The error "cannot index into a null array" in PowerShell occurs when you attempt to access an element of an array that hasn't been initialized, as shown in the following example:
# Attempting to access the first element of a null array
$array = $null
Write-Host $array[0] # This will trigger the error
Understanding the Basics of Arrays in PowerShell
What is an Array?
An array in PowerShell is a collection of items stored under a single variable. This makes it easier to manage and manipulate groups of data. You can have various types of arrays, including single-dimensional arrays, which are linear lists, and multi-dimensional arrays, which can hold data in a structured format.
Why Use Arrays?
Arrays promote efficiency by allowing you to group similar data together, thereby simplifying the management of your scripts. They also provide flexibility, as PowerShell arrays dynamically resize, allowing for numerous operations without a predefined limit.
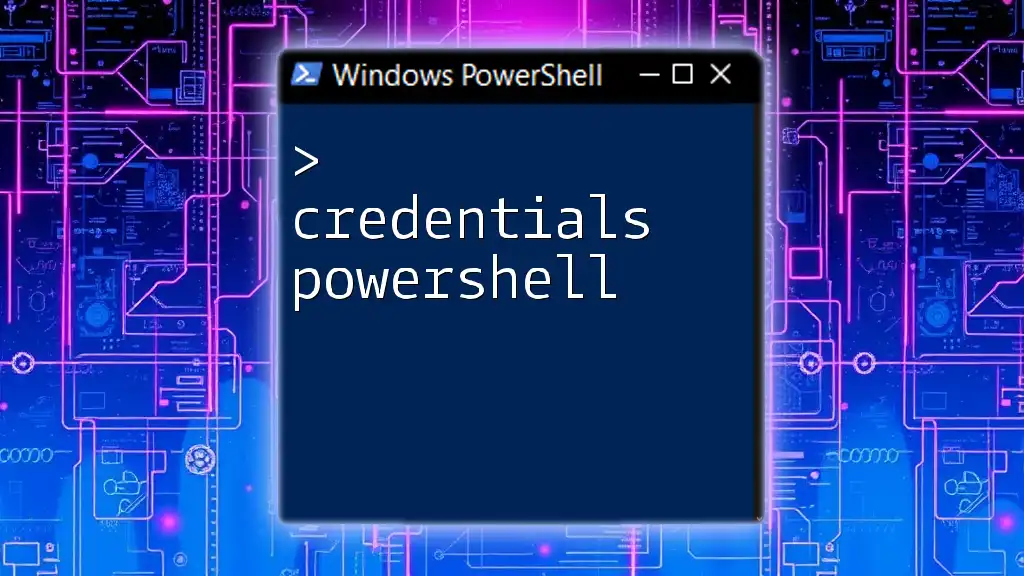
The Null Array Concept in PowerShell
What Does Null Mean?
In PowerShell, null denotes the absence of any value. It indicates that a variable holds no data at that moment. Understanding the distinction between null and an empty array is crucial. An empty array indicates that the array exists but contains no items, whereas null signifies that the array itself isn’t defined.
How Arrays Become Null
Arrays can become null due to several reasons, often linked to improper initialization or a lack of assigned values. Common scenarios include:
- Forgetting to instantiate the array.
- A function intended to populate the array returns nothing or null.
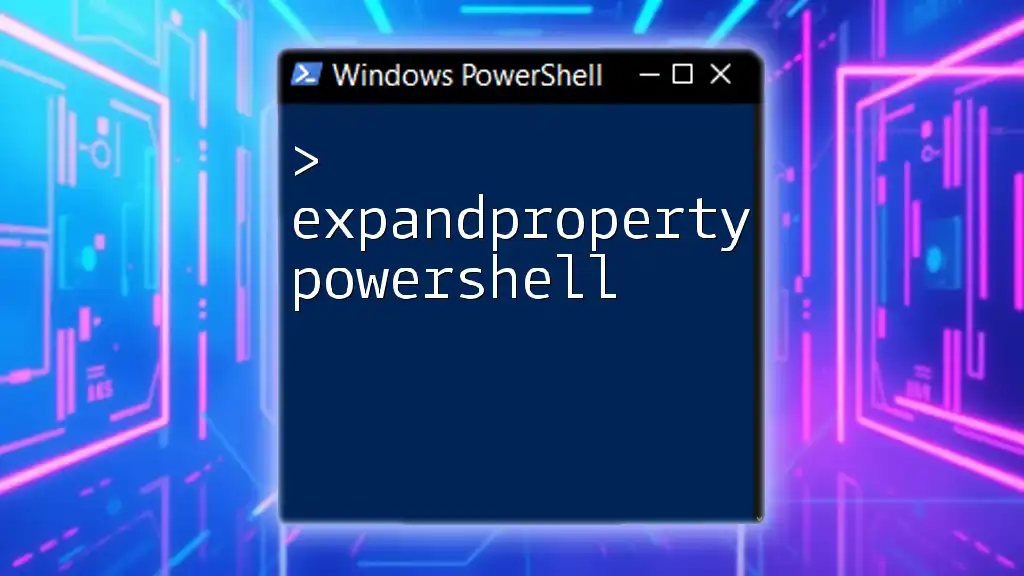
The Error: "Cannot Index Into a Null Array"
What Triggers the Error?
