A PowerShell query is a command used to retrieve specific data or interact with system resources efficiently and can be executed in a concise manner for quick results.
Here’s a simple example that queries the list of running processes on your machine:
Get-Process
Understanding PowerShell Queries
What is a PowerShell Query?
A PowerShell query is a command that retrieves data or system information from various sources such as local machines, remote servers, files, or databases. It is essential for automating administrative tasks, monitoring system performance, and managing data efficiently. The main objective of a PowerShell query is to provide users with the ability to extract necessary information quickly, enabling better decision-making.
Common Uses of PowerShell Queries
PowerShell queries can be used across various domains:
-
Administrative Tasks: System administrators frequently use queries to gather performance metrics, audit system states, and manage services and processes.
-
Data Extraction: Queries allow users to efficiently extract data from files (like CSV or XML) and databases.
-
Automation of Repetitive Tasks: By scripting queries, users can automate routine operations, thus saving time and reducing human error.
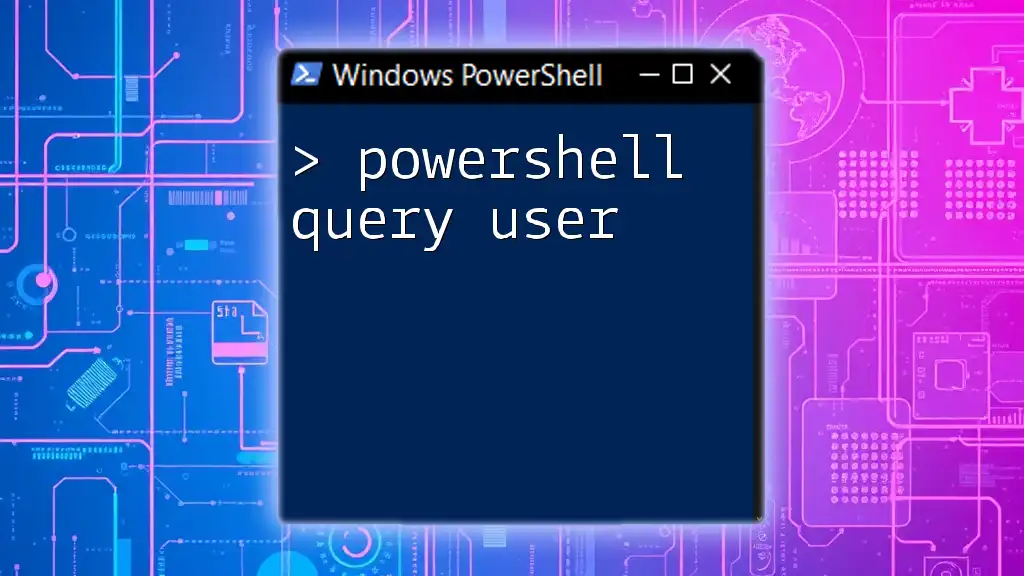
Basic Syntax of PowerShell Queries
Cmdlets Overview
In PowerShell, a cmdlet is a lightweight command used by the shell. Cmdlets are used for obtaining data, managing systems, and running tasks. Several cmdlets are prevalent for querying data, including:
- `Get-Command`: Lists all available cmdlets, functions, and aliases.
- `Get-Item`: Returns the item at the specified location.
- `Get-Content`: Retrieves the content of a file.
Constructing a Simple Query
The basic structure of a PowerShell query starts with a cmdlet that specifies what type of information to retrieve, followed by any parameters or options.
For instance, to get information about all currently running processes, you can use:
Get-Process
This command provides a list of running processes, including crucial information such as their names and IDs.
Filtering Results with Where-Object
Filtering is integral when working with queries, as it enables you to narrow down results to significant data. The `Where-Object` cmdlet facilitates this by allowing you to specify conditions.
Example:
Get-Process | Where-Object {$_.CPU -gt 100}
In this case, the command retrieves processes with a CPU usage greater than 100, showcasing how filtering can enhance data relevance.
