The "PnP PowerShell app registration" process allows users to register Azure AD applications to manage resources in Microsoft 365 through PowerShell commands.
Here’s a code snippet to create an app registration using PnP PowerShell:
$App = New-PnPApp -DisplayName "MyApp" -SignInUri "https://myapp.example.com" -ReplyUrls "https://myapp.example.com/auth" -AppOnly
Understanding App Registration
What is App Registration?
App Registration is a vital concept within Azure Active Directory (AD) that allows developers to create and manage applications that can interact with Microsoft cloud services. In the context of SharePoint Online, app registration enables third-party applications to authenticate users, access resources, and perform various operations programmatically. This is crucial for automating tasks, integrating systems, and enhancing security through scoped access.
Common Use Cases for App Registration
Several scenarios benefit from app registration:
- Automating Tasks in SharePoint: Automate repetitive tasks such as content migration, user provisioning, and site management.
- Integrating Third-Party Applications: Seamlessly connect with tools or services like CRM systems, analytics platforms, or document management solutions.
- Enhancing Security through Scoped Access: Control what parts of SharePoint are accessible to the application, resulting in minimized exposure of sensitive data.
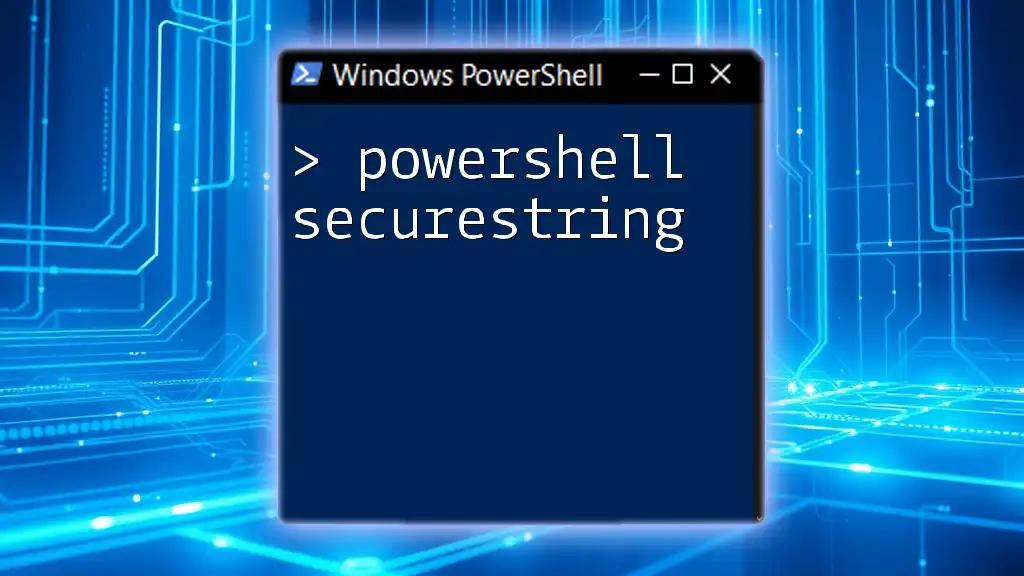
Prerequisites for App Registration
Required Permissions and Roles
Before starting the app registration process, ensure that you have the necessary permissions in Azure Active Directory, typically requiring a SharePoint Administrator role. This role provides the needed access rights to create and manage app registrations effectively.
Installing PnP PowerShell
To operate with PnP PowerShell, the first step is to install the PnP.PowerShell module. You can do this via the following command in PowerShell:
Install-Module -Name PnP.PowerShell
After installation, verify that the module is available by running:
Get-Module -ListAvailable PnP.PowerShell
This step is crucial; having the right tools ensures that you can execute commands and interact with SharePoint Online effectively.
