To encode a file in Base64 using PowerShell, you can utilize the `Get-Content` cmdlet along with `[Convert]::ToBase64String()` within a script.
$bytes = [System.IO.File]::ReadAllBytes('C:\path\to\your\file.txt'); [Convert]::ToBase64String($bytes) | Out-File 'C:\path\to\your\encodedfile.txt'
Understanding Base64 Encoding
What is Base64 Encoding?
Base64 encoding is a method of converting binary data into a text format using 64 different ASCII characters. This is useful because many data transmission protocols can only handle text data, making Base64 encoding essential when transmitting files over such mediums. Essentially, it converts the binary representation of a file into a string that can be easily read and processed by various systems.
Benefits of Using Base64 Encoding
- Data Integrity: When transferring files, encoding them reduces the likelihood of data corruption. Base64 ensures that the data remains untouched during transmission.
- Compatibility: Some systems may not support certain file types or characters. Base64 encoding allows the transfer of these files by avoiding issues related to incompatible characters.
- Embedding Files: Base64 encoding is also used for embedding images or files directly into HTML, CSS, or JSON, making it easier to include resources directly within code.
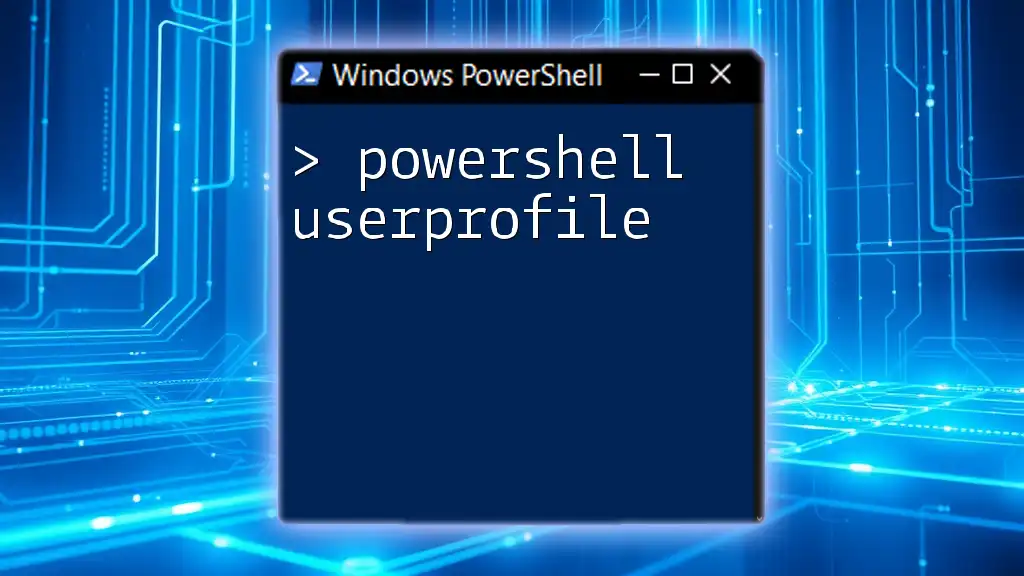
Preparing Your Environment
Installing PowerShell
To encode files using PowerShell, you need to ensure that it is installed on your machine. PowerShell comes pre-installed on Windows but can also be installed on macOS and Linux. Make sure you are using the latest version of PowerShell to access all the features and functionalities.
Opening PowerShell
To start PowerShell, you can use several methods:
- Windows Terminal: Open the Windows Terminal and choose PowerShell from the dropdown menu.
- Run Dialog: Press `Win + R`, type `powershell`, and hit Enter.
