The `irm` (Invoke-RestMethod) cmdlet in PowerShell is used to send HTTP requests to RESTful APIs and retrieve the response, typically in a simplified format like JSON or XML.
Here’s a code snippet demonstrating its usage:
irm https://api.example.com/data | ConvertFrom-Json
What is PowerShell IRM?
PowerShell IRM, or Invoke-RestMethod, is a powerful cmdlet that allows users to interact with RESTful web services. It simplifies the process of making HTTP requests and processing the responses by automatically converting JSON or XML formatted data into PowerShell objects. This functionality is crucial for developers, system administrators, and IT professionals who need to retrieve or send data via APIs.
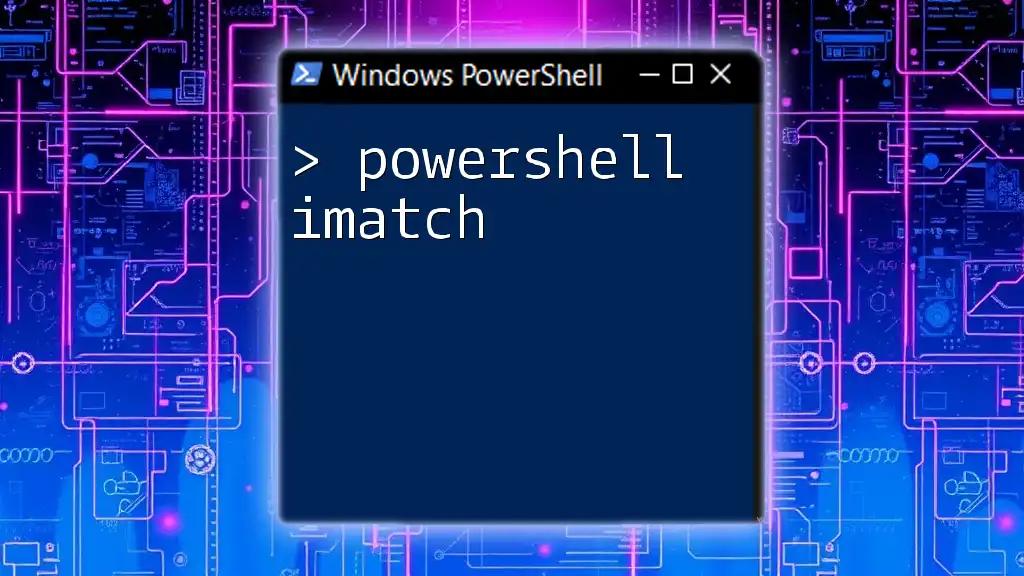
Why Use PowerShell IRM?
The advantages of utilizing PowerShell IRM include:
- Efficiency: With IRM, making API calls becomes a seamless task, reducing the complexity of parsing data formats manually.
- Simplicity: The intuitive syntax of IRM makes it easy for those familiar with PowerShell, reducing the learning curve for new users.
- Versatility: PowerShell IRM can handle both GET and POST requests, making it suitable for a wide range of applications.
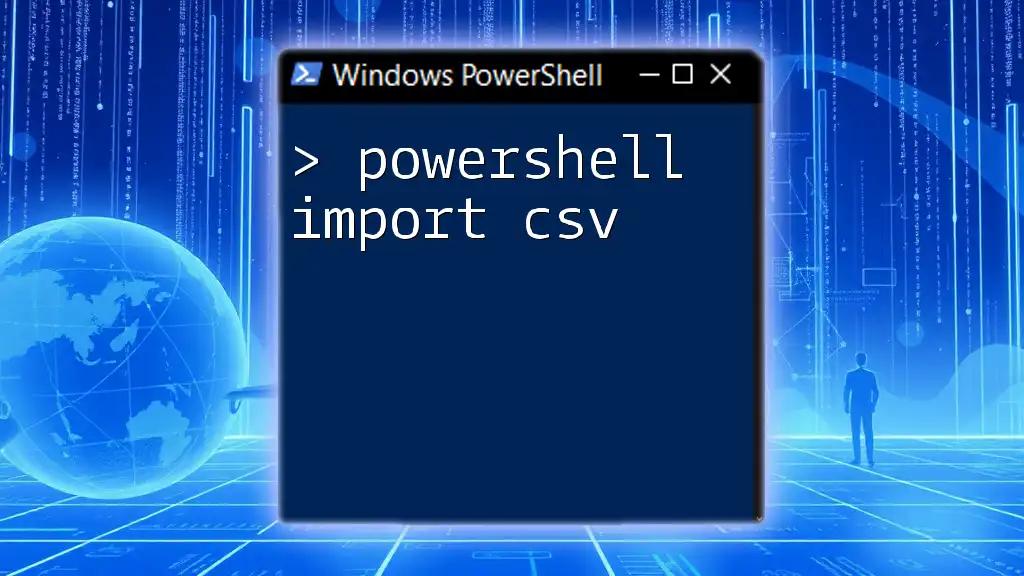
Getting Started with IRM
Setting Up Your PowerShell Environment
Before using PowerShell IRM, ensure that your PowerShell environment is properly configured. This applies whether you're using Windows, Linux, or macOS.
