PowerShell Gzip is a method used to compress and decompress files efficiently using the Gzip algorithm within PowerShell scripts.
Here’s a simple code snippet for compressing a file using Gzip in PowerShell:
# Compress a file using Gzip
Compress-Archive -Path "C:\Path\To\Your\File.txt" -DestinationPath "C:\Path\To\Your\File.gz"
What is Gzip?
Gzip is a widely used file compression format that allows users to reduce the size of files and folders, making them easier to store and share. Understanding how to utilize Gzip effectively can help streamline data transfer and optimize storage, especially in IT environments where large files are commonplace.
How Gzip Works
Gzip operates by applying a compression algorithm, which reduces the size of data files while retaining the original information. It works by identifying and eliminating redundant data, which allows for a significant reduction in file size. The benefits of using Gzip include faster upload and download times, reduced bandwidth usage, and improved overall storage efficiency.
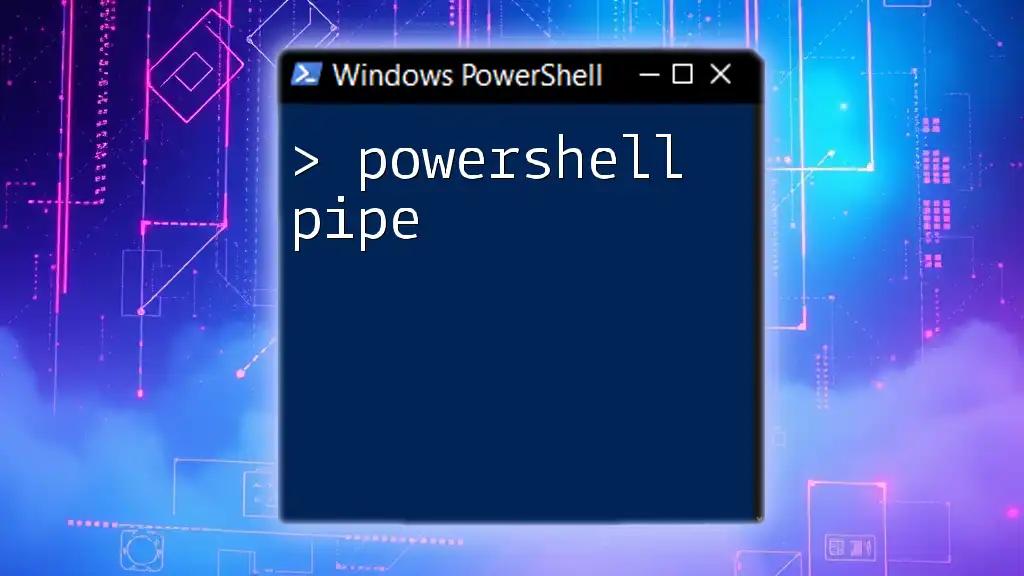
Setting Up PowerShell for Gzip
Before diving into Gzip commands, it's important to ensure that your PowerShell environment is ready for use.
Installing PowerShell (if necessary)
For those who may not have PowerShell installed, please refer to the official Microsoft documentation for detailed installation instructions. This will typically involve downloading the desired version of PowerShell compatible with your operating system.
Required Modules
The good news is that PowerShell natively supports Gzip functionality. You generally won't need to install additional modules to work with Gzip, as the Cmdlets for compression and decompression are built into the framework.
