The `powershell n` command is a shorthand notation typically used to run PowerShell scripts or commands in a new PowerShell process, allowing for isolated execution of scripts or functionality.
Here's a code snippet example:
Start-Process powershell -ArgumentList "-NoProfile -Command Write-Host 'Hello, World!'"
Understanding New Line Characters in PowerShell
What is a New Line Character?
The new line character is a special character that signifies the end of one line of text and the beginning of another. In scripting, it is crucial for displaying output that is more readable and organized. The functionality of new lines often affects how users perceive the output of scripts, making it easier to read when properly utilized.
ASCII Representation of New Line
New line characters can be represented using ASCII values. The most common representations are:
- Line Feed (LF): ASCII value 10 (commonly used in Unix/Linux environments).
- Carriage Return + Line Feed (CRLF): ASCII values 13 and 10 (commonly used in Windows environments).
You can visualize their usage in PowerShell with the following code snippet:
# ASCII representation of new line
[char]10 # Line Feed (LF)
[char]13 # Carriage Return (CR)
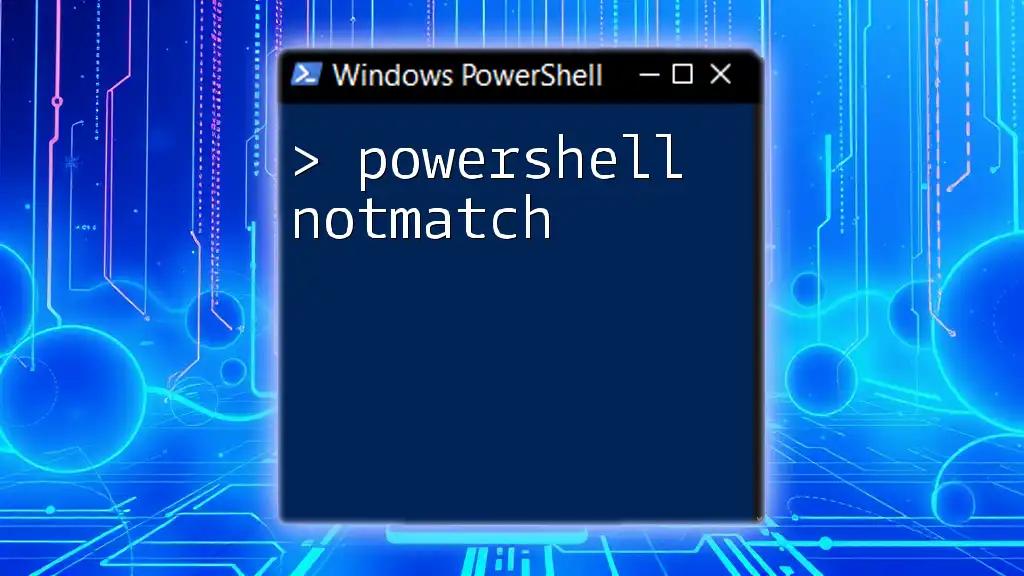
PowerShell String Manipulation Basics
Creating String Variables
In PowerShell, you can create string variables easily. This creates the foundation for manipulating and displaying your strings later in the script. Here's a simple example:
$string = "Hello World"
Important: Remember that strings in PowerShell are immutable. This means that when you modify a string, you are actually creating a new string rather than changing the original.
Joining Strings with New Lines
You can concatenate strings with newline characters to create multi-line outputs efficiently. This is done using the backtick (`), which is the escape character in PowerShell:
$string1 = "Line 1"
$string2 = "Line 2"
$combinedString = "$string1`n$string2"
Write-Output $combinedString
This will display:
Line 1
Line 2
By using `n`, you instruct PowerShell to insert a new line between the two strings.
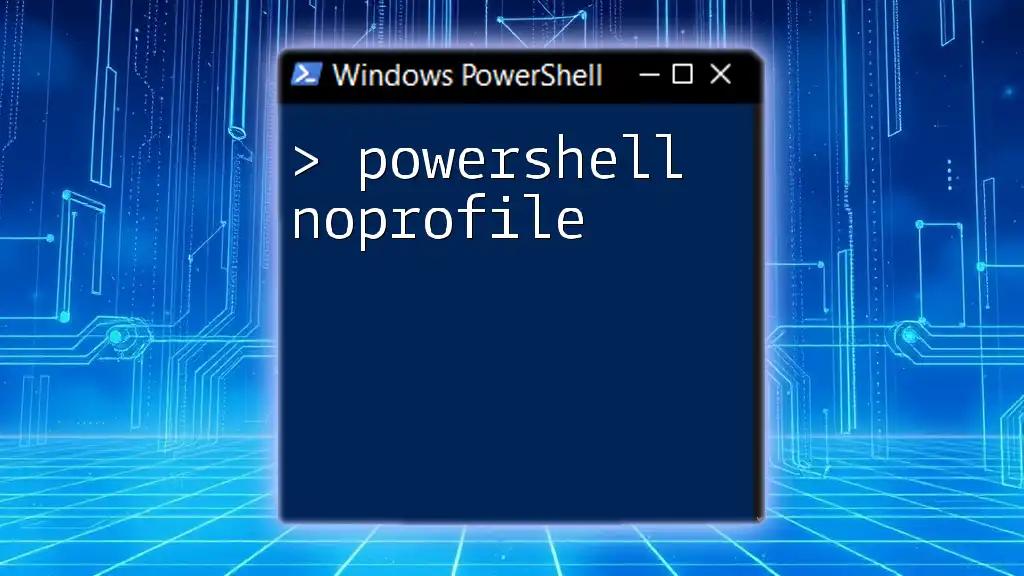
Methods to Add New Lines in PowerShell
Using Backtick `n`
One of the simplest ways to add a new line in PowerShell is utilizing the backtick (`) followed by `n`. This escape character tells PowerShell to interpret the following character as a special instruction. For instance:
$message = "Hello`nWorld"
Write-Host $message
This will print:
Hello
World
Tip: The backtick is a unique character in PowerShell. Ensure it is used correctly to avoid unintended output.
