Converting VBScript to PowerShell involves translating the syntax and logic of the original script into PowerShell's format, allowing for more powerful and versatile automation.
Here's a simple example of a VBScript that outputs "Hello, World!" and its PowerShell equivalent:
VBScript
MsgBox "Hello, World!"
PowerShell
Write-Host 'Hello, World!'
Understanding the Basics
What is VBS?
Visual Basic Scripting (VBS) is a lightweight scripting language derived from Microsoft's Visual Basic. Often used for automating tasks in Windows environments, VBS allows users to manipulate the Windows file system, configure settings, and create simple scripts for repetitive tasks. Despite its capabilities, VBS has several limitations, especially in terms of data handling, error management, and integration with modern environments.
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language built on the .NET framework. Designed specifically for system administrators, PowerShell allows not just automation of tasks but also management of system resources, applications, and cloud services. With its object-oriented architecture, PowerShell can handle complex data types and integrate seamlessly with various systems, making it an essential tool for modern IT professionals.
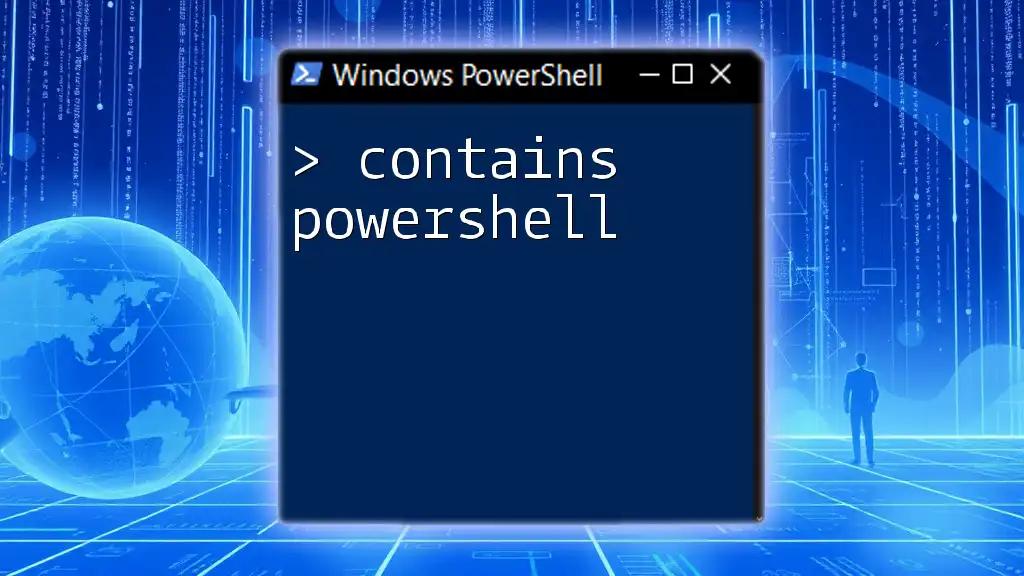
Key Differences Between VBS and PowerShell
Syntax Differences
The syntax in VBS differs significantly from that of PowerShell. For example, variable declarations in VBS use the `Dim` keyword, while in PowerShell, you can simply assign a value to a variable without a specific declaration format. Here’s a brief comparison:
VBS:
Dim myVariable
myVariable = "Hello, World!"
PowerShell:
$myVariable = "Hello, World!"
Data Handling
VBS primarily uses arrays and collections for data handling. It lacks advanced structures that PowerShell offers. For example, PowerShell supports hash tables, which allow for key-value pair storage that is not easily achieved in VBS.