The error message "cannot index into a null array" appears when you attempt to access an element of an array that hasn’t been initialized or that is set to null. This commonly occurs in situations where you might be looping through an array or trying to access a specific index without confirming the array's existence.
Error Message Breakdown
When this error occurs, PowerShell informs you that the array you are trying to access is null. Such a message serves as a cue to check the array's initialization and assignment status.
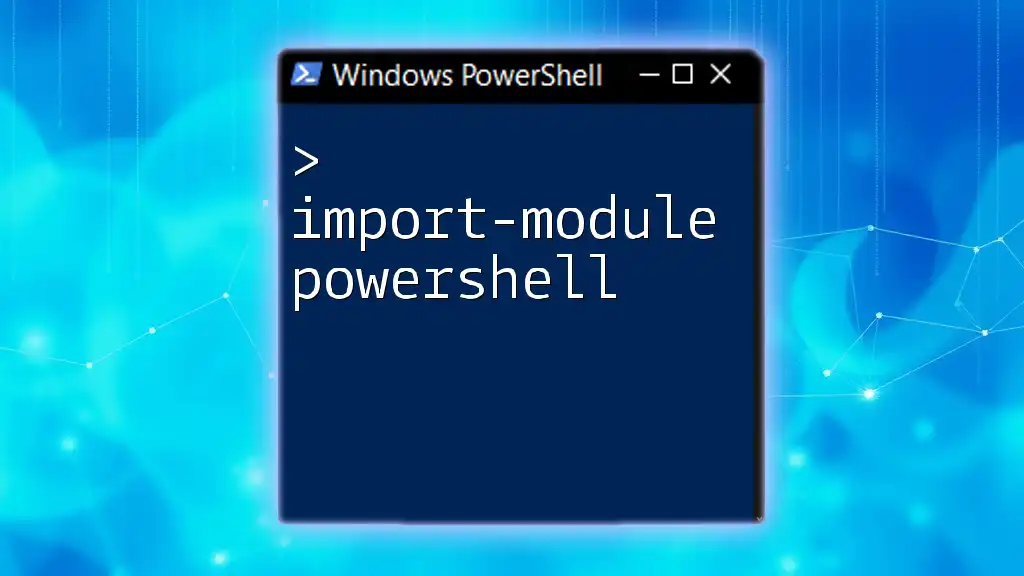
Troubleshooting the "Cannot Index Into a Null Array" Error
Identifying Null Arrays
When troubleshooting the error, the first step is to identify whether your array is null. You can use the `GetType()` method to inspect your variable:
$array = $null
if ($array -eq $null) {
Write-Host "The array is null"
}
This check is crucial to understanding the state of your variable before you attempt any operations on it.
Checking for Array Initialization
Always ensure your arrays are properly initialized. Here’s a way to safely create an empty array:
$array = @() # This creates an empty array
This establishes a baseline that can prevent the cannot index into a null array error later on.
Validating Array Contents Before Accessing
A best practice is to validate that your array holds values before trying to access them. Consider this approach:
$array = @()
if ($array.Length -gt 0) {
$item = $array[0]
} else {
Write-Host "Array is empty or null."
}
By checking the length, you can ensure that you’re not trying to index an empty or null array.