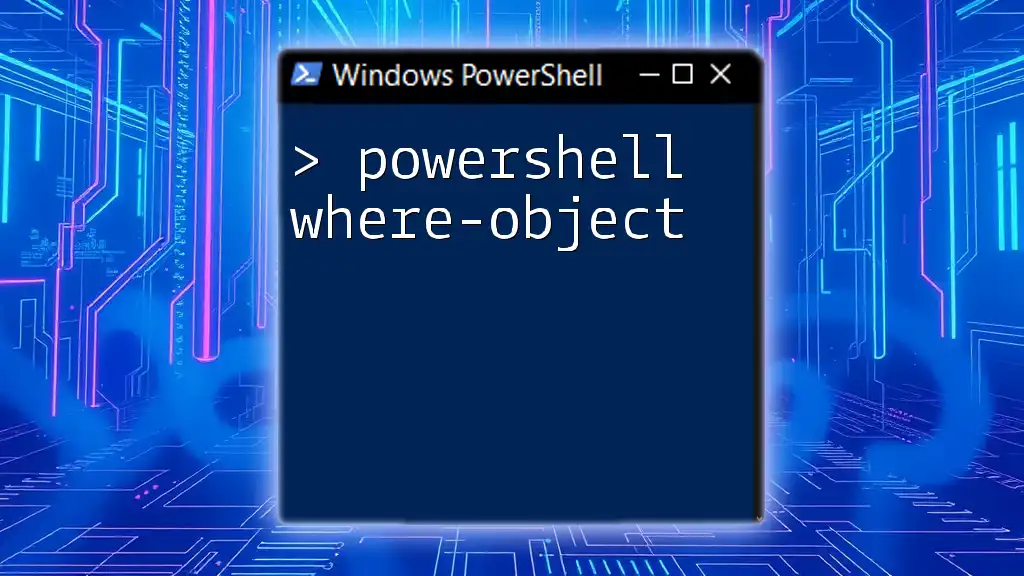
Advanced Query Techniques
Using Select-Object for Data Presentation
Another vital aspect of PowerShell queries is presenting the data in useful formats. The `Select-Object` cmdlet allows you to specify which properties to display.
For example:
Get-Process | Select-Object Name, CPU, Id
This command will return only the Name, CPU, and Id properties of each process, making it easier to analyze the data.
Sorting and Grouping Results
Sorting with Sort-Object
Organizing results can significantly improve readability. The `Sort-Object` cmdlet sorts query output based on specified properties.
For example:
Get-Service | Sort-Object Status
This command will display services sorted by their status (Running, Stopped), allowing users to quickly assess overall system status.
Grouping with Group-Object
Grouping data can reveal patterns and summaries. The `Group-Object` cmdlet provides a mechanism for this.
For instance:
Get-Service | Group-Object Status
The output will group services based on their status, facilitating a quick overview of all services by their operational states.
Working with Remote Queries
Understanding how to perform queries on remote systems is crucial, especially in enterprise environments. The `Invoke-Command` cmdlet serves this purpose.
With `Invoke-Command`, you can run commands on remote computers seamlessly.
Invoke-Command -ComputerName "Server01" -ScriptBlock {Get-Process}
This command will execute the `Get-Process` command on the specified remote computer, allowing you to gather data from multiple systems centrally.
