Converting a batch file to PowerShell involves translating the simple command syntax used in batch files to the more powerful and flexible syntax of PowerShell, as shown in this example:
# Batch file command
echo Hello, World!
# Converted PowerShell command
Write-Host 'Hello, World!'
Understanding Batch Files
What Is a Batch File?
A batch file is a text file containing a sequence of commands to be executed by the command-line interpreter in Windows. Its primary purpose is to automate repetitive tasks by executing a set of commands in order. Batch files are commonly used for system administration, setting up environment variables, and managing file operations.
Structure of a Batch File
The structure of a batch file consists of commands, variables, control structures, and execution commands. Here’s a simple example of a batch file:
@echo off
echo Hello, world!
pause
In this example, `@echo off` prevents the commands from being displayed in the terminal, `echo` prints the message on-screen, and `pause` halts the execution until the user acknowledges it.
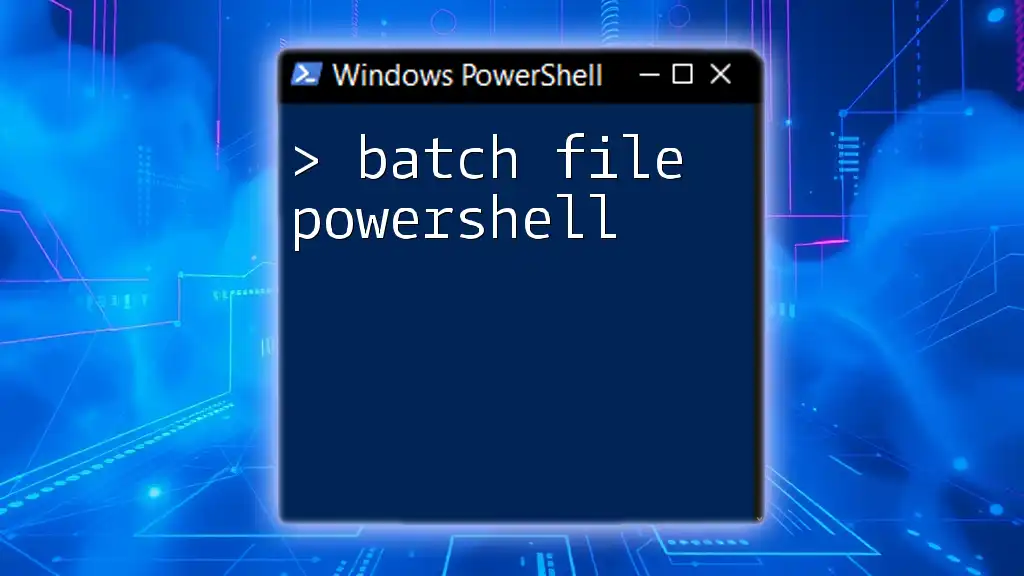
Introduction to PowerShell
What Is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administrators. Unlike batch files, which process text-based commands, PowerShell is built on the .NET framework, allowing it to work with objects and integrate tightly with Windows operating systems.
Key Features of PowerShell
PowerShell provides several features that set it apart from traditional batch scripting:
- Object-oriented scripting: PowerShell commands output objects, allowing for more complex data manipulation.
- Access to the .NET framework: You can leverage a vast library of functions.
- Integrated help system: Built-in cmdlets for help and documentation, making it easier for users to learn.
Here's an example of a simple PowerShell command demonstrating its syntax:
Write-Host "Hello, world!"
