You can easily run a batch file from PowerShell by using the `Start-Process` command followed by the path to the batch file.
Start-Process "C:\Path\To\Your\File.bat"
Understanding Batch Files and PowerShell
What is a Batch File?
A batch file is essentially a text file that contains a sequence of commands that are executed in order. These commands can include operations such as running applications, managing files, or performing complex tasks automatically. Batch files are commonly used to automate repetitive tasks in Windows environments. By saving a series of commands in a `.bat` file, users can execute them simply by double-clicking the file or running it from the command line.
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language designed specifically for system administration. It provides more advanced features compared to the traditional Command Prompt, including access to .NET Framework, the ability to use cmdlets (specialized commands), and robust scripting capabilities. PowerShell is ideal for automating tasks across a network and managing system configurations. Importantly, PowerShell can also run batch files, allowing users to leverage existing scripts within this modern framework.

Running a Batch File in PowerShell
Basic Command to Execute a Batch File
When it comes to running a batch file from PowerShell, the primary method involves using the call operator (`&`). This operator tells PowerShell to execute the command or script that follows. For example, if you want to run a batch file located in `C:\Path\To\Your\File.bat`, you would use the following command:
& "C:\Path\To\Your\File.bat"
Note the quotation marks; they are essential, especially if the path contains spaces.
How PowerShell Executes Batch Files
The call operator (`&`) is crucial for executing batch files directly. Without it, PowerShell might interpret the command differently or may not find the file at all. Specifying the complete path ensures that PowerShell locates the correct file for execution.
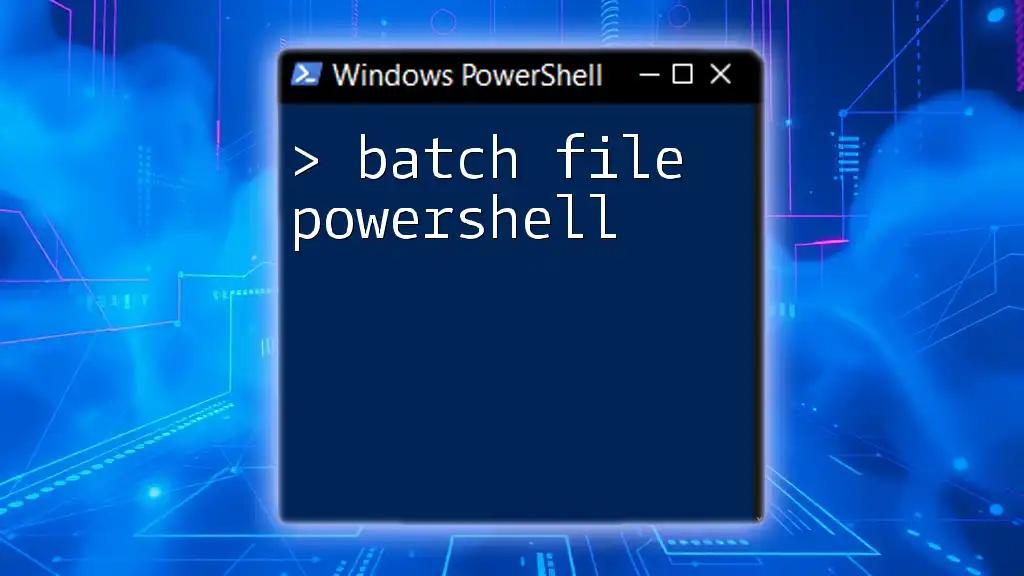
Options for Running Batch Files
Running Batch Files from the Current Directory
Sometimes, you may want to execute a batch file located in the current working directory. In this case, you can use a relative path. For example:
& ".\YourBatchFile.bat"
This will execute the batch file without needing to specify the full path, making it quicker when working within the script's folder.
Executing Batch Files with Parameters
Many batch files require parameters to function correctly, and PowerShell allows you to pass these parameters seamlessly. If your batch file needs two parameters, for example, you can run it like this:
& "C:\Path\To\Your\File.bat" "parameter1" "parameter2"
This provides flexibility and allows for dynamic execution based on your needs.
Running Batch Files as Administrator
In some cases, batch files require elevated permissions to execute properly. To run a batch file as an administrator, PowerShell's `Start-Process` cmdlet comes into play. Here's how to do it:
Start-Process "C:\Path\To\Your\File.bat" -Verb RunAs
This command prompts the User Account Control (UAC) dialog, allowing you to approve elevated permissions before execution.
