Querying LDAP with PowerShell allows you to retrieve information from Active Directory efficiently using specific commands and filters. Here’s a simple example to search for users in a specific organizational unit (OU):
Get-ADUser -Filter * -SearchBase "OU=Users,DC=example,DC=com"
This command fetches all user accounts within the "Users" OU of the specified domain.
Setting Up Your Environment
Before diving into querying LDAP with PowerShell, it's essential to ensure your environment is correctly set up.
Requirements for Running PowerShell Commands
To effectively query LDAP using PowerShell, you need to check:
-
PowerShell Version Compatibility: Ensure you are using at least Windows PowerShell 5.1 or PowerShell Core. You can check your PowerShell version using the following command:
$PSVersionTable.PSVersion
-
Access Permissions for LDAP Queries: Ensure that your user account has the necessary permissions to perform LDAP queries. Typically, this means being a member of the relevant Active Directory groups.
Installing the Required Modules
PowerShell includes a module specifically for interacting with Active Directory. Before proceeding, you'll need to install the `ActiveDirectory` module if it's not already available. To do this, use:
Install-WindowsFeature -Name RSAT-AD-PowerShell
Once installed, you can import the module using:
Import-Module ActiveDirectory
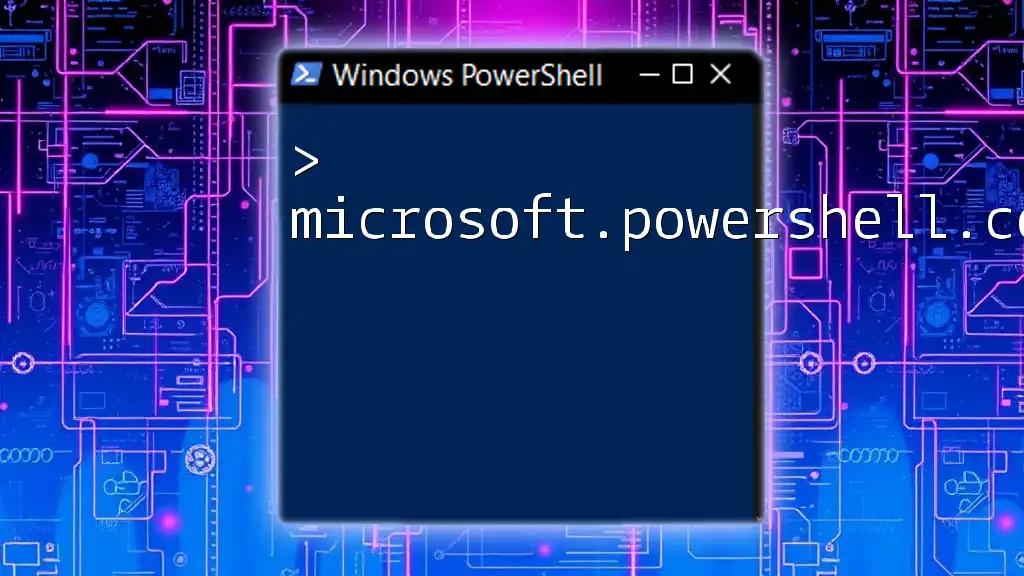
Basic Concepts for LDAP Queries
Understanding the basic elements of LDAP is crucial for crafting effective queries.
Understanding LDAP Structure
LDAP is structured as a tree, where each node represents an object. The primary objects include:
- Directories: Collections of objects that can include users, computers, printers, etc.
- Objects: Individual entries in the directory, such as user accounts or groups.
- Attributes: Properties defining characteristics of an object (e.g., a user's email address, phone number, etc.).
The Distinguished Name (DN) is a unique identifier for each entry in the LDAP directory, providing a full path to the object.
Key LDAP Objects and Attributes
Common LDAP objects you may work with include:
- User: Represents an individual user, typically containing attributes like `cn` (common name), `sn` (surname), `givenName`, `mail`, etc.
- Group: Represents a collection of users, often including attributes such as `member` (listing the individual users in the group).
Understanding these objects and attributes will help you formulate your queries effectively.