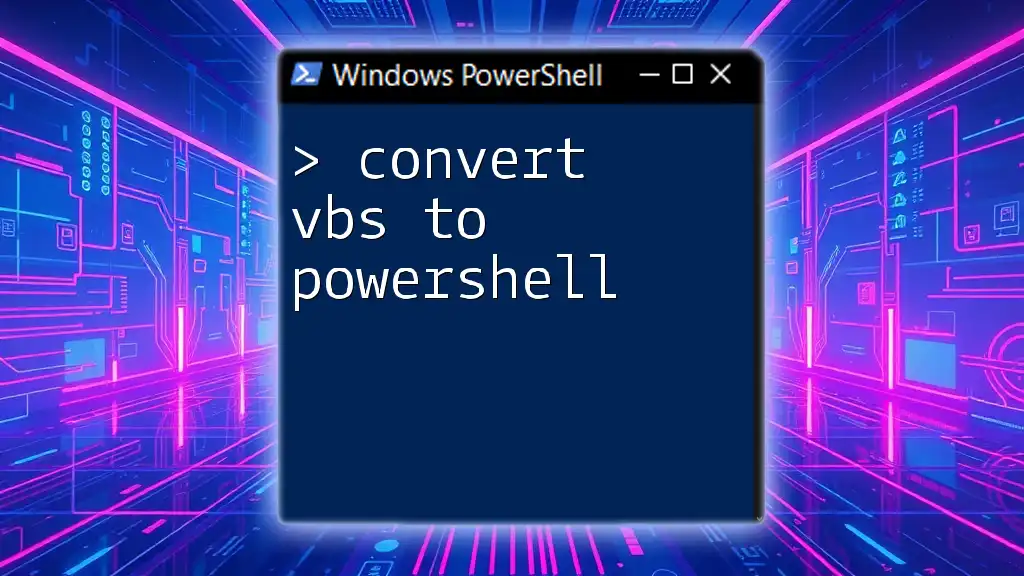
Why Convert Batch to PowerShell?
Advantages of PowerShell Over Batch Files
Converting batch files to PowerShell brings numerous benefits. These include enhanced capabilities, such as handling complex data types, which allows for advanced scripts that would be cumbersome in batch format.
Security is another significant advantage. PowerShell includes features for user authorization and can interact with Windows security features more effectively. These enhancements make PowerShell a preferred choice for modern scripting needs.
Common Scenarios for Conversion
Common situations that demand the conversion of batch scripts to PowerShell include:
- Automation tasks: Situations requiring complex logic and data handling.
- System administration: Managing and configuring systems more efficiently.
- Integration with other tools: PowerShell's ability to communicate with various APIs and services.
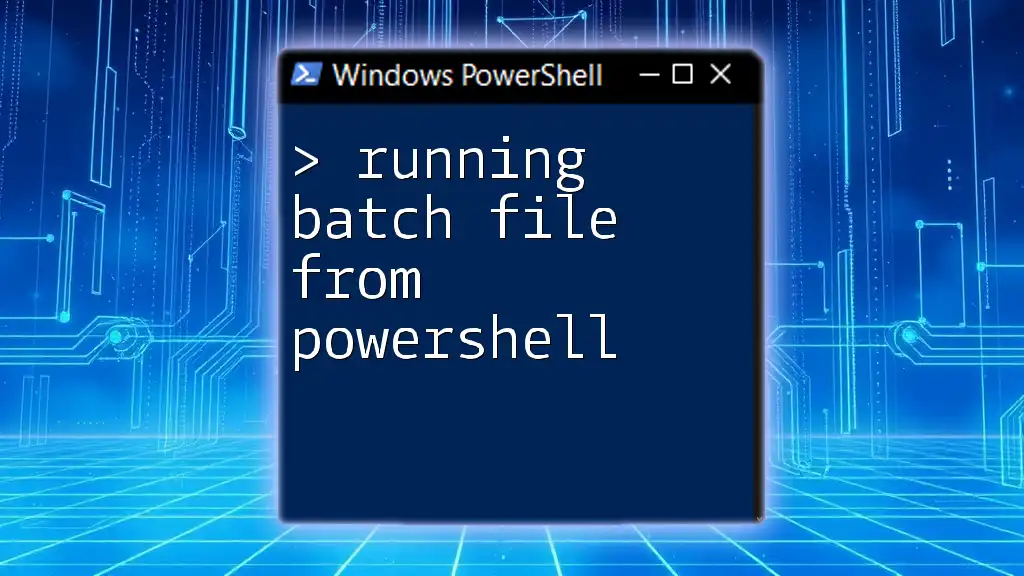
Steps to Convert Batch Script to PowerShell
Analyzing the Batch Script
Before converting batch scripts, analyze their components critically. Identify commands that need conversion, their corresponding variables, and the logic flow within the script. Let’s take a sample batch file for reference:
@echo off
set var=Hello
if %var%==Hello (
echo Welcome to PowerShell!