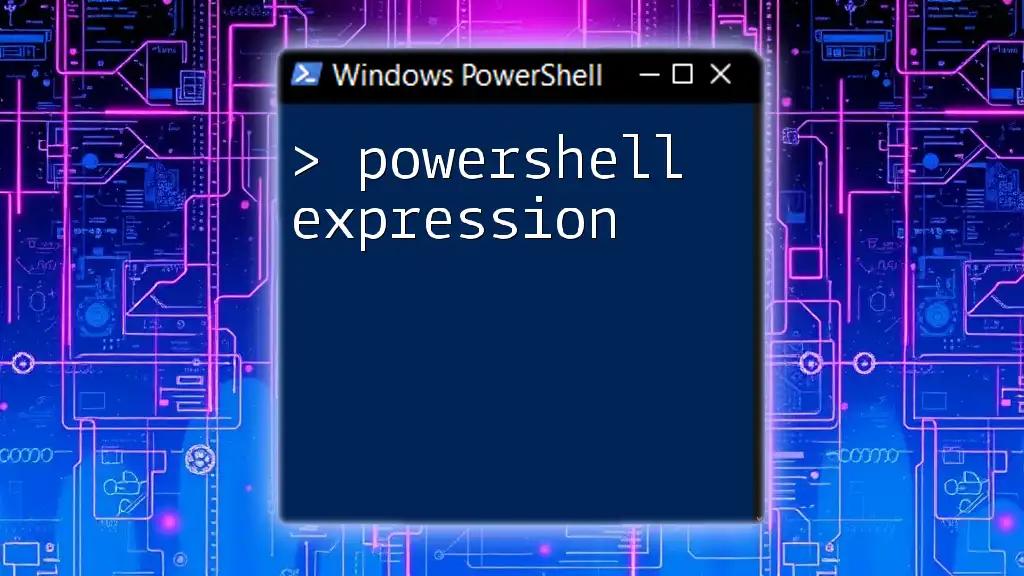
Registering an Application in Azure AD
Accessing Azure Active Directory
To begin the registration process, log in to the Azure portal and navigate to Azure Active Directory. You will find the App Registrations section, which is your gateway to managing application registrations.
Creating a New App Registration
-
From the Azure AD panel, select App Registrations in the left-hand menu.
-
Click on the New registration button.
This action takes you to a form where you will specify the details of the new application.
Configuring App Registration Properties
Setting the Redirect URI
The Redirect URI is essential for authentication flows, particularly for web applications. It represents the location where Azure will redirect users after they authenticate. For example, you might set the Redirect URI as follows:
- `https://localhost/my-app`
This URI must match exactly what you configure in your application code, so ensure it's correctly specified in both places.
Defining Application Permissions
Once you've created the app registration, you will need to set up the necessary application permissions. This includes defining scoped permissions that dictate what your application can access. These might include:
- Sites.Read.All: Allows the application to read all items in SharePoint sites.
- Sites.ReadWrite.All: Grants full read and write access to all items in SharePoint sites.
Be cautious when selecting permissions; always opt for the least privilege needed for the functionality of your application.