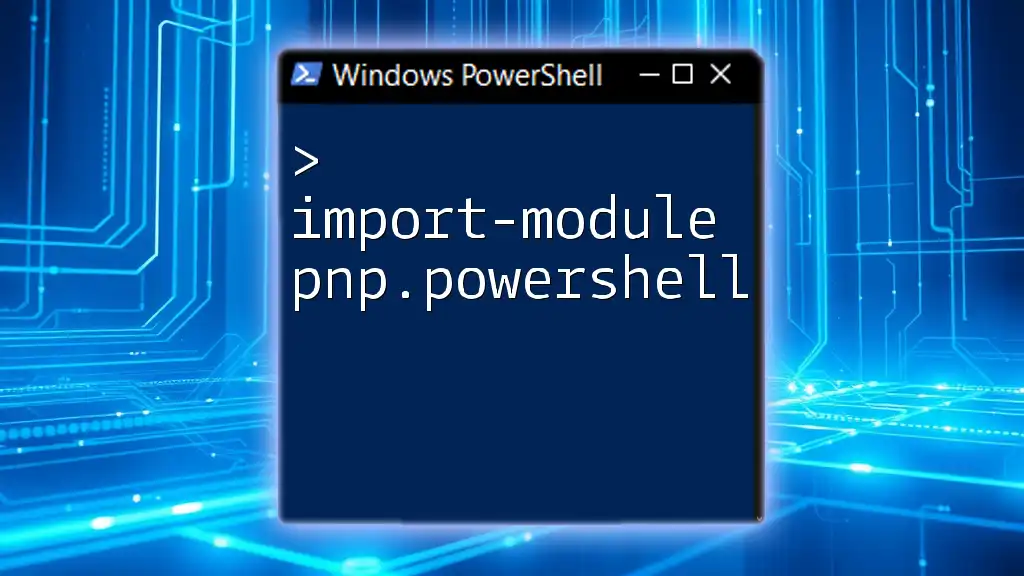
Common Scenarios Leading to the Error
Loop Constructs with Uninitialized Arrays
Looping through uninitialized arrays is a common pitfall. Consider this problematic example:
foreach ($item in $array) {
Write-Host $item
}
If `$array` is null at this point, PowerShell throws the error. To fix this, always validate the array's state inside your loops:
foreach ($item in $array) {
if ($null -ne $item) {
# Execute code
}
}
Function Returns and Null Arrays
Another frequent cause of encountering the cannot index into a null array error is when a function does not return the expected array. For instance:
function Get-Array {
return $null
}
$result = Get-Array
if ($result -eq $null) {
Write-Host "Function returned a null array."
}
Always ensure your functions return valid array objects to avoid unexpected null references.
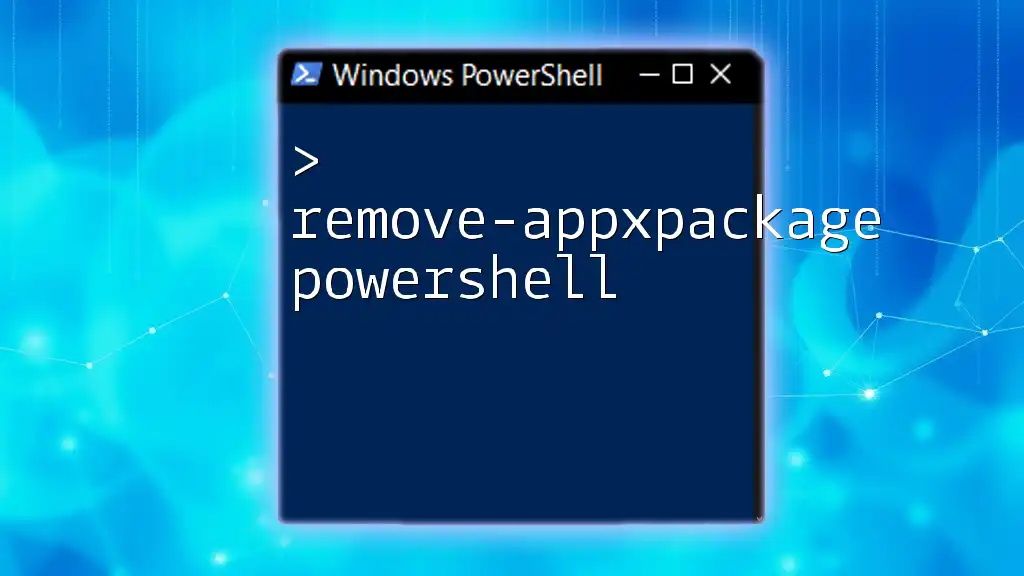
Best Practices to Avoid the Error
Initialize Arrays Properly
Proper initialization of arrays is essential to avoid indexing errors. Always use `@()` or explicitly define the array type:
$myArray = @(1, 2, 3) # Correctly initialized with values
By initializing arrays correctly, you ensure that they are ready for use in your scripts.
Always Validate Before Indexing
Implementing error handling with Try/Catch blocks adds robustness to your scripts. For example:
try {
$value = $myArray[0]
} catch {
Write-Host "An error occurred: $_"
}
This method helps you handle any unexpected issues gracefully.