)
pause
Translating Batch Commands to PowerShell
Understanding common batch commands and their PowerShell equivalents is crucial for a smooth conversion. Here are some examples:
Example Commands:
-
Echo Command
In batch:
echo Hello
In PowerShell:
Write-Host "Hello"
-
Set Variable
In batch:
set var=value
In PowerShell:
$var = "value"
-
Conditional Statements
In batch:
if %var%==value ( echo Variable is value )
In PowerShell:
if ($var -eq "value") { Write-Host "Variable is value" }
Error Handling and Debugging
When converting scripts, it’s vital to incorporate error handling for increased reliability. PowerShell provides a built-in error handling mechanism with `try-catch` blocks. For example:
try {
# Your code here
} catch {
Write-Host "An error occurred: $_"
}
Additionally, to debug PowerShell scripts, you can use the `-Debug` switch alongside your commands or integrate `Write-Debug` statements to output debugging information.
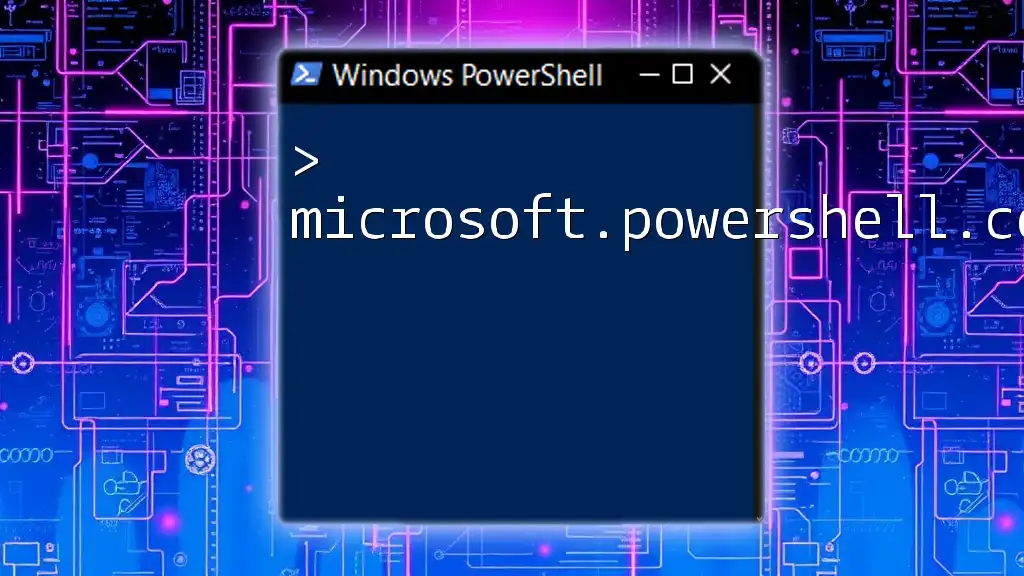
Converting Common Batch File Scenarios
File Operations
Batch scripts often involve basic file operations like copying and moving files. Here’s how to convert these operations:
-
Copying Files
In batch:
xcopy source destination
In PowerShell:
Copy-Item -Path "source" -Destination "destination"
-
Listing Directory Contents
In batch:
dir
In PowerShell, the same can be accomplished with:
Get-ChildItem
Running Executable Files
PowerShell excels in executing external programs. For instance, to open Notepad:
In batch:
start notepad.exe