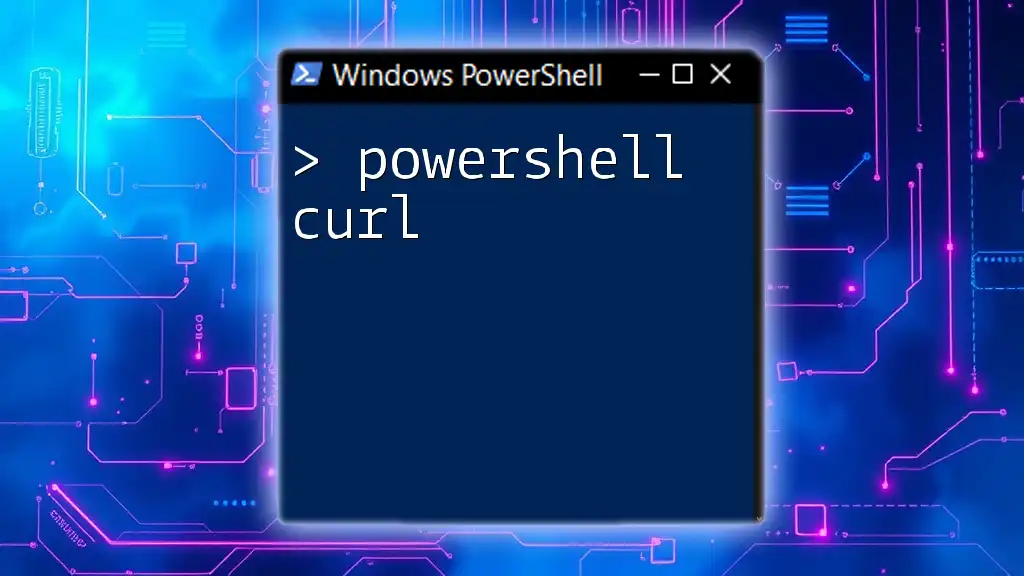
Using PowerShell with Databases
Connecting to SQL Databases
PowerShell not only queries local data but can also connect to SQL databases. This capability is essential for those managing data-driven applications. You can use the `SqlCommand` class to execute SQL queries directly from PowerShell.
Here's an example of how to create a connection and execute a simple SQL query:
$connection = New-Object System.Data.SqlClient.SqlConnection
$connection.ConnectionString = "Data Source=Server;Initial Catalog=Database;Integrated Security=True;"
$command = $connection.CreateCommand()
$command.CommandText = "SELECT * FROM dbo.Users"
$connection.Open()
$reader = $command.ExecuteReader()
In this example, we create a connection to a SQL Server database, prepare a command to select all records from the Users table, and open the connection for execution. Each line plays a vital role in managing data efficiently.
Importing Data from CSV Files
PowerShell makes it easy to work with CSV files, leveraging the `Import-Csv` cmdlet to streamline data ingestion.
For example:
Import-Csv "C:\Data\users.csv"
This command imports the data contained in `users.csv`, enabling you to manipulate and analyze the data within PowerShell.
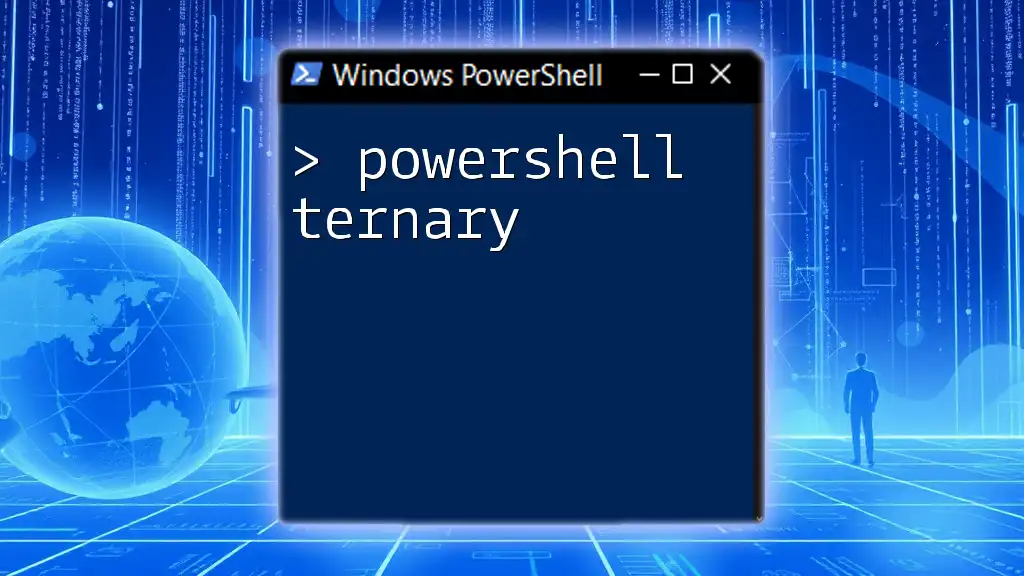
Best Practices for PowerShell Queries
Writing Efficient Queries
When executing PowerShell queries, performance and efficiency are paramount. To improve efficiency:
-
Focus on specific filters to limit the amount of data returned, reducing processing time.
