You can easily batch convert DOC files to DOCX format using PowerShell with the following script:
$word = New-Object -ComObject Word.Application
$docFiles = Get-ChildItem -Path "C:\Path\To\Your\Docs" -Filter "*.doc"
foreach ($file in $docFiles) {
$doc = $word.Documents.Open($file.FullName)
$doc.SaveAs([ref] $file.FullName.Replace(".doc", ".docx"), [ref] 16) # 16 is the file format for docx
$doc.Close()
}
$word.Quit()
Understanding PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and shell designed for system administration. It's built on the .NET framework, which makes it versatile and able to interact seamlessly with various Windows components, making it a go-to tool for administrators.
PowerShell's Role in File Management
One of PowerShell's most significant advantages is its ability to automate routine tasks, including file handling. Whether it's creating, moving, or converting files, PowerShell allows for quick and efficient operations on multiple files, making it essential for anyone looking to streamline their workflow.
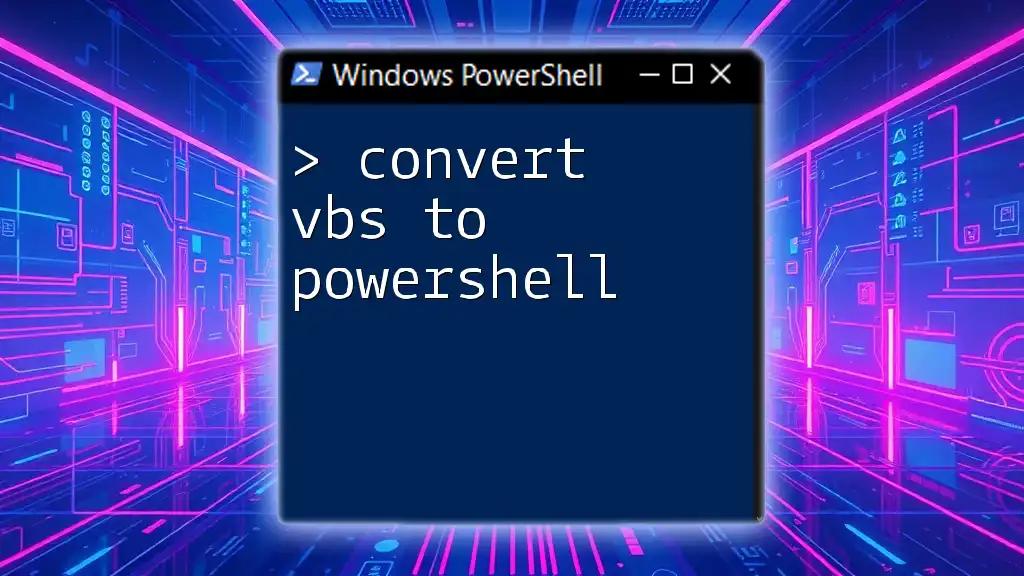
Getting Started with PowerShell for Document Conversion
Setting Up Your Environment
Before diving into the conversion process, ensure that PowerShell is installed on your system. It is typically available by default in modern versions of Windows.
Enabling Scripts in PowerShell
You'll also need to set your Execution Policy to allow scripts to run. You can do this by launching PowerShell as an administrator and executing the following command:
Set-ExecutionPolicy RemoteSigned
Understanding the Dependencies
For batch converting DOC to DOCX using PowerShell, you need Microsoft Word installed on your machine. PowerShell utilizes Microsoft's COM Objects to automate Word functionalities, so ensure you have access to the Word application.
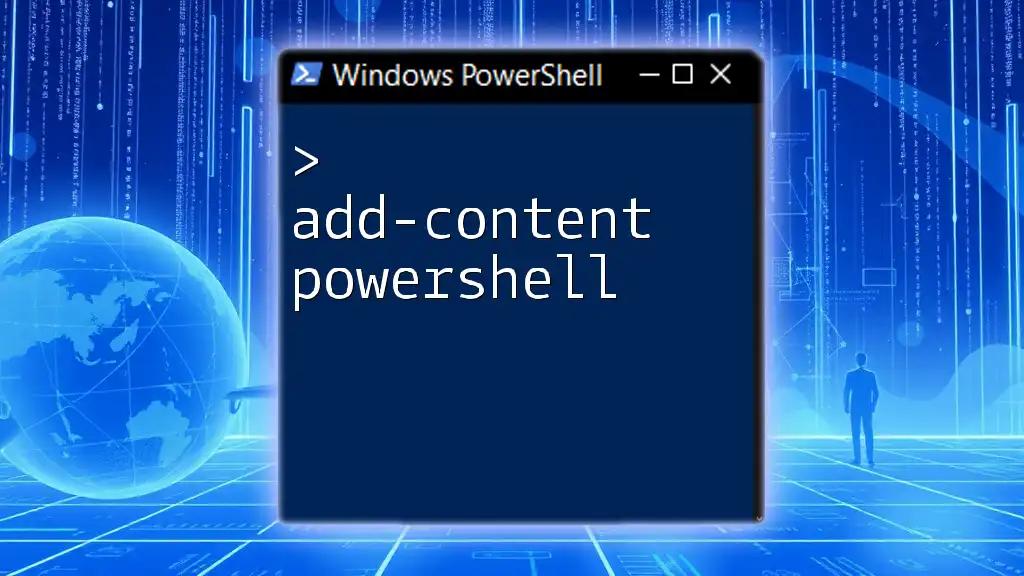
Preparing for the Conversion Process
Identifying the Source Files
First, determine the location of the DOC files you want to convert. It's best to keep these files in a dedicated folder to streamline the conversion process.