PowerShell Example:
$myHashTable = @{'Name'='John'; 'Age'=30}
Error Handling
Error management in VBS relies on basic error handling with `On Error Resume Next`, which can lead to issues going unnoticed. In contrast, PowerShell provides robust error handling capabilities that allow you to handle exceptions with try/catch blocks, making scripts more reliable:
PowerShell Example:
try {
# Some code that may throw an error
} catch {
Write-Host "An error occurred: $_"
}

Tools to Convert VBS to PowerShell
Manual Conversion
Converting VBS scripts to PowerShell manually involves assessing the original functionality and rewriting the code using PowerShell syntax. Here are some best practices to ensure a smooth transition:
- Understand the Logic: Break down the VBS script into logical components—variables, loops, and conditionals.
- Test Incrementally: Convert and test each segment before moving on to the next to identify issues early.
Automated Conversion Tools
Although manual conversion is often necessary due to the contextual nuances in scripts, there are some automated tools that can assist. While a comprehensive tool might not exist for all VBS scripts, a popular tool is VBS2PS. Using it is straightforward; simply provide the VBS code, and the tool attempts to parse it into PowerShell format. Always review the output to ensure it aligns with your original script's intentions.
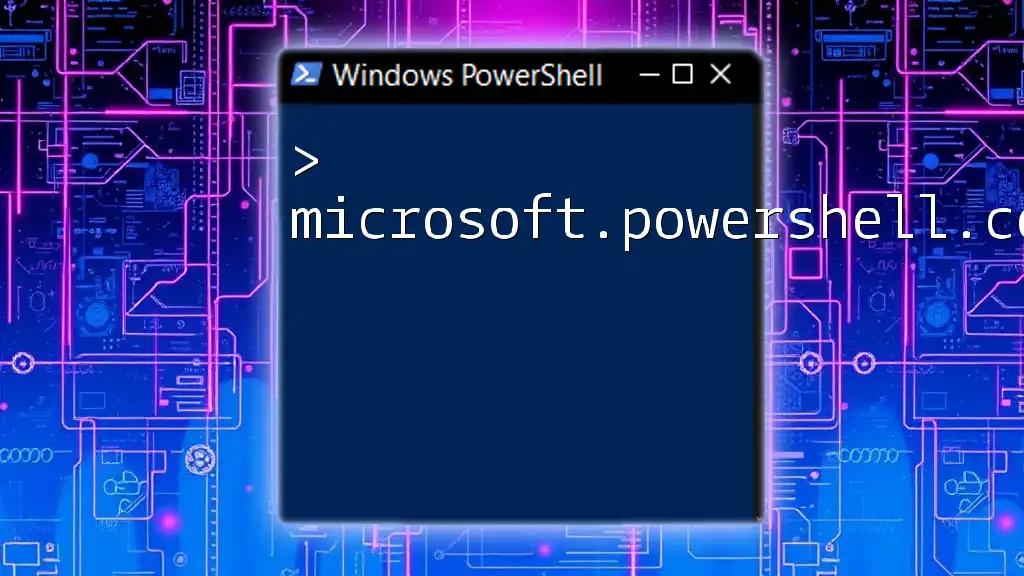
Step-by-Step Conversion Process
Analyzing the Existing VBS Script
Before diving into the conversion, it's crucial to analyze the existing VBS script comprehensively. Identify:
- Key functionalities you need to replicate in PowerShell.
- Any dependencies, such as COM objects, that might require alternatives in PowerShell.
Rewriting the Script in PowerShell
Here's a detailed, step-by-step approach to translating VBS commands to PowerShell:
Variables:
Dim myVar
myVar = "Sample"
becomes:
$myVar = "Sample"
Loops: A `For` loop in VBS:
For i = 1 To 5
MsgBox i
Next
becomes:
for ($i = 1; $i -le 5; $i++) {
Write-Host $i
}
Conditionals: A basic `If` statement in VBS:
If myVar = "Sample" Then
MsgBox "Matched"
End If
translates to:
if ($myVar -eq "Sample") {
Write-Host "Matched"
}
Testing the New PowerShell Script
After writing the PowerShell script, testing is vital to ensure functionality. Use `Write-Host` statements to trace outputs and validate each segment of your script. Leverage PowerShell's integrated debugging features to set breakpoints and inspect variables at runtime. This will help to identify common issues like variable scope errors or incorrect assumptions about data types.