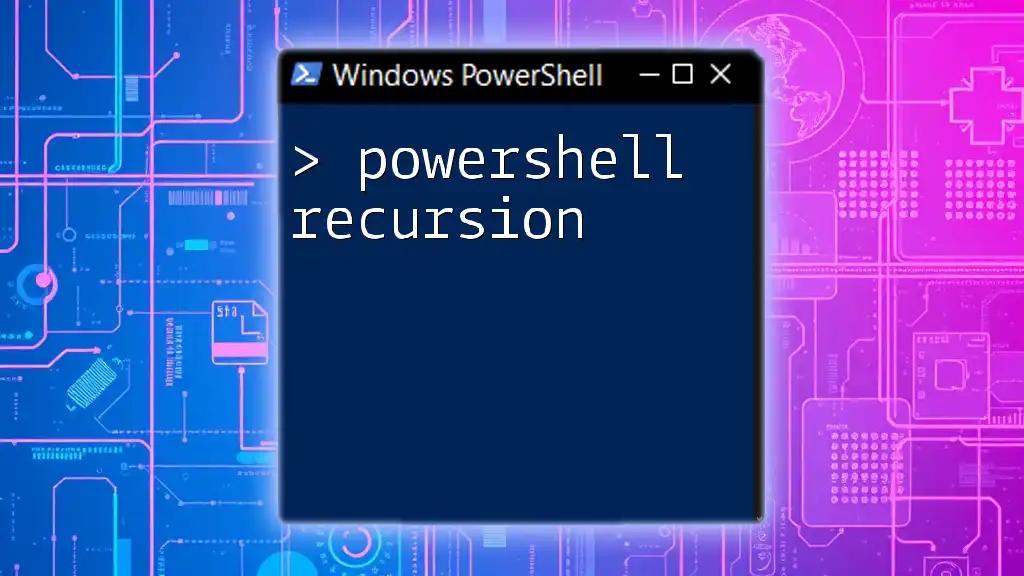
Using PnP PowerShell for App Registration
Authenticating with Azure AD
Before you can execute PnP commands related to your app registration, you'll need to authenticate with Azure AD. Use the following command:
Connect-PnPOnline -Url "https://yourtenant.sharepoint.com" -Scopes "Sites.Read.All"
Replace the URL with your SharePoint site URL, and adjust the scopes according to your application's permission requirements.
Creating an App Registration Programmatically
You can leverage PnP PowerShell to create app registrations programmatically. The following command demonstrates how to create a new app registration:
$app = New-PnPApp -DisplayName "MyApp" -Tenant "yourtenant.onmicrosoft.com" -ReplyUrl "https://localhost/my-app" -RedirectUri "https://localhost/my-app/redirect"
This command generates a new application in Azure AD with the specified display name and settings. Always ensure the values you provide align with the intended functionality of your app.
Retrieving and Managing App Registration Details
Once your application is registered, you may want to retrieve its details. Use the following command to get information on registered applications:
Get-PnPApp -Identity "MyApp"
This command allows you to view the attributes of the app registration. You can also perform updates as needed by using the appropriate PnP PowerShell commands.
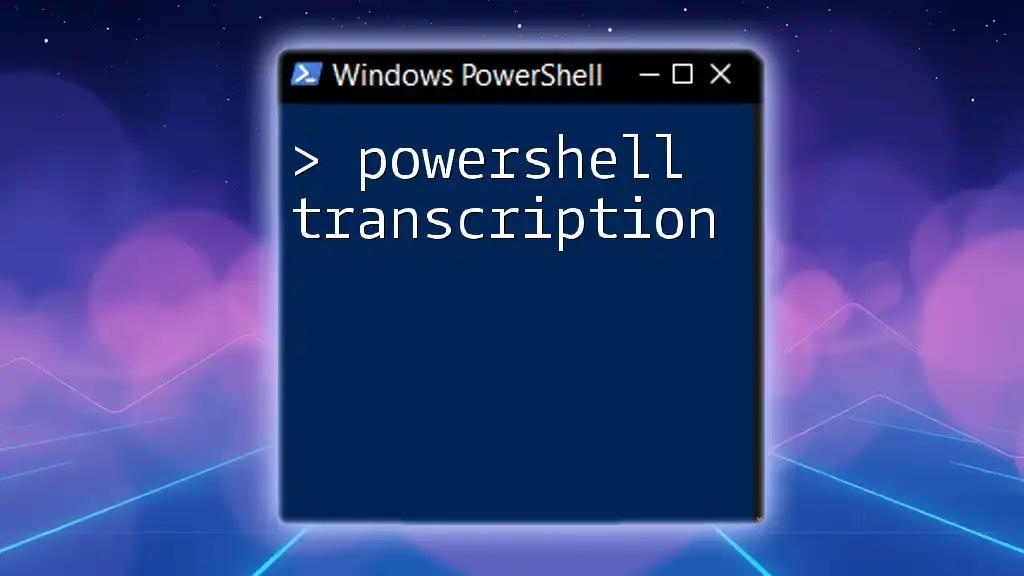
Common Issues and Troubleshooting
Common Errors During Registration
During the app registration process, you may encounter various errors. Some common pitfalls include:
- Invalid Redirect URI: Ensure that your Redirect URI is correctly entered and matches your application code.
