The "PowerShell Send Keys" functionality allows you to simulate keyboard input, sending keystrokes to applications programmatically.
Here's a simple code snippet demonstrating how to send the "Hello" phrase to an active window using PowerShell:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.SendKeys]::SendWait("Hello")
Understanding the Basics of Send Keys
What are Send Keys?
Send Keys is a powerful concept that allows automation of keyboard input in scripts. Essentially, it enables you to simulate keystrokes, sending the same key sequence to an application that a user would enter manually. This capability is particularly useful for tasks such as automating repetitive actions, interacting with GUI applications, or even controlling your own scripts dynamically based on user inputs.
How PowerShell Interacts with Send Keys
PowerShell can utilize the `SendKeys` class from the `System.Windows.Forms` namespace to achieve this behavior. While PowerShell is primarily geared towards managing systems and automation tasks, its versatility allows it to also simulate user interactions through keystrokes, aiding in both convenience and efficiency.
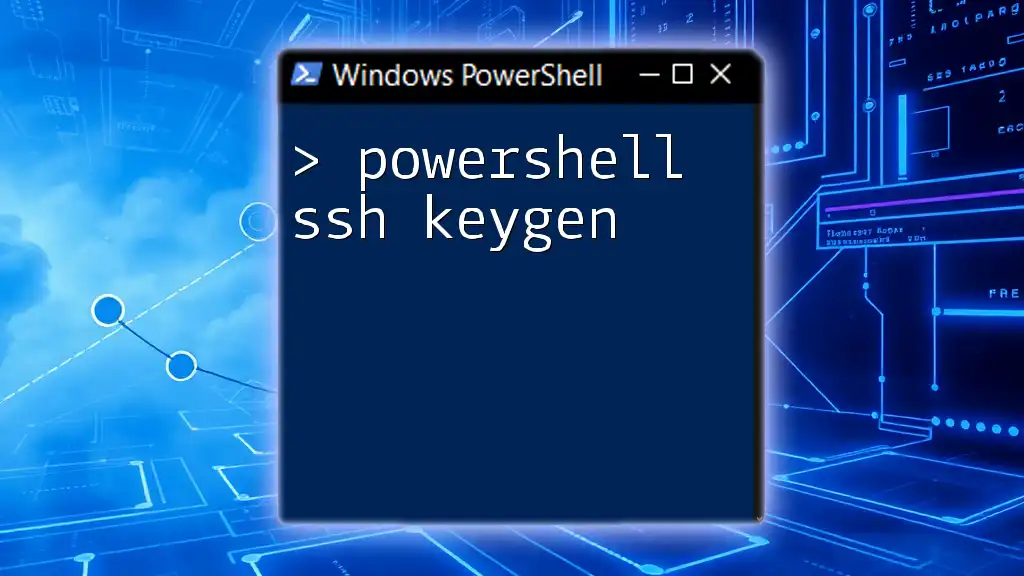
Setting Up Your Environment
Prerequisites
Before you can use PowerShell Send Key, ensure that you are running at least PowerShell 5.1 or later. Additionally, you'll need to have the execution policy set to allow running scripts. You can check your execution policy by running:
Get-ExecutionPolicy
If needed, set it to `RemoteSigned` or `Unrestricted` by executing:
Set-ExecutionPolicy RemoteSigned
Importing Required Assemblies
To begin using SendKeys, you must first import the necessary libraries. This is done easily with the following command:
Add-Type -AssemblyName System.Windows.Forms
This command loads the `System.Windows.Forms` assembly, which contains the `SendKeys` class you'll be using. Without this step, any attempts to call Send Keys methods will result in errors.