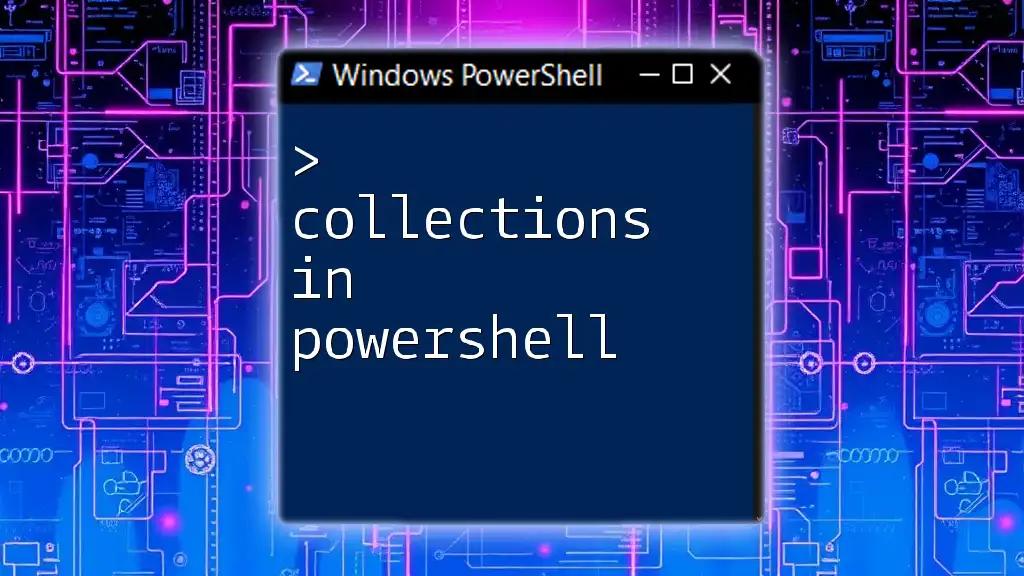
Example Conversion Guide
VBS Example Script
Consider a simple VBS script that generates a message box greeting the user:
Dim userName
userName = InputBox("Enter your name:")
MsgBox "Hello, " & userName
Converted PowerShell Script
Here's the equivalent script in PowerShell:
$userName = Read-Host "Enter your name"
Write-Host "Hello, $userName"
Notice how the `InputBox` in VBS has been replaced with `Read-Host`, which is a more suitable method for getting user input in PowerShell. This conversion simplifies the code while enhancing readability.
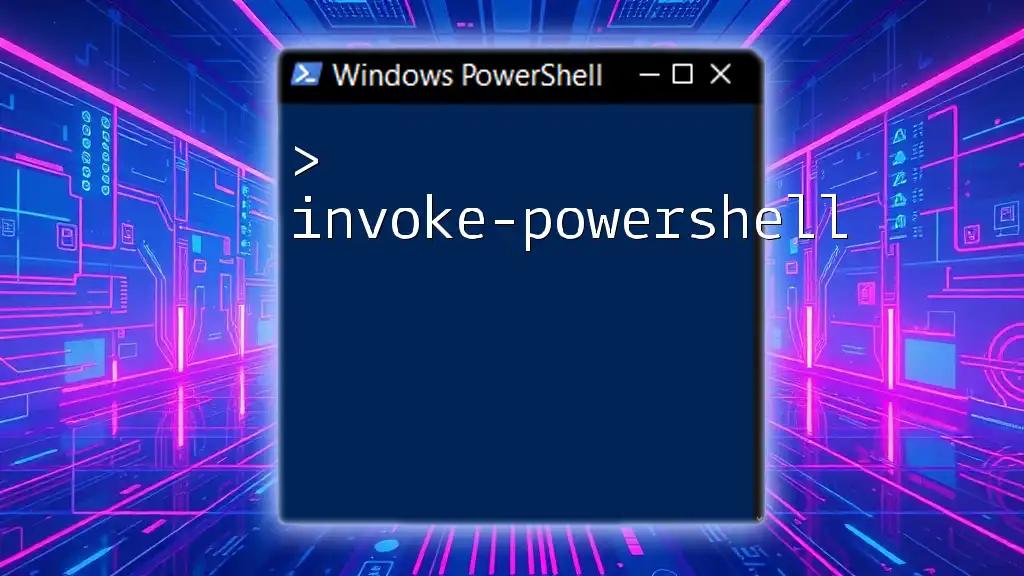
Best Practices for Writing PowerShell Scripts
Structuring Your Scripts
Always aim for a modular design by breaking down scripts into functions. This not only enhances readability but also promotes reusability in future projects. For example:
function Greet-User {
param ($name)
Write-Host "Hello, $name"
}
Documentation and Comments
Well-documented code can save time and effort in the long run. Use comments in your scripts to explain complex logic or to highlight important sections. A good practice is to use comments using `#` for single-line comments, and block comments can be done between `<#` and `#>`.