-
Select only the necessary properties with `Select-Object` to minimize resource consumption.
Error Handling in Queries
It is crucial to implement error handling, especially when executing queries that might fail, such as retrieving data from remote systems. The `Try-Catch` block is a practical way to manage errors gracefully.
For instance:
Try {
Get-Process -Id 9999
} Catch {
Write-Host "Process not found."
}
This code attempts to retrieve a process with a specific ID and provides a user-friendly message if the process does not exist.
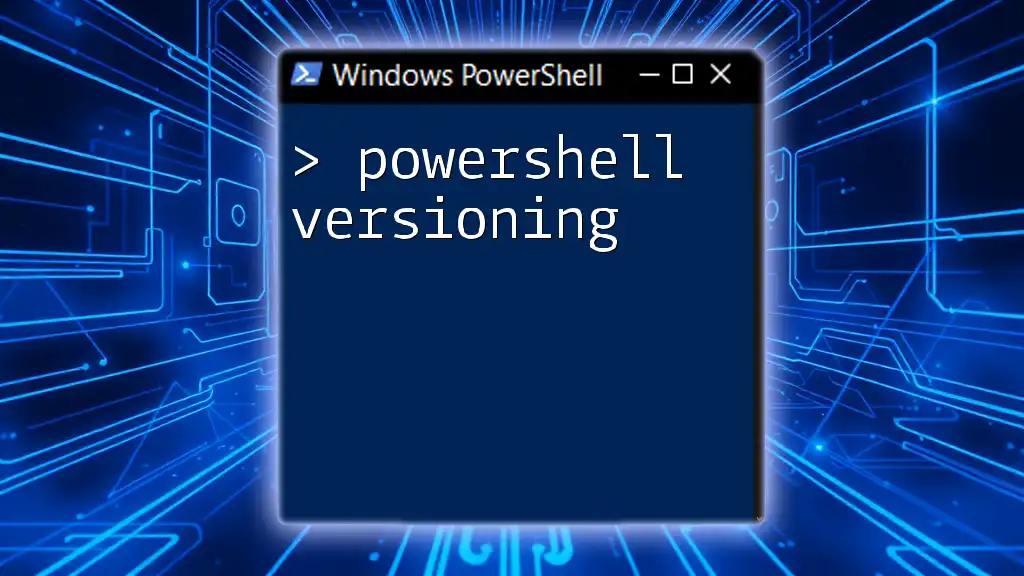
Resources for Further Learning
Several resources can aid your PowerShell journey:
-
Books such as "Windows PowerShell in Action" offer in-depth insights.
-
Online platforms like Microsoft Learn provide extensive documentation and guided tutorials.
-
Community forums and user groups can be excellent for sharing knowledge and experiences.
