To search the Windows Registry using PowerShell, you can utilize the `Get-ItemProperty` cmdlet to retrieve specific keys and values based on a given path and search terms.
Here’s a code snippet to demonstrate this:
Get-ItemProperty -Path "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion" | Where-Object { $_.DisplayName -like "*search_term*" }
Replace `search_term` with the term you are looking for in the registry.
Understanding the Windows Registry
What is the Windows Registry?
The Windows Registry is a crucial database that stores low-level settings for the operating system and for applications that opt to use the Registry. These settings can include anything from user preferences to system configurations and installed software information. The Registry serves as a central hub that Windows and installed applications consult to retrieve or store settings.
Key Components of the Registry
To effectively search the registry in PowerShell, it’s important to understand its key components:
-
Hives: These are the top-level hierarchical data structures in the Registry, where each hive contains a specific set of keys. Common hives include:
- HKEY_LOCAL_MACHINE (HKLM): Contains configuration information for the operating system and installed applications.
- HKEY_CURRENT_USER (HKCU): Holds information related to the user currently logged into the system.
- HKEY_CLASSES_ROOT (HKCR): Provides information about registered applications, file types, and OLE (Object Linking and Embedding) information.
-
Keys: These are organizational units within hives containing values and can be thought of as folders that store data.
-
Values: These are the actual data held within keys, consisting of name-value pairs.
-
Data Types: Each value has a specific type, such as string, binary, DWORD (32-bit number), etc.
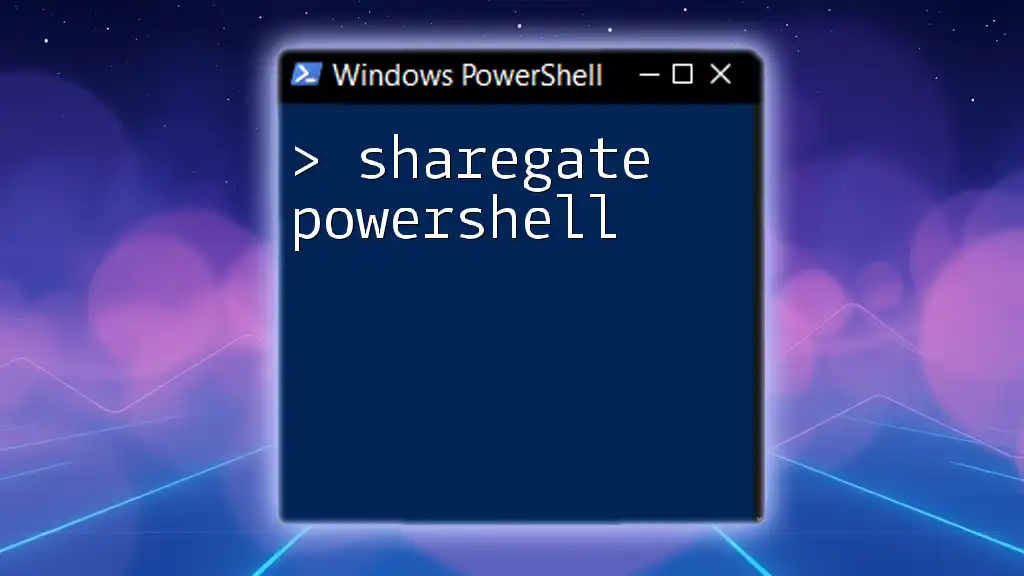
Getting Started with PowerShell
Why Use PowerShell for Registry Searches?
Using PowerShell for searching the Registry is not only powerful but efficient. It allows users to automate and execute complex tasks with just a few commands. Compared to other methods like using the Registry Editor, PowerShell provides a scripting interface that can quickly filter, modify, and process Registry data.
Setting Up Your PowerShell Environment
To begin, you'll need to open PowerShell. You can do this by:
- Pressing Win + X and selecting Windows PowerShell (Admin) for elevated permissions, which are often required when interacting with certain Registry keys.