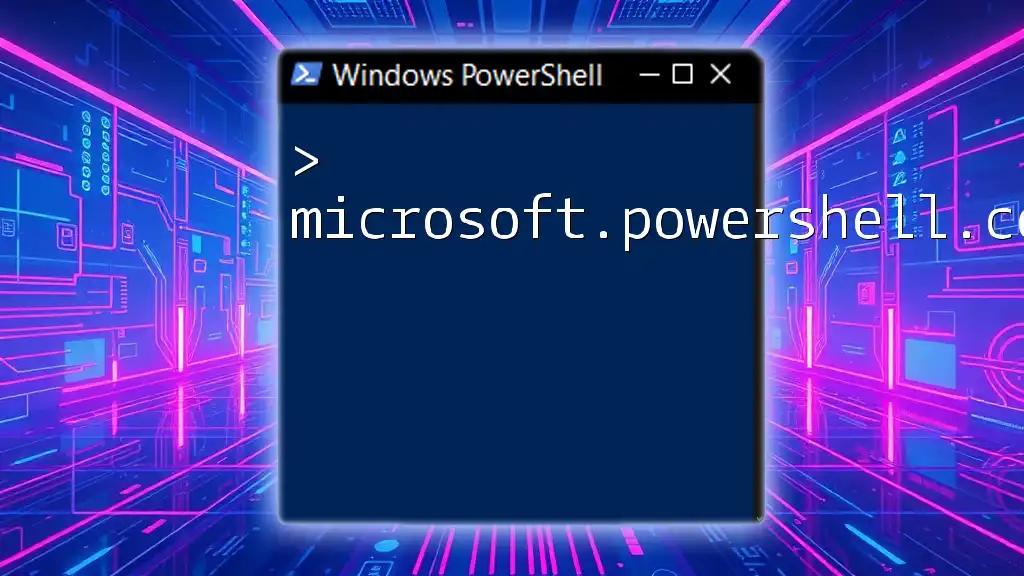
Connecting to an LDAP Server
To begin querying LDAP, you first need to establish a connection to the LDAP server.
Using PowerShell to Establish a Connection
You can create a connection to the LDAP server using the `DirectorySearcher` object. Here’s a basic connection example:
$ldapConnection = New-Object DirectoryServices.DirectorySearcher
When creating a connection, you might specify the LDAP server's path and credentials. Ensure you have the connection string accurately configured. For example:
$ldapConnection.SearchRoot = [ADSI]"LDAP://YourLdapServerName"

Performing LDAP Queries
Now that you're connected to the LDAP server, you can begin querying.
Basic Query with PowerShell
A straightforward LDAP query can be executed by setting the filter property of the `DirectorySearcher` object. Here's how you can query all users:
$searcher = New-Object DirectoryServices.DirectorySearcher
$searcher.Filter = "(objectClass=user)"
$searcher.PropertiesToLoad.Add("cn")
$results = $searcher.FindAll()
In this example, the filter `"(objectClass=user)"` fetches all entries classified as users.
Advanced Query Techniques
Using Filters to Refine Results
You can refine your search using more complex filters. For instance, to find users whose last name is "Smith" or given name is "John," combine logical operators:
$searcher.Filter = "(&(objectClass=user)(|(sn=Smith)(givenName=John)))"
This query uses the AND operator to specify the object class and the OR operator to search by either last name or first name.
Paging Through Large Result Sets
When working with large directories, you might only receive a partial result set. To handle this, you can implement pagination:
$searcher.PageSize = 1000
$results = $searcher.FindAll()
This ensures that you retrieve 1000 entries at a time consecutively, preventing performance bottlenecks.
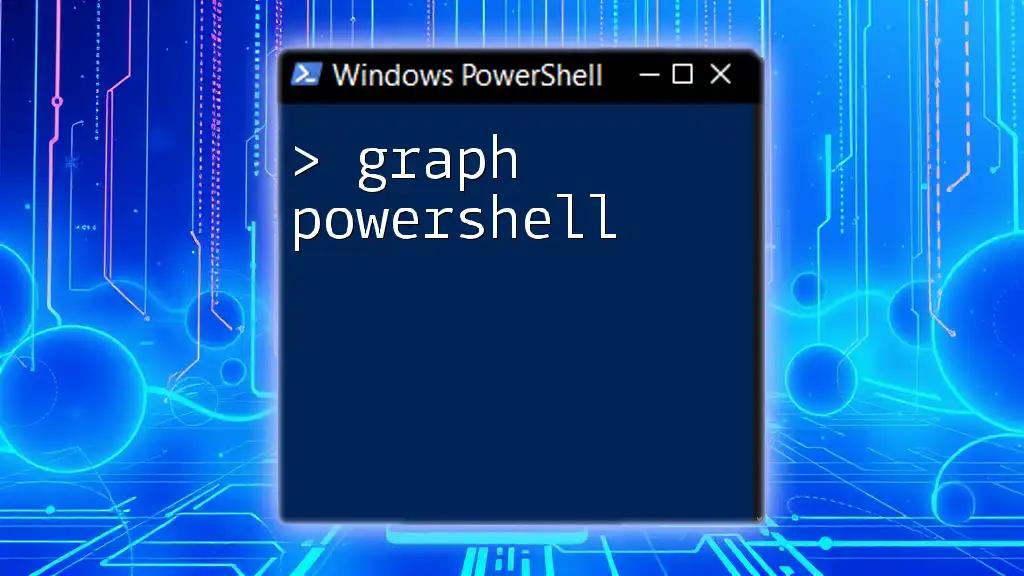
Working with Query Results
Once your queries yield results, handling that data is the next step.
