To connect to Microsoft Graph using PowerShell, utilize the following command to authenticate and set up your session:
Connect-MgGraph -Scopes "User.Read.All", "Group.ReadWrite.All"
What is Microsoft Graph?
Microsoft Graph is a powerful tool that acts as a unified endpoint for accessing and interacting with a wide range of Microsoft services. From managing user accounts and mail to accessing OneDrive and Teams data, Microsoft Graph provides a streamlined approach to working with these services through a single API.
Using Microsoft Graph not only simplifies development but also enhances operational efficiency by enabling integration with various Microsoft 365 services. With this API, you can automate tasks, visualize data, and create applications that interact seamlessly with Microsoft products.
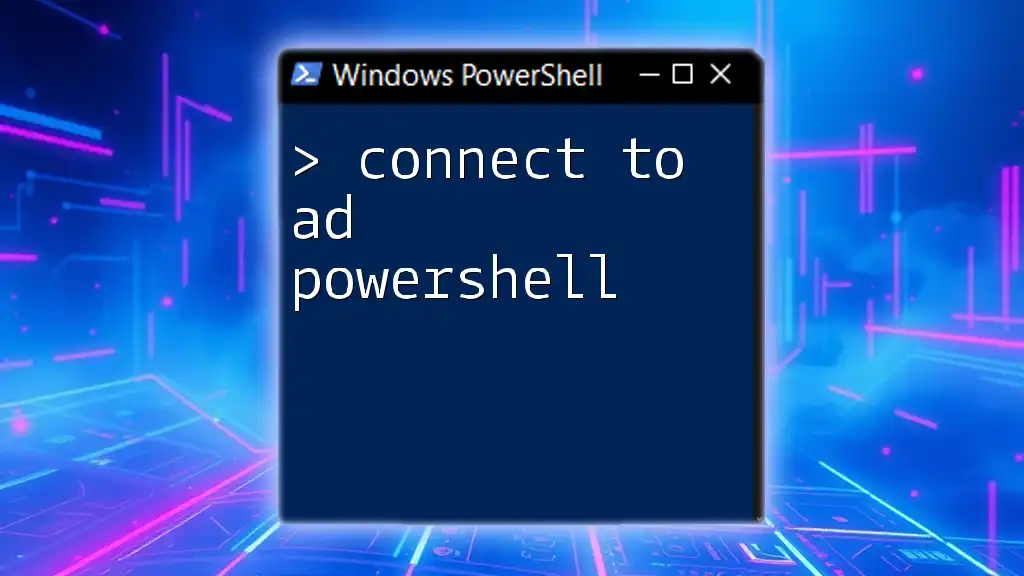
Benefits of Using Microsoft Graph
The benefits of Microsoft Graph are numerous:
-
Unified API Endpoint: Rather than having to interact with multiple APIs for different services, Microsoft Graph centralizes this interaction, simplifying development efforts.
-
Access Control and Permissions: Microsoft Graph allows for sophisticated access control mechanisms, letting you specify what data users can access and what actions they can perform.
-
Real-Time Data Access: The API provides real-time data updates and notifications, which can significantly improve the responsiveness of applications built atop Microsoft technologies.
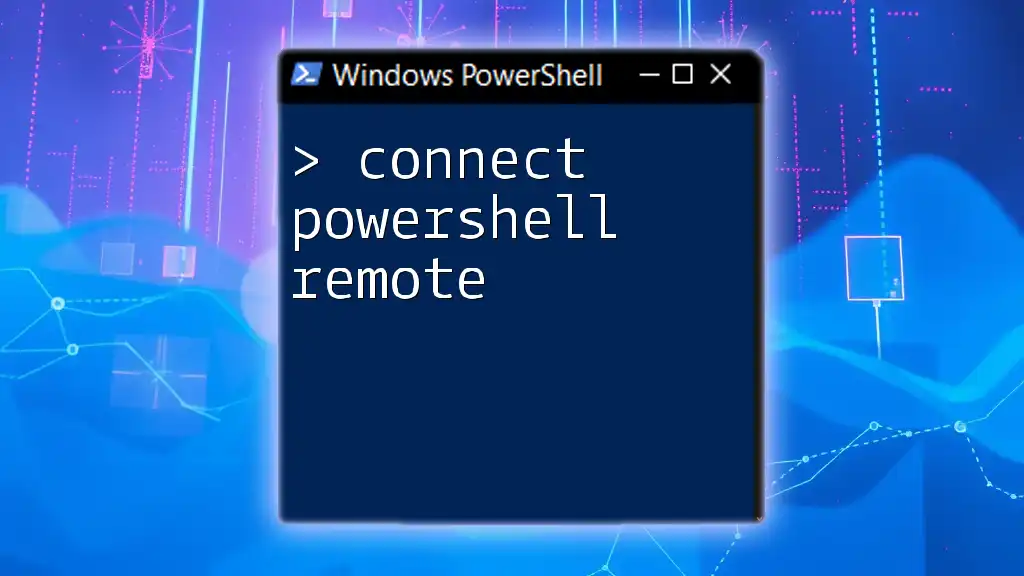
Getting Started with PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administration and automation. Unlike traditional command lines, PowerShell integrates with .NET and offers a systemic way to work with system utilities and applications.