- Alternatively, search for "PowerShell" in the Start menu.
Ensure you are comfortable with the PowerShell environment and have the necessary permissions to access the Registry.
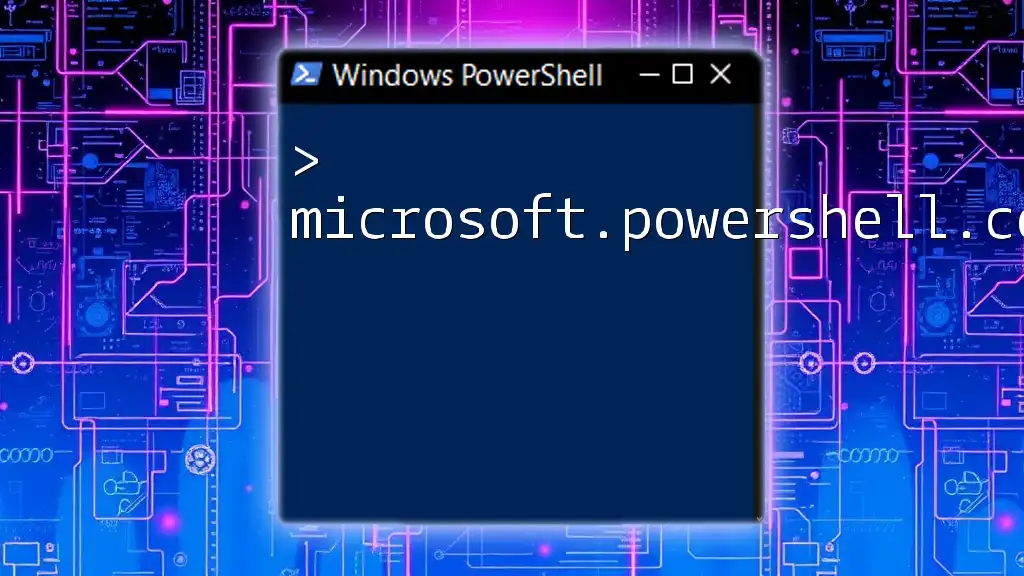
Searching the Registry with PowerShell
Introduction to the `Get-Item` and `Get-ItemProperty` Cmdlets
PowerShell provides several cmdlets for interacting with the Registry. Two of the most fundamental are `Get-Item` and `Get-ItemProperty`.
- Get-Item retrieves the specified Registry key.
- Get-ItemProperty retrieves the properties (or values) of the specified Registry key.
Here’s how you can use these cmdlets:
Get-Item 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE\YourKey'
Get-ItemProperty 'Registry::HKEY_CURRENT_USER\SOFTWARE\YourKey'
Using the `Get-ChildItem` Cmdlet
The `Get-ChildItem` cmdlet is essential when you want to list Registry keys and values.
To list all keys within a specific hive recursively, use the command:
Get-ChildItem -Path 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE' -Recurse
This command will display all subkeys and provide you with insight into the structure under the specified key.
Filtering Registry Searches with `Where-Object`
To filter the results returned by `Get-ChildItem`, you can utilize the `Where-Object` cmdlet. This is particularly useful when you are looking for specific keys or values.
For example, to search for specific keys:
Get-ChildItem 'Registry::HKEY_CURRENT_USER\SOFTWARE' | Where-Object { $_.Name -like '*TargetKey*' }
Searching for Specific Values
Searching for Strings
You might often need to search for string values in the Registry. Here’s how to achieve that:
Get-ChildItem 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE' | Where-Object { $_.GetValue('YourValueName') -eq 'YourTargetString' }
This command will search through the specified hive and check each key for the value name you provide, returning keys that match the target string.
Searching for Numeric Values
Similarly, if you're looking for numeric values, you can filter based on that as well:
Get-ChildItem 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE\YourKey' | Where-Object { $_.GetValue('NumericValue', 0) -gt 10 }
This command retrieves keys with a specified numeric value greater than 10.