Using `System.Environment` Class
Another effective way to introduce new line characters is by leveraging the `System.Environment]::NewLine`. This method provides a platform-agnostic way to handle newline characters, making your scripts more portable. Here’s how you can use it:
$message = "Hello" + [System.Environment]::NewLine + "World"
Write-Host $message
This will produce the same output:
Hello
World
Pros: Using `System.Environment` is beneficial when scripts are destined to run on different platforms, ensuring compatibility.
Using String Formatting
You can also create formatted strings that include new lines. Using the `-f` operator along with the `NewLine` property provides a clear and organized way to format your text:
$formattedString = "This is line 1{0}This is line 2" -f [Environment]::NewLine
Write-Output $formattedString
This method allows for dynamic string creation, making maintenance easier as your scripts grow.
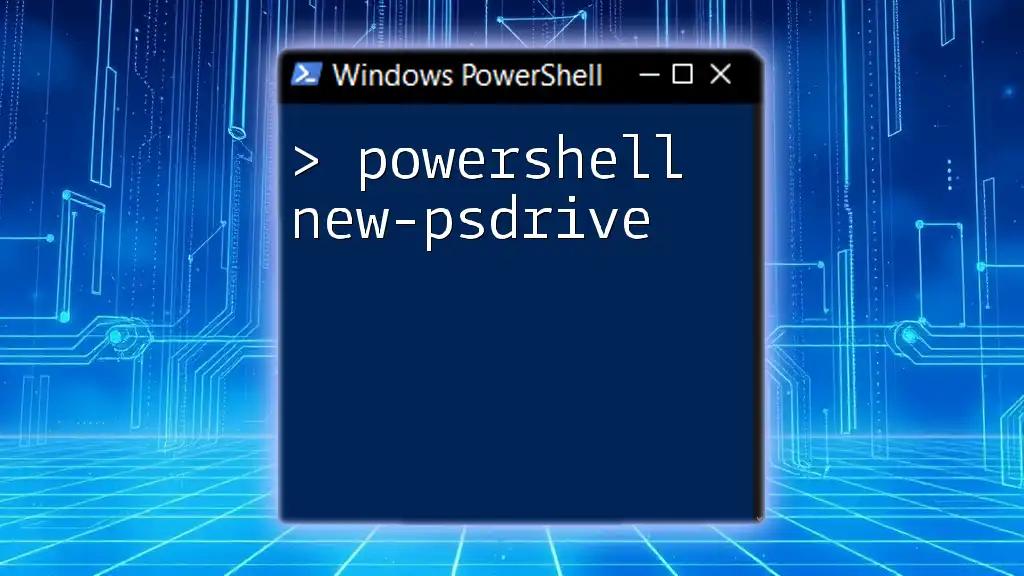
Common Use Cases for New Lines in Scripts
Creating Multi-line Output
When scripting, producing output that is easy to read can significantly enhance user experience. Using new lines effectively can help break down complex messages into digestible parts. For example:
Write-Output "Task 1 complete`nTask 2 complete`nTask 3 complete"
This concise output would clearly show the completion of multiple tasks line by line, enhancing readability.
Logging with Time Stamps
When creating logs in PowerShell, incorporating new lines can make records much clearer. Here’s an example of logging tasks with start and end timestamps:
$logs = "Start Time: $(Get-Date)`nTask running...`nEnd Time: $(Get-Date)"
Write-Output $logs
This approach provides a straightforward view of task execution and timing, making analysis easier.

Troubleshooting Common Issues with New Lines
New Lines Not Appearing
At times, you may find that new lines do not render properly in outputs. Common reasons include:
- Incorrect usage of escape characters.
- Pasting scripts into environments that do not interpret certain characters correctly.
