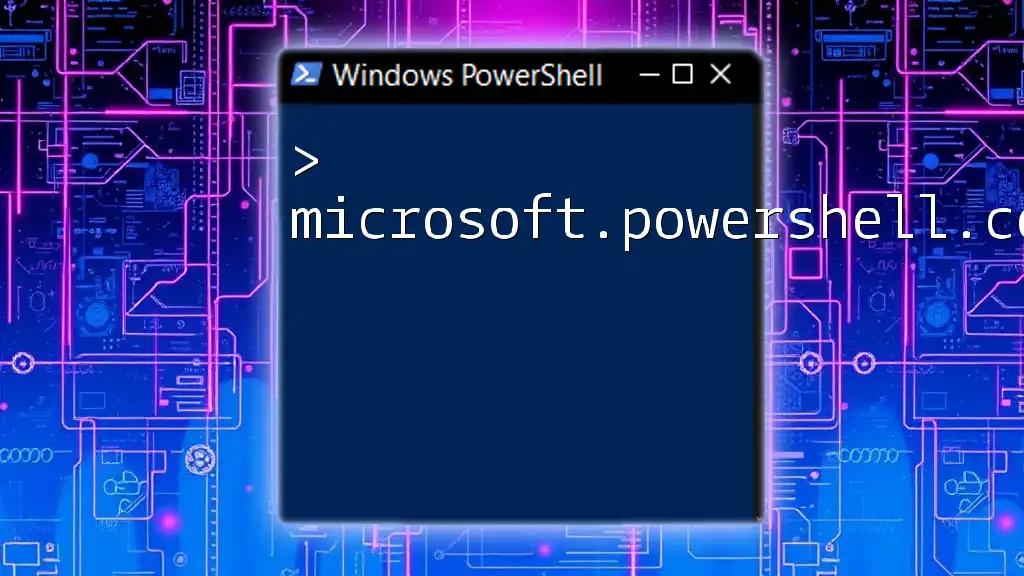
Prerequisites
PowerShell Version Requirements
Before diving into the commands to set your printer as default in PowerShell, it is essential to check your PowerShell version. You can do this by executing the following command:
$PSVersionTable.PSVersion
Ensure that you are using a version that supports the `Set-Printer` cmdlet, which is available in Windows 8/Windows Server 2012 and later versions.
Permissions Needed
To set a printer as default using PowerShell, you need the appropriate permissions. Typically, administrative rights are required to modify printer settings. Verify your user account controls and permissions accordingly.
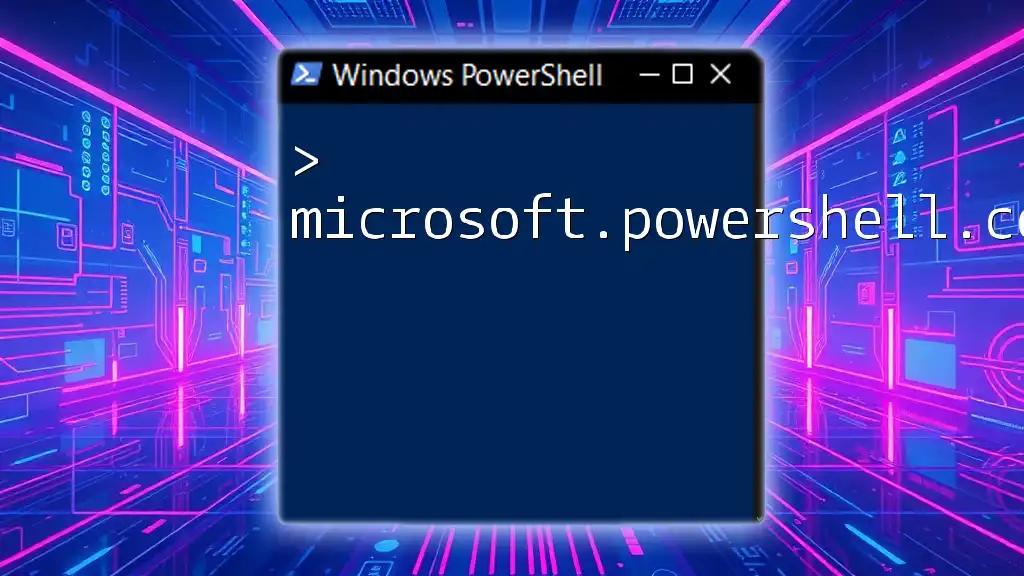
Setting the Default Printer using PowerShell
Basic Command Structure
The primary command to set a printer as default is straightforward. The syntax generally follows this structure:
Set-Printer -Name "YourPrinterName" -IsDefault $true
In this command:
- `-Name` specifies the printer's name as it appears on your system.