To check your PowerShell version, you can use the following command:
$PSVersionTable.PSVersion
Syntax of Invoke-RestMethod
The basic structure of the `Invoke-RestMethod` command is as follows:
Invoke-RestMethod -Uri <uri> -Method <HTTP method>
For instance, if you want to make a simple GET request to a public API, you can use this command:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
$response
The `$response` variable will contain the data returned by the API, automatically converted into a PowerShell object.
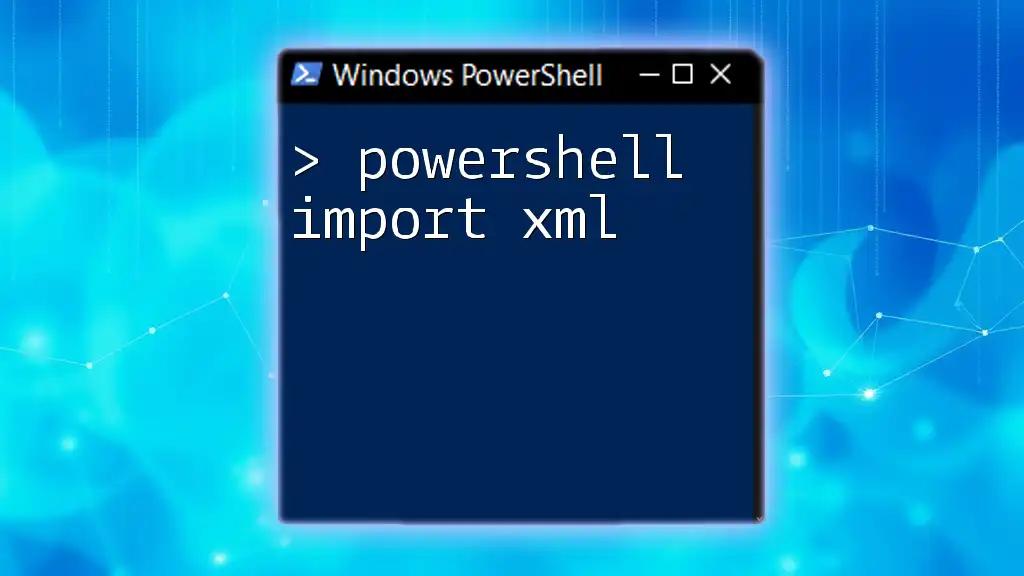
Common Scenarios for Using IRM
Retrieving Data from APIs
The primary use case for PowerShell IRM is retrieving data from RESTful APIs. The IRM cmdlet simplifies this process, allowing you to focus on utilizing the data rather than the intricacies of the HTTP protocol.
Consider the following example, which fetches JSON data from an API:
$jsonData = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
$jsonData
In this example, the response from the API will be parsed into a PowerShell object, making it easy to manipulate the data.
Sending Data to APIs
PowerShell IRM is not limited to just fetching data; it also enables sending data to APIs. You can send various data types, including JSON and XML, depending on the API's requirements.
Here’s an example of how to send a POST request with a JSON body:
$body = @{ name = "John"; age = 30 }
$response = Invoke-RestMethod -Uri "https://api.example.com/create" -Method Post -Body ($body | ConvertTo-Json)
$response
This command sends a JSON object to the specified endpoint and returns the API's response.
