In PowerShell, you can automate the acceptance of prompts by using the `-Confirm:$false` parameter, which effectively bypasses the confirmation requirement for commands that normally ask for user input.
Remove-Item C:\path\to\your\file.txt -Confirm:$false
Understanding the Need for Auto Confirmation
Auto confirmation is an essential feature in PowerShell used to eliminate the tedious process of manual confirmations during script execution. It allows scripts to run more efficiently, especially in scenarios where multiple confirmations are required.
Why Auto Confirmation is Important
When a script requires user confirmation for each operation, it can hinder automation and slow down processes that are meant to be executed in bulk. Auto confirming ensures a smooth and unbroken execution, allowing developers to focus on other areas of their workflow without constant interruptions.
Common Use Cases for Auto Confirm
Several scenarios highlight the need for auto confirmation, including:
- Bulk deletions: When you need to remove several files or directories, confirmations can become cumbersome.
- System configurations: Adjusting multiple settings across systems can involve numerous confirmations.
- Automated deployments: Deploying applications or updates across numerous systems typically requires user validation for each action undertaken.

PowerShell Cmdlets and Parameters for Auto Confirmation
PowerShell contains several cmdlets that feature confirmation prompts. Understanding these commands is key to leveraging auto confirmation effectively. Key cmdlets include `Remove-Item`, `Set-ExecutionPolicy`, and more.
Using the -Confirm Switch
Most cmdlets expose a `-Confirm` parameter that prompts the user before an action is executed. Here’s a simple syntax for using the `-Confirm` switch:
Remove-Item 'C:\path\to\your\file.txt' -Confirm
Using this switch, a confirmation popup appears before proceeding with the removal of the specified file.
Example: Using -Confirm for Confirmation
Consider the following command, which removes all items in a directory while prompting for a user confirmation:
Remove-Item 'C:\Temp\*' -Confirm
When executed, PowerShell will seek validation from the user before completing the deletion process.
The -Force Switch
The `-Force` parameter is another powerful option in PowerShell. It allows you to bypass confirmation prompts altogether. This is particularly useful when you are confident about the operations you are about to perform.
To demonstrate the effectiveness of the `-Force` parameter, consider the command below:
Remove-Item 'C:\path\to\file.txt' -Force
In this case, PowerShell will delete the specified file without asking for confirmation, streamlining the process.
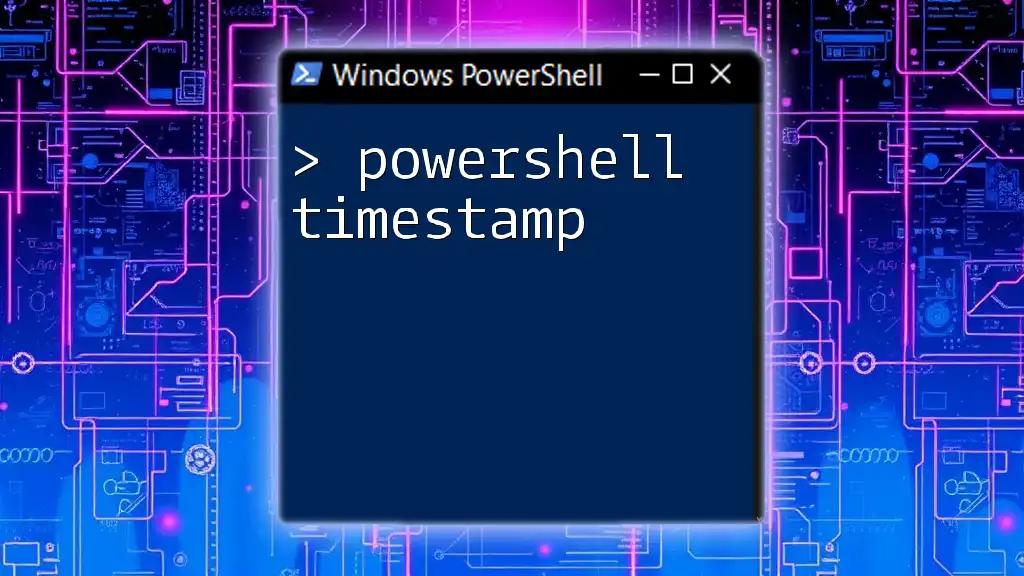
Automating Confirmation for Entire Scripts
For users seeking a more global solution, PowerShell offers the variable `$ConfirmPreference`. This variable controls the confirmation behavior for your current session. By setting `$ConfirmPreference` to `'None'`, you can suppress all confirmation prompts:
$ConfirmPreference = 'None'
While this can be beneficial for executing non-destructive scripts efficiently, caution is advised. Global settings can lead to unintentional consequences. It's best to carefully review your scripts to prevent accidental data loss.