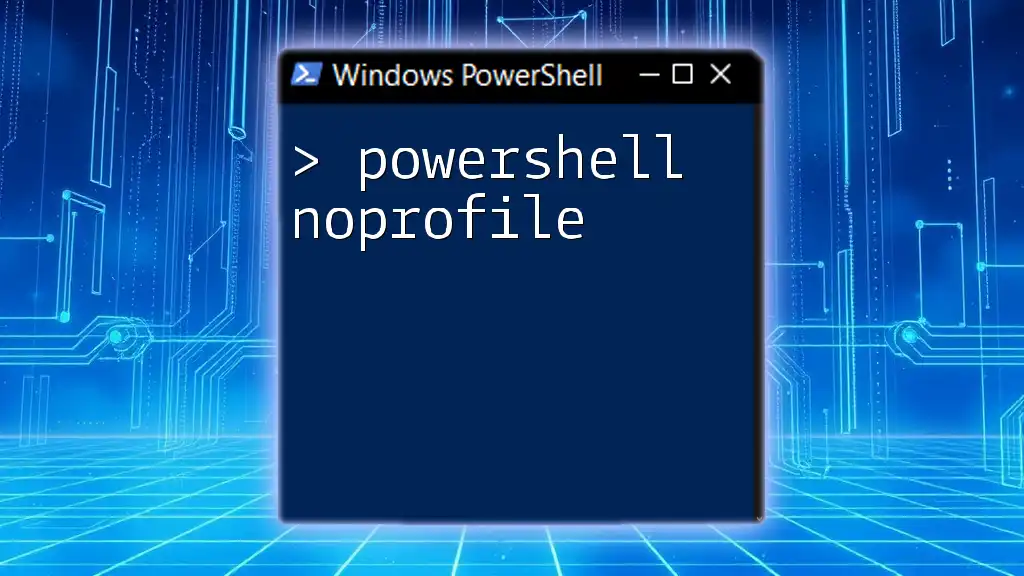
Base64 Encoding a File in PowerShell
Using Get-Content and [Convert]::ToBase64String
Encoding a file in PowerShell can be accomplished easily with the combination of two commands: `Get-Content` to read the file and `[Convert]::ToBase64String` to perform the encoding. Here’s how it is done:
$filePath = 'C:\path\to\your\file.txt'
$fileBytes = [System.IO.File]::ReadAllBytes($filePath)
$base64Encoded = [Convert]::ToBase64String($fileBytes)
In this code:
- $filePath specifies the location of the file you wish to encode.
- [System.IO.File]::ReadAllBytes($filePath) reads the file as binary data.
- [Convert]::ToBase64String($fileBytes) converts the byte array into a Base64 encoded string.
Saving the Base64 Encoded Output to a File
To save the Base64 encoded string into a new file, you can use the `WriteAllText` method, which allows you to write the content directly to a specified file path.
[System.IO.File]::WriteAllText('C:\path\to\output.txt', $base64Encoded)
Here, you direct the encoded string to a new file, allowing for easy retrieval and sharing.
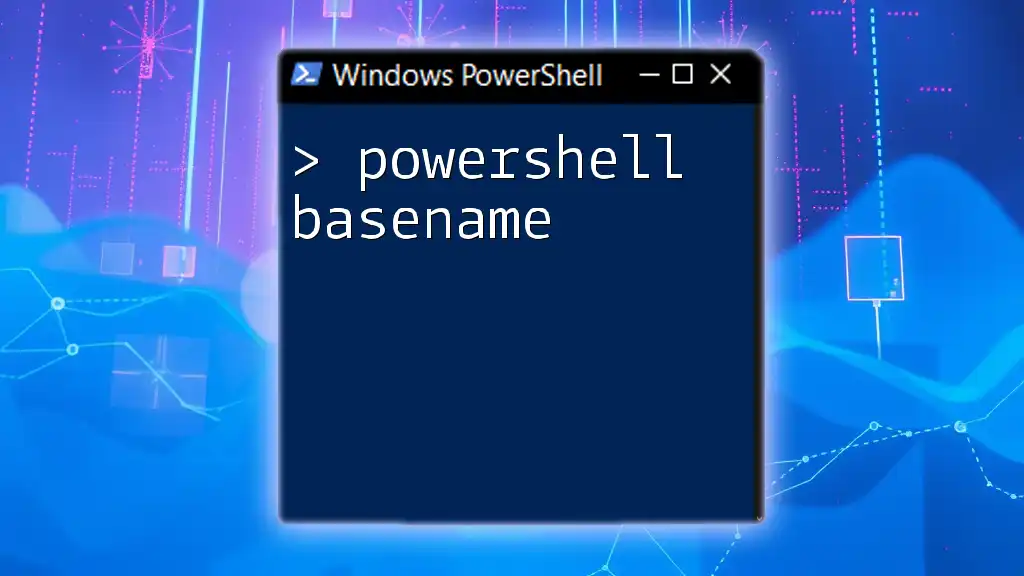
Base64 Decoding in PowerShell
Understanding the Need for Decoding
There may come a time when what you've encoded needs to be accessed in its original format. Base64 decoding is equally essential, particularly for scenarios where you need to recover data or extract binaries from encoded strings.
Using [Convert]::FromBase64String
Decoding a Base64 encoded file is just as simple as encoding it. You use the `[Convert]::FromBase64String` method to convert the encoded string back into its original byte format. Here’s a concise example:
$base64Content = Get-Content 'C:\path\to\output.txt'
$decodedBytes = [Convert]::FromBase64String($base64Content)
[System.IO.File]::WriteAllBytes('C:\path\to\decodedFile.txt', $decodedBytes)
In this code:
- Get-Content retrieves the Base64 encoded string from your file.