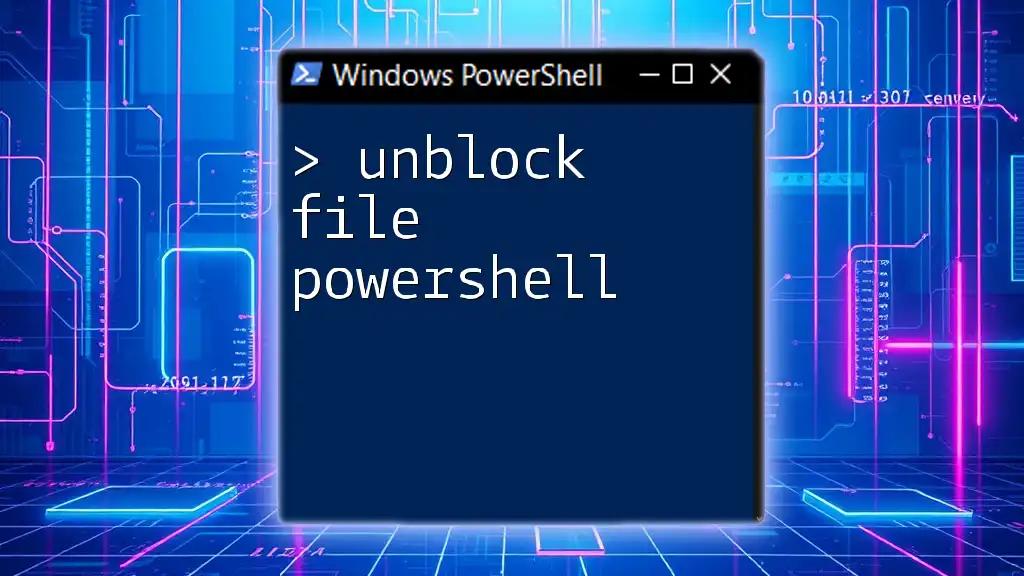
Best Practices for Running Batch Files in PowerShell
Checking if a Batch File Exists
Before executing a batch file, it is prudent to check if the specified file exists. This will prevent unnecessary errors during execution. You can use the `Test-Path` cmdlet for this purpose:
if (Test-Path "C:\Path\To\Your\File.bat") {
& "C:\Path\To\Your\File.bat"
} else {
Write-Host "File not found."
}
This snippet ensures that the batch file is only executed if it exists, providing a safety check.
Error Handling in PowerShell
When executing batch files, especially in larger scripts, error handling becomes vital. PowerShell offers `Try-Catch` blocks that allow you to manage errors gracefully. Here’s an example:
try {
& "C:\Path\To\Your\File.bat"
} catch {
Write-Host "An error occurred: $_"
}
This setup captures any errors that occur during the execution of the batch file and provides informative messages, enhancing script reliability.
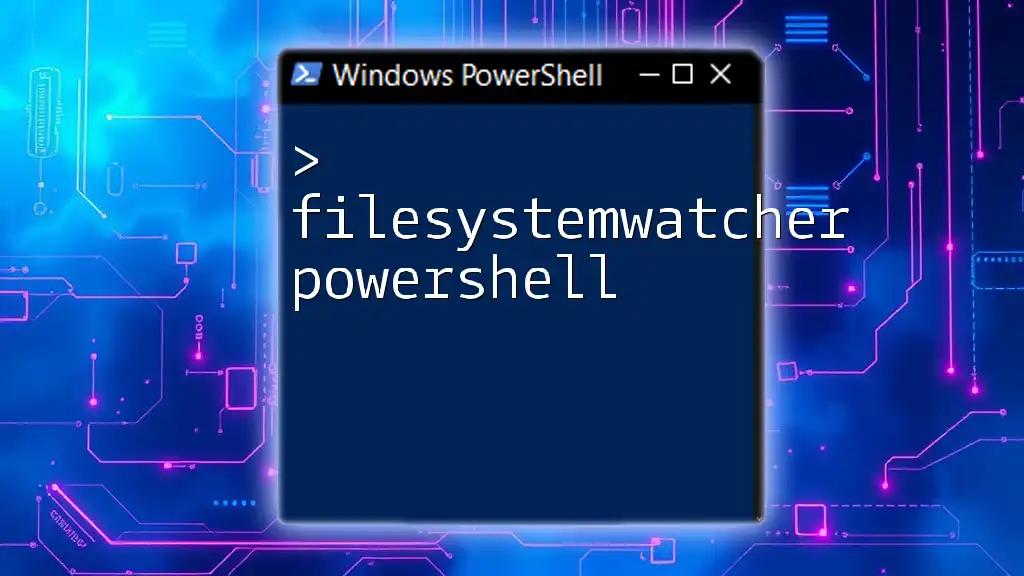
Advanced Techniques
Running Batch Files from PowerShell Scripts
You can integrate batch file execution directly within PowerShell scripts. This is a powerful way to combine the scripting capabilities of PowerShell with existing batch processes. For example, a simple PowerShell script might look like this:
# Sample PowerShell script
Write-Host "Starting batch file execution..."
& "C:\Path\To\Your\File.bat"
Write-Host "Batch file execution completed."
In this script, you can include additional logic and commands that complement the batch file's functionality.
