The PowerShell Zoom API allows users to interact with Zoom's features programmatically, such as creating meetings or managing users, using PowerShell commands for automation.
Here’s a simple code snippet to create a Zoom meeting using the Zoom API with PowerShell:
# Replace your API key and secret
$apiKey = "YOUR_API_KEY"
$apiSecret = "YOUR_API_SECRET"
$zoomUserId = "YOUR_ZOOM_USER_ID"
$meetingDetails = @{
topic = "Test Meeting"
type = 2
start_time = "2023-10-10T10:00:00Z"
duration = 30
timezone = "UTC"
agenda = "Discussing important topics"
} | ConvertTo-Json
$uri = "https://api.zoom.us/v2/users/$zoomUserId/meetings"
$headers = @{
"Authorization" = "Bearer $(New-JwtToken -ApiKey $apiKey -ApiSecret $apiSecret)"
"Content-Type" = "application/json"
}
$response = Invoke-RestMethod -Uri $uri -Method Post -Headers $headers -Body $meetingDetails
$response
Make sure to replace `YOUR_API_KEY`, `YOUR_API_SECRET`, and `YOUR_ZOOM_USER_ID` with your actual Zoom API credentials.
Understanding the Zoom API
What is the Zoom API?
The Zoom API allows developers to access Zoom's functionality programmatically. Whether it’s managing meetings, users, or reports, the API provides extensive features that cater to various operational needs. By using the API, businesses can automate common tasks, integrate Zoom functionalities into their existing systems, and enhance workflows significantly.
You can perform actions such as creating new meetings, retrieving user information, generating reports, and much more. This API is particularly useful for companies utilizing Zoom for remote communication and collaboration, ensuring smooth operations without manual intervention.
Why Use PowerShell for the Zoom API?
Using PowerShell to interact with the Zoom API offers several advantages:
- Simplicity: PowerShell provides an intuitive syntax that makes API interactions straightforward, even for those new to programming.
- Native Commands: Built-in cmdlets like `Invoke-RestMethod` streamline the process of sending requests and handling responses.
- Automation: PowerShell scripts can run automatically at set times or triggers, allowing for seamless integrations with scheduled tasks or system events.
Comparing PowerShell with other scripting languages, it excels in integrating into Windows environments, making it an ideal choice for enterprises heavily invested in Windows technologies.
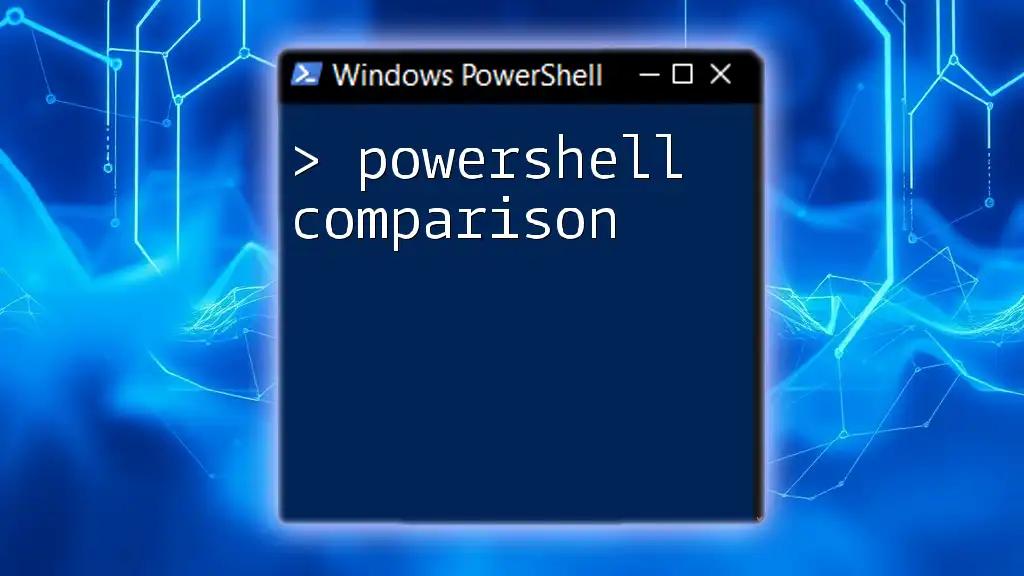
Setting Up Your Environment
Prerequisites
Before diving into the world of PowerShell Zoom API, ensure you have the necessary environment set up:
- A valid Zoom account is essential for obtaining API credentials.