Prerequisites for Using PowerShell with Microsoft Graph
Before delving into connecting to Microsoft Graph using PowerShell, ensure you meet the following prerequisites:
-
PowerShell Version: Make sure you are running an appropriate version of PowerShell (ideally, PowerShell 7 or newer) that supports the necessary cmdlets.
-
Installing PowerShell: If you aren’t on the right version, installing the latest PowerShell version on Windows, macOS, or Linux ensures compatibility and access to the latest features.
-
Microsoft Graph PowerShell SDK: This module offers a series of cmdlets specifically designed for Microsoft Graph, taking the hassle out of writing API calls.
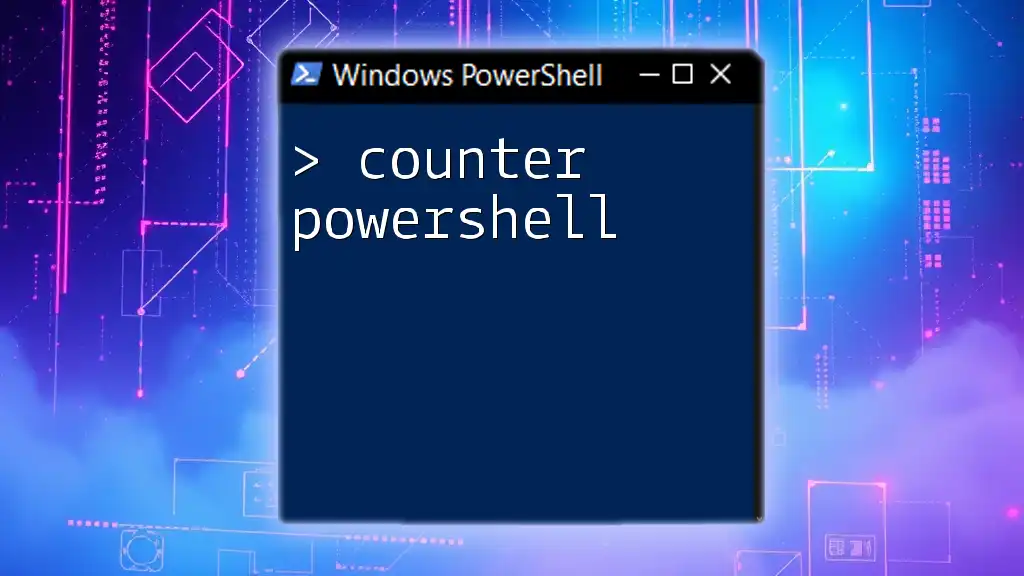
Installing the Microsoft Graph PowerShell SDK
Why Use the SDK?
Using the Microsoft Graph PowerShell SDK simplifies the process of interacting with Microsoft Graph. Unlike REST API calls that require familiarity with HTTP methods, the SDK abstracts these details away, allowing you to utilize straightforward cmdlets.
Step-by-Step Installation
To install the SDK, follow these steps:
-
Open PowerShell as an Administrator.
-
Execute the following command to install the SDK:
Install-Module Microsoft.Graph -Scope CurrentUser
-
Verify the installation with the following command:
Get-Module -Name Microsoft.Graph
By ensuring a successful installation, you're now prepared to interact with Microsoft Graph through PowerShell.
