PowerShell is a powerful scripting language and command-line shell that offers advanced functionality and automation capabilities compared to the simpler command-line interface (CMD) found in Windows operating systems.
Here's a basic example of a PowerShell command:
Write-Host 'Hello, World!'
What is Command Prompt?
Command Prompt (CMD) is a command-line interpreter application available in most Windows operating systems. Its primary purpose is to execute commands, automate tasks through batch scripts, and manage file systems. Originating in the MS-DOS era, it has evolved to provide basic functionalities for users who prefer working outside the graphical user interface.
Common Commands in CMD
Some frequently used CMD commands include:
- `dir`: Lists the contents of a directory.
- `cd`: Changes the current directory.
- `copy`: Copies files from one location to another.
- `del`: Deletes specified files.
For example, using `dir` gives a simple overview of your current directory:
C:\Users\YourName> dir
This command quickly shows files and folders within the directory without requiring additional software.
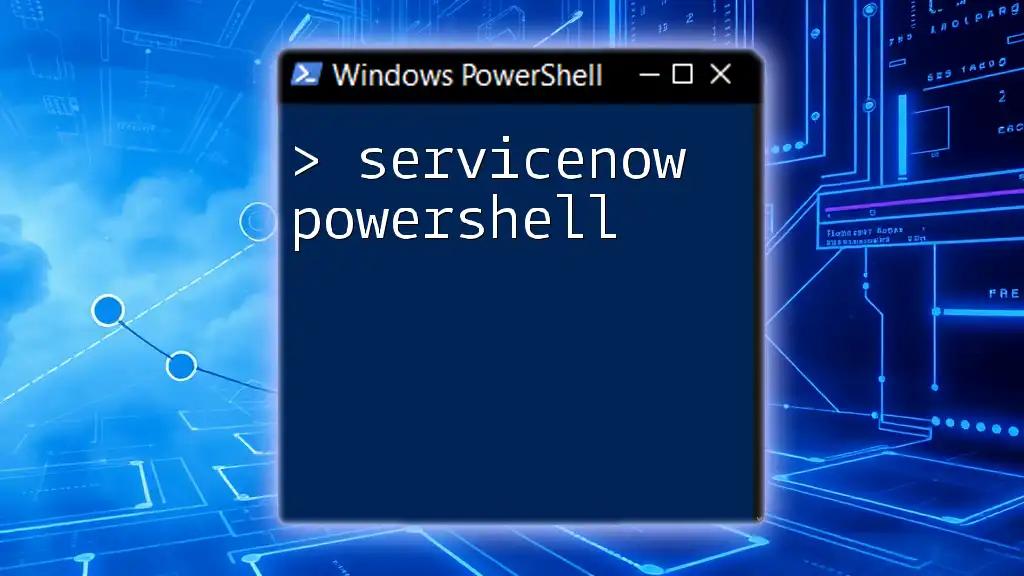
What is PowerShell?
Definition and Purpose
PowerShell was developed as a more advanced shell environment, introduced to provide system administrators with powerful tools for automation and configuration management. Unlike CMD, PowerShell is built on the .NET framework, allowing users to harness the power of objects, making it a robust replacement for traditional command-line operations.
Common Cmdlets in PowerShell
Cmdlets are lightweight commands used in the PowerShell environment. Examples include:
- `Get-Help`: Displays help information about PowerShell commands and concepts.
- `Get-Process`: Retrieves a list of active processes on your system.
- `Set-Location`: Changes the current directory in PowerShell.
An example of using `Get-Process` would be:
Get-Process
This command retrieves and displays a list of all processes currently running on your system, presenting them as objects rather than plain text.
