To quickly retrieve free disk space on your system using PowerShell, you can utilize the following command:
Get-PSDrive -PSProvider FileSystem | Select-Object Name, @{Name='FreeSpace(GB)';Expression={[math]::round($_.Free/1GB,2)}}
Understanding PowerShell Commands
What are PowerShell Commands?
PowerShell commands are designed to interact with the operating system and automate tasks. They are encapsulated in what are known as cmdlets, which follow a simple Verb-Noun naming convention (e.g., `Get-Process`, `Set-Location`). Understanding this structure is essential for performing any operations in PowerShell effectively.
Basic Structure of a PowerShell Command
The syntax is straightforward. A basic command will often look like this:
Verb-Noun -Parameter Value
For instance, the command `Get-Help` retrieves the help documentation for a specific cmdlet. This structure allows users, even those new to PowerShell, to grasp the commands quickly.
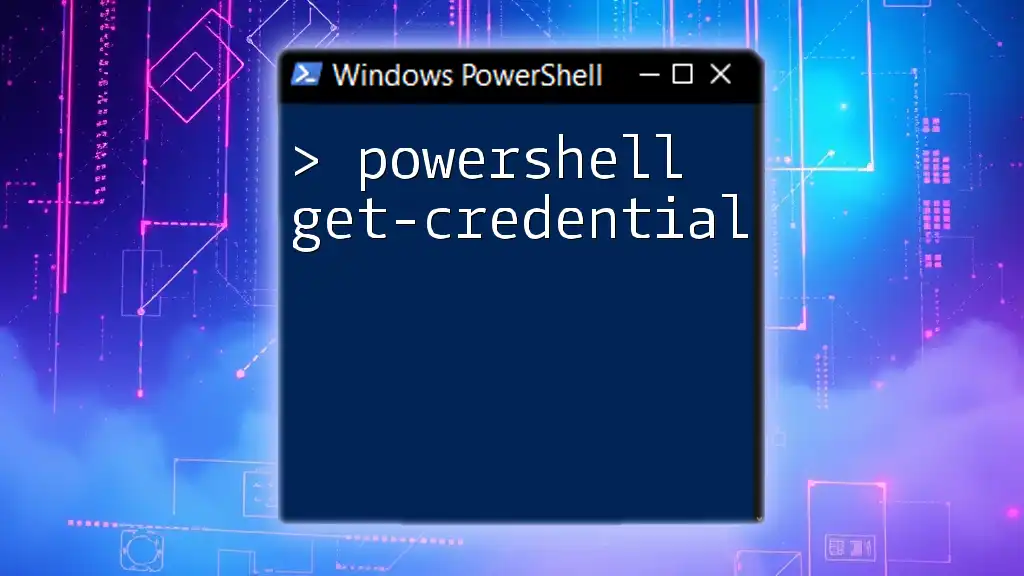
Checking Free Disk Space with PowerShell
Using `Get-PSDrive`
The `Get-PSDrive` cmdlet is fundamental when checking the available disk space. It lists all the drives in the PowerShell session.
Example Usage of `Get-PSDrive`
To quickly view the available disk space, run:
Get-PSDrive -PSProvider FileSystem
This command retrieves all file system drives, displaying information such as used and free space. You will find output fields like `Used`, `Free`, and `Provider` that help you understand your current disk space allocation.
Using `Get-WmiObject`
The `Get-WmiObject` cmdlet allows you to query various management information from your operating system, including disk space details.
Example Command to Get Free Disk Space
To get a more detailed view of your drive's free space, use:
Get-WmiObject Win32_LogicalDisk | Select-Object DeviceID, FreeSpace, Size
In this command:
- `Win32_LogicalDisk` represents the WMI class you are querying.
- The `Select-Object` cmdlet formats the output to include only the device ID, free space, and total size of the drives.
This will yield output that illustrates how much free space exists in bytes, which may be difficult to read at first glance.
Using `Get-CimInstance` (Preferred Method)
The `Get-CimInstance` cmdlet is a newer, more efficient way to retrieve management information compared to `Get-WmiObject`.