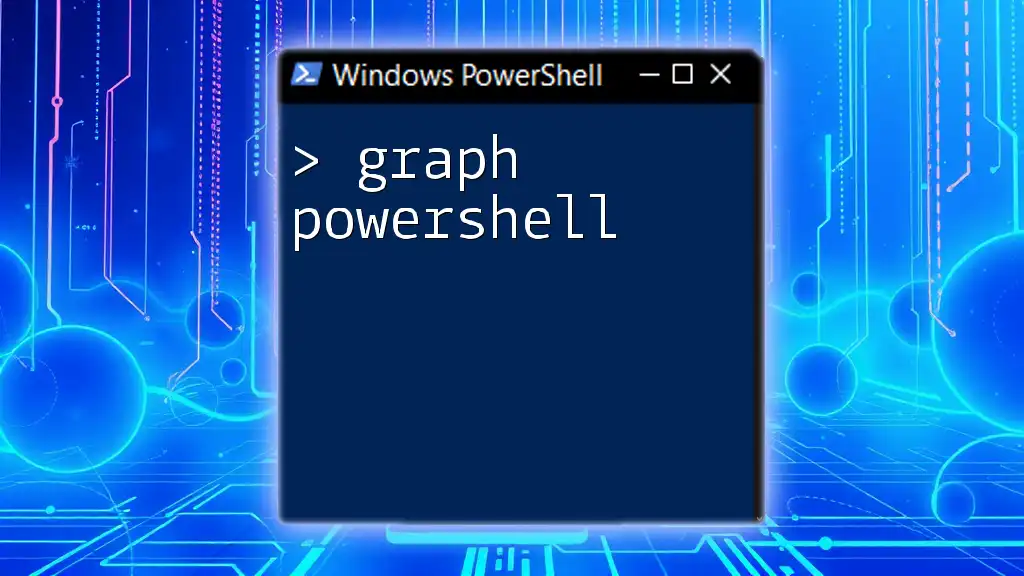
Authentication to Microsoft Graph
Understanding Authentication Flows
Authentication is a crucial aspect of accessing Microsoft Graph. Different authentication methods exist, including:
- Client Credentials: Ideal for application-to-service calls.
- Authorization Code Flow: Best suited for user-login scenarios.
Choosing the right flow depends on your use case—whether you need user interactivity or automated scripting.
Setting Up Azure AD App Registration
To connect to Microsoft Graph, you need to interact with an Azure Active Directory (AD) app. Follow these steps to set it up:
-
Create an Azure AD application:
- Log in to the Azure portal.
- Navigate to Azure Active Directory > App registrations > New registration.
- Fill in the necessary details like name and redirect URI, then click Register.
-
Configure API permissions:
- Under your app registration, go to the API permissions tab.
- Click on Add a permission, choose Microsoft Graph, and select the appropriate permissions, deciding between delegated and application permissions based on your need.
Connecting to Microsoft Graph
Using Device Code Authentication
To connect using the device code flow, follow this simple method:
Connect-MgGraph -Scopes "User.Read.All"
This initiates an interactive session where you'll authenticate via a user-friendly interface.
Using Client Credentials Authentication
For service-to-service interactions, authenticate using client credentials. You'll need your Client ID, Client Secret, and Tenant ID.
$clientId = "YOUR_CLIENT_ID"
$clientSecret = "YOUR_CLIENT_SECRET"
$tenantId = "YOUR_TENANT_ID"
$AppIdUri = "https://graph.microsoft.com"
$Token = (New-MgGraphAuthContext -ClientId $clientId -ClientSecret $clientSecret -TenantId $tenantId).GetToken()
Connect-MgGraph -AccessToken $Token
By utilizing this approach, you establish a backend connection that allows for non-interactive scripts and automation of tasks.
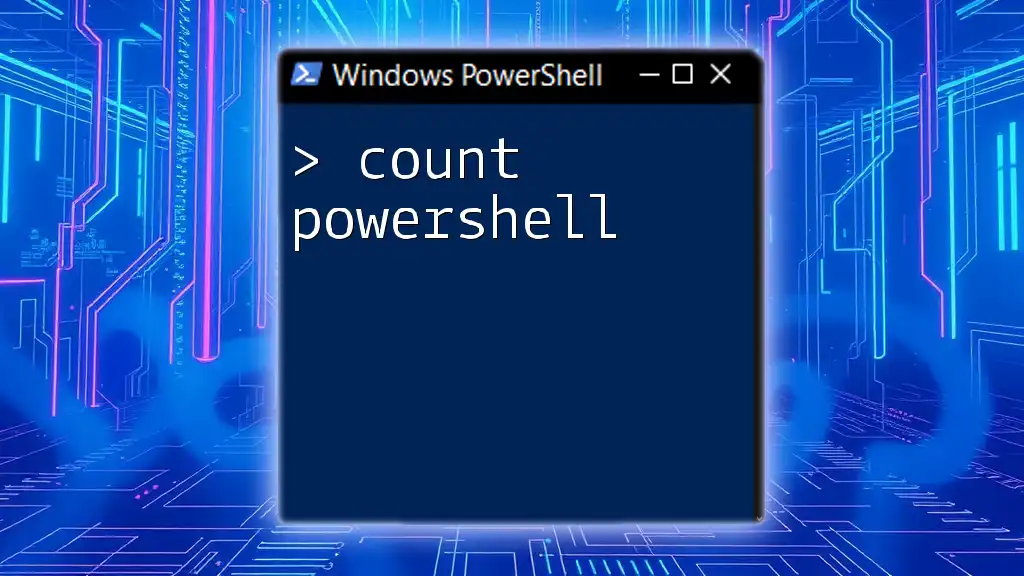
Common PowerShell Commands for Microsoft Graph
Listing Users
Getting a list of users in your organization is straightforward. You can accomplish this using the `Get-MgUser` cmdlet:
Get-MgUser -All
This command retrieves all users, making it invaluable for administrative tasks and user management.