Version Control
Implementing version control is critical in managing your scripts effectively. Tools like Git allow you to track changes, collaborate with others, and roll back changes as necessary. Integrating version control into your routine can significantly improve your scripting workflow.
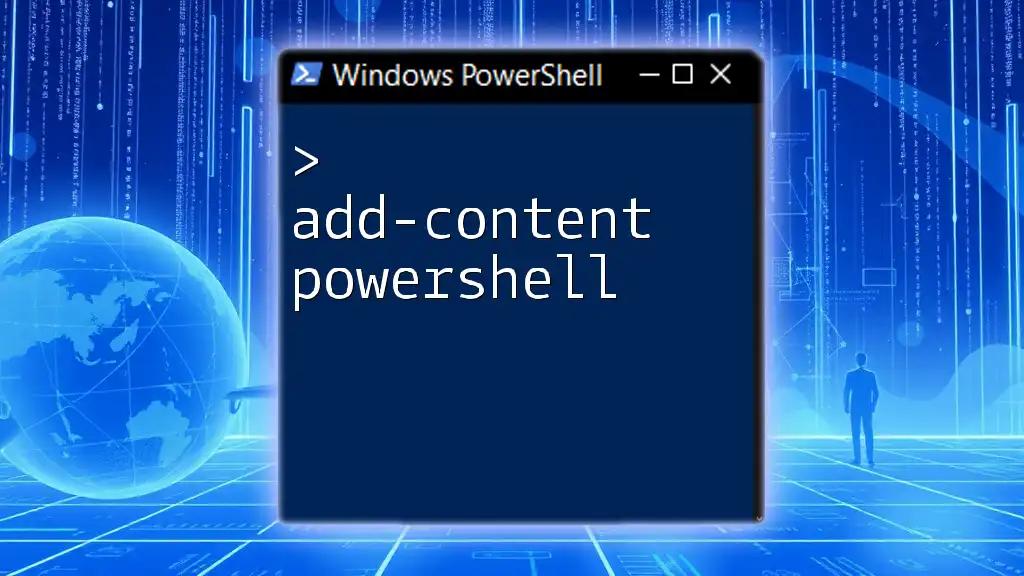
Conclusion
Converting VBS to PowerShell opens up a world of possibilities in terms of flexibility, efficiency, and modern capabilities. By understanding the key differences, utilizing appropriate tools, and following a structured conversion process, you can enhance your scripting skills and align your scripts with current technologies. As you practice and refine your newly acquired skills, you’ll discover greater efficiency in automating tasks and managing systems with PowerShell.