- Insufficient Permissions: Double-check that your Azure AD account has the required roles to register applications.
Debugging Authentication Issues
Authentication failures can be frustrating but are usually straightforward to resolve. Some tips for managing these issues include:
- Inspecting Error Messages: Carefully read the error messages, as they often indicate the nature of the problem.
- Revisiting Permissions: Make sure that all required permissions are granted to your application in Azure AD.
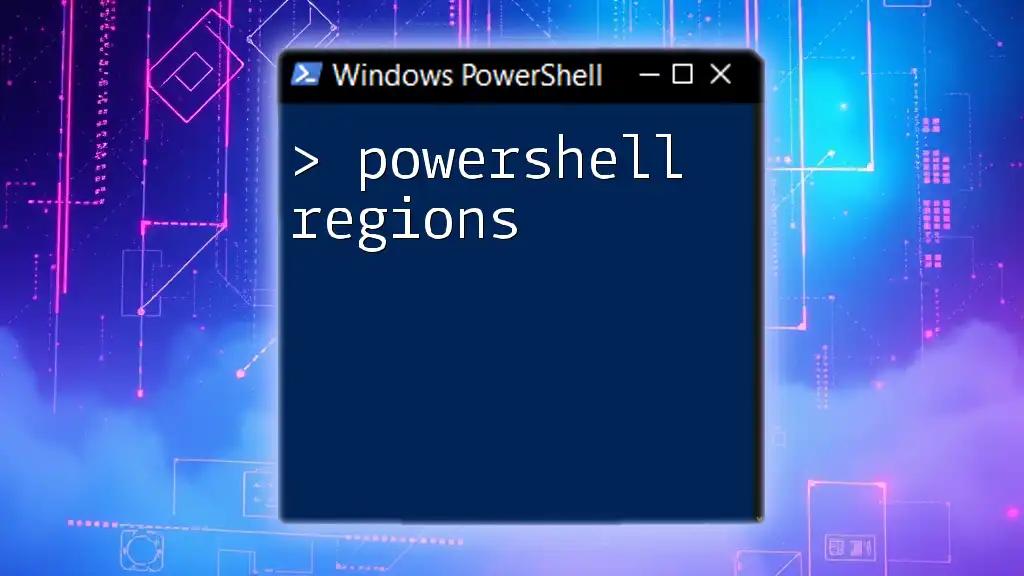
Best Practices for App Registration
Security Best Practices
Maintaining application security is paramount. Here are some recommendations:
- Manage Secrets and Certificates: Use Azure Key Vault to manage sensitive information securely and avoid hardcoding any secrets in your application code.
- Utilize Least Privilege in API Permissions: Only grant the permissions essential for your app's functionality to minimize security risks.
Regular Maintenance of App Registrations
Regularly reviewing app permissions is crucial for security. Make it a habit to:
- Check and update application permissions quarterly or bi-annually.
- Remove any app registrations that are no longer in use or necessary.