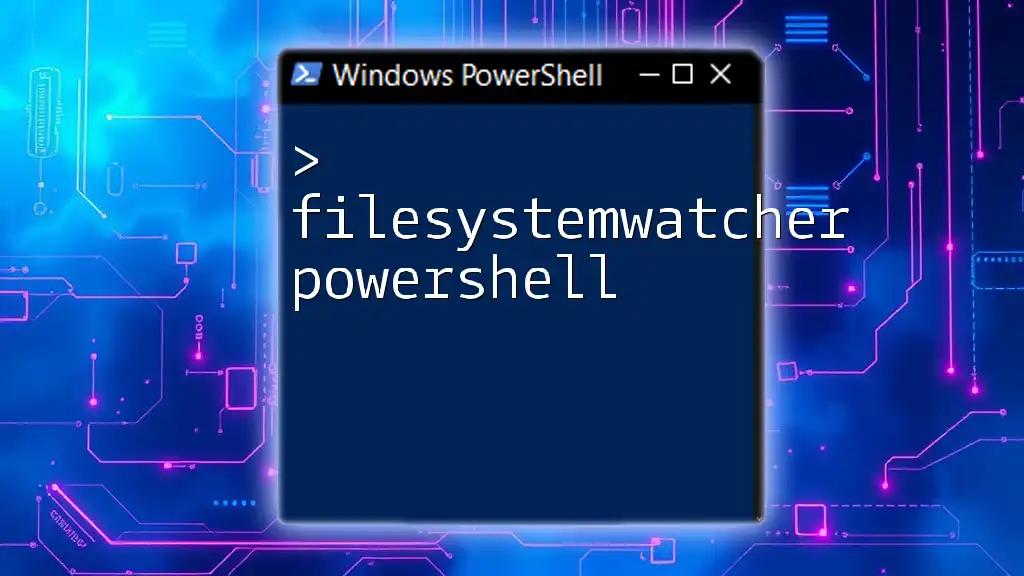
Main Differences Between PowerShell and CMD
Scripting Capabilities
PowerShell shines when it comes to scripting. It uses a rich language designed for creating advanced scripts far beyond the capabilities of traditional batch files found in CMD. PowerShell scripts can easily interact with .NET objects, enabling actions ranging from simple automation of repetitive tasks to complex workflows across multiple systems.
For example, consider a simple script in PowerShell that retrieves and outputs the names of all running processes:
$processes = Get-Process
foreach ($process in $processes) {
Write-Host $process.Name
}
In contrast, CMD scripts are limited and can quickly become complex with an increase in functionality, often leading to intricate batch files that are less readable and harder to maintain.
Input and Output Handling
The way both environments handle input and output drastically differs. PowerShell's piping mechanism allows users to pass the output of one cmdlet into another seamlessly, working with objects rather than plain text.
For instance, the following PowerShell command retrieves active processes and filters for those using more than 100 MB of memory:
Get-Process | Where-Object { $_.WorkingSet -gt 100MB }
In CMD, piping is limited. You can pipe text output (e.g., using the `|` operator), but any data manipulation typically relies on simple commands like `find` or `findstr`, making complex data interaction cumbersome.
Object vs. Text-Based
PowerShell operates on the concept of objects. Each command returns objects that can be manipulated, allowing more intricate interactions between data. For example, each process retrieved by `Get-Process` can be accessed as an object with properties like `Name`, `Id`, and `WorkingSet`.
For comparison, CMD is fundamentally text-based. Commands produce text output, requiring further parsing if you want to extract specific data. This restricts users in terms of functionality and usability.
Syntax and Command Structure
The syntax between CMD and PowerShell is also distinctly different. PowerShell commands, known as cmdlets, follow the Verb-Noun structure, making them intuitive and self-descriptive.
For example, consider a basic command in both environments:
- CMD:
echo Hello World
- PowerShell:
Write-Host "Hello World"
PowerShell's cmdlet structure simplifies learning and understanding for new users.
