PowerShell web scraping allows users to extract data from websites by sending HTTP requests and parsing the HTML content to retrieve specific information.
Here’s a simple code snippet to demonstrate how to perform basic web scraping in PowerShell:
# Fetching HTML content from a webpage and displaying the title
$response = Invoke-WebRequest -Uri 'https://example.com'
$contents = $response.Content
$title = ($contents -split '<title>')[1] -split '</title>' | Select-Object -First 1
Write-Host "Page Title: $title"
This code retrieves the HTML from 'https://example.com' and extracts the title of the page.
What is Web Scraping?
Web scraping is a method used to extract data from websites. It involves making requests to web pages and retrieving their content, which can then be parsed and analyzed for specific information. Web scraping is essential in various fields, including data journalism, market research, competitive analysis, and academic research.
PowerShell web scraping offers a unique advantage for Windows users. With its built-in commands and capabilities, PowerShell makes it easy to automate the retrieval and manipulation of web data efficiently.
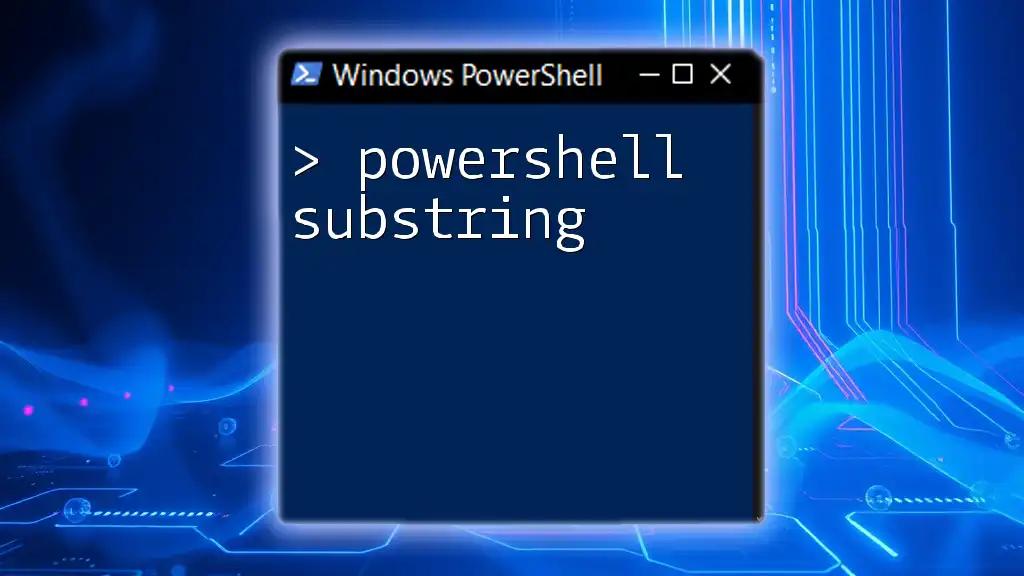
Why Use PowerShell for Web Scraping?
PowerShell stands out for several reasons when it comes to web scraping:
-
Simplicity and Accessibility: PowerShell is user-friendly, especially for those familiar with the Windows environment. Its straightforward syntax makes it easy to craft scripts for web scraping without needing complex programming skills.
-
Integration with Windows Systems: As a Windows-native scripting language, PowerShell can seamlessly interact with the operating system, allowing you to automate tasks that involve file handling, data processing, or other local operations along with web scraping.
-
Automation of Repetitive Tasks: With PowerShell, you can easily automate web scraping processes, which can save time and reduce manual effort. This is particularly beneficial when dealing with large datasets or regularly updated content.