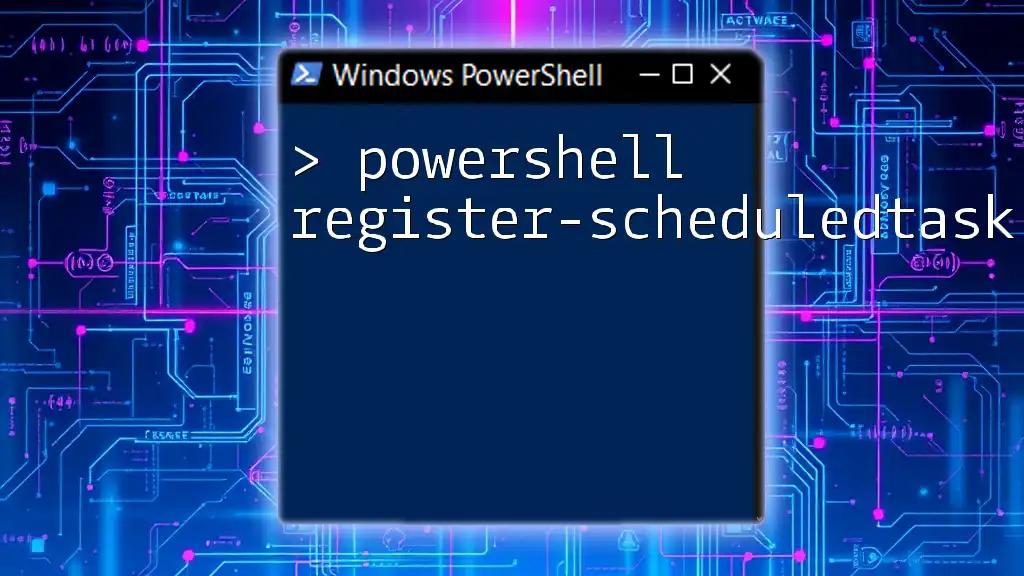
Conclusion
Understanding the process of pnp powershell app registration is central to efficient SharePoint Online management and application integration. By following the steps outlined in this guide, you can successfully register applications, manage their settings, and troubleshoot common issues. Engaging with PnP PowerShell opens up avenues for automating your tasks and enhancing the security of your applications.
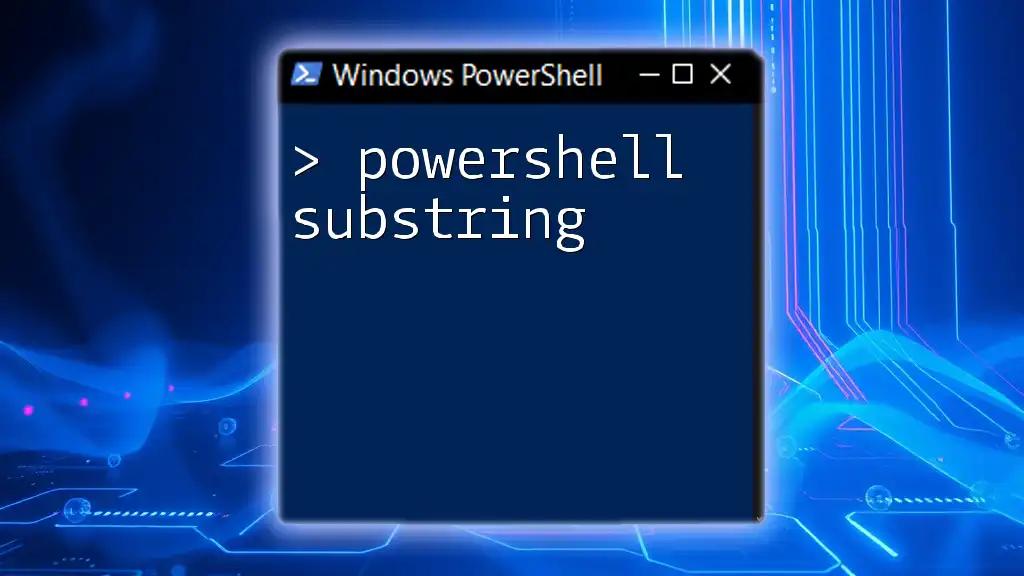
Call to Action
To continue optimizing your skills with PnP PowerShell, explore related articles or consider joining our in-depth training sessions. Stay updated with the latest best practices and features for maximizing your automation capabilities!