To obtain an access token using PowerShell, you can utilize the `Invoke-RestMethod` cmdlet to send a request to the relevant API endpoint for authentication.
$token = Invoke-RestMethod -Uri 'https://yourapi.com/token' -Method Post -Body @{grant_type='client_credentials'; client_id='your_client_id'; client_secret='your_client_secret';} -ContentType 'application/x-www-form-urlencoded'
Write-Host $token.access_token
Understanding Access Tokens
What is an Access Token?
An access token is a security credential that is used to access protected resources. Access tokens are often used in API authentication scenarios, where a client application needs to interact with another service on behalf of a user or itself. The most commonly used types of access tokens are Bearer tokens and OAuth tokens, each serving its own specific purpose and security model.
Importance of Access Tokens
Access tokens play a vital role in ensuring secure data access and maintaining user authentication and authorization. They allow applications to act on behalf of users without requiring them to provide credentials every time they interact with an API. This helps improve user experience while also securing sensitive data through defined scopes and permissions.
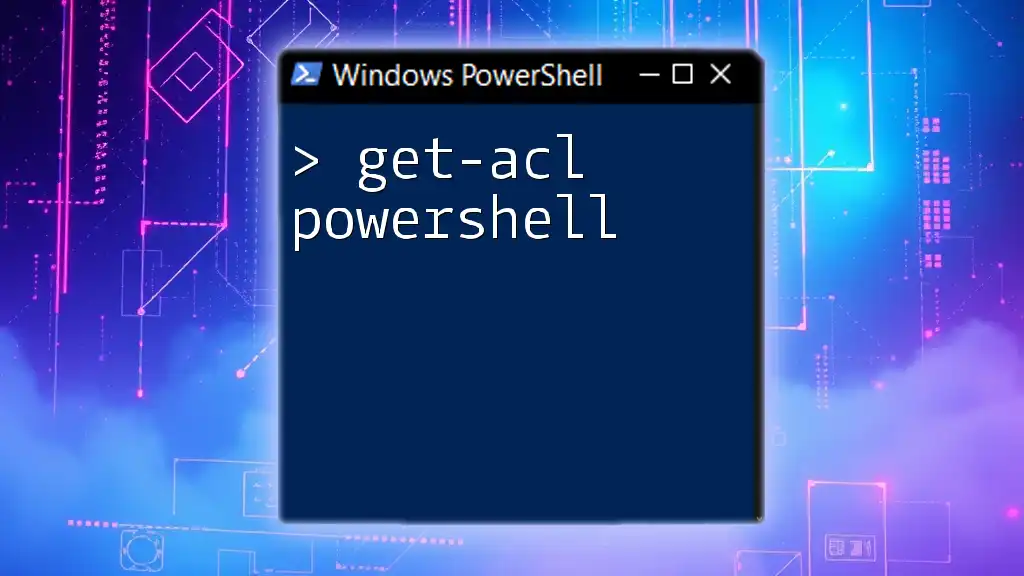
Prerequisites for Using PowerShell to Get Access Tokens
PowerShell Installation
Before you can get started with PowerShell, ensure that you have PowerShell installed on your system. PowerShell is available for various platforms such as Windows, macOS, and Linux. You can download and install it from the official Microsoft website or use platform-specific package managers.
Required Modules and Tools
To successfully request access tokens, you will need specific PowerShell modules. The most relevant ones are:
- AzureAD: This module provides cmdlets to manage your Azure Active Directory resources.
- MSOnline: Useful for managing your Microsoft Online Services.
To install these modules, use the following command in your PowerShell environment:
Install-Module AzureAD
Authentication Mechanisms
Understanding various authentication methods is crucial for obtaining access tokens. The two most common methods are OAuth2 and Service Principal Authentication.
- OAuth2 is a protocol for authorization that allows third-party applications to exchange tokens and access protected resources without exposing user credentials.
- Service Principal Authentication allows applications to authenticate securely without interactive user involvement, which is useful for applications running unattended.