Error Handling with IRM
When making HTTP requests, errors can occur due to various reasons, such as network issues or API malfunctions. To effectively handle these scenarios, incorporating error handling is essential.
Using Try-Catch blocks can help you manage errors gracefully. For example:
try {
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
$response
} catch {
Write-Host "An error occurred: $_"
}
This approach ensures your script will not fail abruptly, providing a controlled way to address issues.
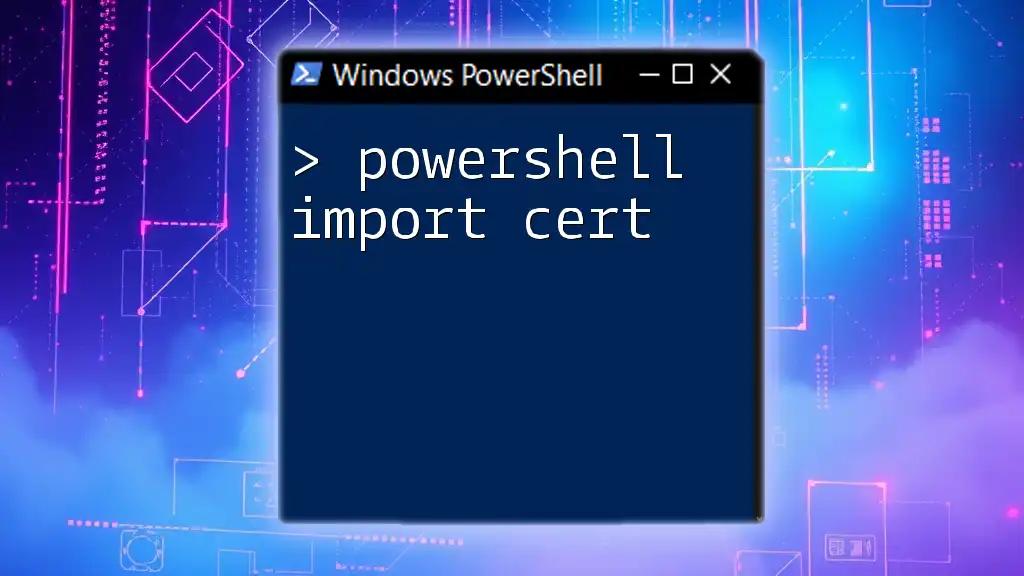
Advanced Use Cases of PowerShell IRM
Custom Headers and Authentication
Many APIs require additional security measures, such as authentication tokens or custom headers. PowerShell IRM allows you to include these headers effortlessly.
For example, you might need to include a Bearer token for authentication:
$headers = @{
"Authorization" = "Bearer YOUR_ACCESS_TOKEN"
}
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get -Headers $headers
$response
This command includes the specified headers in the HTTP request, enabling secure access to protected resources.
Working with Different Content Types
PowerShell IRM is versatile in handling various content types. While JSON is commonly used, some applications require XML.
Here’s how you can make a request to fetch XML data:
$xmlData = Invoke-RestMethod -Uri "https://api.example.com/data.xml" -Method Get
$xmlData
This command retrieves XML data and parses it into a PowerShell object, allowing for easier manipulation.
Using IRM with PowerShell Objects
The beauty of PowerShell IRM is its ability to convert API responses into native PowerShell objects. This functionality streamlines data processing.
Consider the following example, which parses and processes API data:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
$response | ForEach-Object { $_.name }
This command iterates through the returned objects, extracting the `name` property from each.