- `-IsDefault $true` sets the specified printer as the default printer.
Example Command
Here’s a practical example. Suppose you have a printer named "Office_Printer". You would run:
Set-Printer -Name "Office_Printer" -IsDefault $true
This command immediately sets "Office_Printer" as the default, allowing all print jobs to route there automatically.
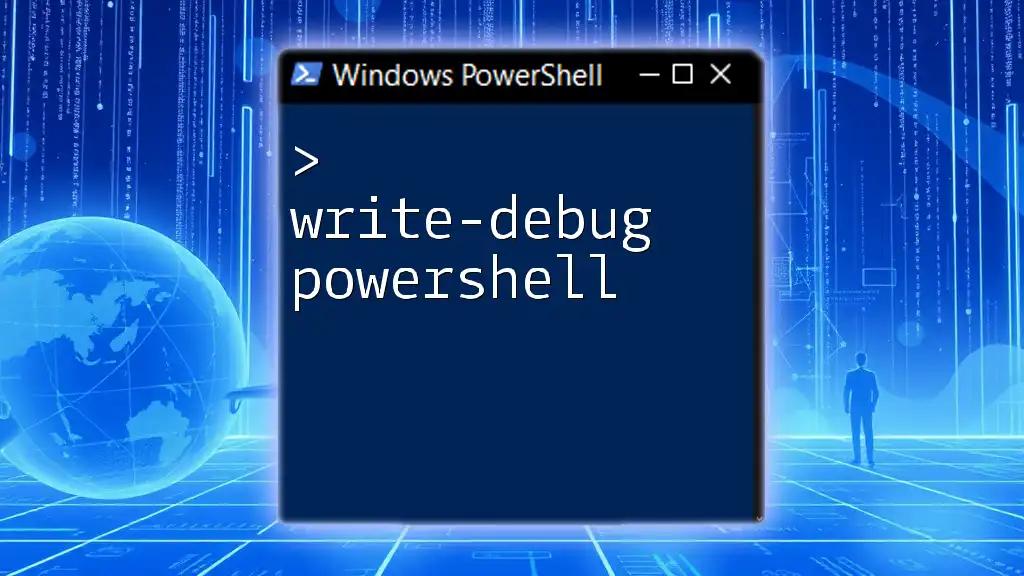
Listing Available Printers
How to View Installed Printers
Before setting a printer as default, you'll often need to know what printers are installed on your machine. You can easily obtain a list of all installed printers with the following command:
Get-Printer
The output will show you essential details such as the printer name, status, and whether it is currently set as the default printer. Look for the printer you want to set and ensure you have the correct name for the next command.