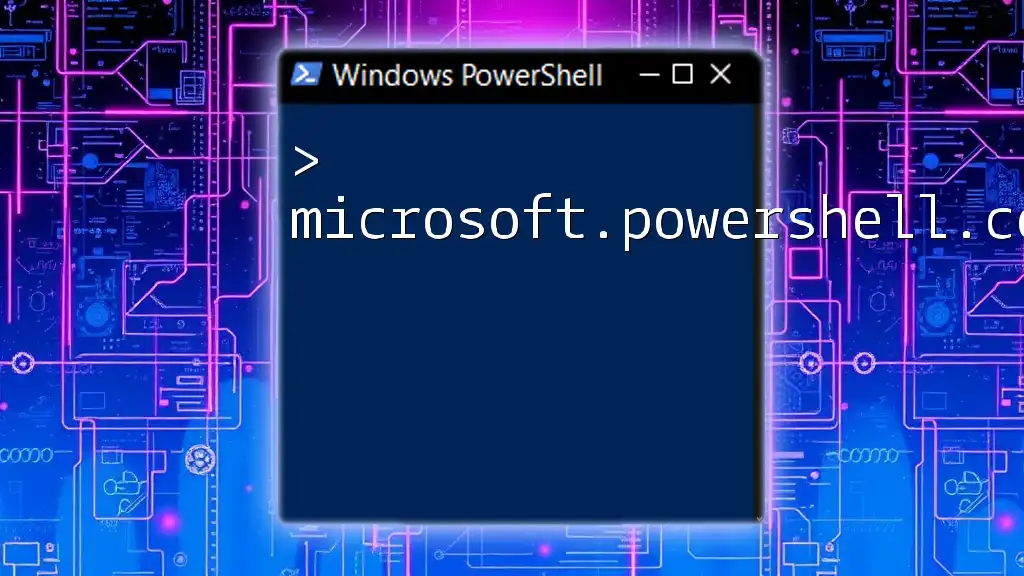
Steps to Get an Access Token with PowerShell
Step 1: Set Up the Azure AD Application
Before you can use PowerShell for obtaining an access token, you need to create an Azure AD application in the Azure Portal. This process involves registering the application and granting it permissions to access specific resources. Ensure you assign the necessary roles to allow your application to perform actions on behalf of users or the organization.
Step 2: Use PowerShell to Request an Access Token
OAuth2 Authentication Flow
The OAuth2 flow consists of various steps that allow the application to obtain access tokens. Here we'll focus on the Client Credentials Grant type of OAuth2 flow, which is suited for service-to-service communication.
Sample Code Snippet
The following PowerShell code snippet demonstrates how to request an access token using the Client Credentials Grant:
$tenantId = "your-tenant-id"
$clientId = "your-client-id"
$clientSecret = "your-client-secret"
$resource = "https://graph.microsoft.com"
$body = @{
grant_type = "client_credentials"
client_id = $clientId
client_secret = $clientSecret
resource = $resource
}
$response = Invoke-RestMethod -Method Post -Uri "https://login.microsoftonline.com/$tenantId/oauth2/token" -ContentType "application/x-www-form-urlencoded" -Body $body
$accessToken = $response.access_token
In this code, we specify essential parameters such as tenant ID, client ID, client secret, and resource that we want to access. The response from the Invoke-RestMethod cmdlet contains our access token, which we can later use for authentication.
Step 3: Making API Calls with the Access Token
Basic API Call Example
Once you have the access token, you can make authenticated API requests. Below is an example of a basic API call to Microsoft Graph API:
$uri = "https://graph.microsoft.com/v1.0/me"
$headers = @{
Authorization = "Bearer $accessToken"
}
$response = Invoke-RestMethod -Uri $uri -Headers $headers -Method Get
In this snippet, we are sending a GET request to the `/me` endpoint of Microsoft Graph API while including the access token in the `Authorization` header. You can expect the response to contain user information, provided that your application has the proper permissions.