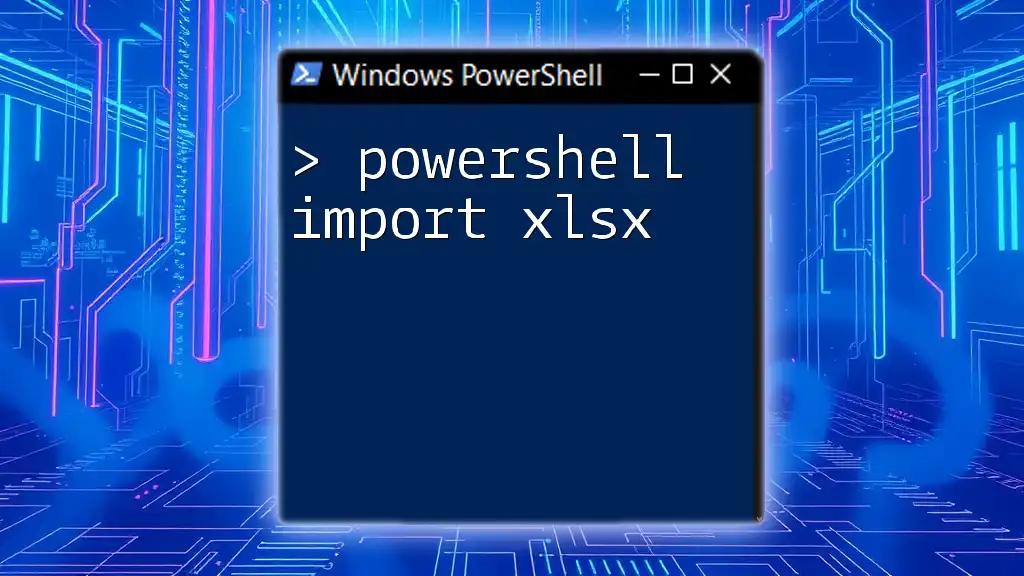
Best Practices for Using PowerShell IRM
Securing API Calls
Security is paramount when working with APIs. Always ensure your API calls are secure by using HTTPS and implementing proper authentication measures.
Consider utilizing API keys or OAuth tokens as needed to safeguard your requests.
Performance Considerations
Optimizing your IRM calls can reduce latency and improve the efficiency of your scripts. Consider minimizing the frequency of requests and avoiding unnecessary data retrieval.
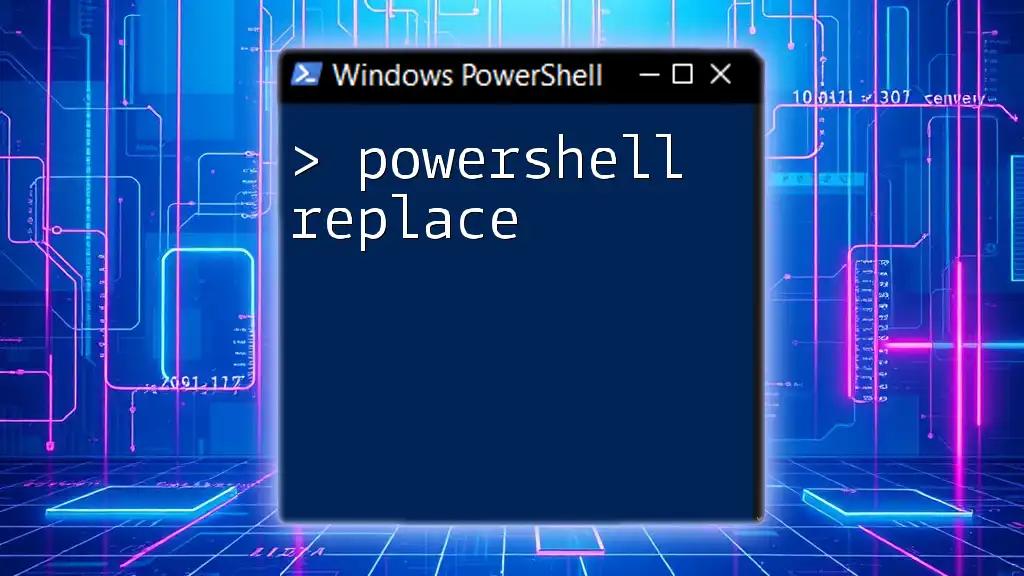
Troubleshooting Common Issues with PowerShell IRM
Common Errors and Solutions
When using PowerShell IRM, you may encounter common errors, such as:
- Network errors: Ensure your network connection is stable.
- Authorization errors: Double-check your API keys or access tokens.
Testing Your IRM Commands
Before implementing IRM commands into larger scripts, it's a good practice to test them with tools like Postman or Curl. This ensures your requests function as expected before automating them in PowerShell.