Cautions When Using $ConfirmPreference
Setting `$ConfirmPreference` universally can be risky, particularly in scripts where destructive actions are possible. To safeguard against issues, always ensure that you have a way to revert changes or validate critical operations through other means.

Best Practices for Using Auto Confirm
Understanding when and how to use auto confirmation is vital. It’s important to adopt a strategy that ensures safety and effectiveness.
When You Should Use Auto Confirm
Auto confirmation can be beneficial during operations where:
- The actions are non-destructive and have limited risk.
- You are certain of the context, such as removing temporary files.
- You are running scripts in a controlled environment, such as during testing.
Safeguards You Can Put in Place
To steer clear of unwanted data loss, consider implementing safeguards such as:
- Safety checks within your scripts. For example, prompt for confirmation prior to executing commands that could lead to irreversible changes.
- Validating the existence of files or directories before attempting deletion.
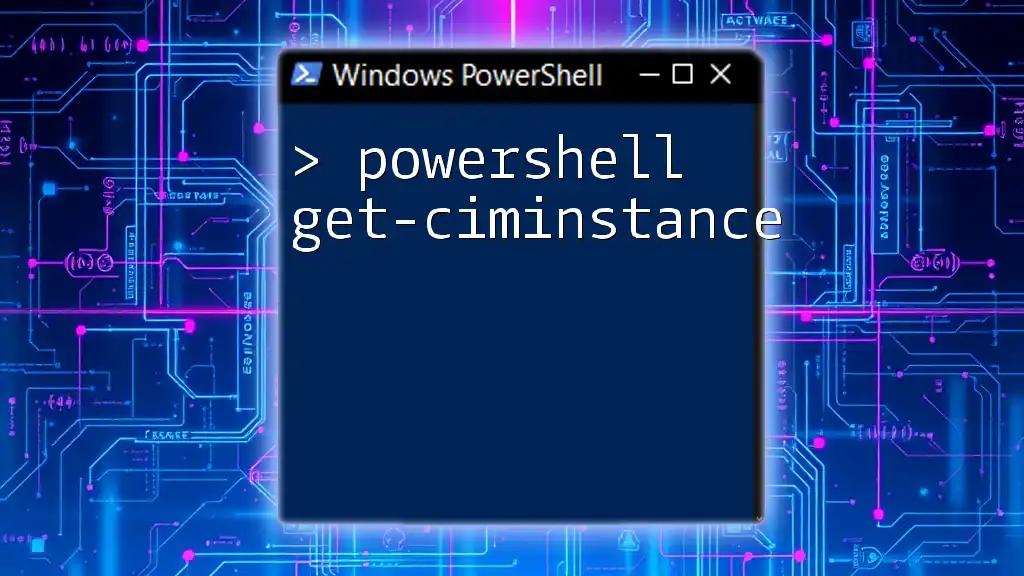
Examples of Auto Confirm in Action
Scenario 1: Bulk Deletion of Files
The following script demonstrates the use of auto confirmation through `Remove-Item` with a `-Force` attribute:
Get-ChildItem 'C:\Temp\*' | Remove-Item -Force
This command retrieves all items in the specified directory and removes them without requiring any confirmation.
Scenario 2: Updating Multiple System Settings
Another scenario where auto confirmation is vital involves setting execution policies across multiple systems:
Set-ExecutionPolicy RemoteSigned -Confirm:$false
By using `-Confirm:$false`, you can change the execution policy without being interrupted by confirmation prompts.