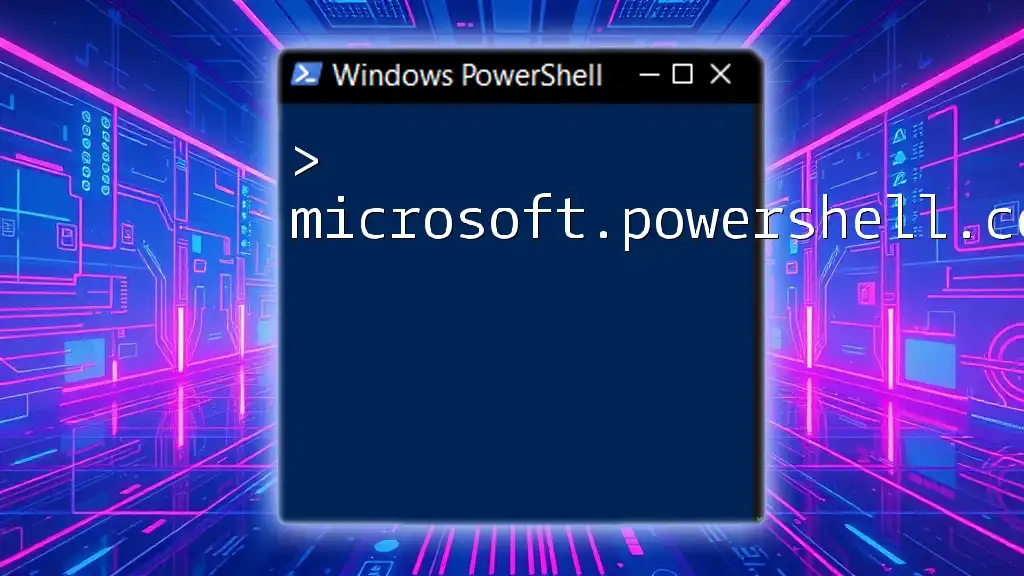
Handling Errors and Troubleshooting
Common Issues and How to Resolve Them
When working with access tokens, you may encounter various issues. Here are a few common error messages and their resolutions:
- Invalid client: This often occurs when the client ID or client secret is incorrect. Double-check the credentials you are using.
- Token expiration: Access tokens are typically short-lived. If you face a token expiration error, you will need to implement a refresh token flow.
- Scopes and permissions: Ensure your application has the correct permissions assigned in the Azure Portal to access the required resources.
Debugging Tips Using PowerShell
Using PowerShell's `Try-Catch` block can help handle errors gracefully. Here’s how you can implement error handling during API calls:
Try {
# Your API Call Here
} Catch {
Write-Host "Error encountered: $_"
}
With this approach, any errors during the API call will be captured and displayed, aiding in troubleshooting.
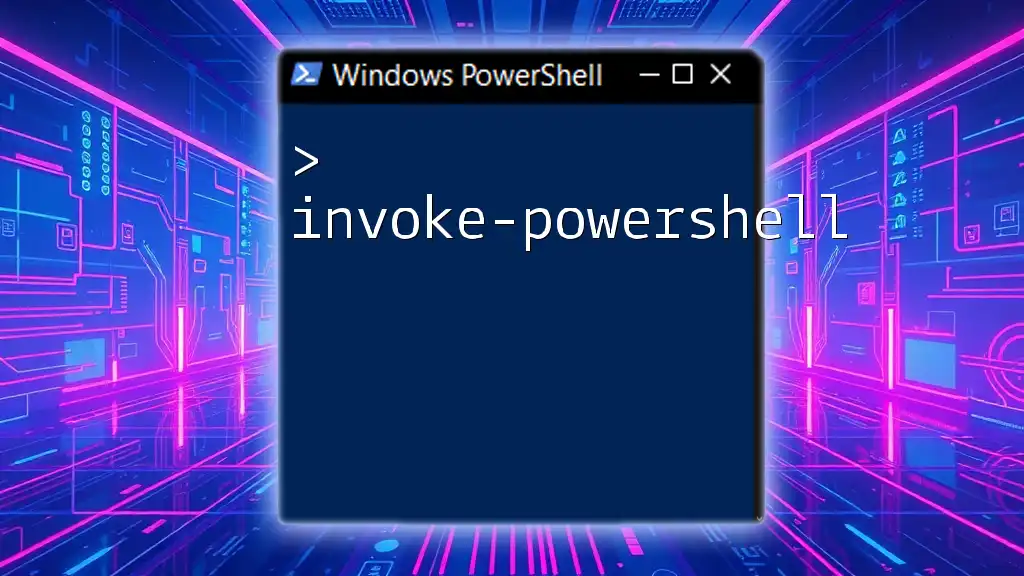
Best Practices for Access Token Management
Secure Storage of Access Tokens
Security should be a top priority when handling access tokens. Store your tokens securely, preferably in a safe storage solution like Azure Key Vault, which provides a secure way to store and access sensitive information.