- [Convert]::FromBase64String($base64Content) decodes the Base64 string back into a byte array.
- [System.IO.File]::WriteAllBytes writes the byte array back to a new file, restoring it to its original format.
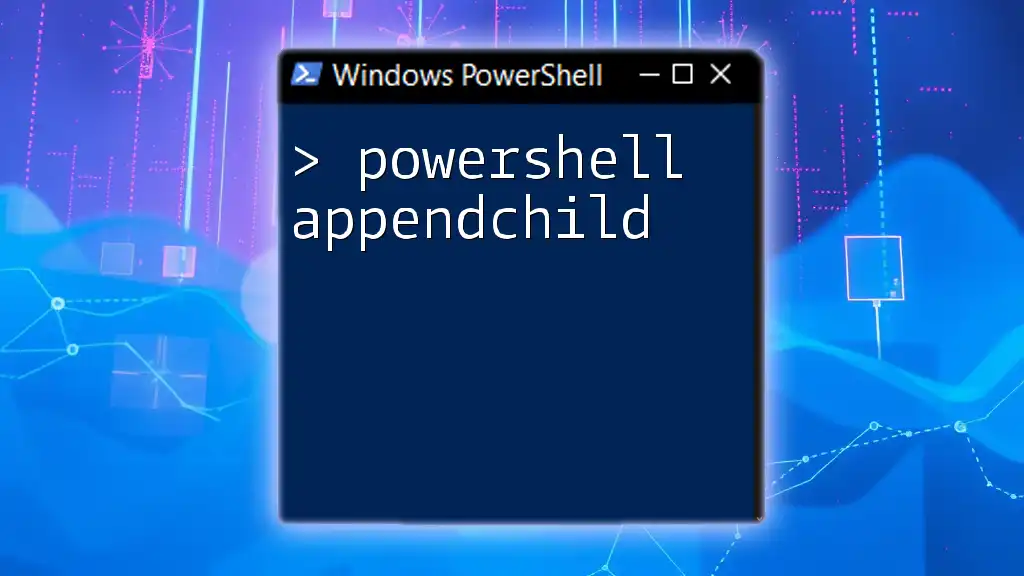
Common Use Cases for Base64 Encoding in PowerShell
Encoding Images
Base64 encoding is often used for images, especially in web development and email applications. It allows images to be included directly in HTML or CSS files, reducing the need for separate file requests. This can enhance loading times and streamline file management.
Embedding Configuration Files
Another common use of Base64 encoding is for embedding configuration files or scripts in deployment processes, allowing for complete file integrity during transfers.
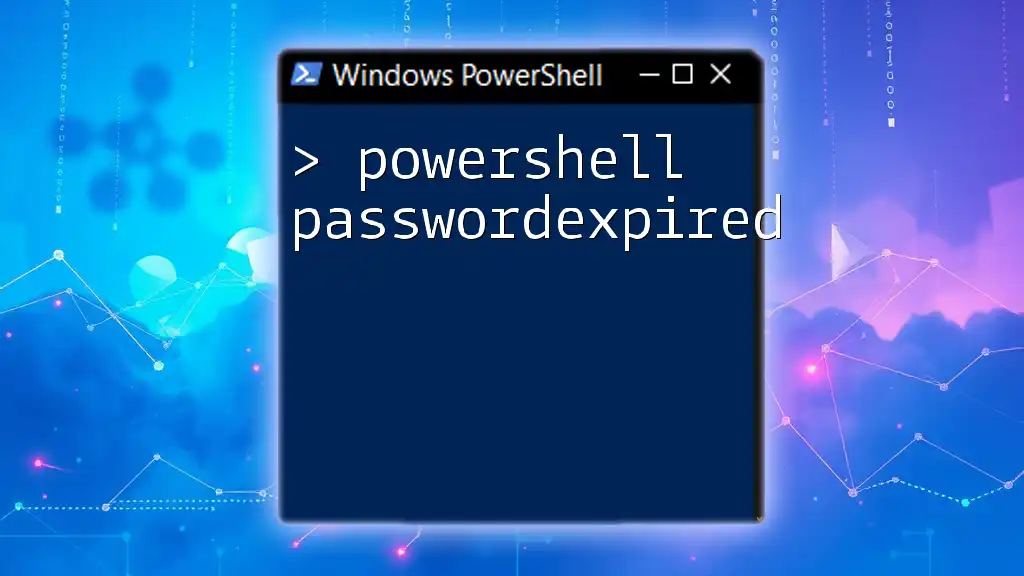
Troubleshooting Common Issues
Errors and Warnings
When working with file encoding and decoding in PowerShell, you may encounter several common issues:
- File Not Found: Ensure the file path is correct.