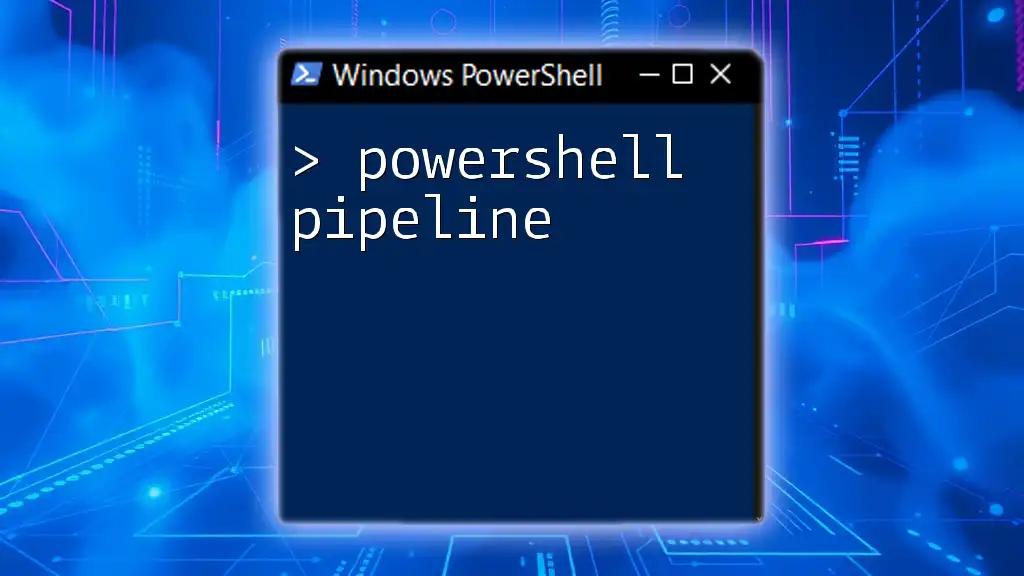
Basic Gzip Commands in PowerShell
Now that your PowerShell environment is set up, let's explore some basic Gzip commands.
Compressing Files with Gzip
To compress a file using Gzip, you can use the Compress-Archive command. The basic syntax for this command is straightforward:
Compress-Archive -Path "C:\example\file.txt" -DestinationPath "C:\example\file.gz"
In this example, PowerShell takes the file located at "C:\example\file.txt" and creates a compressed version called "file.gz" in the same directory. This is an efficient way to minimize file size for storage or transmission.
Decompressing Gzip Files
When you need to restore the original file, you can use the Expand-Archive command. Its syntax is similarly simple:
Expand-Archive -Path "C:\example\file.gz" -DestinationPath "C:\example\"
This command extracts the contents of "file.gz" back to its original format in the specified destination.
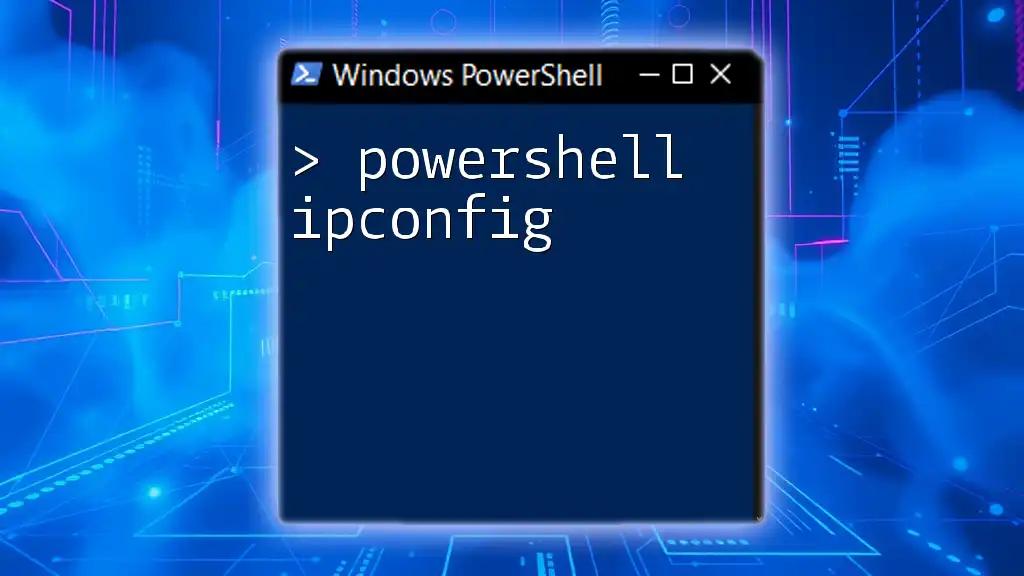
Advanced Gzip Techniques in PowerShell
Once you are comfortable with basic commands, you can move into more advanced techniques.
Using Gzip with Multiple Files
Compressing Multiple Files
To compress multiple files at once, you can utilize the Get-ChildItem command combined with Compress-Archive. This approach is particularly useful for batching.
Get-ChildItem "C:\example\*.txt" | Compress-Archive -DestinationPath "C:\example\archive.zip"
In this example, all text files in the specified directory are compressed into a single ZIP archive named "archive.zip."