Token Expiration Handling
Access tokens come with short lifetimes for security reasons. To mitigate issues with expired tokens, implement a mechanism to refresh tokens periodically. Automate this process with PowerShell scripts to ensure seamless application operation.
Monitoring Access Token Usage
Constantly log and monitor your API calls for better oversight. Tools like Application Insights can help keep track of the performance and usage of your application, allowing you to identify any potential issues with token usage.
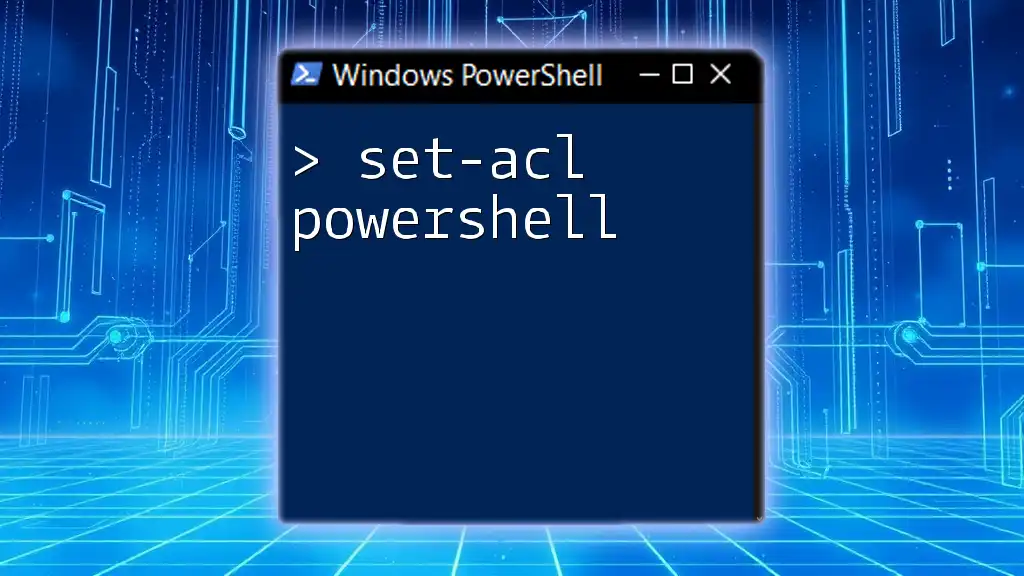
Conclusion
In this guide, we covered how to get an access token PowerShell in a clear, systematic manner. You learned about the significance of access tokens, steps to obtain them, and best practices for secure management and troubleshooting. By implementing these strategies, you can enhance your workflows and ensure secure interactions with APIs.