- Familiarity with tools like cURL or Postman can be helpful for testing API interactions.
- Ensure that PowerShell is installed and accessible on your machine.
Installing PowerShell Modules
To get started with utilizing the API effectively, you may want to install certain PowerShell modules, enhancing your scripting capabilities. For instance, the `PowerShellGet` module is invaluable for managing other packages.
To install and verify the modules, you can run the following command:
Install-Module -Name PowerShellGet -Force -AllowClobber
With this setup, you're ready to explore the Zoom API with PowerShell.
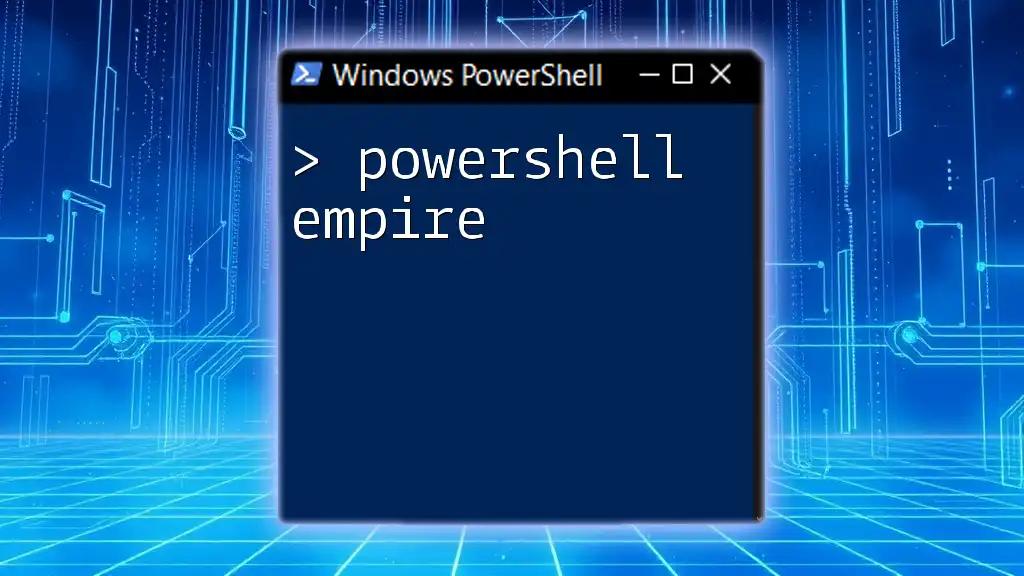
Authenticating with the Zoom API
Understanding OAuth and JWT
Authentication is crucial when connecting to any API. The Zoom API supports two primary authentication methods: OAuth and JWT (JSON Web Token).
- OAuth is widely used for applications needing to perform tasks on behalf of users, making it suitable for third-party applications accessing user data. However, managing tokens can be complex.
- JWT, on the other hand, is simpler and is ideal for server-to-server communication, where the identity is verified through tokens without user interaction.
Determining the best method for your needs will depend on your application's architecture and user engagement requirements.
Obtaining OAuth Tokens
If you choose to use OAuth, the first step is to obtain an OAuth token. Here’s how to do it:
- Navigate to your Zoom App Marketplace and create a new app.
- Select OAuth as the app type and fill in the required details.
- After creating the app, you'll receive a Client ID and Client Secret.
To request an OAuth token via PowerShell, you can use the following example code snippet (substituting your credentials):
$clientId = "your_client_id"
$clientSecret = "your_client_secret"
$tokenUrl = "https://zoom.us/oauth/token"
$authHeader = [Convert]::ToBase64String([Text.Encoding]::ASCII.GetBytes(“$clientId:$clientSecret”))
$response = Invoke-RestMethod -Uri $tokenUrl -Method Post -Headers @{Authorization=("Basic $authHeader")} -Body @{grant_type="account_credentials"}
$token = $response.access_token
Using JWT for Authentication
To authenticate using JWT, you will need to generate a JWT token that includes the necessary claims. Here's how to do that:
$jwtHeader = @{ alg = "HS256"; typ = "JWT" }
$jwtPayload = @{ iss = $clientId; exp = (Get-Date -UFormat %s) + 3600 }
// Example function to generate the JWT
$header = [Convert]::ToBase64String([Text.Encoding]::UTF8.GetBytes(($jwtHeader | ConvertTo-Json)))
$payload = [Convert]::ToBase64String([Text.Encoding]::UTF8.GetBytes(($jwtPayload | ConvertTo-Json)))
$signature = [Convert]::ToBase64String([Text.Encoding]::UTF8.GetBytes("your_signature")) // Use your own algorithm for signing
$jwt = "$header.$payload.$signature"
With your JWT token in hand, you can proceed to make API calls.