Scheduling Batch File Execution using PowerShell
PowerShell can also help automate the scheduling of batch file executions using the Task Scheduler. You can create a scheduled task that runs your batch file at specified intervals, effectively automating repetitive tasks. This can be accomplished via the `New-ScheduledTask` cmdlet in PowerShell. Consult the official documentation for further details on setting up these tasks.
Combining Batch Files with PowerShell Functionality
Leveraging both batch files and PowerShell scripts can yield powerful results. For instance, consider a scenario where a batch file processes data, and PowerShell gathers metrics on that data. By running the batch file from PowerShell and then using PowerShell commands to handle the output, you can create a robust data processing pipeline.
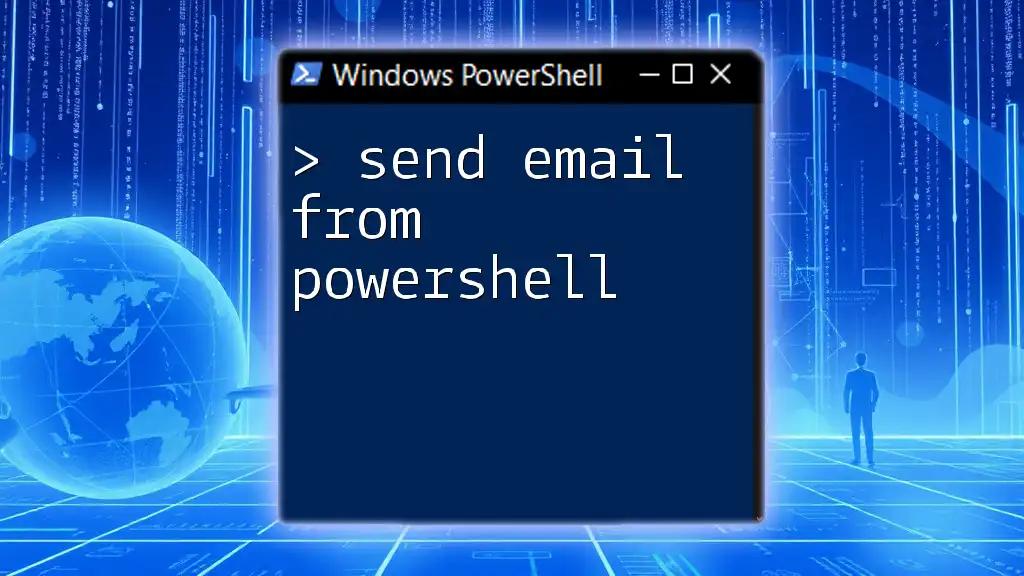
Conclusion
Running batch files from PowerShell can open up new avenues for automation and system management. By understanding how to utilize the `&` operator, manage parameters, and incorporate error handling, you can enhance both your PowerShell scripts and your overall productivity. Whether you're a seasoned administrator or just exploring the world of scripting, mastering the execution of batch files within PowerShell is a valuable skill.
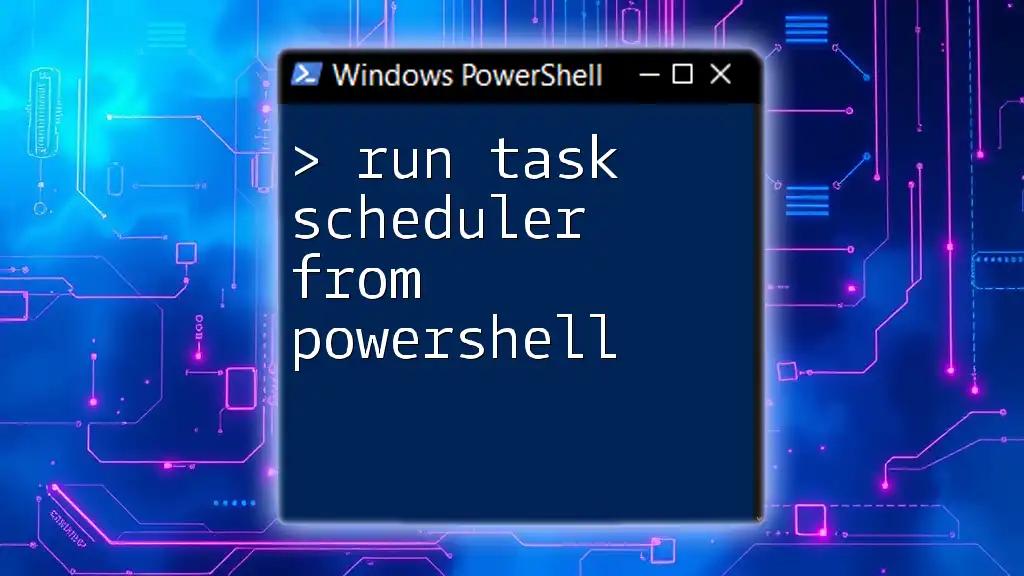
Call to Action
Explore additional resources to deepen your knowledge of PowerShell and batch file execution. Consider signing up for our newsletter or enrolling in our PowerShell mastery course to enhance your scripting skills!