Debugging Techniques for Null Arrays
Use PowerShell cmdlets like `Get-Member` and `Measure-Object` to debug arrays and verify their state. Additionally, create a logging strategy to monitor array conditions, thus helping you troubleshoot effectively throughout script execution.
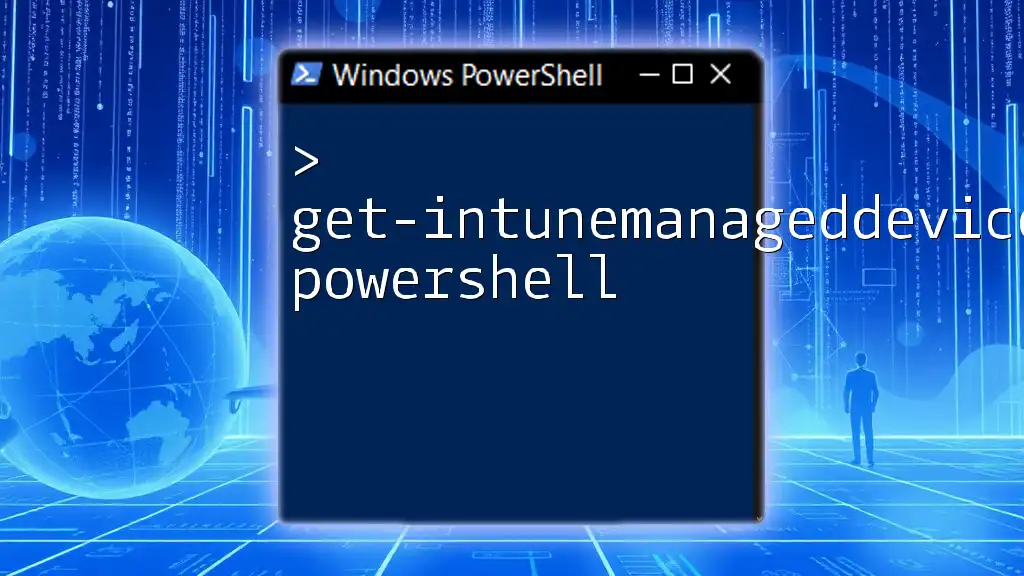
Conclusion
To recap, understanding the intricacies of arrays in PowerShell is vital to avoid the cannot index into a null array error. With proper initialization, validation checks, and debugging techniques, you can confidently manage arrays and harness their power in your PowerShell scripts. By practicing these concepts, you reinforce your learning, enhancing your proficiency in PowerShell and its commands.
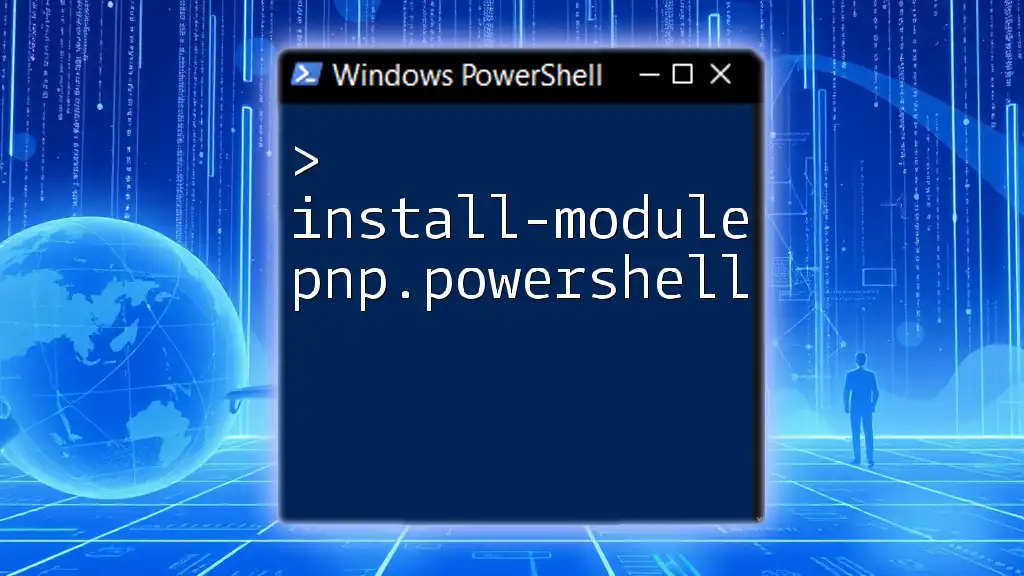
Further Reading and Resources
For more insights, consider exploring quality PowerShell documentation and relevant community forums. Engaging with the community can also provide valuable knowledge as you continue to refine your skills.