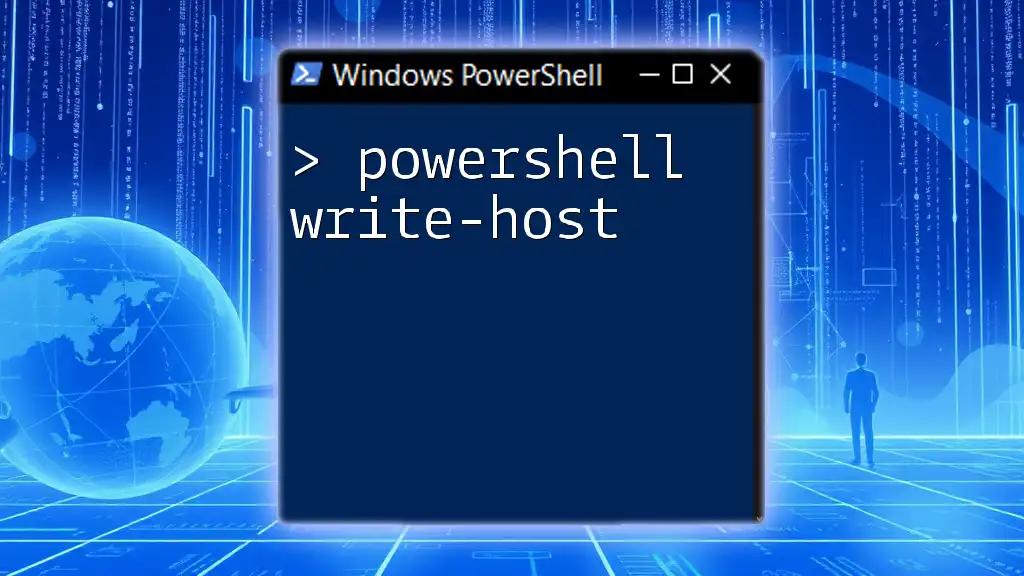
Conclusion
PowerShell IRM is a powerful tool that enhances the ability to interact with web APIs, making data retrieval and submission straightforward. By understanding its features and applying best practices, you can efficiently leverage IRM in your PowerShell environment.
Encouragement to Practice
Don't hesitate to experiment with various APIs using PowerShell IRM. Hands-on practice is key to mastering this essential tool, allowing you to streamline workflows and enhance your scripting capabilities.
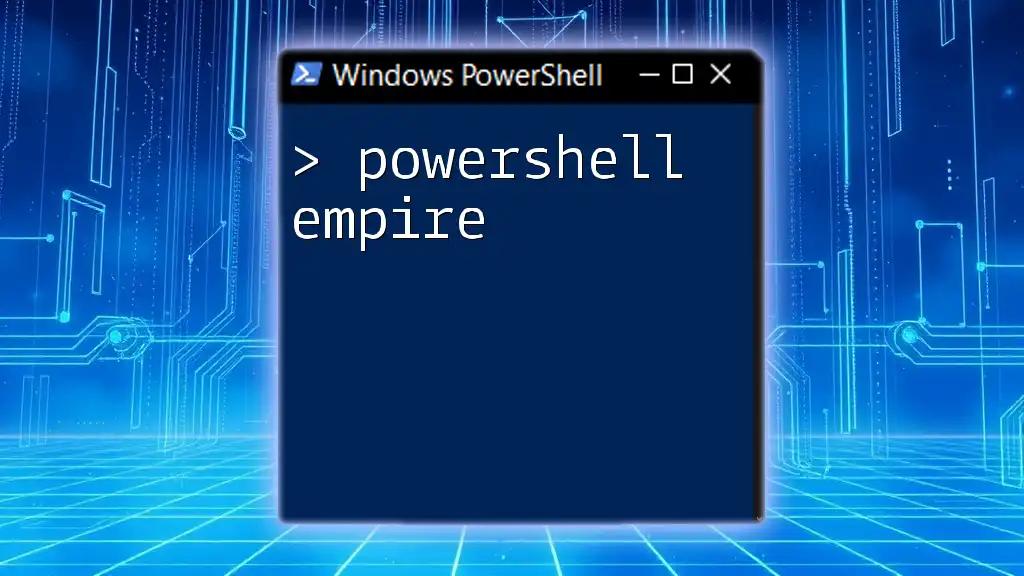
Additional Resources
Consult the official Microsoft documentation for further information on PowerShell IRM and explore additional articles and tutorials to strengthen your understanding.