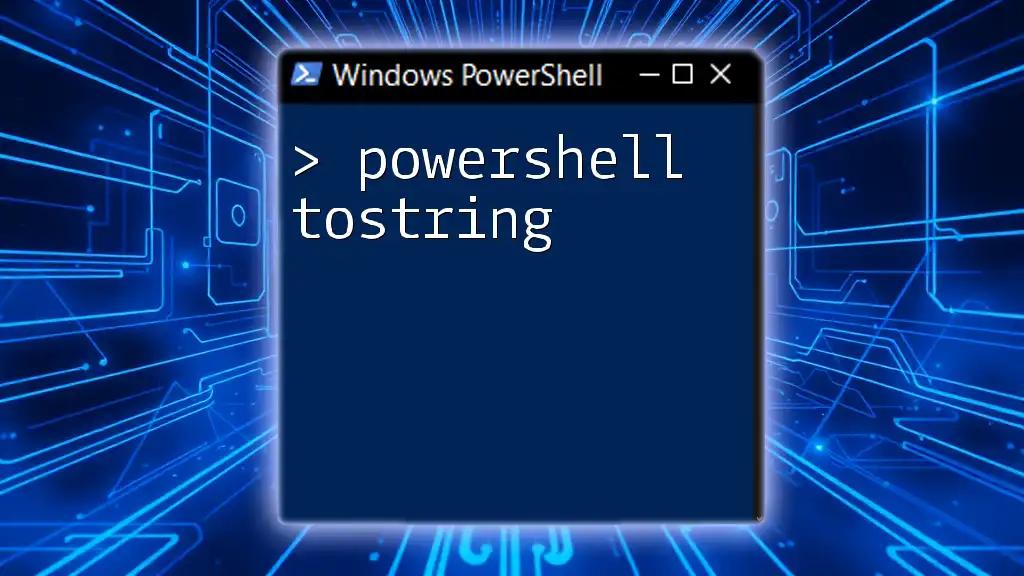
Getting Started with PowerShell
Installing Required Modules
Before diving into PowerShell web scraping, make sure you have the necessary modules installed. You will typically work with the `Invoke-WebRequest` cmdlet (built-in) and may want to use `Html Agility Pack` for more advanced HTML manipulation.
To install the Html Agility Pack, run the following command in your PowerShell environment:
Install-Package HtmlAgilityPack
Basic Commands for Web Scraping
Using `Invoke-WebRequest`
The `Invoke-WebRequest` cmdlet is the cornerstone of web scraping in PowerShell. It allows you to send HTTP requests to sites and retrieve their responses.
Here’s an example of a simple web scraping request:
$response = Invoke-WebRequest -Uri "http://example.com"
This command fetches the contents of the specified URL and stores the response in the `$response` variable.
Understanding the Response Object
When you receive a response from a web request, it comes with rich information encapsulated in a response object.
To access the main content of the web page, you can use:
$content = $response.Content
Additionally, you can check the status code to ensure your request was successful:
$status = $response.StatusCode
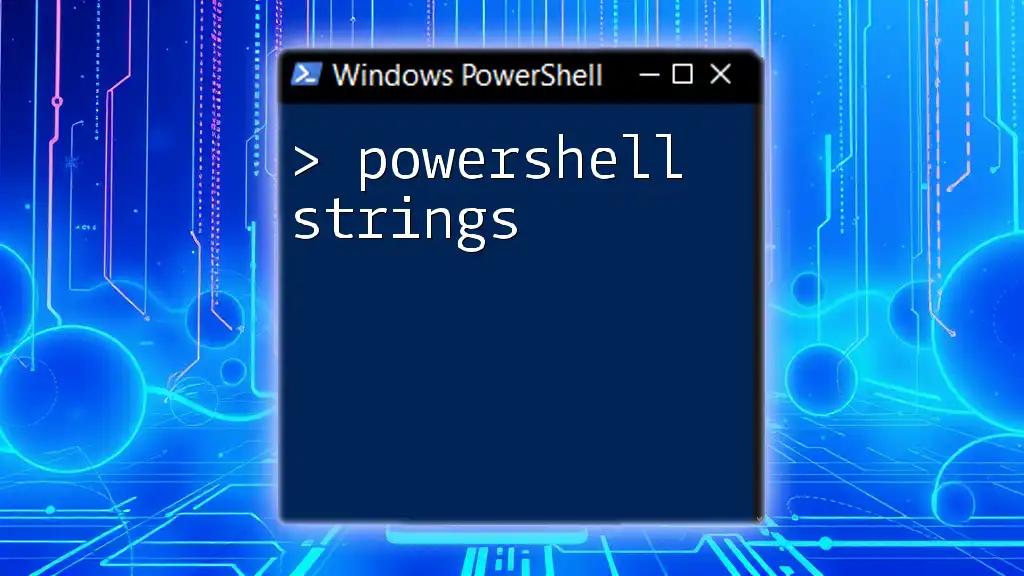
Practical Web Scraping Examples
Scraping Data from a Static Website
Case Study: Scraping Headlines from a News Site
Let’s consider a scenario where you want to extract headlines from a news website. Here's how you can achieve that:
- First, target a website you want to scrape.
