The PowerShell backtick (`) is an escape character used to allow special characters to be included in strings or to continue a command on the next line.
Here's a code snippet demonstrating its use:
Write-Host 'Hello, World! This is a backtick:`nNext line!'
The Importance of the Backtick in PowerShell
The PowerShell backtick (`) serves as a vital tool in scripting, particularly for those who need to maintain complex scripts without sacrificing readability. Whether you're a beginner or an experienced scripter, understanding the significance of the backtick can elevate your coding practices.
Handling Line Continuation
In PowerShell, commands can often become lengthy and unwieldy. The backtick acts as a line continuation character, allowing you to split a single command over multiple lines. This practice not only enhances readability but also simplifies debugging and maintenance.
Line Continuation Example
Consider the following example, which demonstrates how to effectively use the backtick for a multiline command:
Get-Process `
| Where-Object { $_.CPU -gt 100 } `
| Select-Object Name, CPU
In this snippet, the backtick allows us to break the command into separate lines. Each line logically flows into the next, making it easier to understand the intent of the script at a glance.
Explanation of the Example
By using the backtick, this command becomes more organized. It clearly delineates each step of the process: retrieving all processes, filtering those with CPU usage greater than 100, and finally selecting relevant properties.
Usage in String Literals
The backtick is also essential when working with strings that contain special characters, like quotes or other backticks. When you need to include these within a string, the backtick acts as an escape character.
Example of Using Backticks in Strings
Here’s how you can use the backtick to include a backtick in your string:
$exampleString = "This is a test string with a backtick: `` `"
Explanation of the Example
In this case, you need to use double backticks to escape a single backtick within a string. This helps prevent any unintended behavior from the PowerShell parser, ensuring the string is preserved exactly as intended.

Common Mistakes and Troubleshooting
Common Errors with Backticks
While the backtick is a powerful feature, misuse can lead to frustrating errors. One of the most frequent problems arises from unintended line breaks when a backtick is placed incorrectly.
Example of a Mistake
Consider the following code snippet that illustrates this issue:
Write-Host "This is a string `
that continues but breaks."
Explanation of the Mistake
In this case, the backtick at the end of the first line should indicate a continuation, but instead, it creates an unexpected result. The command effectively "breaks," leading to confusion and the potential for scripting errors.
Tips for Avoiding Mistakes
To evade these common pitfalls, consider adopting a few best practices:
- Keep Lines Short: Aim for shorter lines when possible. This reduces the chance of errors and makes the script easier to read.
- Double-Check Placement: Always verify that your backtick is the last character on the line. This ensures you're effectively signaling a line continuation.