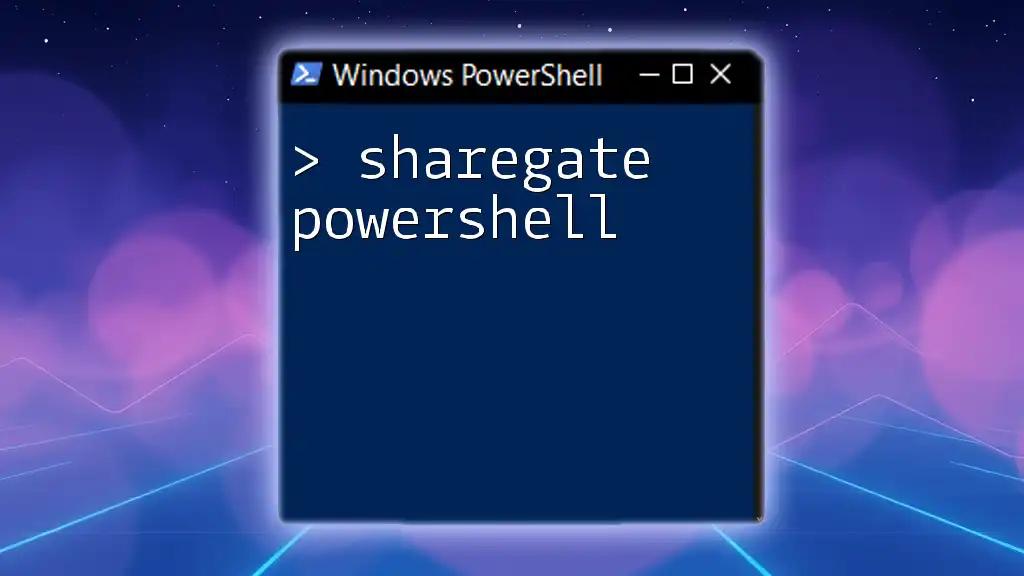
Use Cases for PowerShell and CMD
When to Use Command Prompt
CMD is ideal for simple tasks and for those who are beginners. It provides a straightforward interface for quick commands such as file navigation and basic operations. It's beneficial when compatibility with older programs and scripts is essential.
When to Use PowerShell
PowerShell stands out for advanced administration tasks and operations that require extensive automation. Its ability to manage system configuration, handle complex data types, and integrate numerous Windows features makes it indispensable for IT professionals. Common use cases for PowerShell include:
- Automating software installations.
- Managing servers and services in enterprise environments.
- Conducting system audits and inventory management.
For example, running a PowerShell script to update multiple systems at once showcases its superiority over CMD.
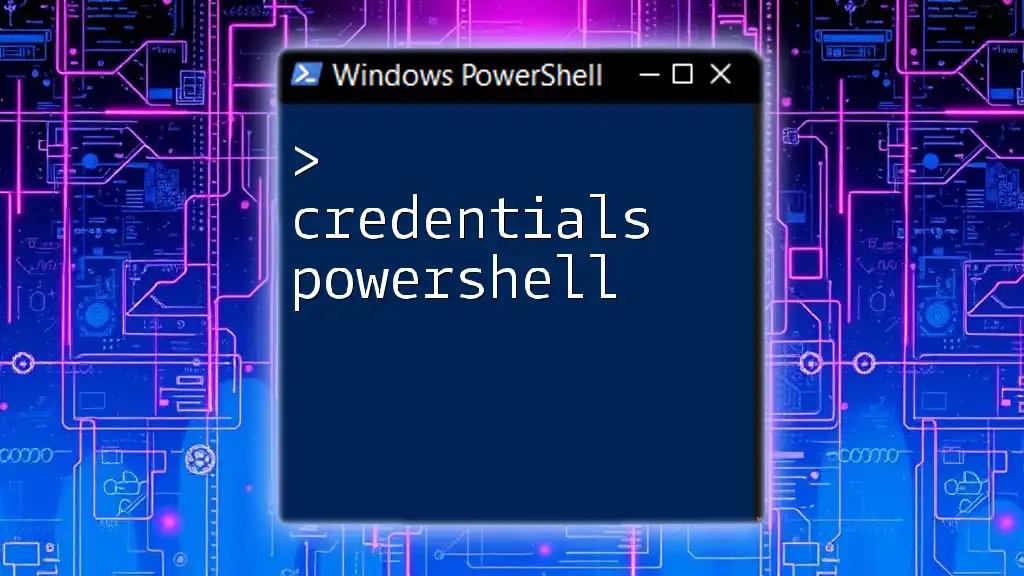
Installing and Running PowerShell
Versions of PowerShell
PowerShell has seen several iterations, evolving from Windows PowerShell, which is integrated into the Windows OS, to PowerShell Core, a cross-platform version that runs on Windows, macOS, and Linux.