- Use `Invoke-WebRequest` to fetch its HTML content.
- Parse the necessary elements using the appropriate selectors.
Here’s a sample code snippet to scrape headlines:
$response = Invoke-WebRequest -Uri "http://newswebsite.com"
$headlines = $response.ParsedHtml.getElementsByTagName("h2")
foreach ($headline in $headlines) {
Write-Output $headline.innerText
}
The above code retrieves the `<h2>` elements from the HTML and outputs their inner text, which typically corresponds to news headlines.
Scraping Dynamic Content
Using APIs for Data Extraction
Many websites nowadays use APIs to serve content dynamically. PowerShell is well-equipped to handle API requests as well.
To send a GET request to a REST API and retrieve data, you can use:
$response = Invoke-RestMethod -Uri "http://api.example.com/data"
This command will return the response as an object that you can manipulate further. If the API endpoint returns data in JSON format, PowerShell will automatically convert it into a usable PowerShell object.
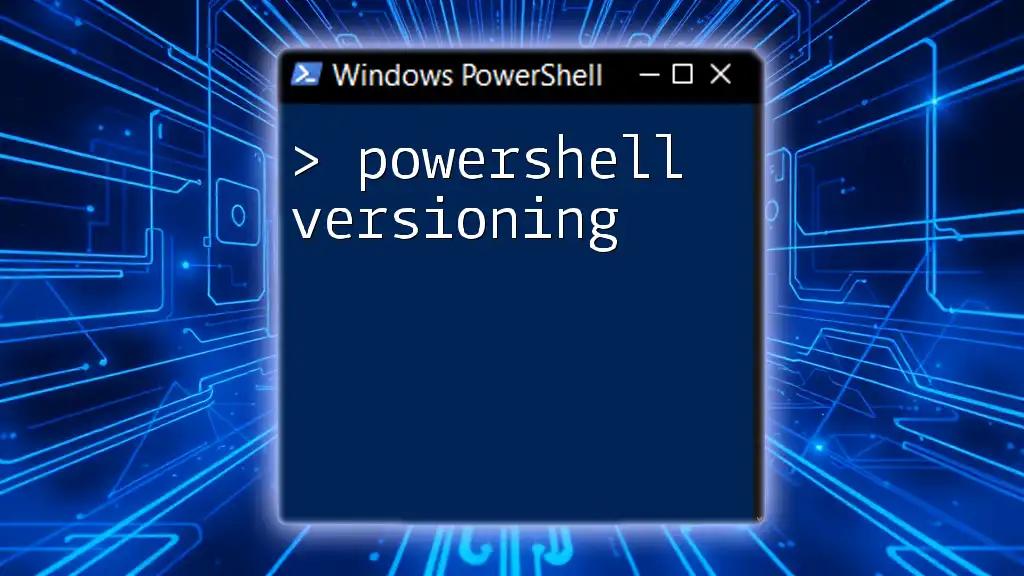
Advanced PowerShell Web Scraping Techniques
Handling Pagination
Websites often paginate their content, and scraping multiple pages requires handling the pagination logic in your code.
Here’s a simple method to scrape multiple pages:
$page = 1
do {
$url = "http://example.com/page/$page"
$response = Invoke-WebRequest -Uri $url
# Process the content here
$page++
} while ($response.StatusCode -eq 200)
In this code, the script keeps incrementing the page number until it receives a status code indicating the page does not exist anymore.