- Invalid Base64 String: Check the format of your encoded string for any discrepancies.
Tips for Successful Encoding
- Always ensure that you are working with paths that are accessible.
- When encoding large files, be mindful of memory limits and performance depending on your system’s capabilities.
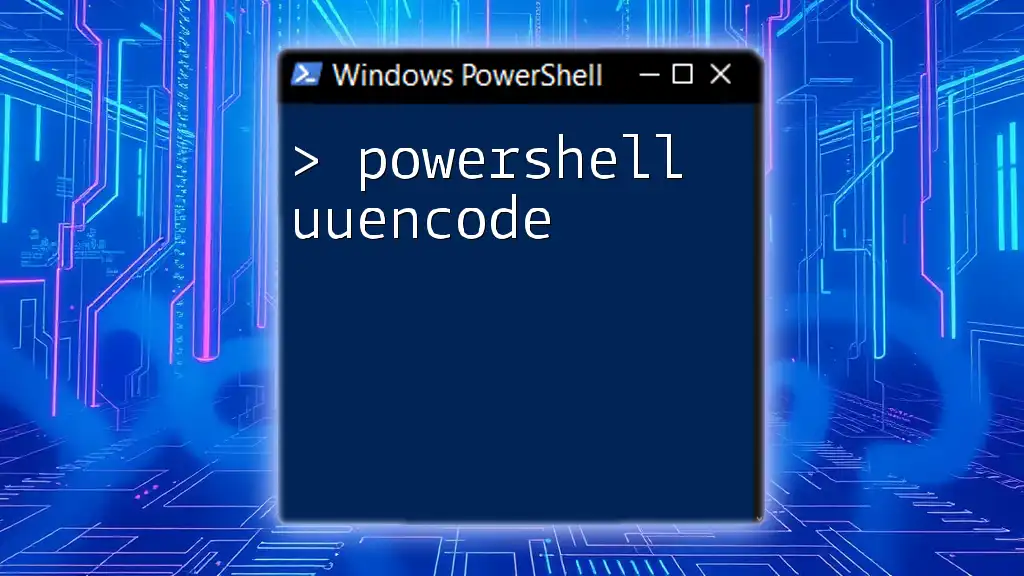
Conclusion
PowerShell provides a powerful and straightforward way to encode files using Base64 encoding. This not only enhances data integrity but also facilitates better compatibility across systems. Whether you are looking to encode simple text files or more complex data types like images and configuration files, mastering PowerShell Base64 encode file techniques can significantly benefit your data management processes.
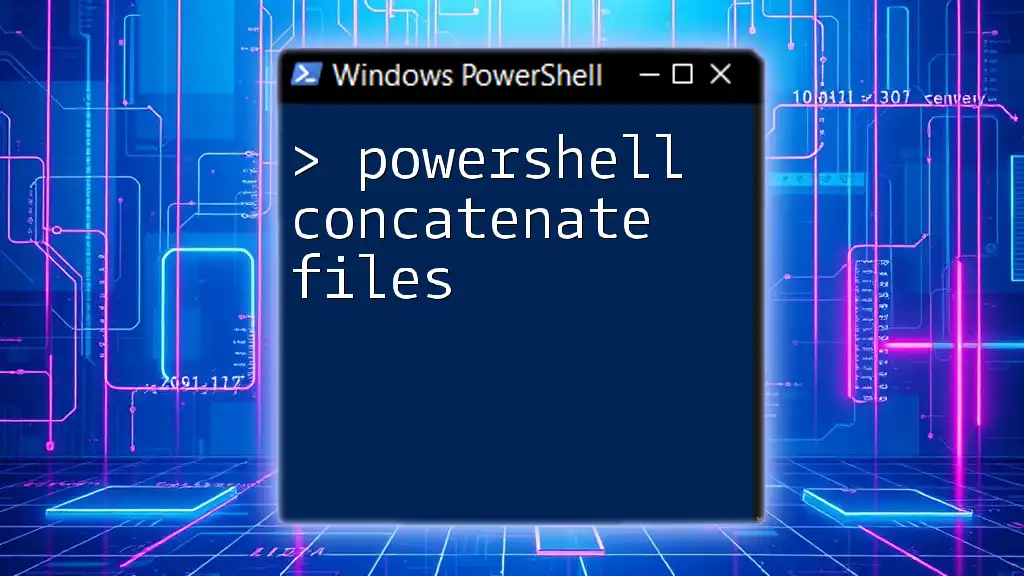
Additional Resources
For further reading, refer to the official PowerShell documentation and other online resources that delve deeper into Base64 encoding and PowerShell functionalities.