Retrieving and Displaying Data
You can iterate through the results and extract desired properties. For instance, to display user common names (cn):
foreach ($result in $results) {
Write-Output $result.Properties.cn
}
This simple loop allows you to print each user's common name into the console, giving a quick overview of the results.
Exporting Results
If you need to share or further analyze the query results, exporting them is straightforward. A typical export to CSV can be accomplished using:
$results | Export-Csv -Path "LDAPResults.csv" -NoTypeInformation
This command saves the results into a CSV file, making it easy to open in applications like Excel.

Common Use Cases for LDAP Queries
LDAP queries can serve several practical purposes in an organization.
Finding Users in Active Directory
Querying users is one of the most common tasks. For example, if you need to gather all users in the "Sales" department, you might use a filter like this:
$searcher.Filter = "(&(objectClass=user)(department=Sales))"
Querying Group Membership
Another frequent use case involves checking group memberships. You can query a specific group and list its members with a query like:
$searcher.Filter = "(&(objectClass=group)(cn=SalesTeam))"
This retrieves the specified group's details, including all users associated with it.
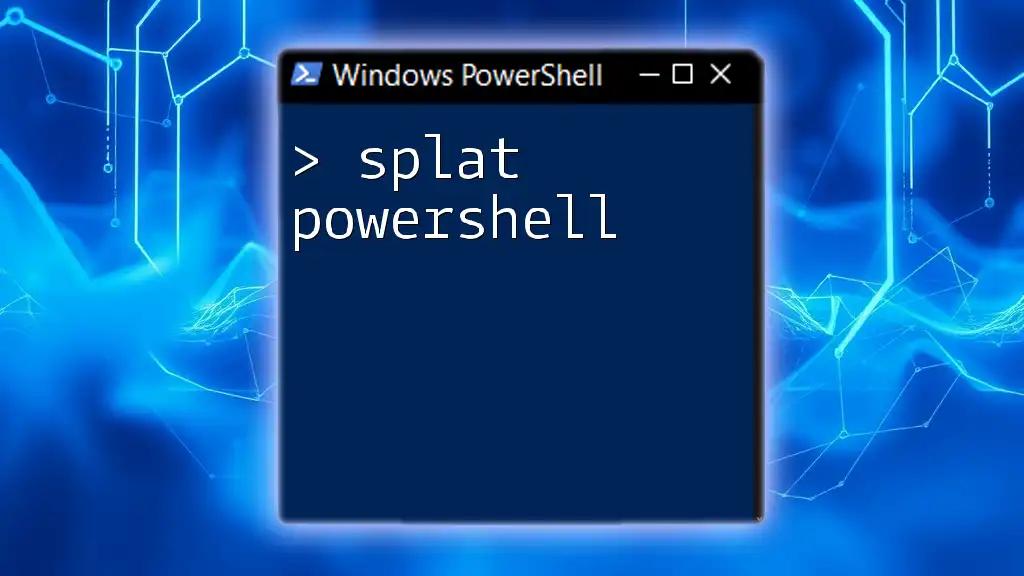
Troubleshooting LDAP Queries
Queries may not always produce the expected results; thus, troubleshooting is essential.
Common Errors and Their Solutions
Some frequent issues might include:
- Incorrect DN Format: Always ensure the DN strings are well-formed.
- Insufficient Permissions: Verify that your user account has the necessary access rights for the LDAP directory.
Using the PowerShell Debugging Features
If you're having trouble with your scripts, enabling debugging can provide more insights. Use the `-Debug` switch in your command, for example:
$results = $searcher.FindAll() -Debug
This will show additional details potentially helpful for identifying issues.