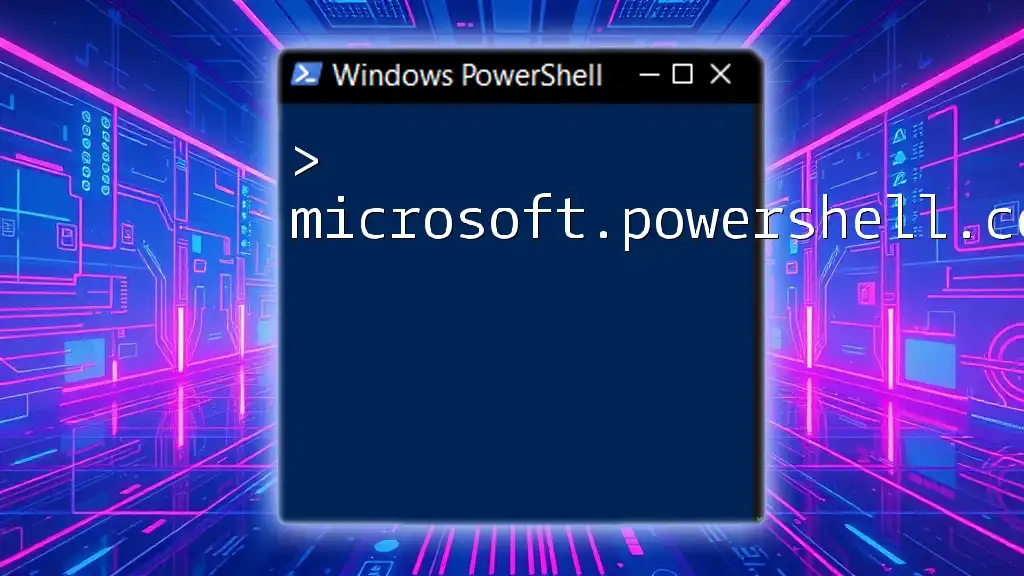
Advanced Registry Search Techniques
Using the `Select-Object` Cmdlet
After performing a search, you may want to see specific properties of the results. This is where `Select-Object` becomes handy, allowing you to specify which properties to display.
For example:
Get-ChildItem 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE\YourKey' | Select-Object Name, Property1
This command returns only the Name and Property1 of each matching Registry key, simplifying the output.
Exporting Search Results
PowerShell also enables you to export your search results for further analysis. For instance, you can export the results to a CSV file, making them easy to manage:
Get-ChildItem 'Registry::HKEY_LOCAL_MACHINE\SOFTWARE' | Export-Csv -Path 'RegistryData.csv' -NoTypeInformation
This exports the current list of Registry keys under `HKEY_LOCAL_MACHINE\SOFTWARE` into a file named `RegistryData.csv`.
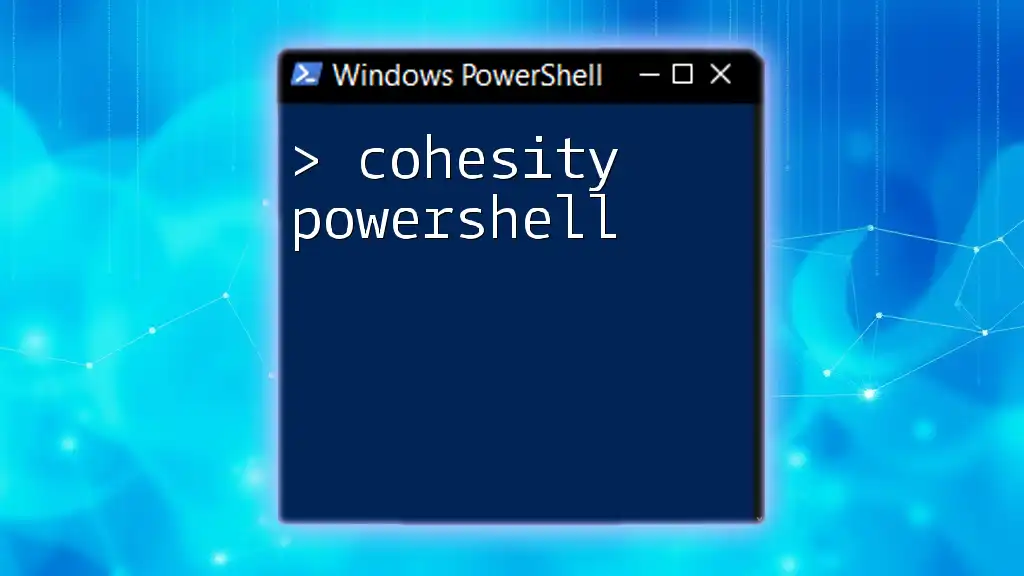
Troubleshooting Common Issues
Permission Denied Errors
One common issue that users encounter while searching the registry is permission denied errors. This typically occurs when the running user lacks the necessary permissions to read certain keys. To resolve this, ensure that PowerShell is running with administrative privileges.