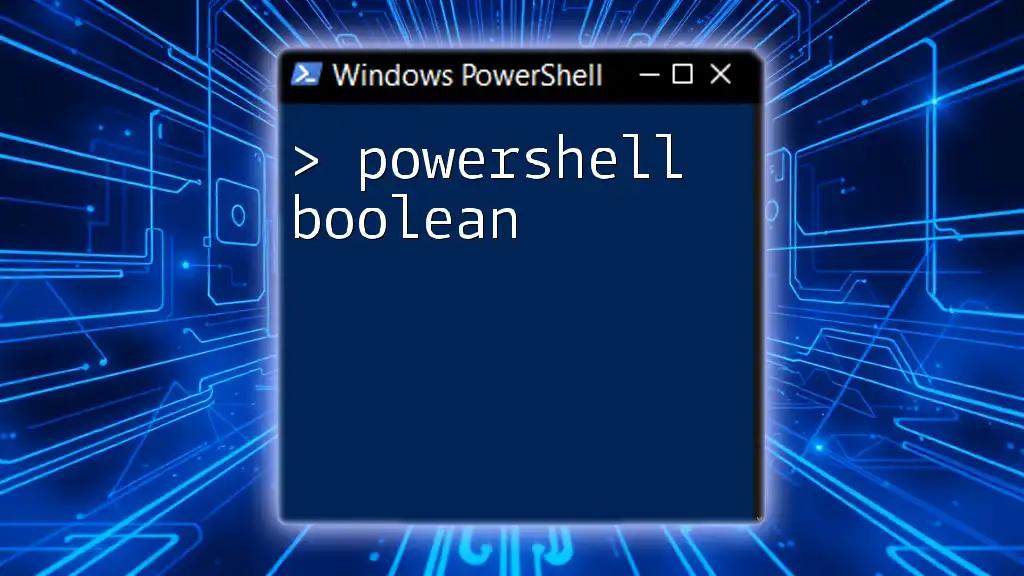
Common Endpoints of the Zoom API
Overview of Key API Endpoints
The Zoom API offers several endpoints, each designed for specific actions. Here are some of the most commonly used endpoints:
- Meetings: Manage meeting creation, updates, and deletions.
- Users: Retrieve, create, or update user data.
- Reports: Generate reports on meeting occurrences, participants, and analytics.
Familiarizing yourself with these endpoints can help streamline your development processes.
Accessing the Meetings API
To interact with the API and manage meetings, the following example demonstrates how to list, create, and delete meetings, showcasing the power of PowerShell:
Listing Meetings
To list existing meetings for a user, you can use:
$meetingsUrl = "https://api.zoom.us/v2/users/me/meetings"
$response = Invoke-RestMethod -Uri $meetingsUrl -Method Get -Headers @{Authorization = "Bearer $token"}
$response
Creating a Meeting
Creating a new meeting can be executed with the following command:
$createMeetingUrl = "https://api.zoom.us/v2/users/me/meetings"
$body = @{
topic = "Project Update"
type = 2
start_time = "2023-10-15T12:00:00Z"
duration = 30
timezone = "UTC"
agenda = "Discuss project milestones"
}
$response = Invoke-RestMethod -Uri $createMeetingUrl -Method Post -Headers @{Authorization = "Bearer $token"} -Body ($body | ConvertTo-Json) -ContentType "application/json"
$response
Deleting a Meeting
Deleting an existing meeting can be achieved using:
$meetingId = "your_meeting_id_here"
$deleteMeetingUrl = "https://api.zoom.us/v2/meetings/$meetingId"
$response = Invoke-RestMethod -Uri $deleteMeetingUrl -Method Delete -Headers @{Authorization = "Bearer $token"}
$response
Managing Users through the API
Managing users is equally important. Here’s how you can retrieve, create, and delete users via the API:
Retrieving User Information
To obtain details about users within your Zoom account, the command below is useful:
$usersUrl = "https://api.zoom.us/v2/users"
$response = Invoke-RestMethod -Uri $usersUrl -Method Get -Headers @{Authorization = "Bearer $token"}
$response
Creating a New User
To add a new user to your Zoom account:
$userUrl = "https://api.zoom.us/v2/users"
$body = @{
action = "create"
user_info = @{
email = "newuser@example.com"
first_name = "New"
last_name = "User"
type = 1
}
}
$response = Invoke-RestMethod -Uri $userUrl -Method Post -Headers @{Authorization = "Bearer $token"} -Body ($body | ConvertTo-Json) -ContentType "application/json"
$response
Deleting a User
If you need to delete a user:
$userId = "user_id_here"
$deleteUserUrl = "https://api.zoom.us/v2/users/$userId"
$response = Invoke-RestMethod -Uri $deleteUserUrl -Method Delete -Headers @{Authorization = "Bearer $token"}
$response
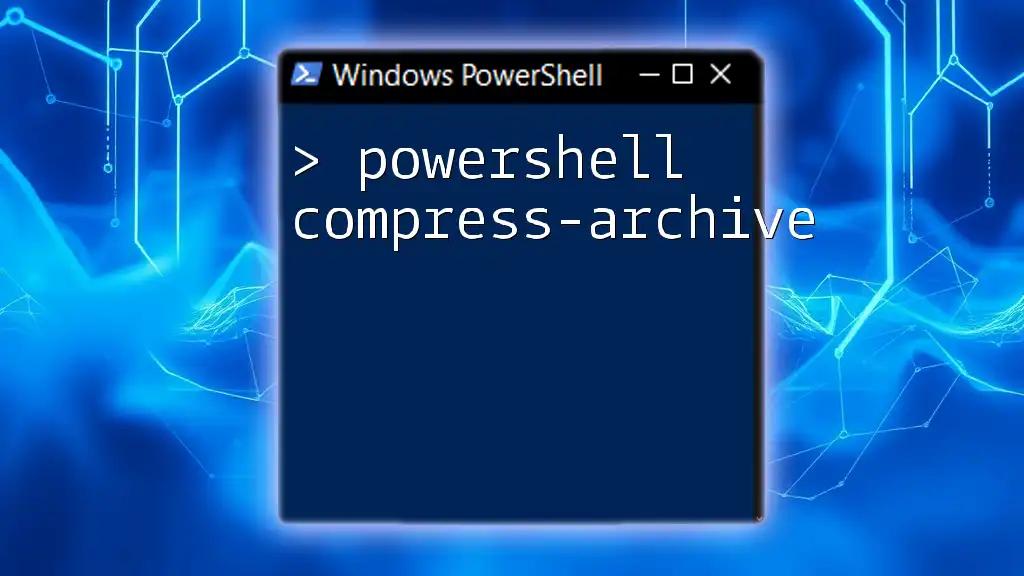
Advanced Usage
Handling Errors and Responses
When making API calls, errors may occur. It’s essential to implement robust error handling. Use `Try-Catch` blocks to capture exceptions and ensure your script remains resilient, providing appropriate output in case of error.
try {
# Your API Call
} catch {
Write-Host "An error occurred:" $_.Exception.Message
}
Additionally, inspect response statuses to respond accordingly, ensuring your scripts are both informative and user-friendly.
Automating Common Tasks
PowerShell can be used to automate repetitive tasks easily. For example, you might want to schedule daily recurring meetings or automate user reports. By combining earlier examples into functions and leveraging Windows Task Scheduler, you can execute your scripts on a schedule. Here’s a simplified function example that can be scheduled:
function Schedule-DailyMeeting {
param (
[string]$topic,
[string]$agenda
)
