PowerShell does not natively use `awk`, as it's primarily a Unix/Linux command-line tool, but you can achieve similar text processing tasks in PowerShell using the `Select-String` or `ForEach-Object` cmdlets.
Here’s a simple example that mimics `awk` by filtering lines containing "error" from a log file:
Get-Content 'logfile.txt' | Where-Object { $_ -match 'error' } | ForEach-Object { $_.Split(' ')[0] }
What is PowerShell?
PowerShell is a powerful scripting and automation framework created by Microsoft. It provides a command-line shell and an associated scripting language designed specifically for system administration. PowerShell enables users to automate tasks and control system settings through commands known as cmdlets. With its ability to access COM and WMI, it provides an efficient way for administrators to manage hardware and software without requiring extensive programming knowledge.
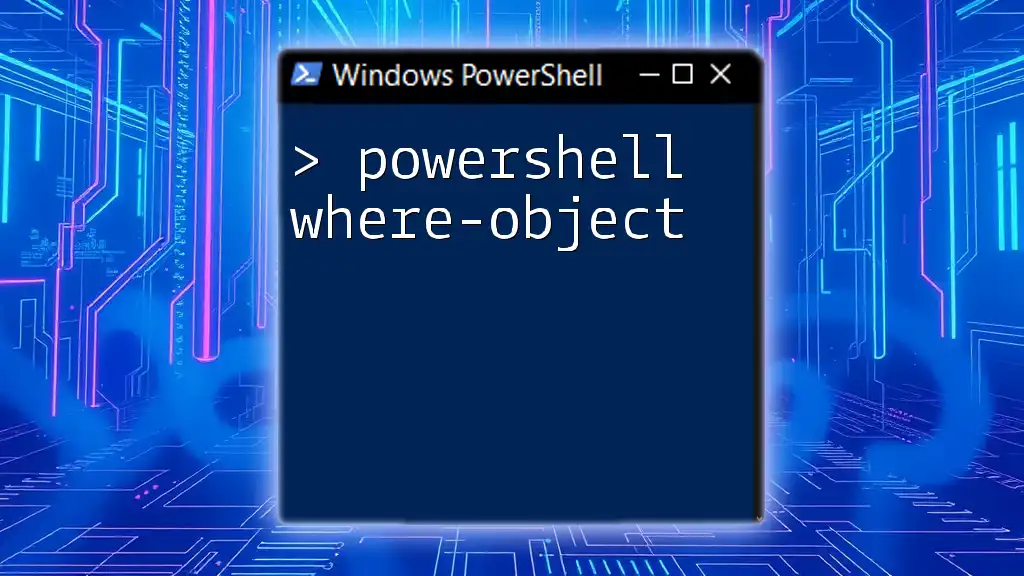
What is AWK?
AWK is a versatile programming language primarily used for pattern scanning and processing. Named after its authors—Alfred Aho, Peter Weinberger, and Brian Kernighan—AWK excels in processing text files and is often utilized for reporting and data extraction tasks. Typical use cases include parsing logs, generating reports, and extracting specific data fields from structured text files, such as CSVs.
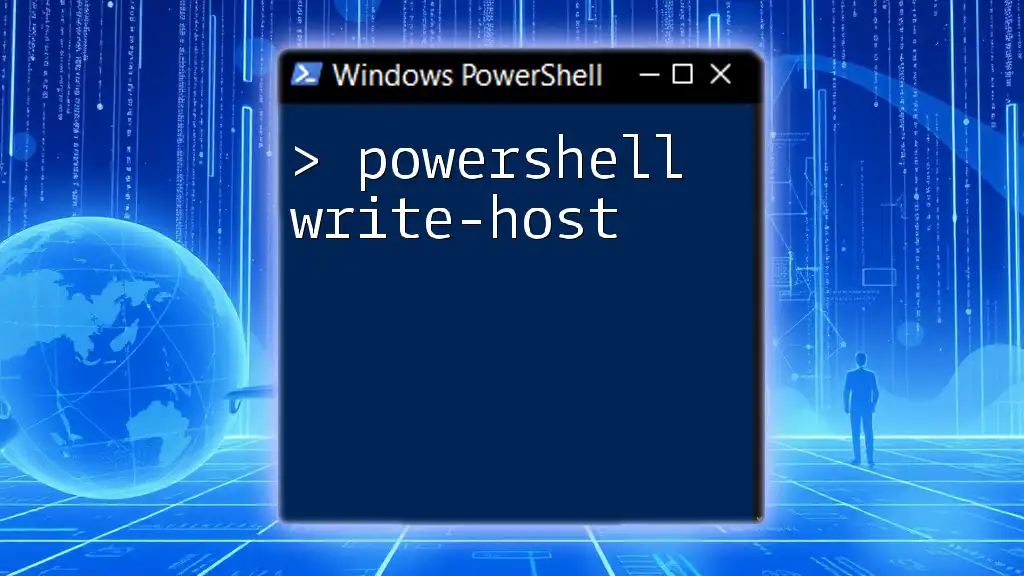
PowerShell vs. AWK: Understanding the Differences
While both PowerShell and AWK serve similar purposes for text and data manipulation, they differ significantly in syntax and functionality.