Example Command for Disk Space
You can easily check your disk space using:
Get-CimInstance Win32_LogicalDisk | Select-Object DeviceID, FreeSpace, Size
This cmdlet performs similarly to `Get-WmiObject`, offering an output with the same essential data. However, it uses the CIM framework, which leads to improved performance and compatibility.
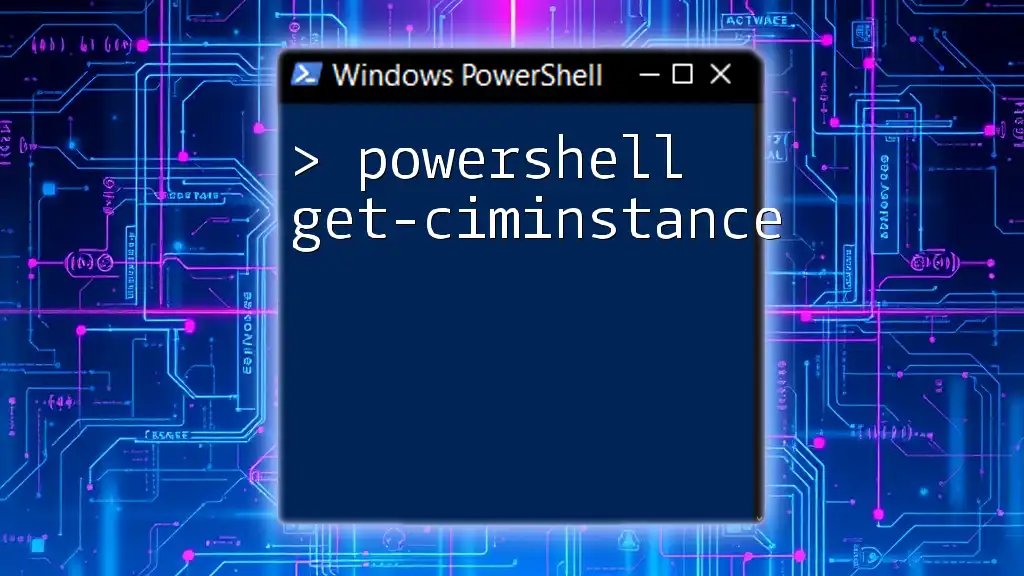
Formatting the Output
Presenting Disk Space in GB or MB
When managing disk space, displaying data in more user-friendly units can be incredibly helpful. You can convert bytes to gigabytes or megabytes by using calculated properties.
Example Code for Conversion
To display the free space in gigabytes, use the following command:
Get-CimInstance Win32_LogicalDisk | Select-Object DeviceID, @{Name="FreeSpace(GB)";Expression={[math]::round($_.FreeSpace/1GB, 2)}}, @{Name="Size(GB)";Expression={[math]::round($_.Size/1GB, 2)}}
In this example:
- The `@{Name=...;Expression=...}` syntax creates calculated properties to show free space and total size in more digestible formats.
Using Measure-Object for Summarization
You can summarize total free space across all drives using `Measure-Object` in combination with `Get-CimInstance`.
Example for Summarizing
Here’s a command to get the total free space in gigabytes:
(Get-CimInstance Win32_LogicalDisk | Measure-Object -Property FreeSpace -Sum).Sum / 1GB
This command effectively aggregates the `FreeSpace` property of each logical disk, providing a quick snapshot of your total free disk space in gigabytes.