# Creating a recurring meeting logic...
}
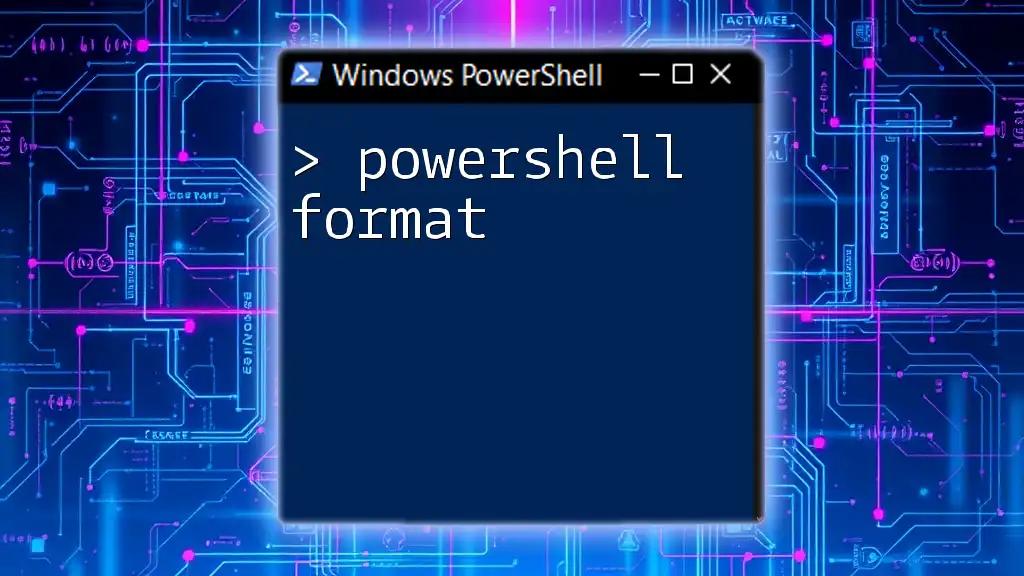
Conclusion
The PowerShell Zoom API opens doors to immense capabilities for automating and managing your Zoom environment efficiently. By following the steps outlined in this guide, you can seamlessly integrate Zoom functionalities into your scripting processes.
Exploring this technology will empower you to maximize your Zoom usage, drive productivity, and enhance your collaborative efforts. Don’t hesitate to dive deeper and start exploring these methodologies in your projects.
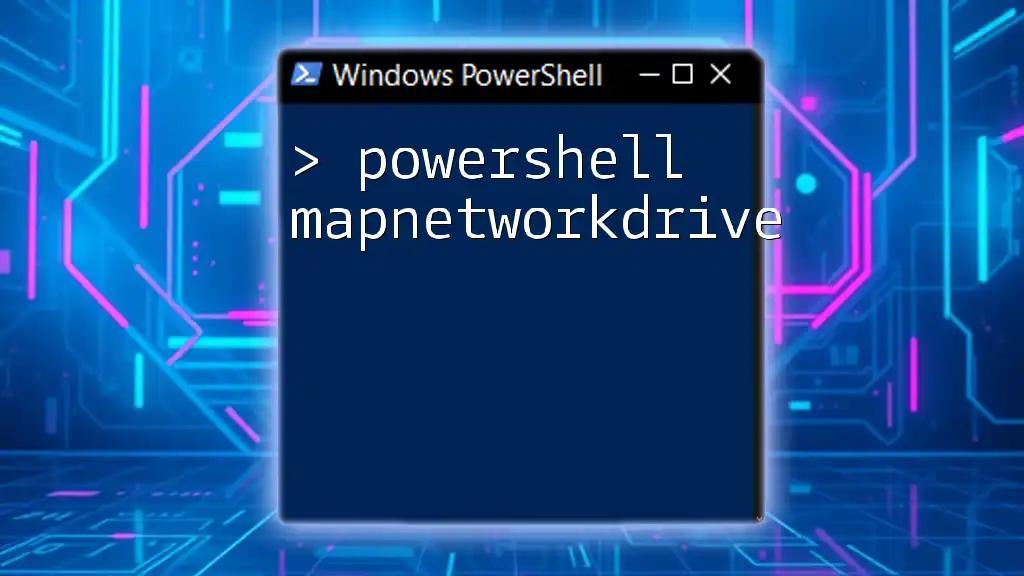
Additional Resources
Official Documentation
For additional learning, make sure to refer to the [Zoom API Documentation](https://marketplace.zoom.us/docs/api-reference/zoom-api) and the [PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.1).
Community and Support
Engaging with the community through forums or Zoom user groups is a great way to share knowledge and learn from the experiences of others.
Get in Touch
We encourage you to share your progress, ask questions, and keep learning about the potential of PowerShell and the Zoom API.