- Syntax: PowerShell utilizes cmdlets, which are objects, enhancing readability and interaction with the Windows ecosystem. Conversely, AWK employs a concise programming syntax that focuses on line-by-line processing of text.
- Functionality: PowerShell is designed for system administration, providing deep integration with Windows, whereas AWK is purely for text manipulation and pattern matching.
Both tools have their strengths, and choosing between them often depends on the task at hand.
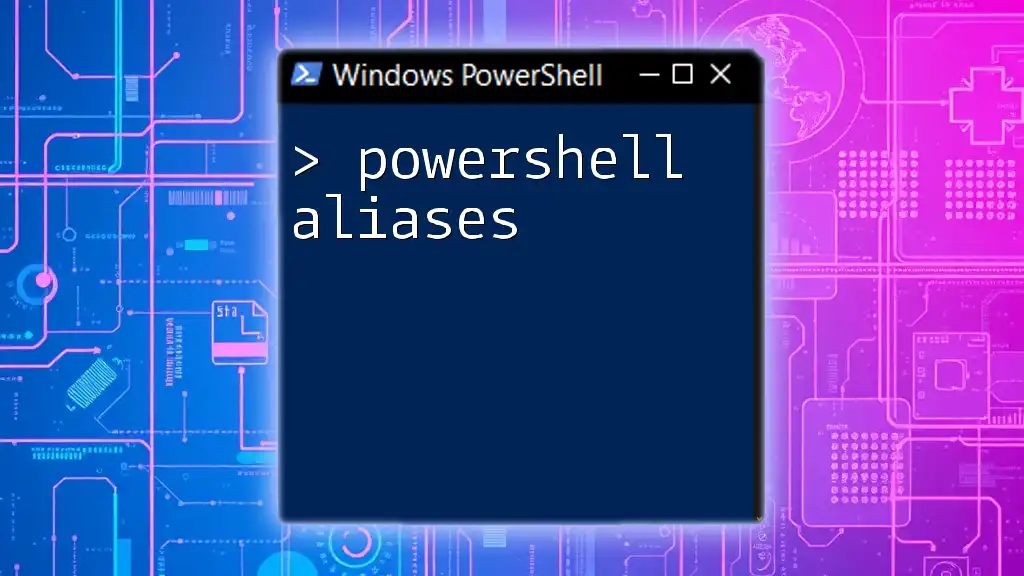
Getting Started with PowerShell AWK
How to Invoke AWK in PowerShell
Using AWK within PowerShell is straightforward. You can invoke AWK commands through the pipeline, allowing seamless integration with PowerShell's robust features. For example, to print the first field of a text file, you can utilize the following command:
Get-Content 'file.txt' | awk '{print $1}'
This command reads the contents of 'file.txt' and passes it to AWK, which processes it line by line.