Example for Locating Files
$sourcePath = "C:\Documents\OldFiles"
Creating an Output Directory
It’s also crucial to create a separate directory for your converted DOCX files. This organization helps keep your files tidy and easy to navigate.
Example for Creating an Output Folder
$outputPath = "C:\Documents\ConvertedFiles"
New-Item -Path $outputPath -ItemType Directory -ErrorAction SilentlyContinue
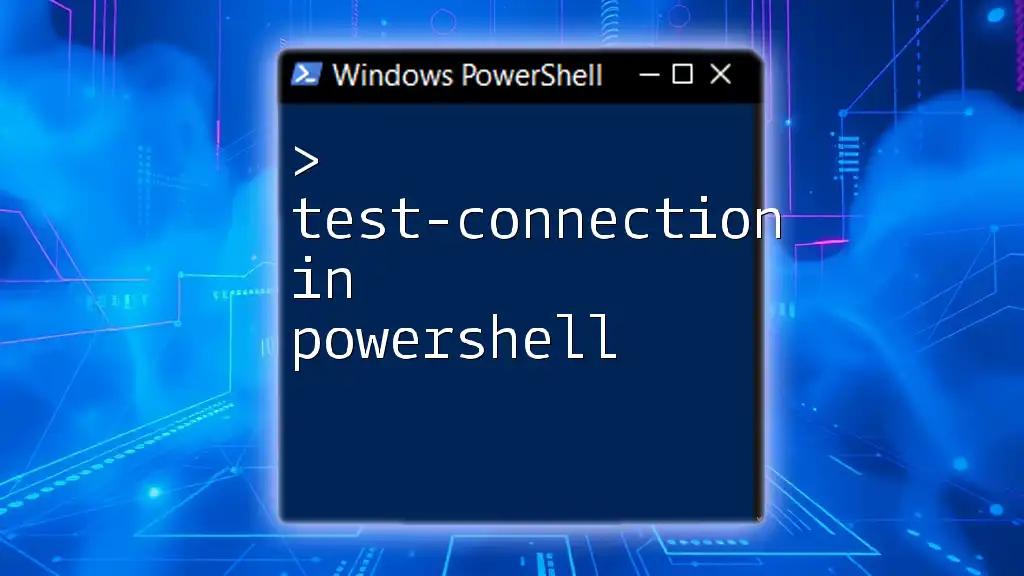
The Conversion Process
Using COM Objects for Conversion
PowerShell allows for interaction with Microsoft Word through COM Objects. This framework enables PowerShell to automate Word's functions, including file conversions from DOC to DOCX.
Example: Create the Word Application Object
$word = New-Object -ComObject Word.Application
$word.Visible = $false
Batch Converting DOC to DOCX
Once you have defined your source and output paths, you can begin the batch conversion process. PowerShell enables you to loop through each file in the specified directory and apply the conversion.
Example for Batch Conversion Loop
Get-ChildItem -Path $sourcePath -Filter *.doc | ForEach-Object {
$doc = $word.Documents.Open($_.FullName)
$newFileName = [System.IO.Path]::ChangeExtension($_.FullName, ".docx")
$doc.SaveAs([ref] $newFileName, [ref] 16) # 16 is the wdFormatXMLDocument
$doc.Close()
}
In this example, `Get-ChildItem` retrieves all DOC files from the specified path. For each document, PowerShell opens it, changes its extension to DOCX, and saves it in the output directory. The SaveAs parameter of `16` signifies the DOCX format.
Finalizing the Process
Once all documents are converted, it’s important to close the Word application to free up system resources.