In PowerShell:
Start-Process notepad.exe
Looping Structures
Loops in batch files can be converted to their PowerShell counterparts seamlessly. For example:
In batch:
for %%i in (1 2 3) do echo %%i
In PowerShell:
foreach ($i in 1..3) {
Write-Host $i
}
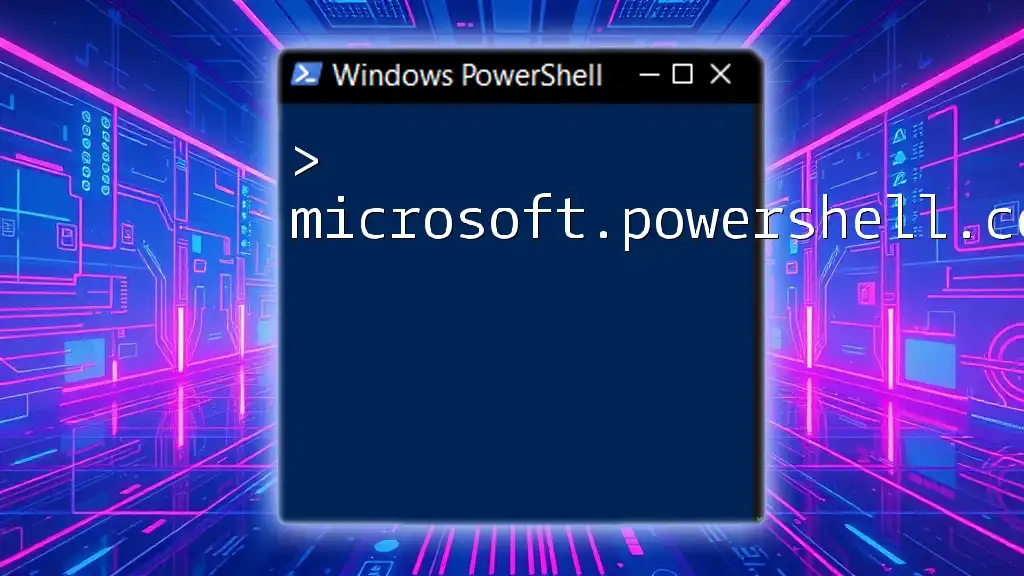
Best Practices for PowerShell Scripts
Optimization Tips
When writing PowerShell scripts, focus on efficiency and readability. Reuse code with functions, comment your code thoroughly, and avoid unnecessary repetitions. Also, leverage PowerShell's pipeline functionality to pass objects between commands without storing intermediate results.
Commenting and Documentation
Properly documented scripts are easier to maintain. Use comments to describe complex logic or important decisions made within the code. For example:
# This function retrieves the list of active users
function Get-ActiveUsers {
# Code for retrieving users goes here
}
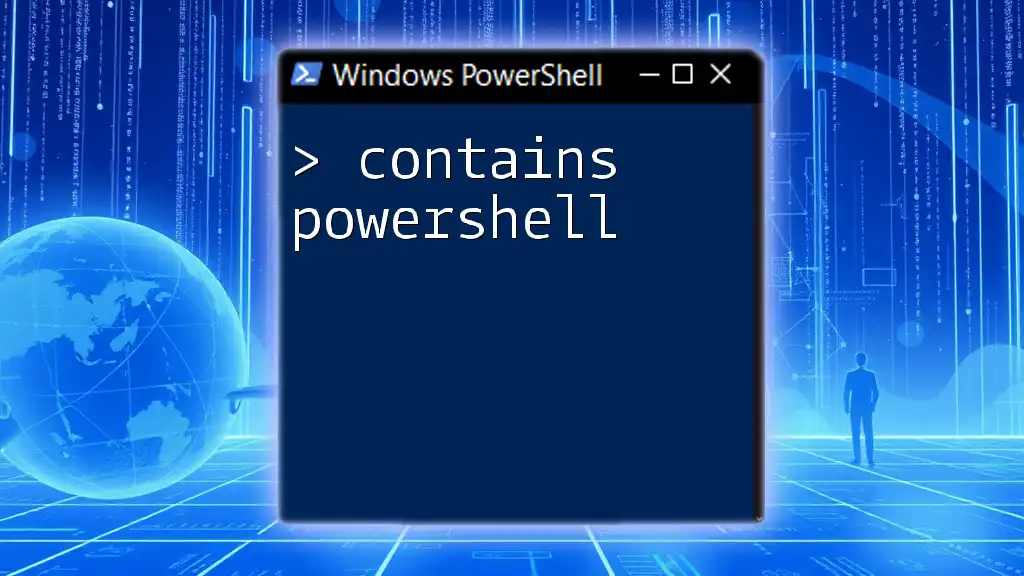
Tools for Batch to PowerShell Conversion
Manual Conversion
While manual conversion ensures deep understanding and flexibility, it can be time-consuming. Therefore, always double-check the translated script against the requirements of the original batch script.
Automated Tools
Certain automated tools can facilitate the conversion process by generating PowerShell equivalents from batch commands. However, it’s essential to review the output before using it in production, as automated tools might not perfectly capture the nuances of your original scripts.