Scraping Data from Tables
If you want to extract structured data from an HTML table, PowerShell makes it straightforward.
Here's an example code snippet to scrape table data:
$tableRows = $response.ParsedHtml.getElementsByTagName("tr")
foreach ($row in $tableRows) {
$cells = $row.getElementsByTagName("td")
# Process cells for desired information
}
Iterating through the rows allows you to collect and manipulate structured data efficiently, enabling you to pull out just the info you need.
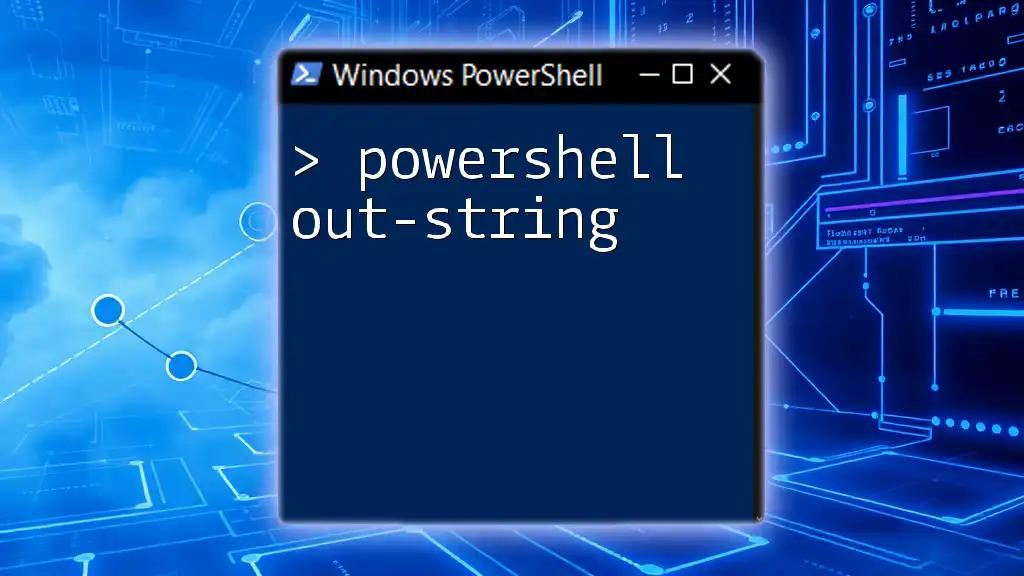
Best Practices for PowerShell Web Scraping
Respecting Website Terms of Service
Before scraping any website, it’s crucial to review their terms of service to ensure compliance. Many sites disallow scraping, and ignoring these guidelines could lead to IP bans or legal consequences.
Managing Request Rates
To avoid overwhelming servers, it's vital to implement rate limiting. Introducing pauses between your requests can also help mimic human behavior and reduce the likelihood of being blocked. A simple example is:
Start-Sleep -Seconds 1
This command makes the script wait for one second before making the next request, thus easing the load on the website’s server.

Troubleshooting Common Issues
Handling Errors and Exceptions
When working with web scraping, errors are bound to occur. Utilizing Try-Catch blocks helps organize your script and manage errors gracefully.
Here's an example:
try {
$response = Invoke-WebRequest -Uri "http://example.com"
} catch {
Write-Error "Error occurred: $_"
}
This structure allows your script to continue running even if a request fails, giving you the chance to troubleshoot.
Dealing with Website Changes
Websites often update their HTML structures, which may break your scraping scripts. To mitigate this, ensure your code is flexible and always test your scripts to validate their functionality after making updates.

Conclusion
PowerShell web scraping is an invaluable skill for anyone looking to harness the power of web data. Whether you're gathering information for analysis or automating repetitive tasks, mastering the concepts outlined in this guide will unlock a new level of efficiency and insight.
For further exploration, consider utilizing community forums and online resources to deepen your understanding and tackle more complex scraping challenges. Happy scraping!