Example to Close the Application
$word.Quit()
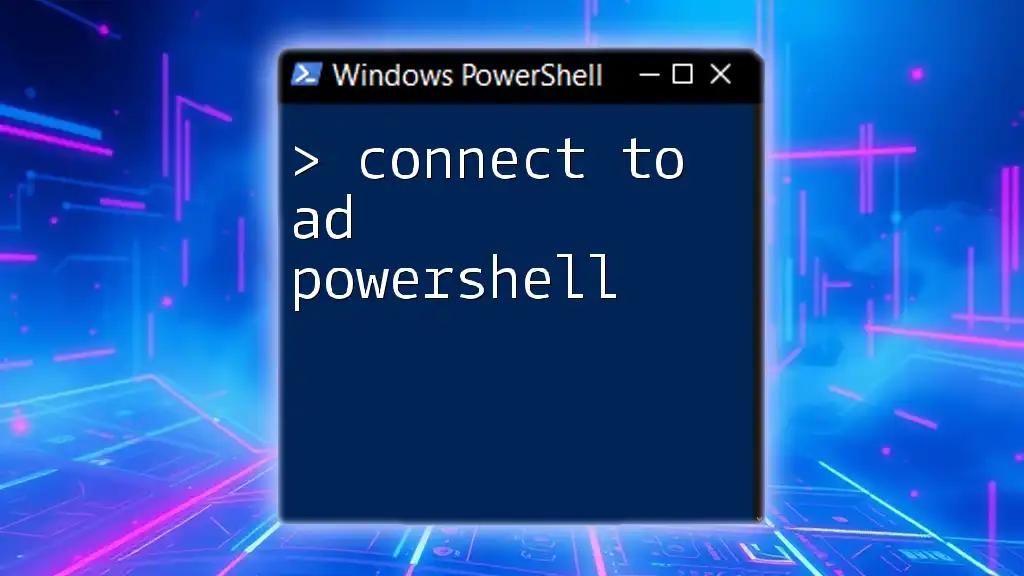
Error Handling and Best Practices
Common Errors and Troubleshooting
During the conversion process, it’s not uncommon to encounter errors. Some users may find that files are in use or that they have incompatible formats. If you experience a problem, check that no documents are open and ensure all files are valid DOC formats.
Best Practices for a Smooth Conversion
- Run as Administrator: When executing scripts, running PowerShell as an administrator can help avoid permission issues.
- Backup Your Files: To prevent data loss, always back up your original DOC files before running batch conversions.
- Test with a Few Files: Before launching a bulk conversion, test the process on a couple of files to iron out any issues.
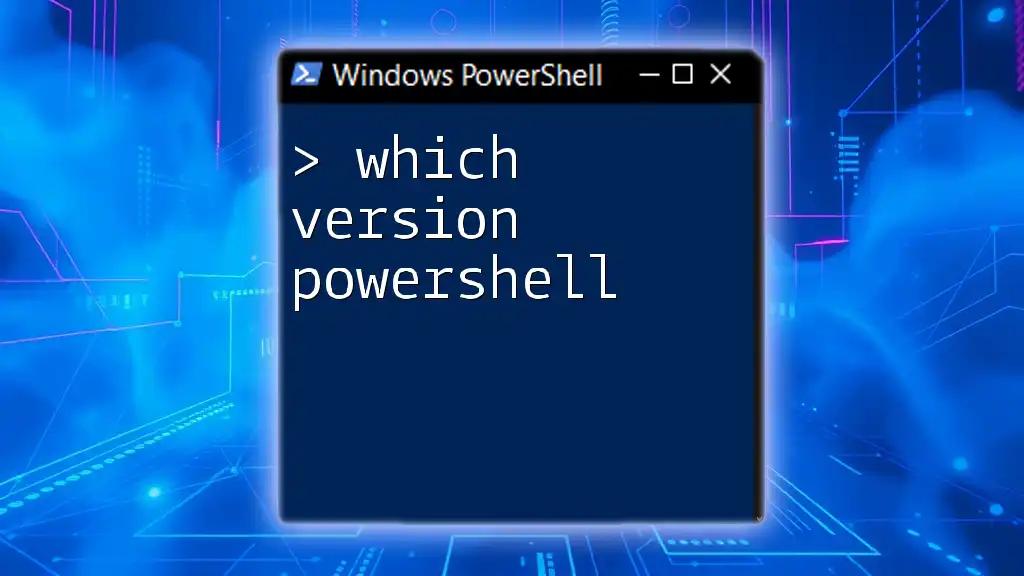
Conclusion
Using PowerShell to batch convert DOC to DOCX files significantly enhances your efficiency, saving time and reducing human error. PowerShell’s scripting capabilities provide an excellent platform for automating tedious tasks, such as file conversions.