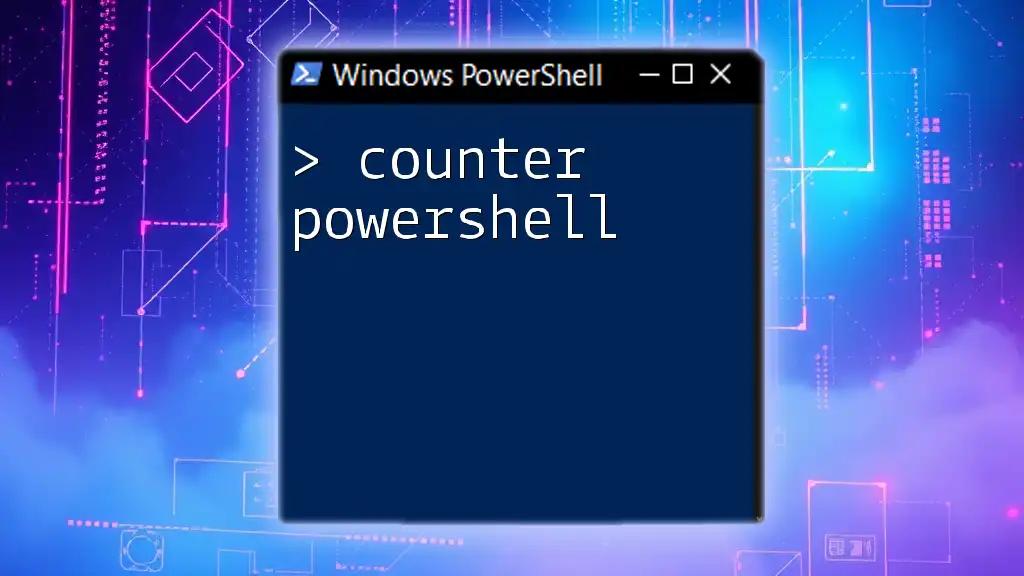
Additional Resources
For further learning, check out the official Microsoft PowerShell Documentation and explore forums and user groups for community support. There are also many study guides and books available that delve deeper into PowerShell scripting.
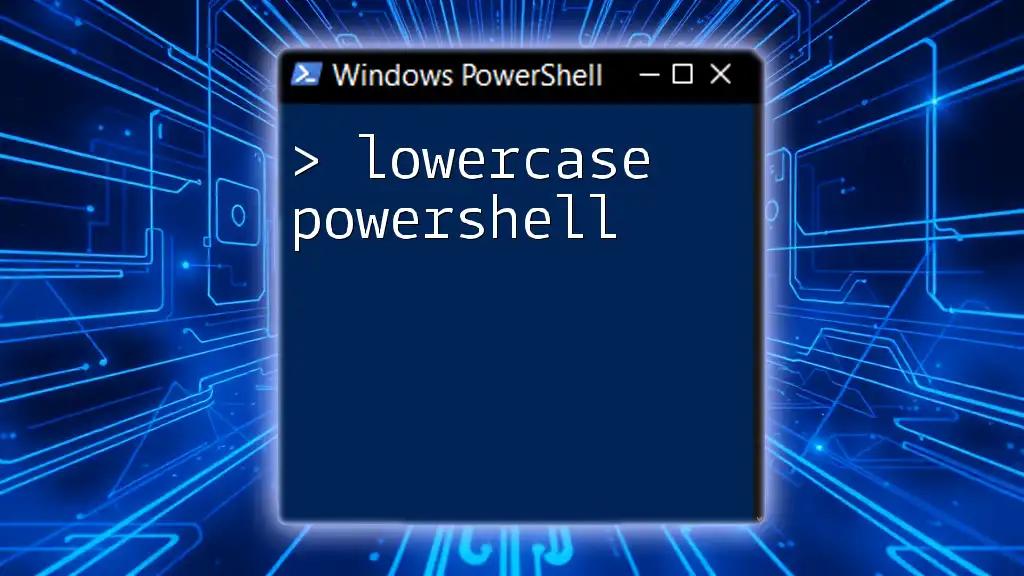
Call to Action
Subscribe for more insightful guides and tips to enhance your PowerShell knowledge. Join our courses or tutorials to unlock the full potential of PowerShell scripting!