Decompressing Multiple Files
You can also handle the decompression of multiple Gzip files with a simple loop. Here’s how you can achieve this:
Get-ChildItem "C:\example\*.gz" | ForEach-Object { Expand-Archive -Path $_.FullName -DestinationPath "C:\example\uncompressed\" }
This command will decompress each Gzip file found in the specified directory, extracting them to the "uncompressed" directory.
Error Handling and Troubleshooting
When using Gzip commands, you may encounter various errors. Common issues can include file not found errors or permission issues. It's imperative to ensure that the specified paths are correct and that you have the necessary permissions to access those files.
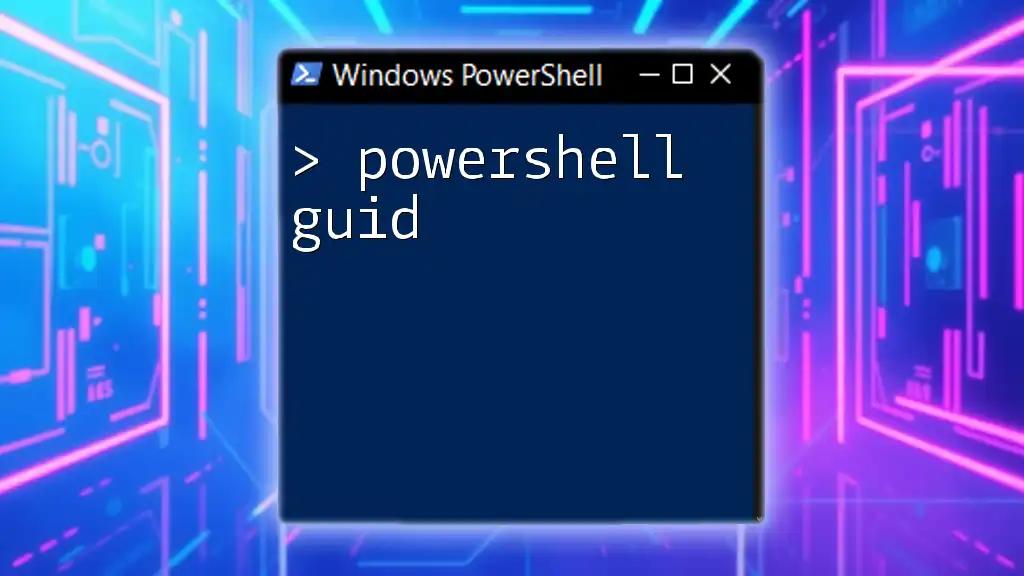
Optimizing Gzip Compression
Choosing the Right Compression Level
PowerShell allows you to choose different compression levels to balance between speed and file size. The levels range from Optimal to Fastest. Here’s an example of how to specify the compression level:
$CompressionLevel = [System.IO.Compression.CompressionLevel]::Optimal
Choosing the right level can significantly affect the time it takes to compress files and the resulting file size.
Performance Considerations
When working with large files, factors such as disk speed and system resources can affect compression time. It's essential to understand the capabilities of your hardware and optimize your scripts accordingly by potentially batching processes or filtering files based on size.

Integrating Gzip in PowerShell Scripts
Creating Automated Scripts
Automating common Gzip tasks can save a great deal of time and effort. Below is an example of a script that compresses and then decompresses files in a specified folder:
$files = Get-ChildItem "C:\example\*.txt"
foreach ($file in $files) {
Compress-Archive -Path $file.FullName -DestinationPath "$($file.BaseName).gz"
Expand-Archive -Path "$($file.BaseName).gz" -DestinationPath "C:\example\decompressed\"
}
This script processes each text file in the directory, compressing it, and then decompressing it immediately afterward.
Scheduling and Automation
Finally, to run your Gzip scripts automatically, you can utilize the Task Scheduler in Windows. By setting up a scheduled task, you can execute your PowerShell scripts at specified intervals, ensuring that your data handling remains efficient without manual intervention.