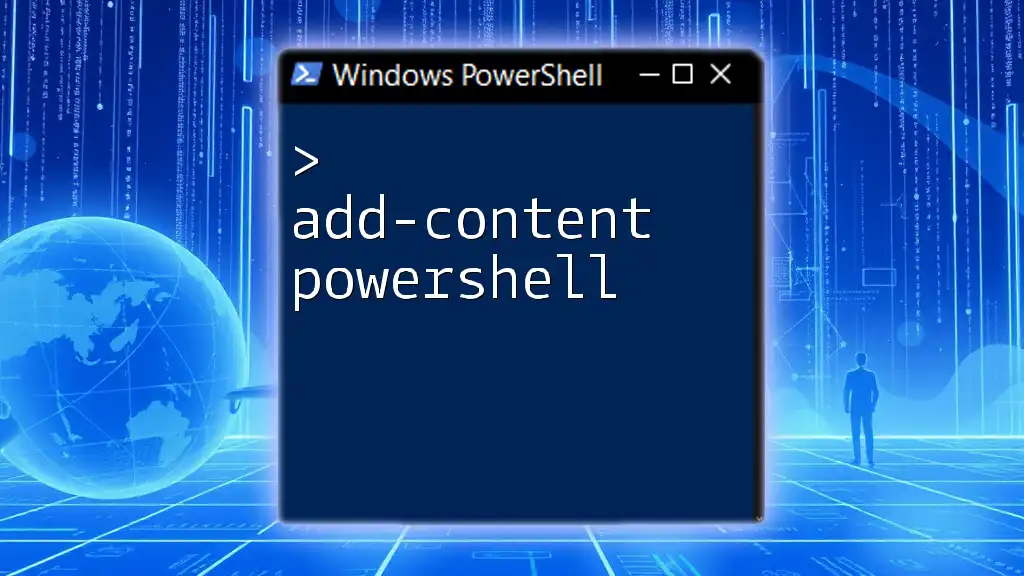
Additional Resources
Links to Official Documentation
- [Microsoft Docs on Azure Active Directory](https://docs.microsoft.com/en-us/azure/active-directory/)
- [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/)
Recommended Courses and Tutorials
To further deepen your understanding, consider exploring additional learning platforms or courses that focus on PowerShell and access token management.
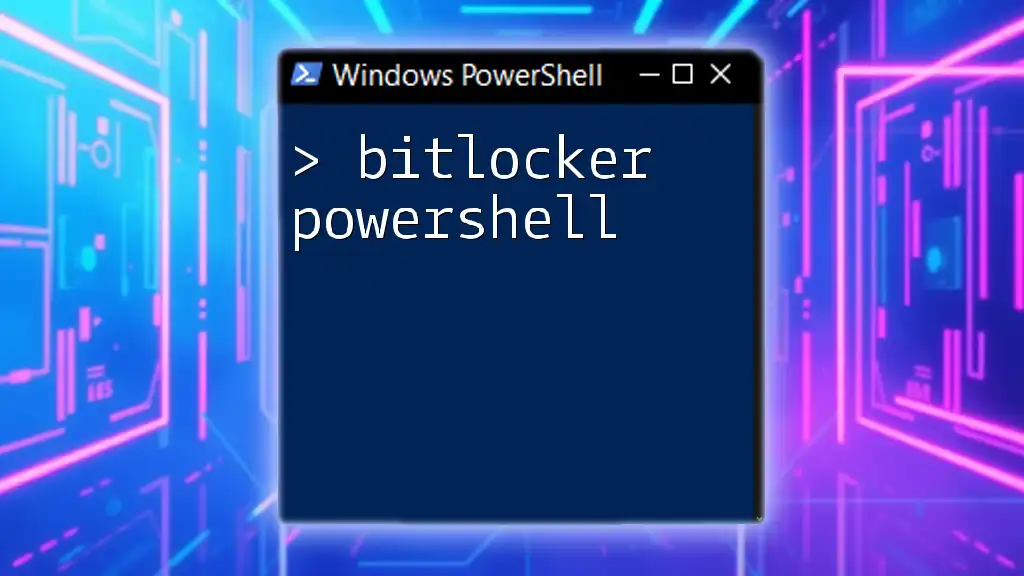
Call to Action
If you found this guide helpful, we encourage you to subscribe to our newsletter or join our community for more updates and PowerShell tips!