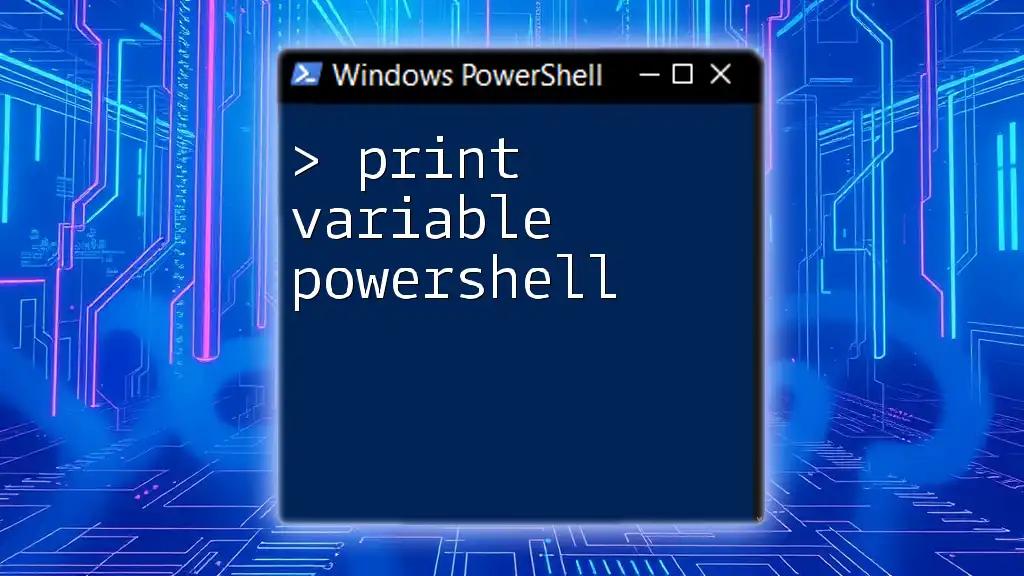
Additional Options with PowerShell
Setting a Default Printer based on Conditions
PowerShell allows for advanced scripting and conditional logic. For instance, if you want to set the default printer based on the user’s geographic location or office, you can incorporate conditions into your scripts. Here is an illustrative example:
if ($env:COMPUTERNAME -eq "Office-PC") {
Set-Printer -Name "Office_Printer" -IsDefault $true
} elseif ($env:COMPUTERNAME -eq "Home-PC") {
Set-Printer -Name "Home_Printer" -IsDefault $true
}
This script checks the computer name and sets the default printer accordingly.
Using Profiles for Different Users
In a multi-user environment, you might want to create custom scripts tailored for each user. This can be done with a user's profile script. Each user could have their specfic printer settings without interfering with others.
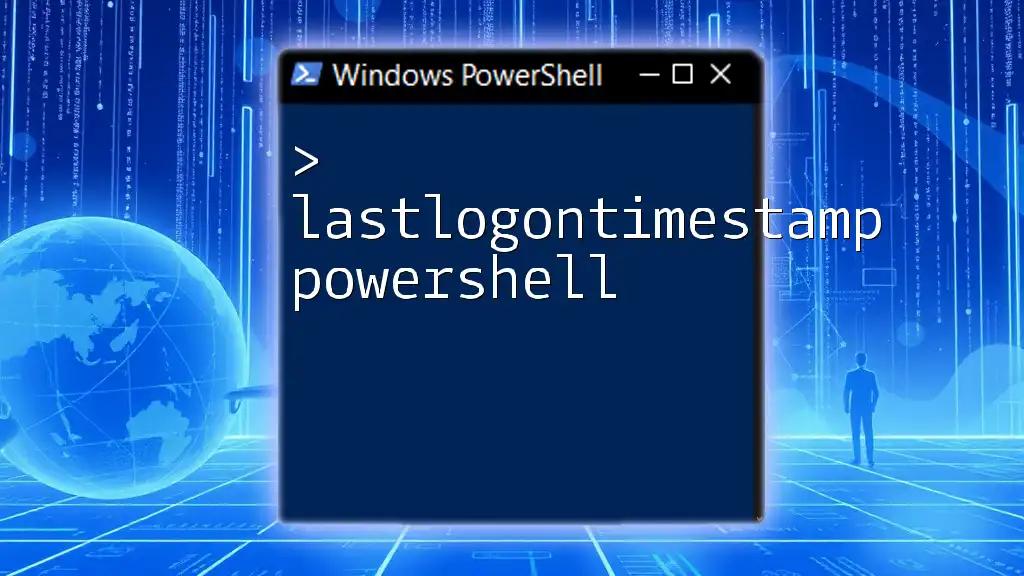
Troubleshooting Common Issues
Common Errors When Setting Default Printer
While setting a printer as default using PowerShell, you may encounter various error messages. Common issues include:
- Printer not found: This could be due to a typo in the printer name or the printer not being installed. Double-check the spelling and ensure the printer is connected and accessible.