Understanding which version to use may depend on your environment and specific needs. PowerShell Core offers broader compatibility across different operating systems, making it a versatile tool for modern administrators.
How to Open PowerShell
You can open PowerShell using various methods:
- Using the Search Bar: Type "PowerShell" in the Windows search bar and select it.
- Using Win + X Menu: Right-click on the Start button and select "Windows PowerShell" or "Windows PowerShell (Admin)."
- Via Command Prompt: Type `powershell` in CMD to switch to PowerShell directly.
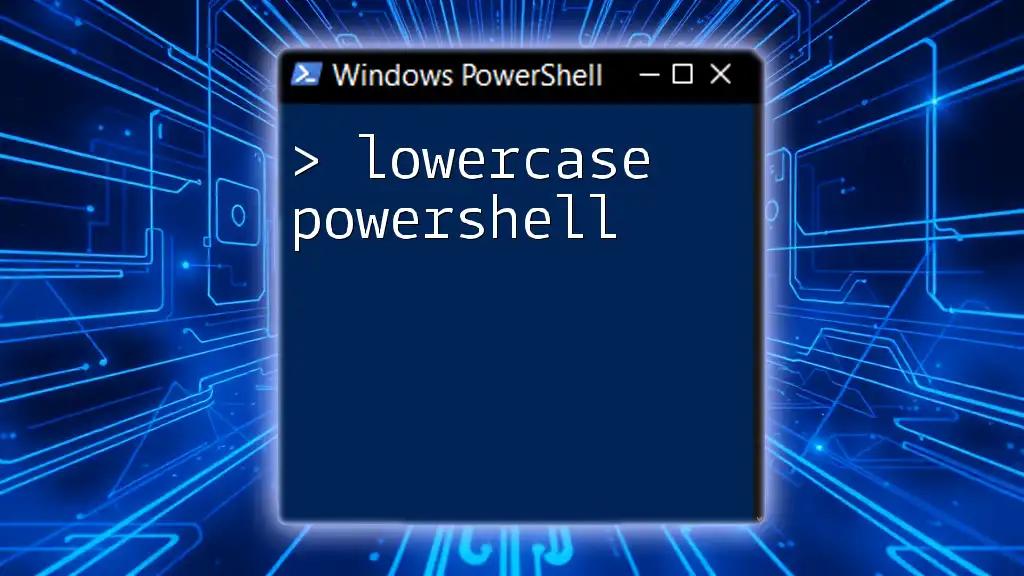
Conclusion
Understanding the difference between PowerShell and CMD is crucial for anyone diving into Windows command-line tools. While CMD remains a fundamental tool for simple tasks, PowerShell elevates user capabilities with its object-oriented design and advanced scripting options. Choosing the right tool helps in maximizing productivity and efficiency in various computing tasks.