Creating a New User
Adding new users to your directory involves utilizing the `New-MgUser` cmdlet. Here's an example of how to create a user:
New-MgUser -DisplayName "John Doe" -MailNickname "johndoe" -UserPrincipalName "johndoe@yourdomain.com" -AccountEnabled $true -PasswordProfile @{ForceChangePasswordNextSignIn=$true; Password="StrongPassword123"}
This command not only creates a user but also assigns a temporary password, requiring the user to change it upon their first sign-in.
Updating User Information
Should you need to modify user properties, PowerShell offers easy commands as well:
Update-MgUser -UserId "johndoe@yourdomain.com" -City "Seattle"
This snippet demonstrates updating a user's city information efficiently.
Deleting a User
For cases where you need to remove users, the `Remove-MgUser` cmdlet is essential:
Remove-MgUser -UserId "johndoe@yourdomain.com"
This command enables you to maintain your directory without unnecessary bloat.
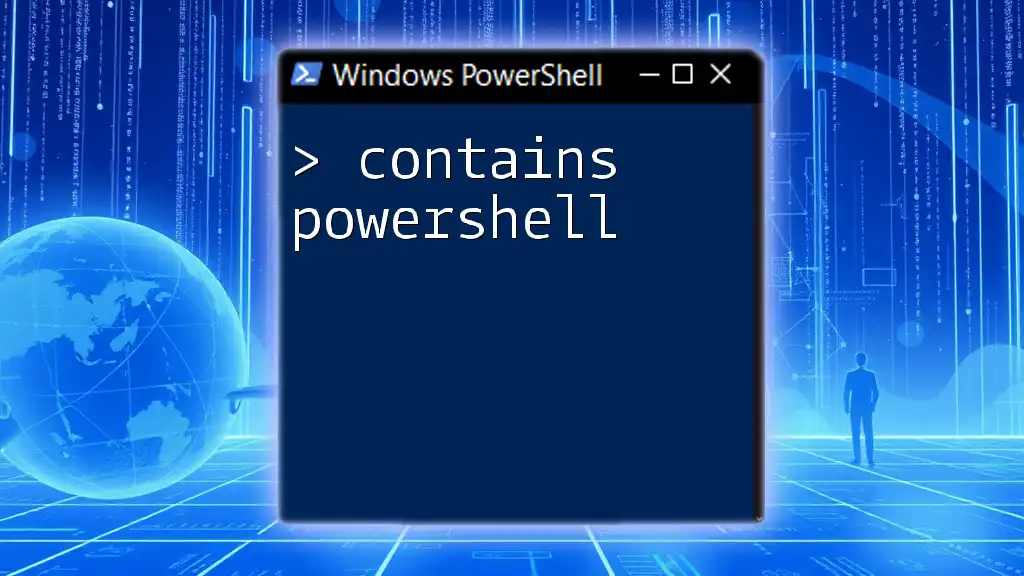
Troubleshooting Common Issues
Authentication Errors
If you encounter authentication errors, they often arise from incorrectly configured Azure AD settings, such as missing permissions or an inappropriate authentication flow. Double-check your app registration settings and consider enabling verbose logging to capture the underlying issues.
Permission Denied Errors
Receiving permission denied errors typically indicates insufficient privileges for the task at hand. Use the Azure AD portal to ensure that the application has been granted the needed API permissions, and remember to grant admin consent for application permissions.