Empty Search Results
Another frequent problem is receiving empty search results. This may happen for several reasons:
- Incorrect Registry Path: Always double-check that the path you are searching in is correct.
- Value Does Not Exist: If your search criteria do not match any existing value, it will yield no results.
Verifying your paths and ensuring the data you're searching for exists can help mitigate this issue.
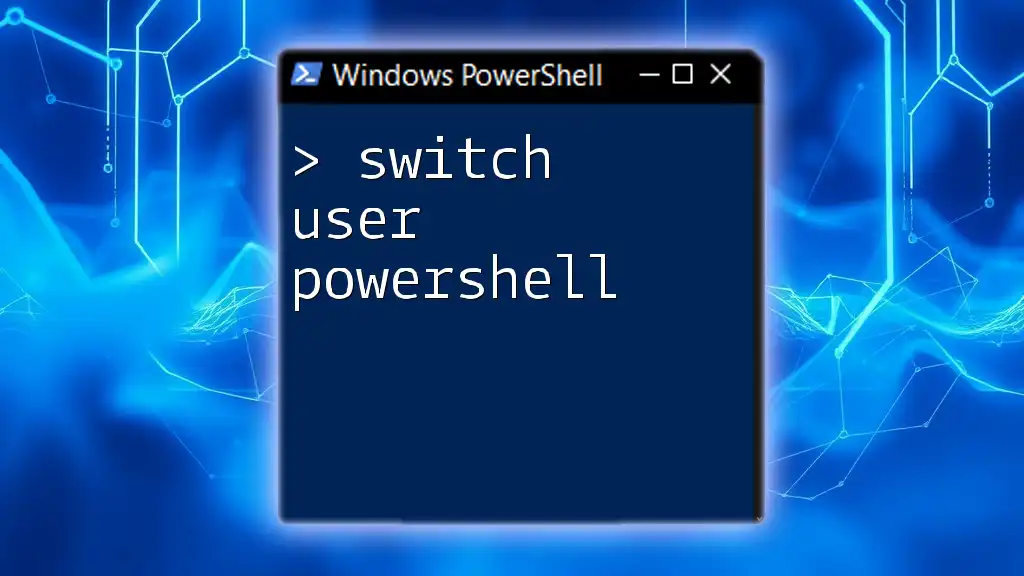
Conclusion
Leveraging PowerShell to search the Registry empowers users to automate and streamline their Windows administration tasks. By mastering these commands and techniques, you can efficiently navigate through the complex structure of the Windows Registry, thus enhancing your system management skills. Continue to practice reaching deeper into your understanding of PowerShell, and explore the wealth of possibilities that come with it.