Empower yourself by exploring more about PowerShell as you can streamline numerous other tasks in your daily workflow!
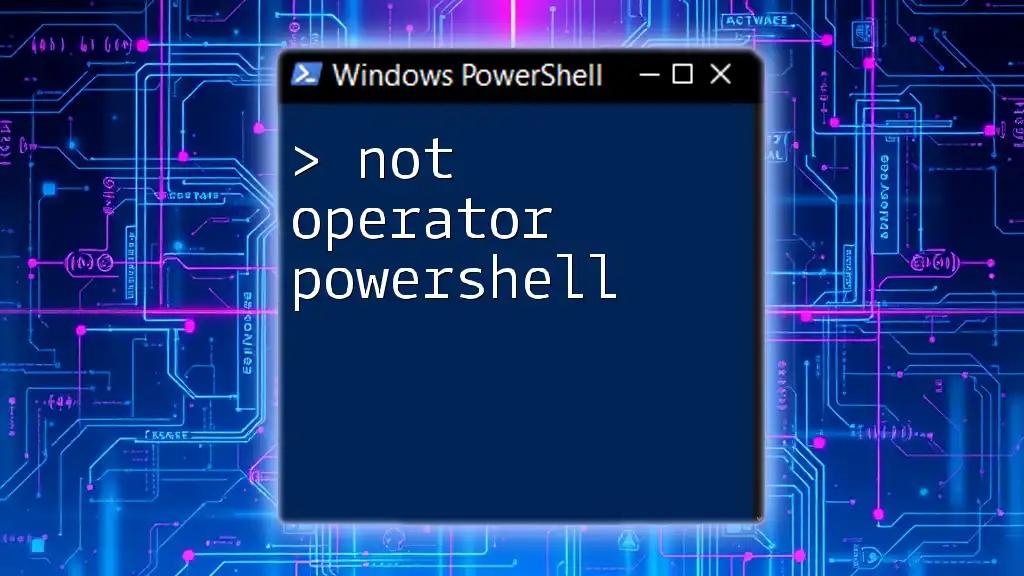
Additional Resources
For further knowledge, refer to the following resources:
- Microsoft Documentation on PowerShell and COM objects.
- Online forums and communities dedicated to PowerShell enthusiasts, where you can ask questions and share insights.
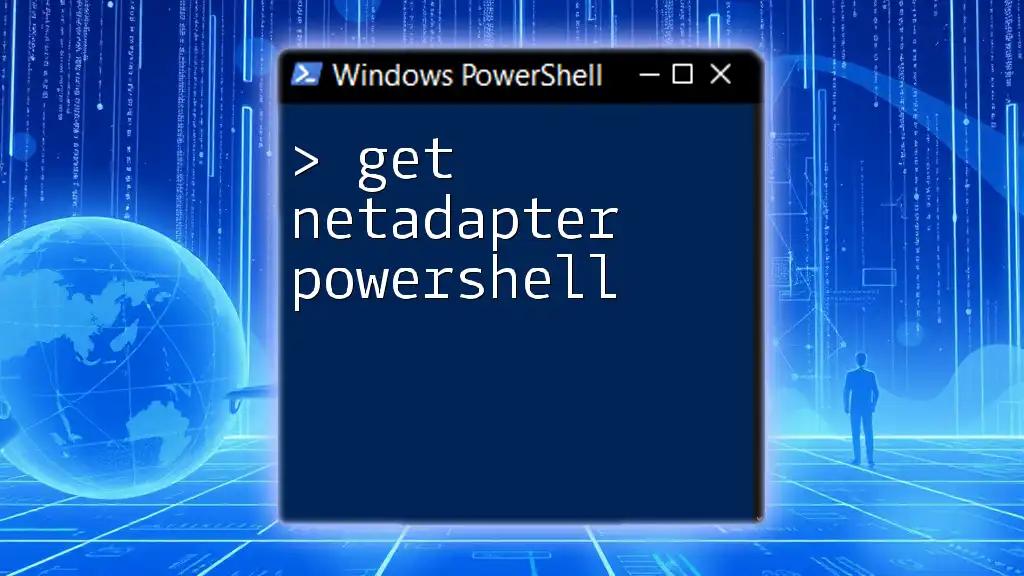
Call to Action
Join our community for more tips, tricks, and tutorials on mastering PowerShell commands. Whether you're an absolute beginner or looking to refine your skills, there's something here for everyone!