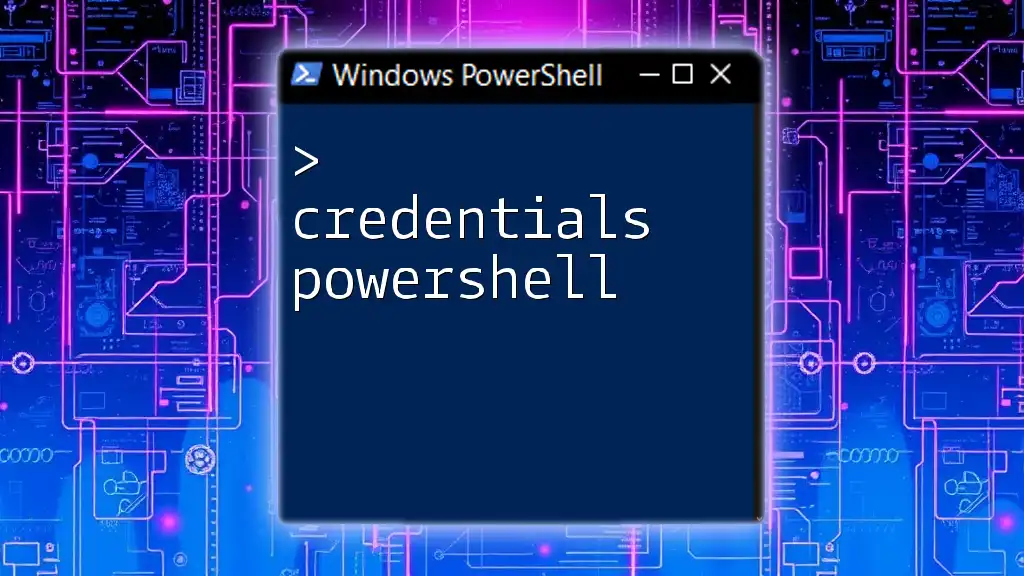
Conclusion
Connecting to Microsoft Graph using PowerShell is a valuable skill that unlocks tremendous potential for managing Microsoft 365 environments. Through this guide, you’ve learned about the benefits of Microsoft Graph, setup essentials, and fundamental commands for effective management.
As you explore further, you'll discover a wealth of functionalities ready to be harnessed in your everyday workflow. Dive into advanced features of the Microsoft Graph PowerShell SDK, and let automation streamline your administrative tasks.
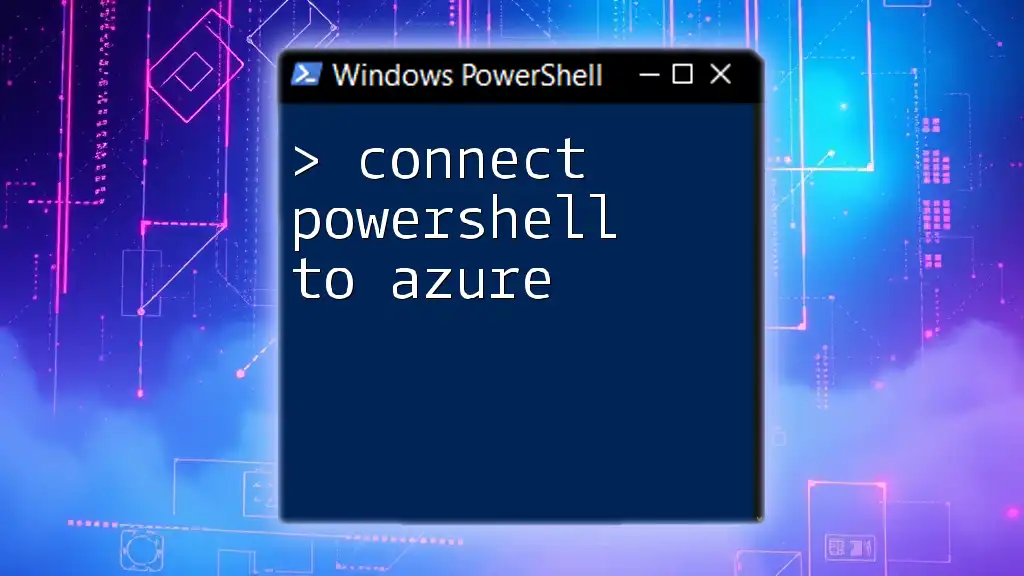
FAQs
What are the differences between Microsoft Graph and the legacy APIs?
Microsoft Graph offers a more cohesive approach to accessing Microsoft services than the older APIs, combining multiple endpoints into one while providing more consistent access and updated features.
Can I use PowerShell on a Mac or Linux?
Yes! PowerShell Core (PowerShell 7+) is cross-platform and can be utilized on macOS and Linux environments, expanding its utility beyond Windows systems.
Where can I find more information and documentation?
For further information, you can refer to the [official Microsoft documentation](https://docs.microsoft.com/graph/) and Microsoft PowerShell community forums to stay updated on best practices and recent enhancements.