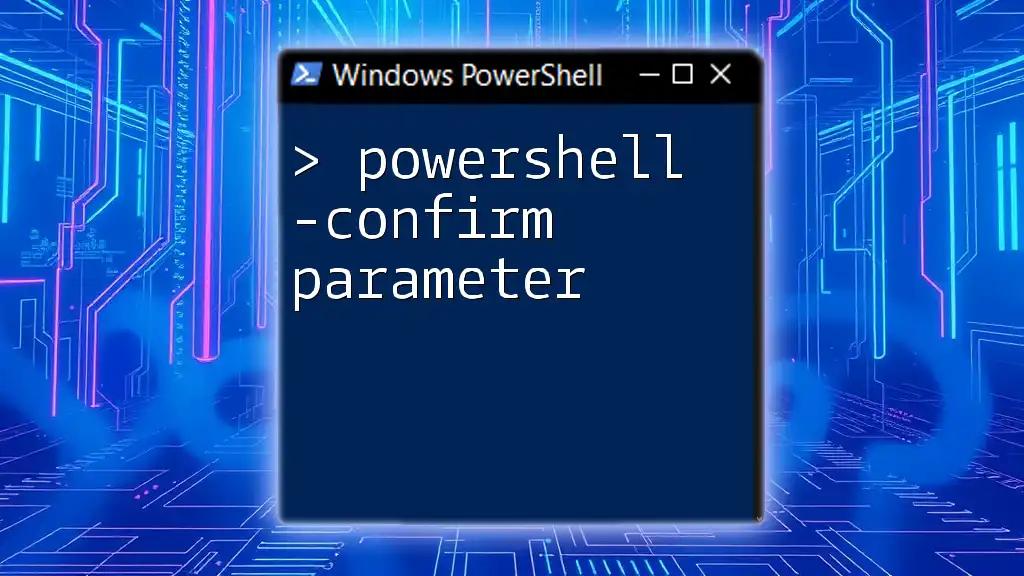
Troubleshooting Common Issues with Auto Confirm
Even with the best intentions, things can go awry. Knowing the common traps associated with PowerShell auto confirm yes to all can help streamline troubleshooting.
When Auto Confirm Doesn't Work as Expected
You might encounter situations where auto confirmation fails. This could arise from:
- Incorrect cmdlet usage or parameters not being applied properly.
- Commands requiring elevated permissions that are not granted in the session.
Debugging Tips
To diagnose issues regarding confirmation prompts, check for:
- Script errors: Use `Try/Catch` blocks to capture issues.
- Validation functions: Ensure that the logic preceding commands is sound and does not inadvertently trigger confirmations.
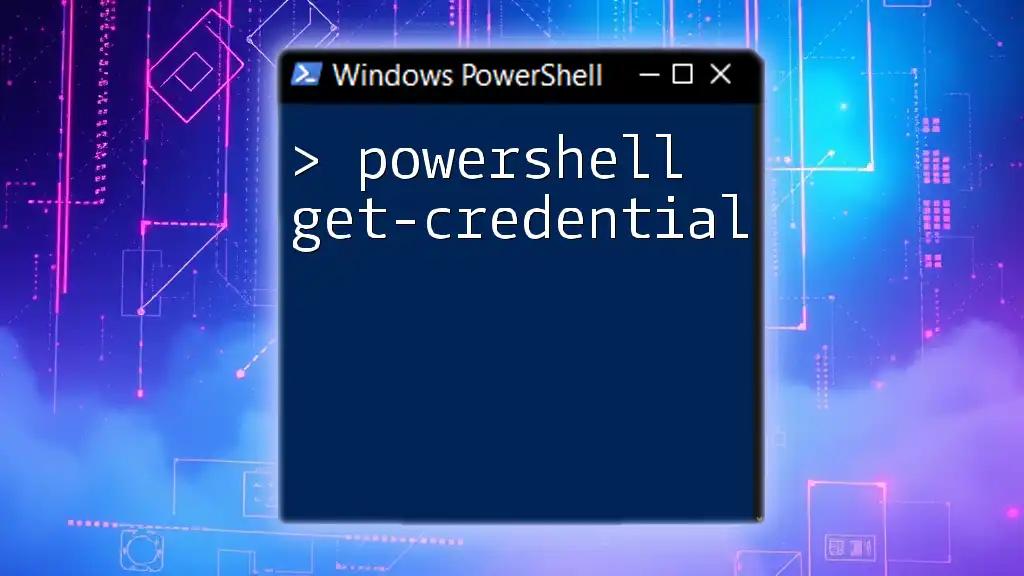
Conclusion
Understanding and leveraging PowerShell auto confirm yes to all can significantly enhance efficiency in your scripting endeavors. By adopting the practices outlined in this guide, you can ensure your PowerShell scripts run seamlessly while avoiding unnecessary interruptions from confirmation prompts.