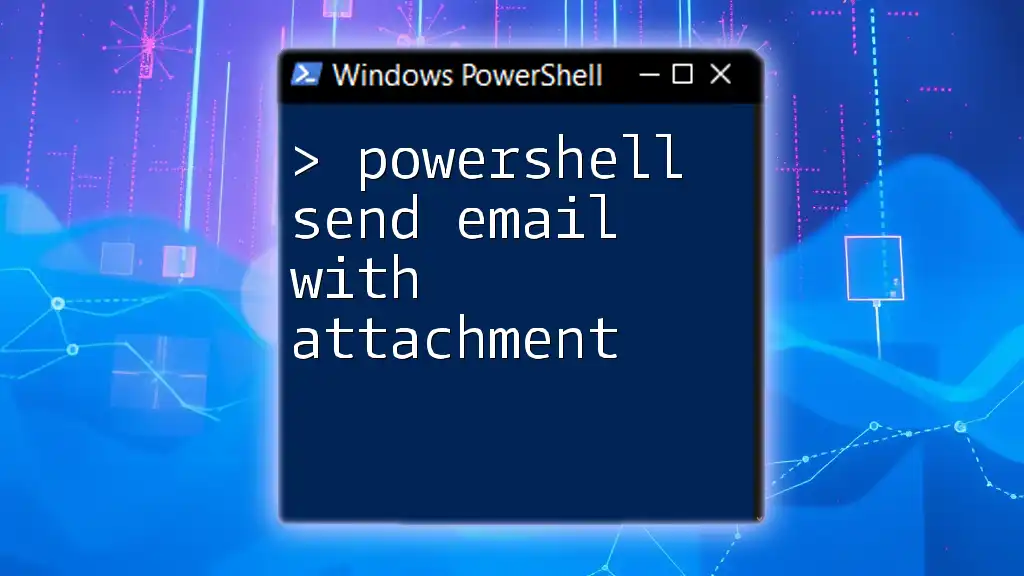
The Process of Sending Keys
Using the `SendKeys` Class
The `SendKeys` class in PowerShell provides an interface to send keystrokes to the active application. It offers a method called `SendWait()`, which sends the keystrokes to the foreground app and waits for the message to be processed. This is vital for ensuring that applications correctly register the input.
Commonly Used Key Codes
Understanding key codes is essential when using Send Keys. Below are some commonly used key codes:
- {ENTER} - Represents the Enter key.
- {TAB} - Represents the Tab key.
- {ESC} - Represents the Escape key.
- % - Represents the Alt key (e.g., `%{TAB}` for Alt + Tab).
- ^ - Represents the Ctrl key.
Here’s an example that demonstrates sending the Enter key:
[System.Windows.Forms.SendKeys]::SendWait("{ENTER}")
This command simulates pressing the Enter key, which can be particularly useful for confirming dialog actions or sending form submissions.
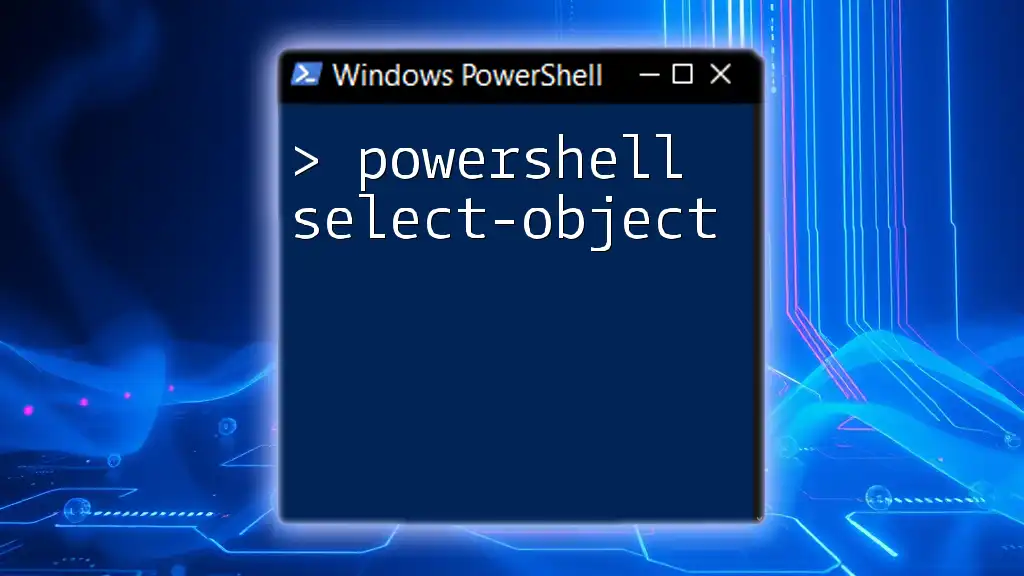
Practical Applications
Automating Form Filling
One of the most practical uses of PowerShell Send Key is automating form filling in various applications. Consider a scenario where you want to fill out a simple Notepad document. You can accomplish this with the following script:
# Open Notepad and send text
Start-Process notepad
Start-Sleep -Seconds 2
[System.Windows.Forms.SendKeys]::SendWait("Hello, this is an automated message.{ENTER}")
In this example, Notepad is launched, followed by a 2-second delay to ensure it is ready for input. The subsequent line sends a custom message along with an Enter key press to break the line.
Navigating Through Applications
You can also use Send Keys to automate navigation between applications. For instance, if you want to switch between the active applications using Alt + Tab, you can do it with:
[System.Windows.Forms.SendKeys]::SendWait("%{TAB}")
This simulates the Alt + Tab action, allowing you to navigate through your open applications seamlessly.
Control Windows Features
Another useful application is controlling Windows features such as launching the Task Manager. Here’s how you can do this:
Start-Process "taskmgr"
Start-Sleep -Seconds 2
[System.Windows.Forms.SendKeys]::SendWait("%{F4}")
This script opens the Task Manager, waits for a couple of seconds to allow it to load, and then sends the Alt + F4 command to close it.