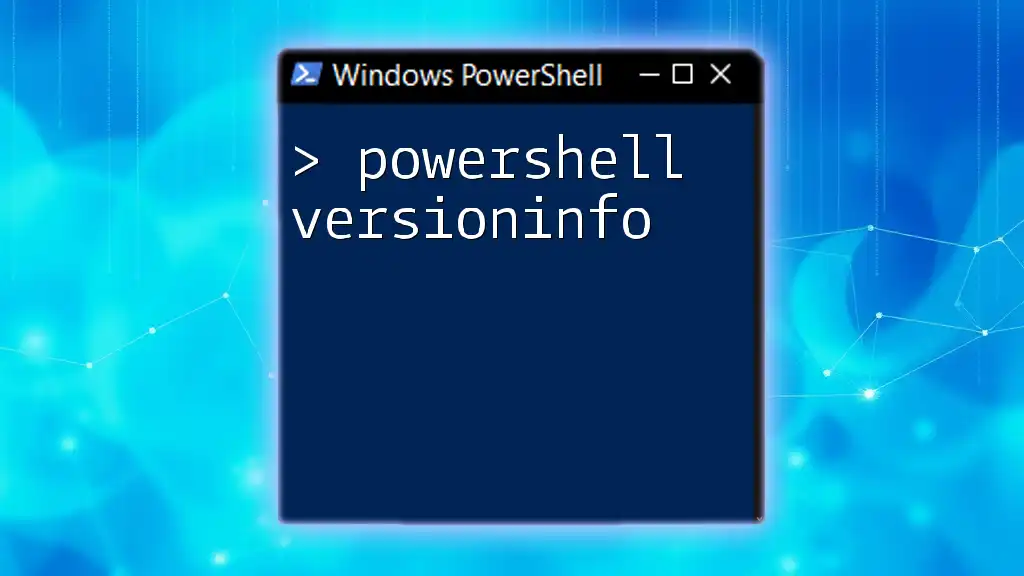
Conclusion
Learning to effectively utilize PowerShell queries is essential for maximizing your productivity, especially in administrative roles. By mastering both basic and advanced querying techniques, you can streamline your workflows and enhance system management. Embrace the power of PowerShell and start querying with confidence!
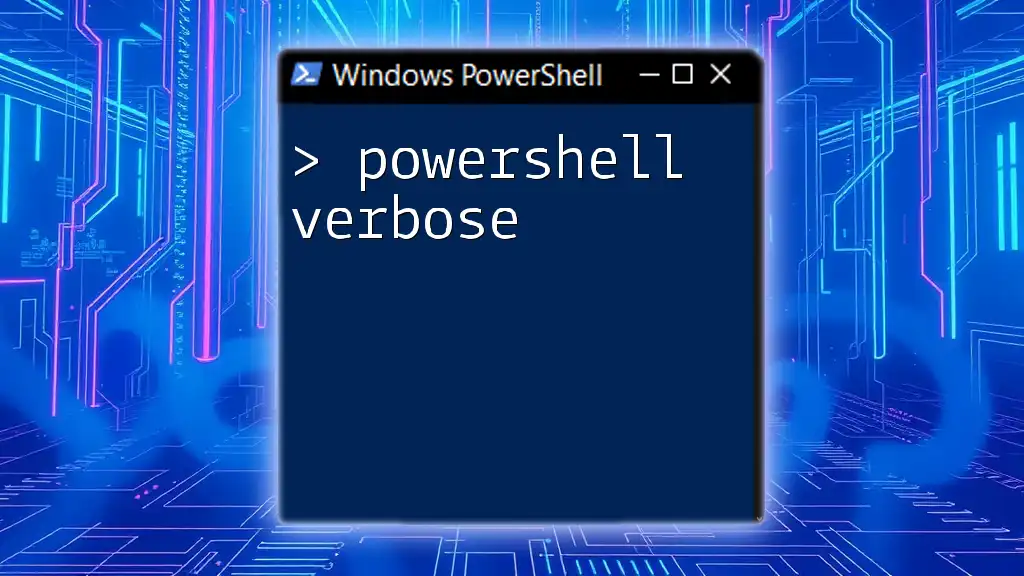
FAQs
Common Questions About PowerShell Queries
-
What are some common errors when using PowerShell queries? Errors can occur due to syntax issues, missing permissions, or unreachable remote hosts.
-
How can I automate PowerShell queries? You can automate queries with scheduled tasks or by creating scripts that execute the necessary commands.
-
Where can I find more examples of PowerShell queries? Online resources, PowerShell documentation, and community forums are great places to discover more examples and scripts.