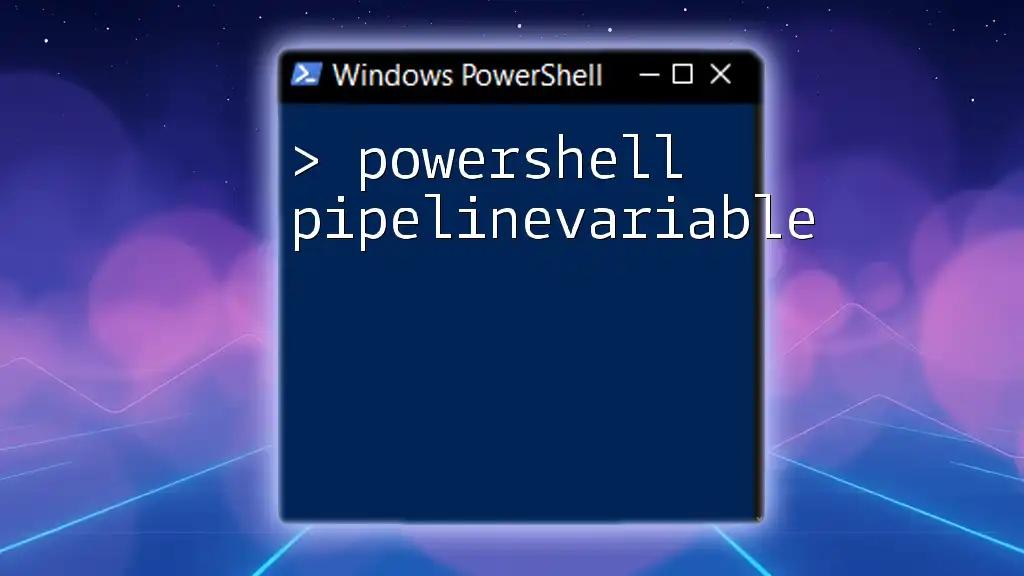
Conclusion
Throughout this guide, we've explored the essential functions of PowerShell Gzip, including compressing and decompressing files, handling multiple files, and optimizing performance. Implementing Gzip commands effectively can significantly enhance your data management skills, leading to faster transfers and better storage solutions. We encourage you to practice these commands and consider joining our course for deeper dives into advanced PowerShell techniques.
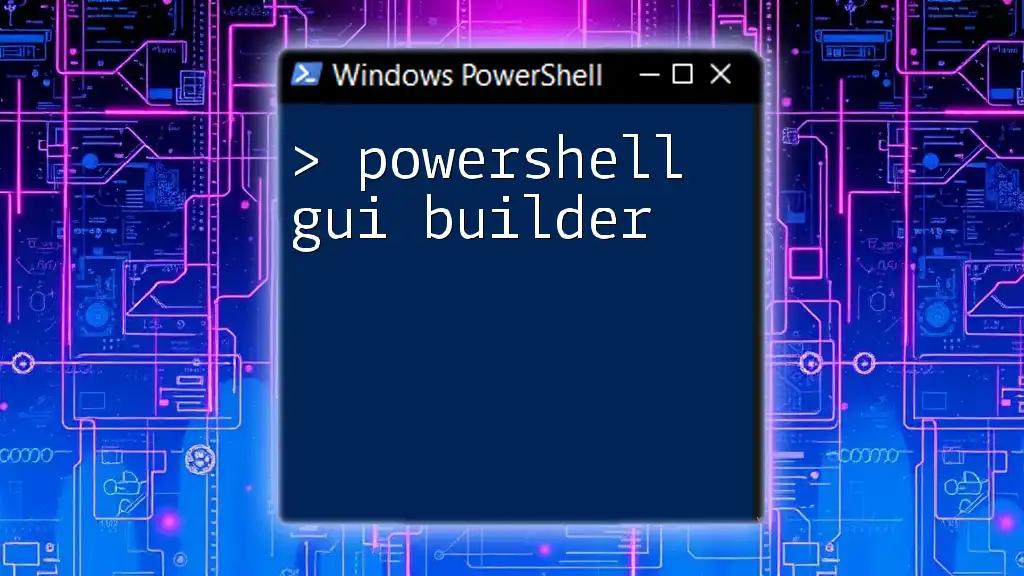
Additional Resources
For those looking to further their knowledge, please check the official PowerShell documentation and explore additional reading materials on file compression algorithms and best practices for effective data management in IT settings.