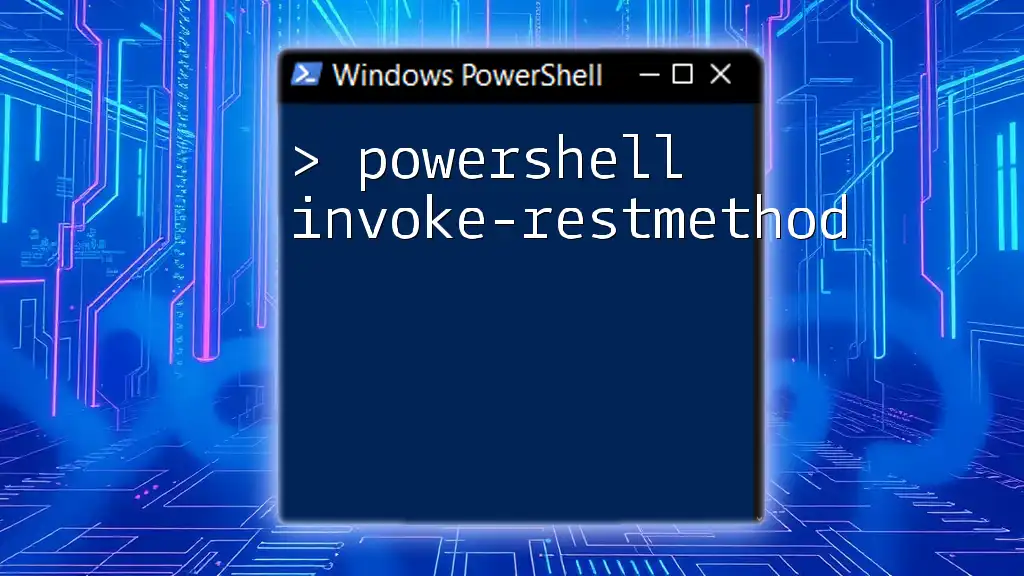
Best Practices and Considerations
Timing and Delays
When working with Send Keys, it's crucial to implement appropriate delays in your scripts. Applications may not respond instantly to key presses, especially if they require time to load. Using `Start-Sleep` ensures that the application is ready to receive input, preventing errors and increasing the reliability of your scripts.
Error Handling
Errors can occur when using Send Keys, particularly if the targeted application is not active or if the keystrokes are sent too quickly. Always test your scripts in a controlled environment to confirm their reliability, and consider wrapping your commands in error-handling structures to catch any unexpected issues.
Security Considerations
While automating keystrokes can be incredibly powerful, it's essential to maintain security. Improper use of Send Keys can lead to unintended actions, especially if sensitive applications are open. Ensure your scripts operate within secure environments and avoid automating actions that could pose risks to sensitive data.
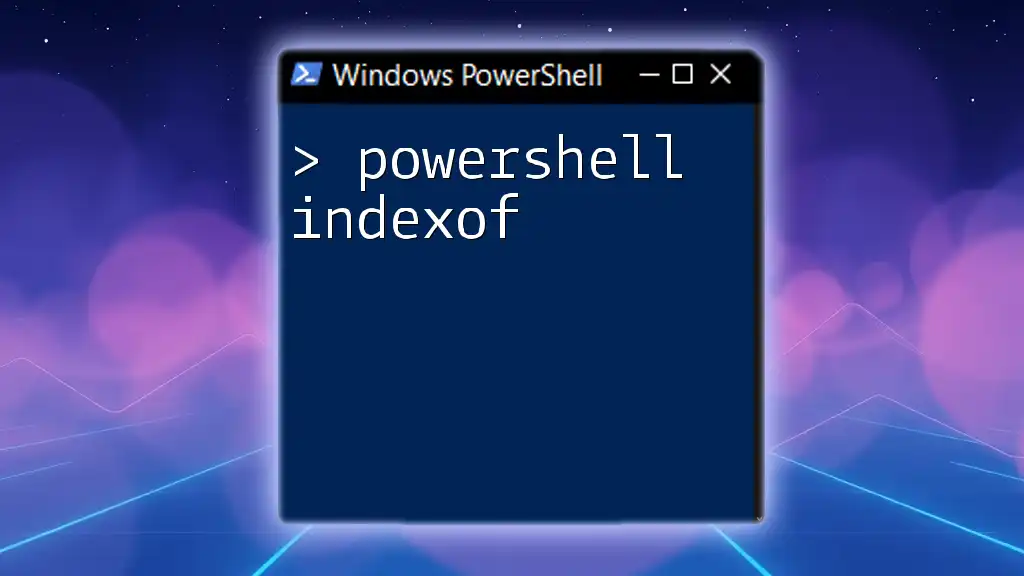
Conclusion
Having explored PowerShell Send Keys, you now possess a solid understanding of how to simulate keystrokes to automate tasks in Windows applications. This functionality allows for enhanced scripting capabilities that save time and increase productivity.