PowerShell vs. Other Scripting Languages
PowerShell's treatment of line continuation with the backtick stands out when compared to other programming languages like Python or JavaScript.
Comparison with Other Languages
- Python: Python uses a backslash (`\`) to indicate line continuation, but it also supports implicit line continuation within parentheses, brackets, and braces.
- JavaScript: While JavaScript allows multiline strings using template literals, traditional line continuation requires careful attention to syntax.
Advantages of PowerShell Backticks
The explicit usage of backticks in PowerShell allows for clear delineation without relying on additional constructs, offering a straightforward approach that many PowerShell users find intuitive.
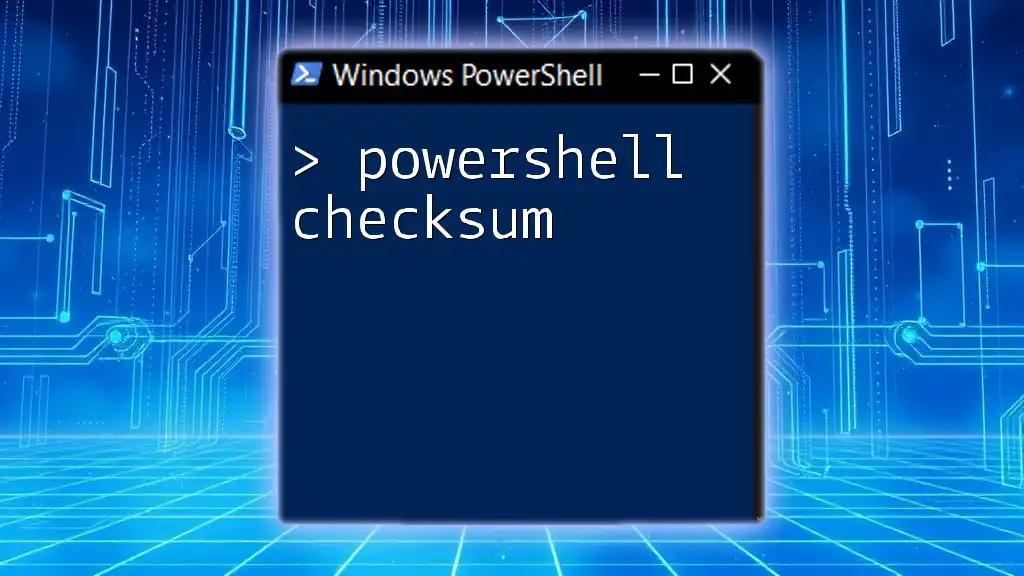
Real-world Scenarios and Applications
Using Backticks for Complex Scripts
In practice, backticks become invaluable when you’re writing more complex scripts. They can help maintain clarity and logical flow in your PowerShell code.
Example of a Complex Script
Here’s an example that showcases the practical use of backticks:
$users = Get-ADUser -Filter * `
| Where-Object { $_.Enabled -eq $true } `
| Select-Object Name, Email, LastLogon
Explain Step-by-step
In this script:
- Get-ADUser is called to retrieve user accounts.
- The backtick allows us to extend this command across multiple lines, keeping each logical portion separate.
- We filter for only enabled users and then select specific properties.
By employing the backtick in this scenario, the overall flow and logic of the script are clear and accessible, enabling easier future modifications.
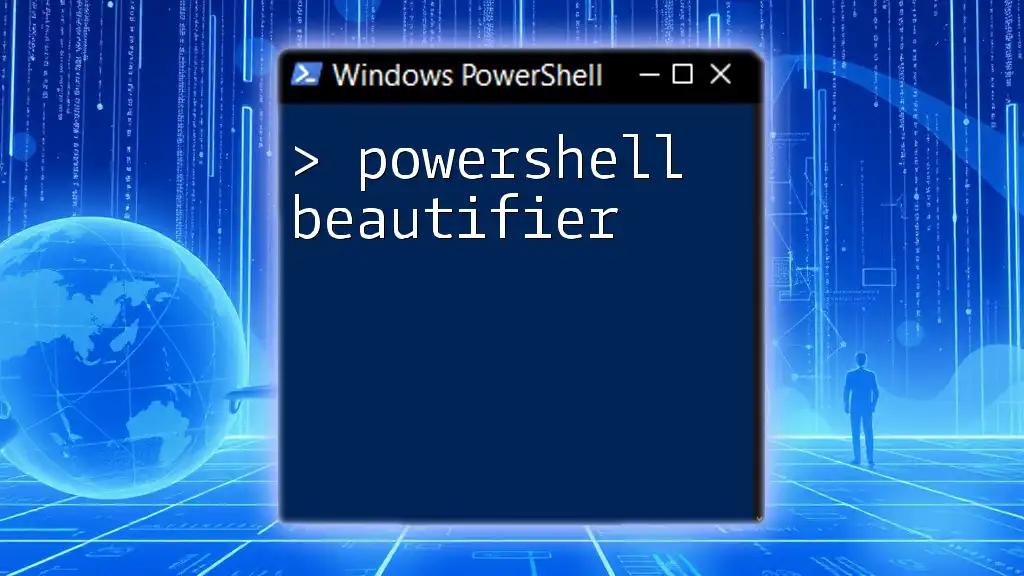
Conclusion
In summary, mastering the PowerShell backtick can significantly enhance the way you write and maintain scripts. By allowing for line continuation and enabling the inclusion of special characters, the backtick is a fundamental aspect of scripting that promotes clarity and efficiency.
Call to Action
Practice using backticks in your scripts and observe how they improve readability and ease of maintenance. Share your experiences and any tips you discover, as communal learning is key in the scripting community.

Additional Resources
For further learning, consider exploring the official Microsoft documentation on PowerShell, engaging with forums, and utilizing community resources to deepen your understanding of PowerShell scripting practices.