To resolve these issues, ensure to go through the list of installed printers using the `Get-Printer` command to confirm the name.
Verifying the Default Printer is Set Correctly
After executing the command to set the default printer, it’s prudent to verify if it has been set successfully. You can check the current default printer with the following command:
Get-Printer | Where-Object { $_.IsDefault -eq $true }
If executed correctly, this command will return the currently set default printer, allowing you to confirm your action was successful.
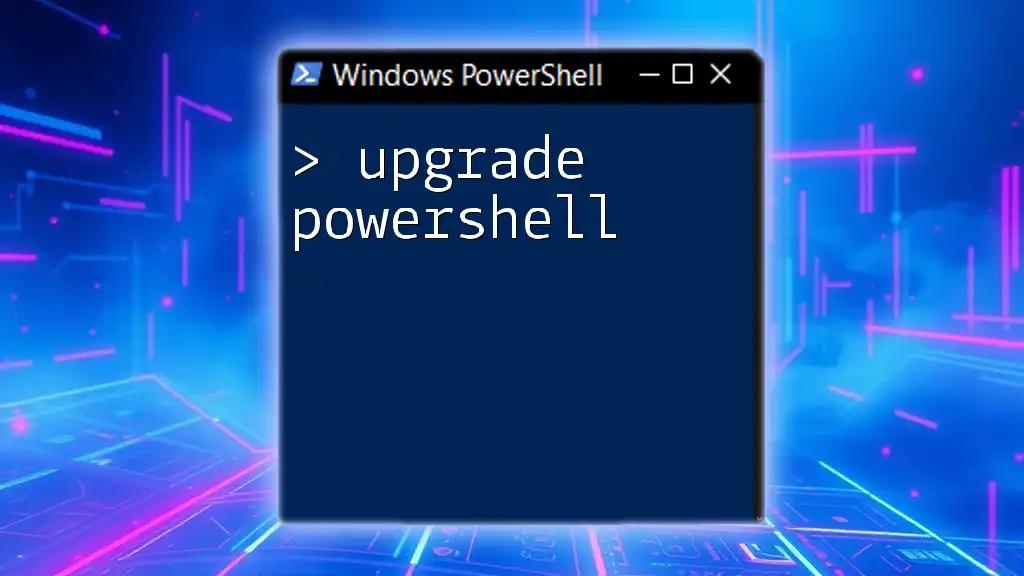
Automating Printer Settings
Creating a Script for Multiple Printers
If you manage several machines or require multiple default printers, it’s logical to create a script that can set various printers as defaults. For example:
$printers = @("Printer1", "Printer2")
foreach ($printer in $printers) {
Set-Printer -Name $printer -IsDefault $true
}
In this script, you define an array of printers and loop through each one to set it as default. While this example sets each printer successively, ensure you customize this logic based on your needs.