Encouraged by the information provided, feel free to experiment further and push the boundaries of what you can achieve with PowerShell Send Key.
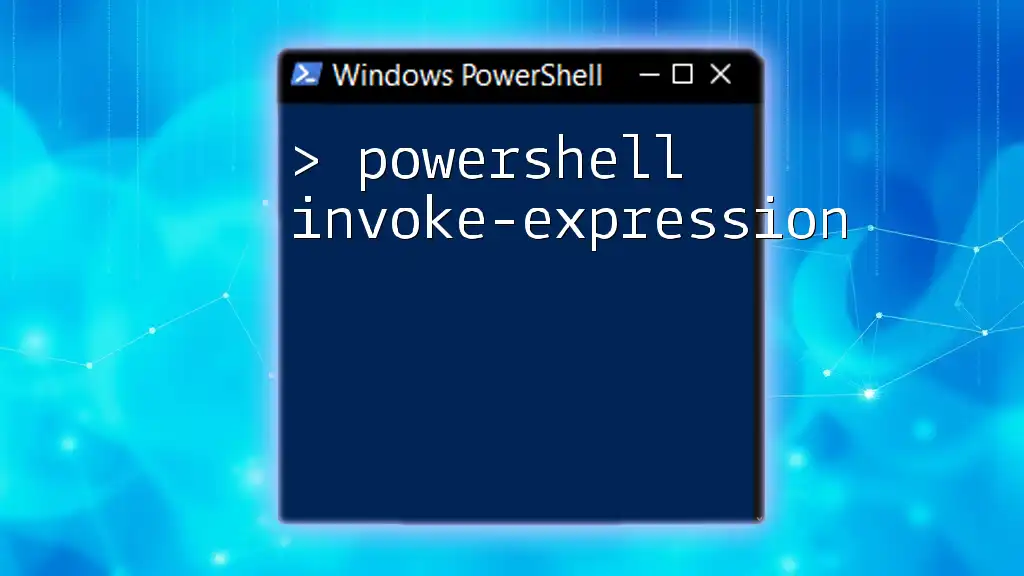
Call to Action
Share your experiences or ask questions related to PowerShell automation in the comments below! Check out our upcoming courses and workshops to deepen your PowerShell skills even more.
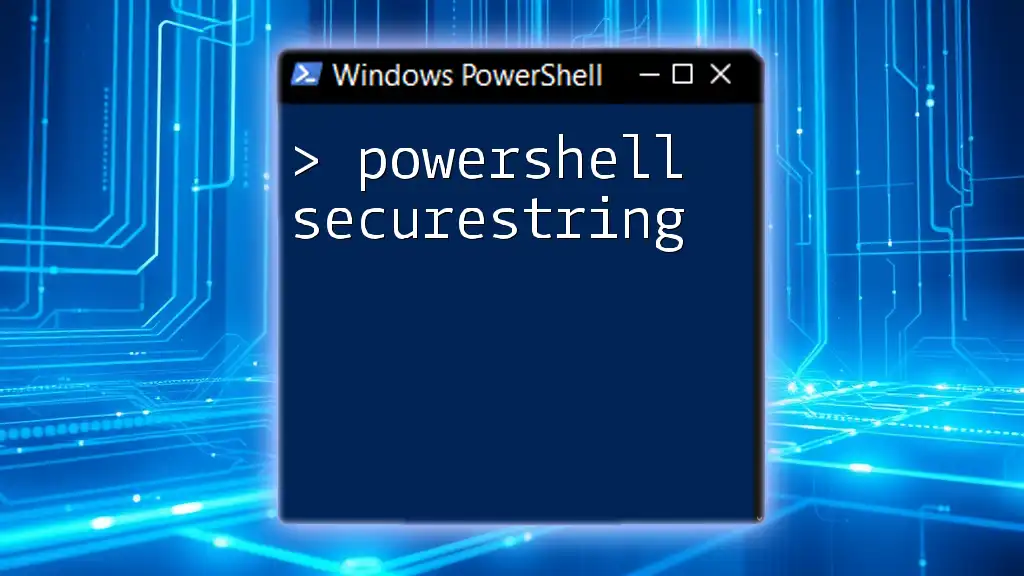
Additional Resources
For more in-depth knowledge, consider visiting the official Microsoft documentation on PowerShell and exploring PowerShell forums and communities to connect with others in your learning journey.