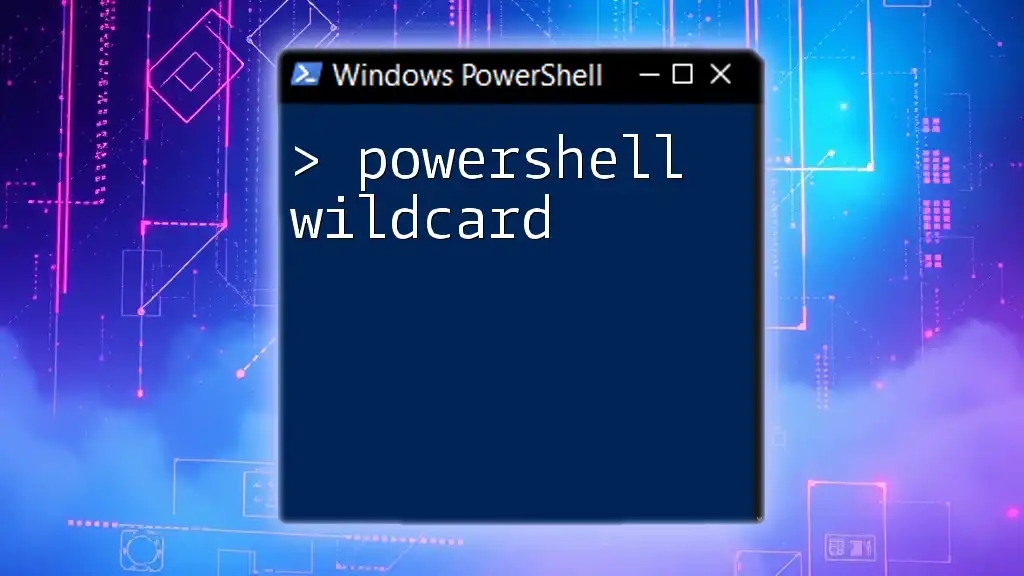
Basic AWK Commands in PowerShell
Understanding Fields and Records
In AWK, a record is typically a line of text, while a field is a component separated by whitespace or a specified delimiter. For example, in a CSV file, each line represents a record, and the individual data points are fields.
Using AWK for Simple Tasks
One of the most common tasks is extracting specific columns from a structured file. Using AWK to print the second column of a CSV can be achieved easily:
Get-Content 'data.csv' | awk -F, '{ print $2 }'
In this command, `-F,` indicates that the fields are separated by commas, allowing AWK to easily identify and extract the desired column.
Defining and Using Patterns
AWK's pattern matching capability is one of its most powerful features. You can filter for particular lines in your text file. For instance, if you want to filter for lines containing the word "error":
Get-Content 'data.txt' | awk '/error/ { print }'
This command will only print lines that match the specified pattern, making it an essential tool for log analysis.
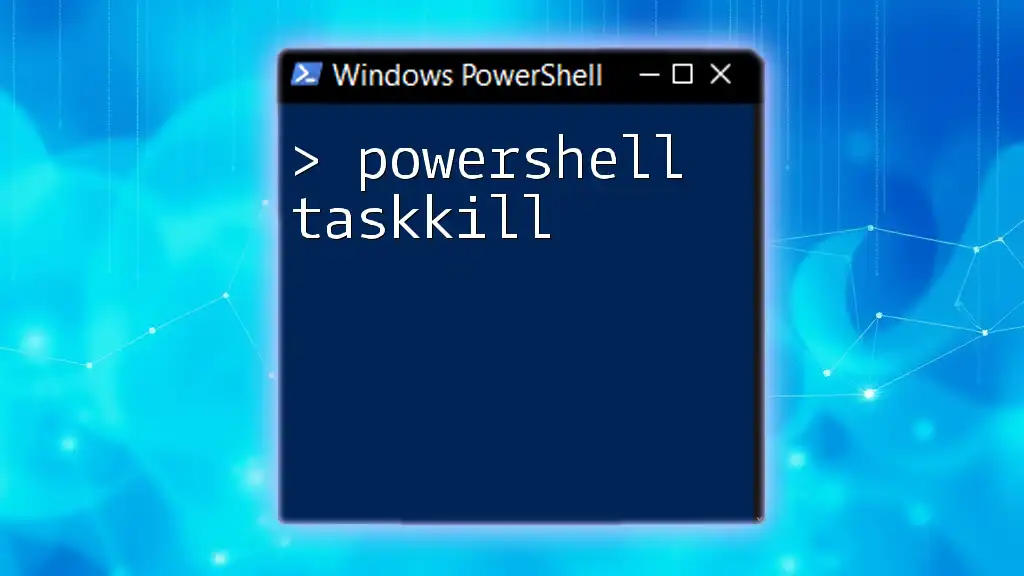
Advanced AWK Operations
Implementing AWK Functions
AWK supports function definitions, allowing you to encapsulate logic for code reuse. For example, if you want to create a function that formats output, you can do it as shown below:
Get-Content 'file.txt' | awk 'function formatOutput(x) { return toupper(x) } { print formatOutput($1) }'
This snippet converts the first field of each line to uppercase using the defined `formatOutput` function.