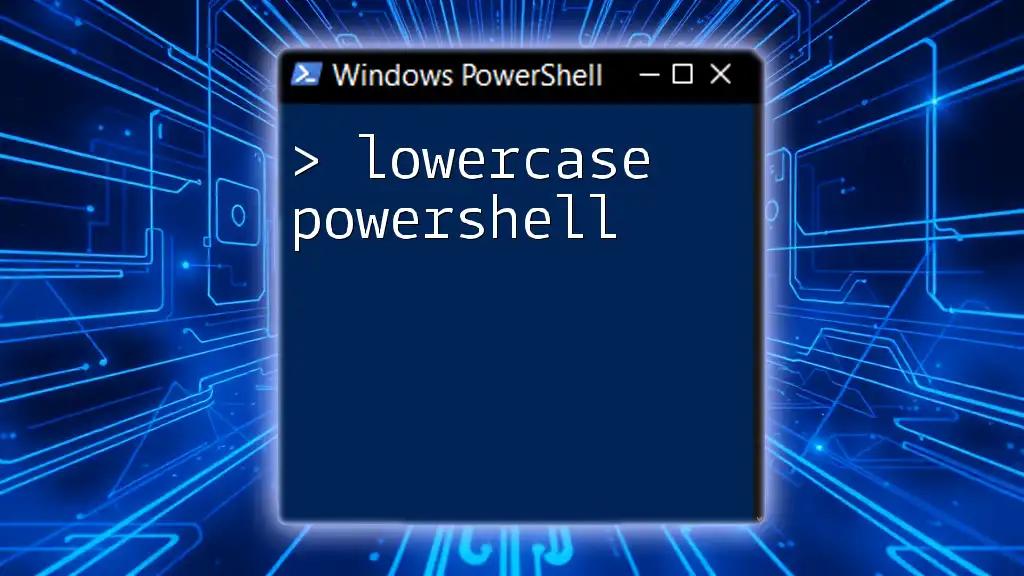
Conclusion
Setting a printer as default using PowerShell not only simplifies your printing tasks but also empowers you with automation and flexibility in multi-printer environments. By following this guide, you've learned how to effectively manage your printer settings through PowerShell commands.
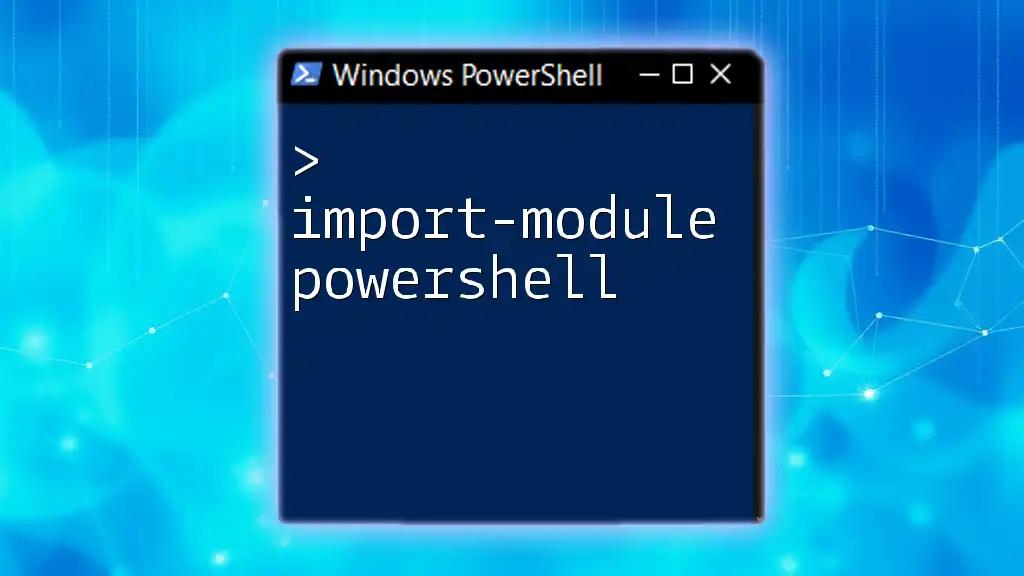
Call to Action
Are you interested in mastering PowerShell? Subscribe to our newsletter for more PowerShell tutorials, tips, and resources. Unlock the full potential of your scripting skills today!