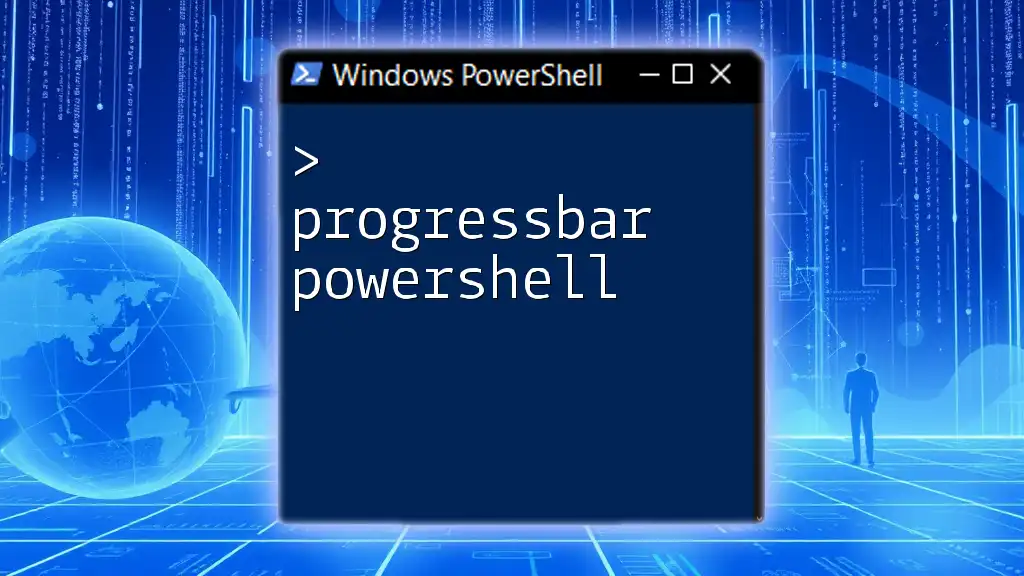
Additional Resources
For further learning, refer to the following resources:
- Microsoft PowerShell Documentation
- PowerShell community forums
- Recommended PowerShell books and online courses
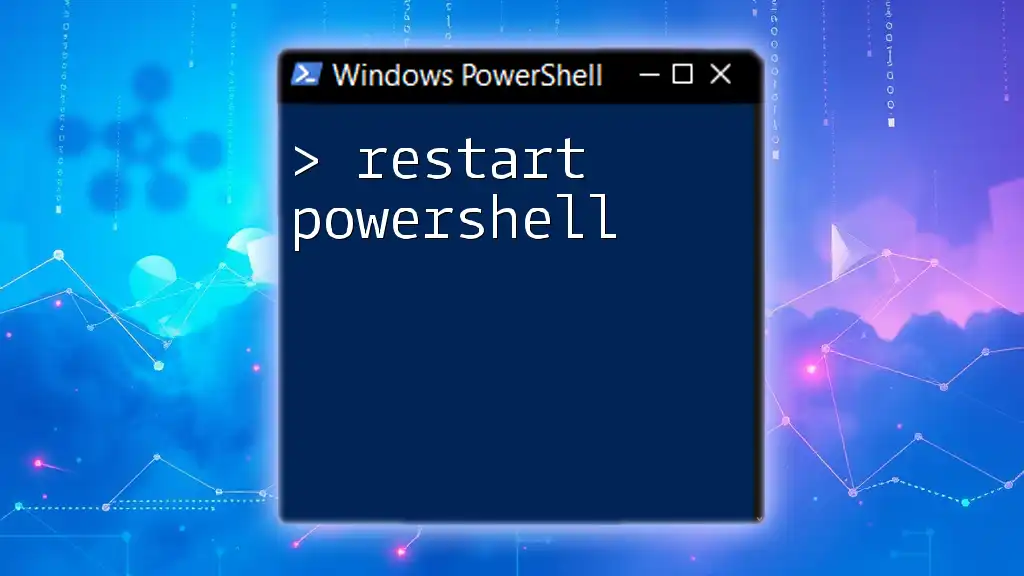
Final Thoughts
Harnessing the power of PowerShell allows for effective and efficient Registry management. By continuing to expand your knowledge and skills, you will not only streamline your processes but also become a proficient user of PowerShell in your daily tasks. Happy scripting!