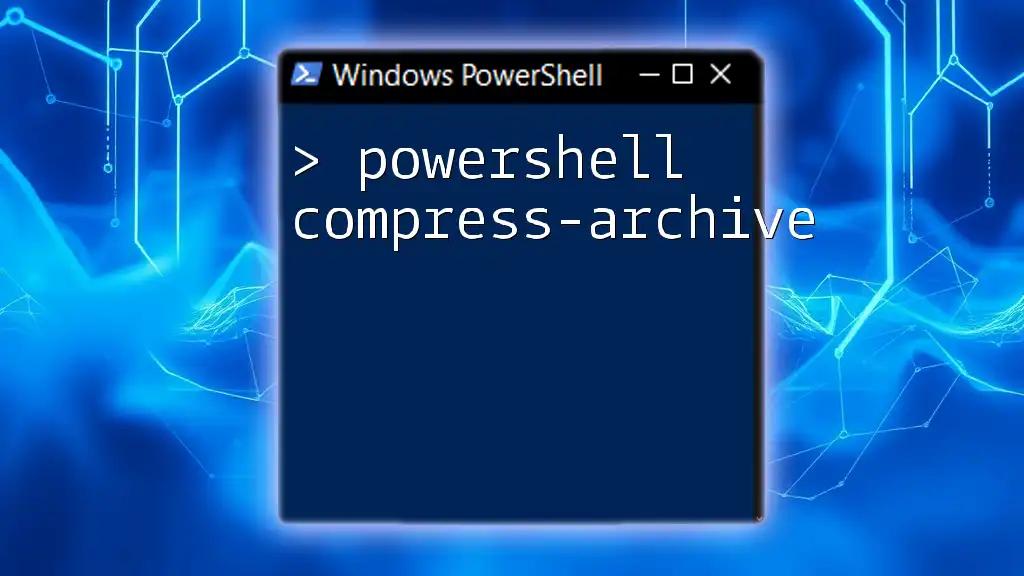
Additional Resources and References
For those eager to delve deeper into PowerShell, consider exploring:
- The official Microsoft documentation on PowerShell cmdlets.
- Online tutorials and courses that emphasize practical scripting skills.
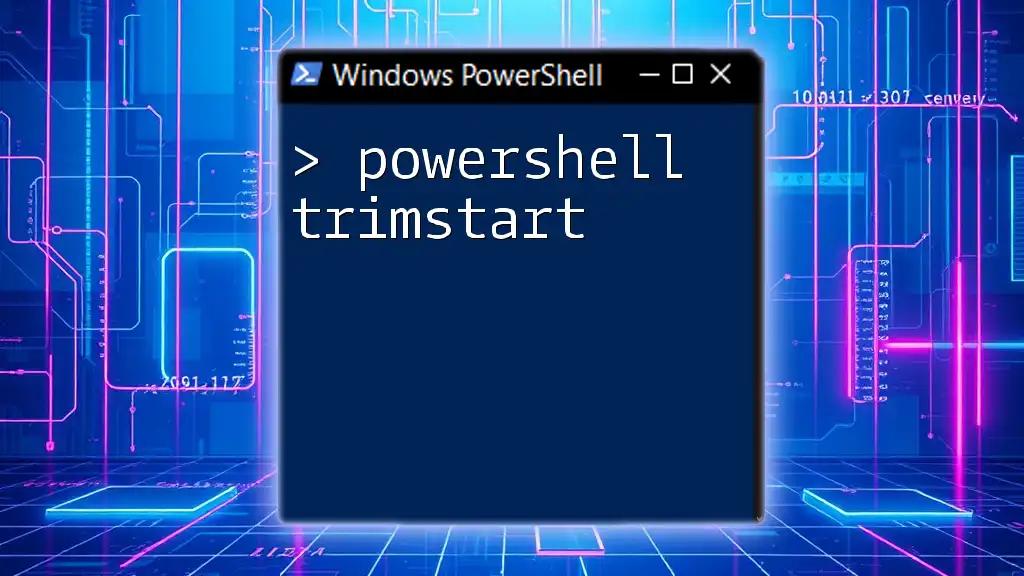
Call to Action
Ready to elevate your PowerShell skills? Join our training program today and learn how to master PowerShell through quick, concise lessons designed for efficiency and effectiveness!