Working Across Different Platforms
When working in multi-platform environments, be aware of differences in newline representations. Windows typically uses CRLF, while Unix systems use LF. You can address this by:
- Normalizing your strings using this command to convert new line characters:
$normalizedString = $combinedString -replace "`r`n", "`n" # Converting CRLF to LF
This ensures your scripts run correctly across any system.
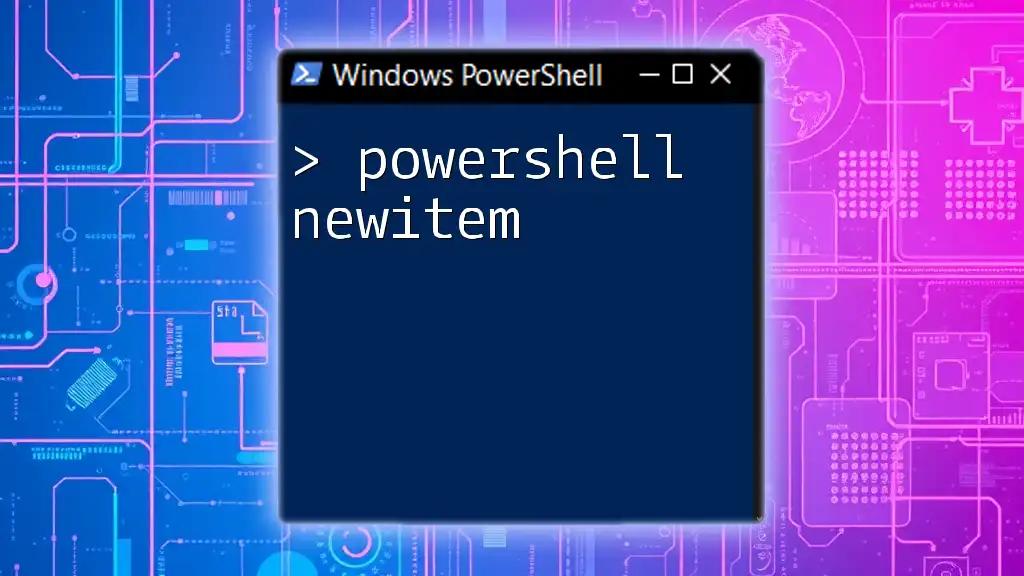
Conclusion
Understanding how to manipulate strings with newlines in PowerShell is essential for creating organized and user-friendly scripts. The versatility of methods like using the backtick, the `System.Environment` class, and string formatting empowers you to create clear, readable outputs easily.
By practicing these techniques and troubleshooting tips, you can enhance the efficiency of your PowerShell scripts and improve the overall readability of your outputs. Continuously experimenting will lead you to better methodologies in your PowerShell scripting journey.

Call to Action
We invite you to subscribe for more PowerShell tips to elevate your scripting skills. Share your experiences with PowerShell string manipulation in the comments below!
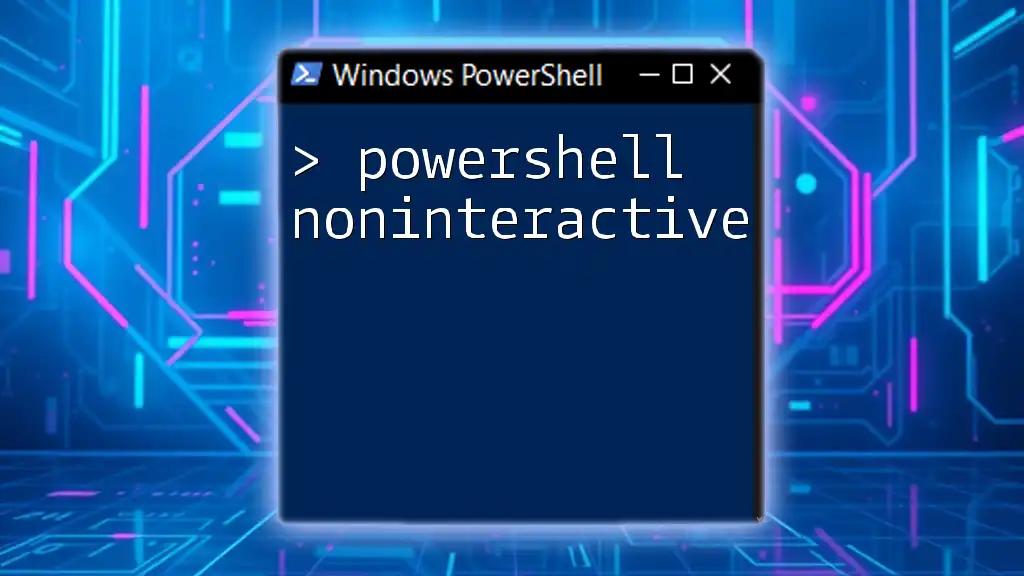
Additional Resources
For further learning, check out related articles in our series or consider investing in books and online courses focused on advanced PowerShell techniques. Such resources can enrich your knowledge and skills significantly.