Using Control Structures
Control structures, such as `if`, `for`, and `while`, enable more complex data processing within your AWK commands. For example, you can use an `if` statement to only print values greater than a certain number:
Get-Content 'file.txt' | awk '{ if ($1 > 10) print $1 }'
This command checks if the first field is greater than 10 and prints it if the condition is true.
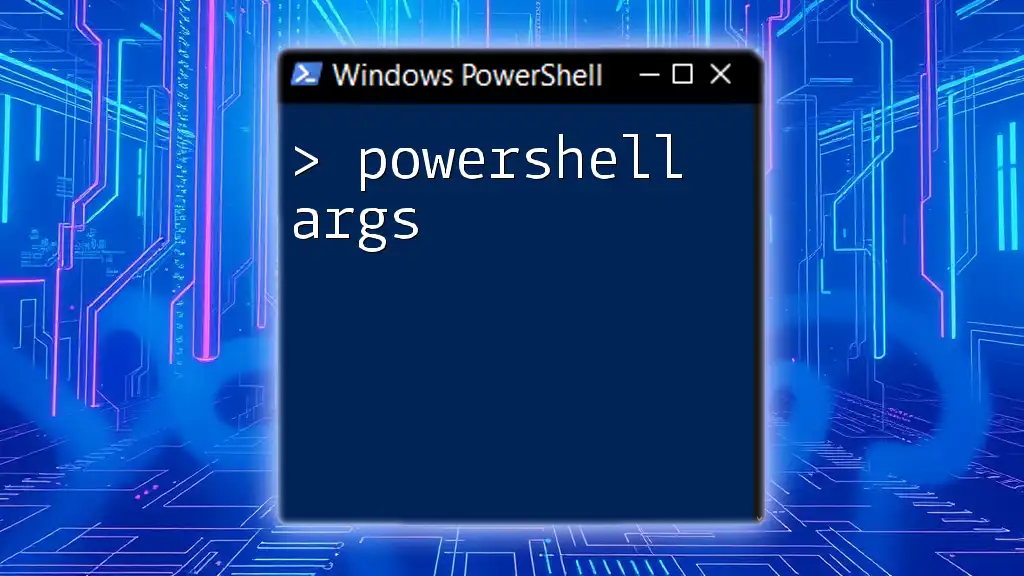
Combining PowerShell and AWK
Using AWK with Other PowerShell Cmdlets
One of the strengths of PowerShell is its ability to combine cmdlets for enhanced functionality. You can utilize AWK alongside cmdlets like `Where-Object` to apply filters before processing text with AWK.
Get-Content 'data.csv' | Where-Object { $_ -match 'active' } | awk -F, '{ print $1 }'
In this example, the command first filters lines containing the word "active" and then extracts the first column from those filtered results.
Exporting AWK Outputs to PowerShell Variables
Sometimes, it may be necessary to capture the output of AWK for further processing within PowerShell. You can store AWK results in a variable as shown:
$results = Get-Content 'file.txt' | awk '{print $1}'
This command allows you to manipulate the stored results using PowerShell's wide array of functionalities.