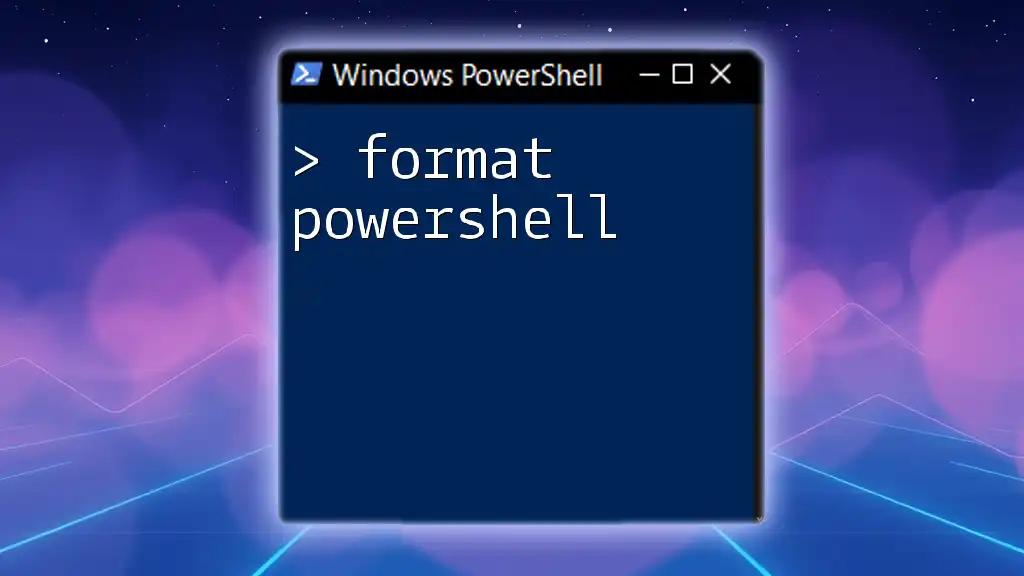
Conclusion
In this guide, you've learned how to query LDAP with PowerShell, covering everything from setting up your environment to executing complex queries and handling results. By understanding the underlying structure of LDAP and employing PowerShell commands, you can efficiently access and manipulate directory information.
Next Steps for Learning More
To expand your capabilities, consider exploring resources such as PowerShell documentation, online tutorials, or training courses specifically focusing on Active Directory and LDAP.
Call to Action
Practice makes perfect! Start experimenting with real LDAP queries and explore the full potential of PowerShell in managing directory services effectively.
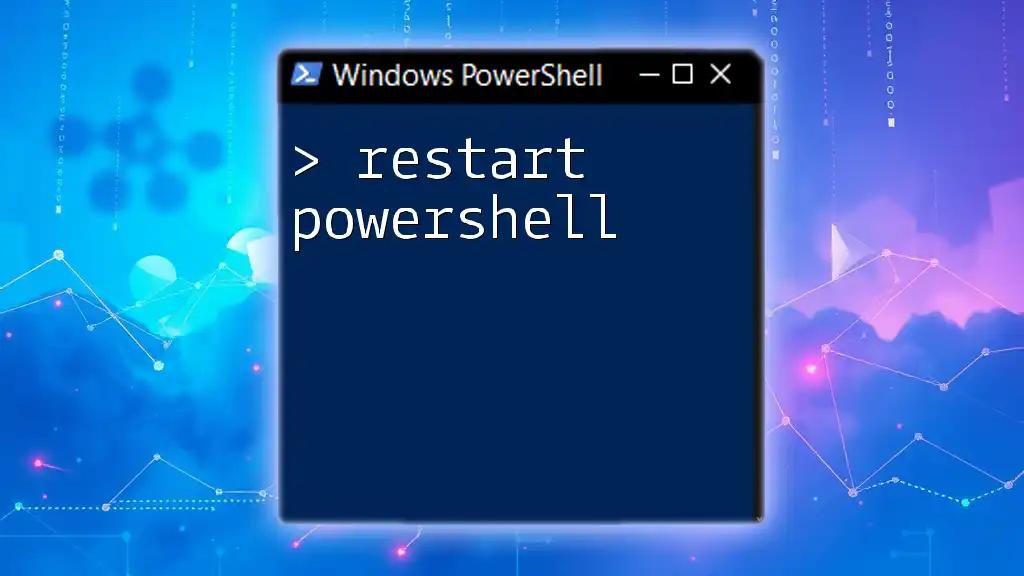
FAQs
-
What are the advantages of using LDAP with PowerShell?: PowerShell offers an intuitive command-line interface with powerful scripting capabilities, making it easy to automate LDAP tasks.
-
Can I run PowerShell scripts remotely to query LDAP?: Yes, with PowerShell remoting, you can run scripts on remote machines and interact with LDAP as needed.
-
Are there any alternatives to querying LDAP in PowerShell?: Other languages and tools, such as Python with the `ldap3` library or LDAP command-line tools, can query LDAP but may not offer the same level of integration with Windows environments as PowerShell does.