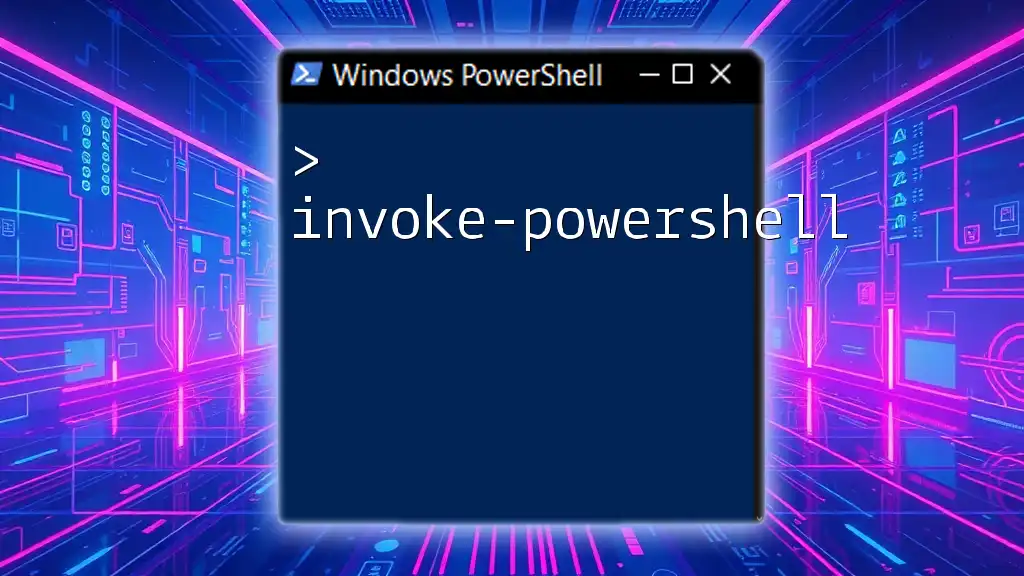
Conclusion
Converting batch files to PowerShell scripts not only modernizes your automation tasks but also enhances your scripting capabilities significantly. With PowerShell's rich feature set and robust design, you’re better equipped to handle complex scripting scenarios effectively. As you practice and implement these conversions, you will grow more adept at leveraging the full potential of PowerShell for your automation needs.
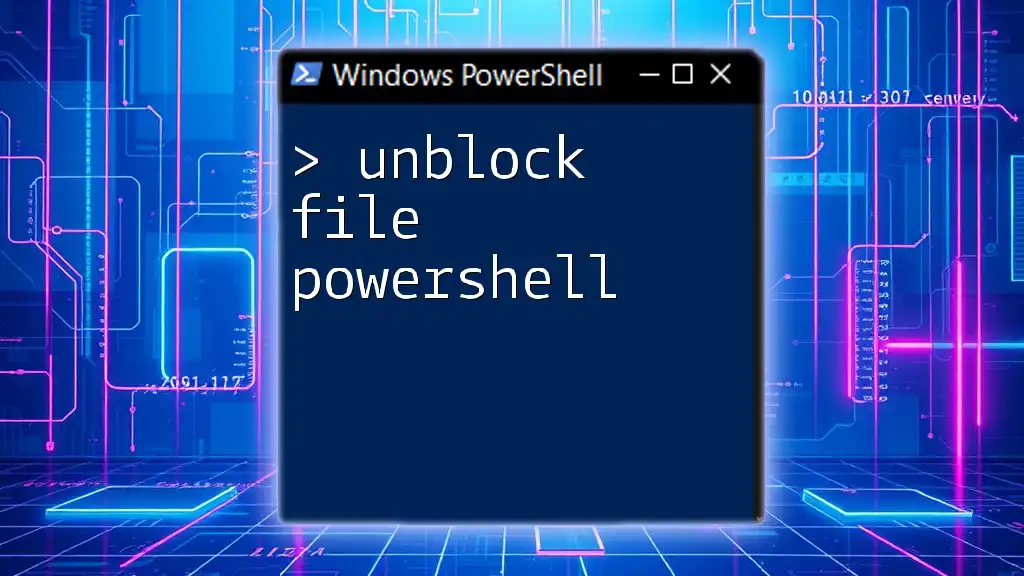
Additional Resources
For further exploration of PowerShell features and scripting techniques, consider visiting the [official PowerShell documentation](https://docs.microsoft.com/powershell/) and seeking out additional tutorials and guides online. Mastery of PowerShell opens up new avenues for automation and efficiency in any technical role.