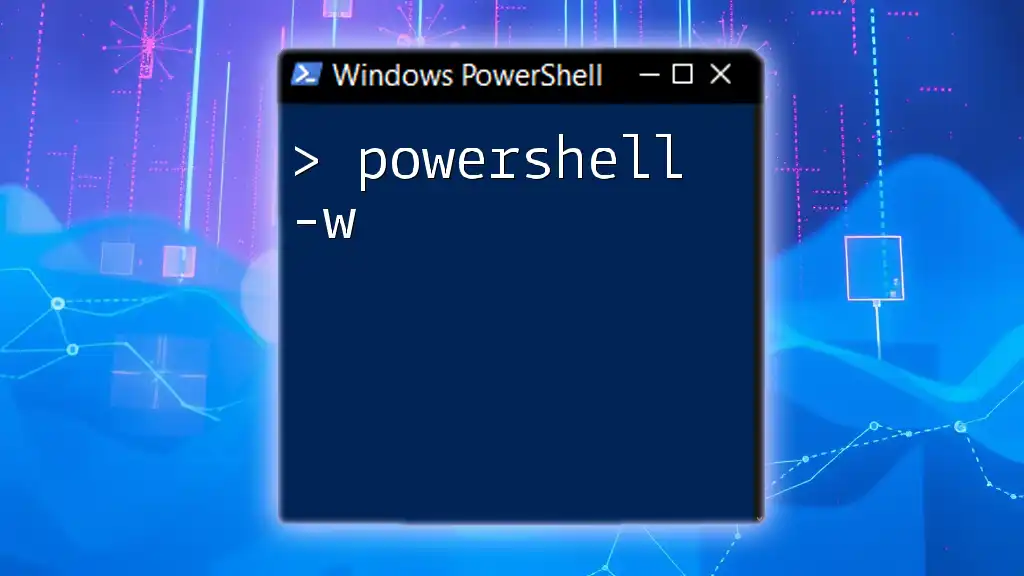
Best Practices for Using AWK in PowerShell
Performance Considerations
When working with large datasets, performance becomes a critical factor. One way to enhance performance is to minimize unnecessary processing. Using PowerShell’s filtering capabilities before sending data to AWK can significantly reduce the amount of data to process.
Error Handling
Implementing error handling is essential for robust scripting. You can use `try` and `catch` to manage errors effectively when using AWK commands:
try {
Get-Content 'data.csv' | awk -F, '{ print $2 }'
} catch {
Write-Host "An error occurred: $_"
}
This structure will help you gracefully manage any unexpected errors that may arise during execution.
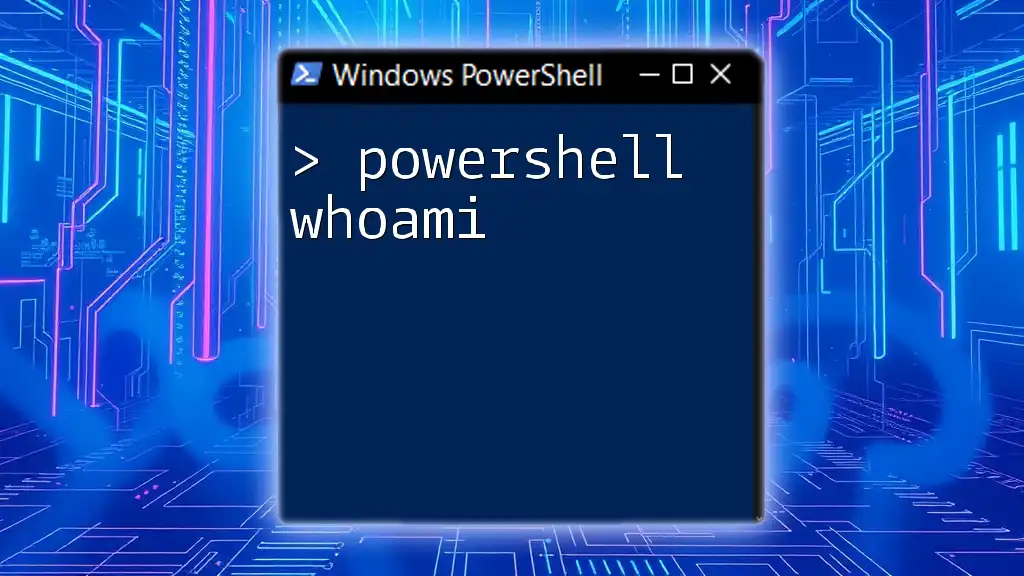
Conclusion
In summary, understanding PowerShell AWK opens up a world of possibilities for efficient data manipulation and analysis within the Windows environment. By mastering the basics of accessing and utilizing AWK commands in PowerShell, you propel your automation and scripting skills to new heights. As you practice and delve deeper into the intricacies of both PowerShell and AWK, consider exploring additional resources to enhance your knowledge further. Whether for system administration, log analysis, or data extraction, the synergy between PowerShell and AWK can lead to streamlined workflows and robust automation solutions.