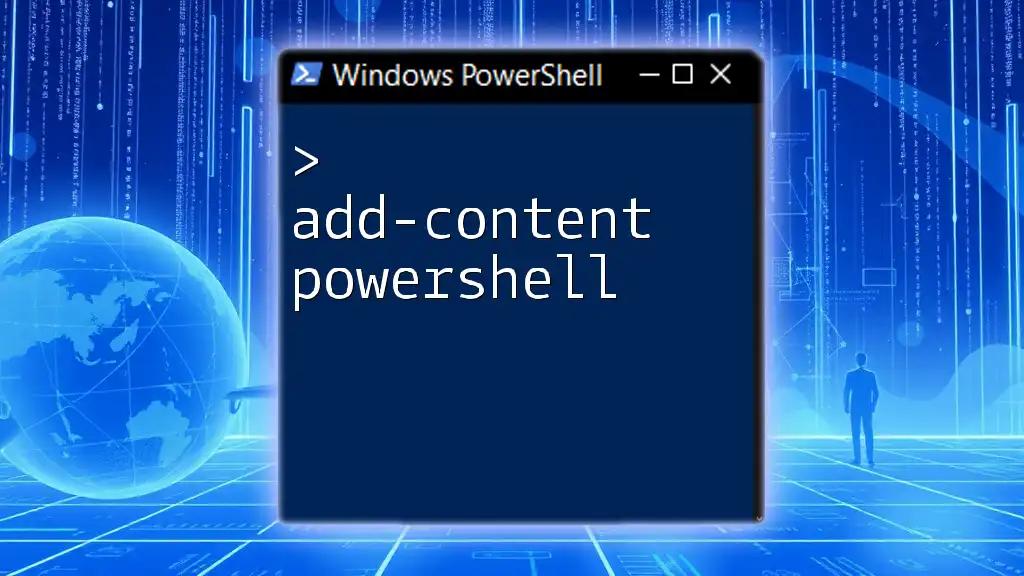
Additional Resources
For those eager to expand their knowledge, consider exploring various courses and tutorials focused on PowerShell’s vast capabilities. Engaging with online communities and forums can also offer ongoing support and shared learning opportunities.
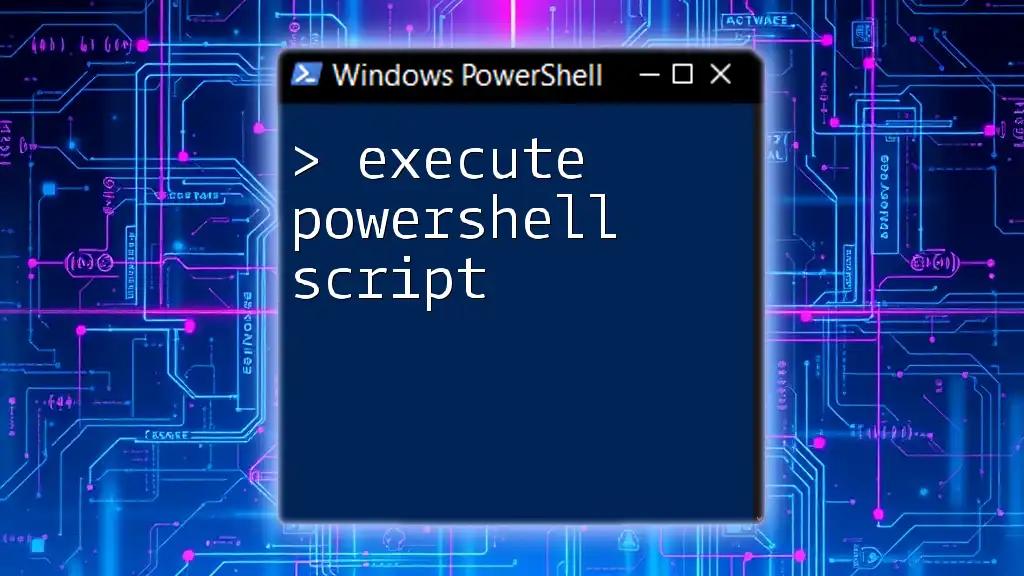
Appendices
Example Scripts and Commands for Reference
Here’s a handy reference table comparing some of the most common commands between CMD and PowerShell:
CMD Command | PowerShell Cmdlet |
---|---|
`dir` | `Get-ChildItem` |
`del` | `Remove-Item` |
`copy` | `Copy-Item` |
`echo` | `Write-Host` |
References and Further Reading
For deeper insights into PowerShell and CMD, explore books, articles, and additional online resources designed to enhance your understanding and practical skills in using these powerful tools.