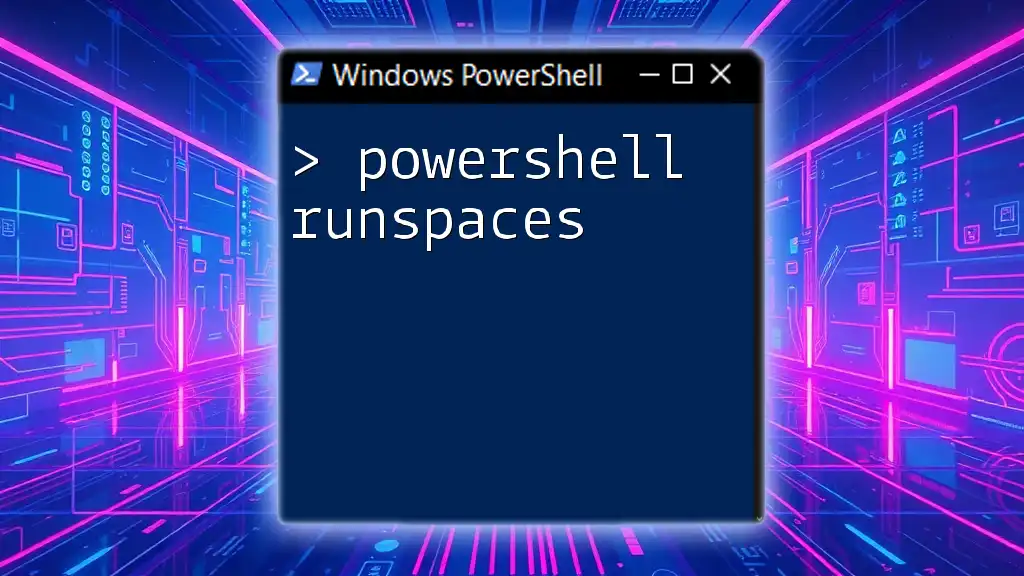
Automating Disk Space Monitoring
Creating a Script to Monitor Disk Space
To maintain system health, routine checks of disk space are beneficial. You can create a PowerShell script to monitor this.
Example Disk Space Monitoring Script
Below is a simple script that displays ads drive with low disk space:
$threshold = 10GB
Get-CimInstance Win32_LogicalDisk | Where-Object { $_.FreeSpace -lt $threshold } | Select-Object DeviceID, FreeSpace
This script checks each logical disk and flags any that fall below a specified threshold—in this case, 10 gigabytes. This is a valuable preventive measure that can help avoid space-related issues.
Scheduling Disk Space Checks Using Task Scheduler
To automate this script, consider using Windows Task Scheduler. Set up a task to run the script at regular intervals (daily or weekly) to ensure you are always informed about your disk's free space status. This can enhance operational efficiency and proactively prevent space shortages.
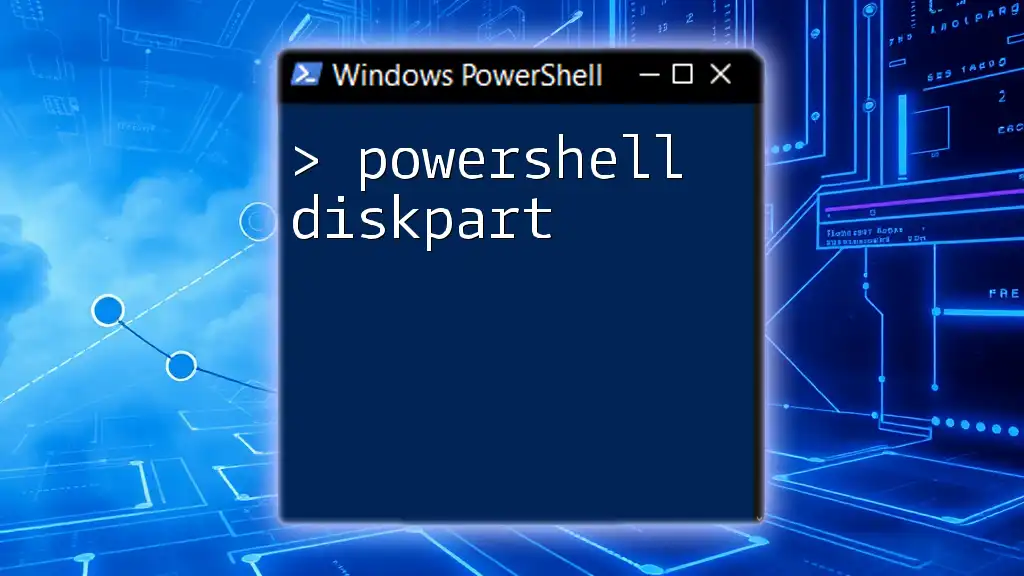
Best Practices for Managing Disk Space
Regular monitoring and maintenance of your disk space are crucial. Failure to do so can lead to decreased system performance and potential data loss.
- Regular Monitoring: Schedule weekly or even daily checks of your disk space.
- Setting Alerts: Use scripts to send alerts or notifications when disk space falls below a certain threshold to address potential issues before they escalate.
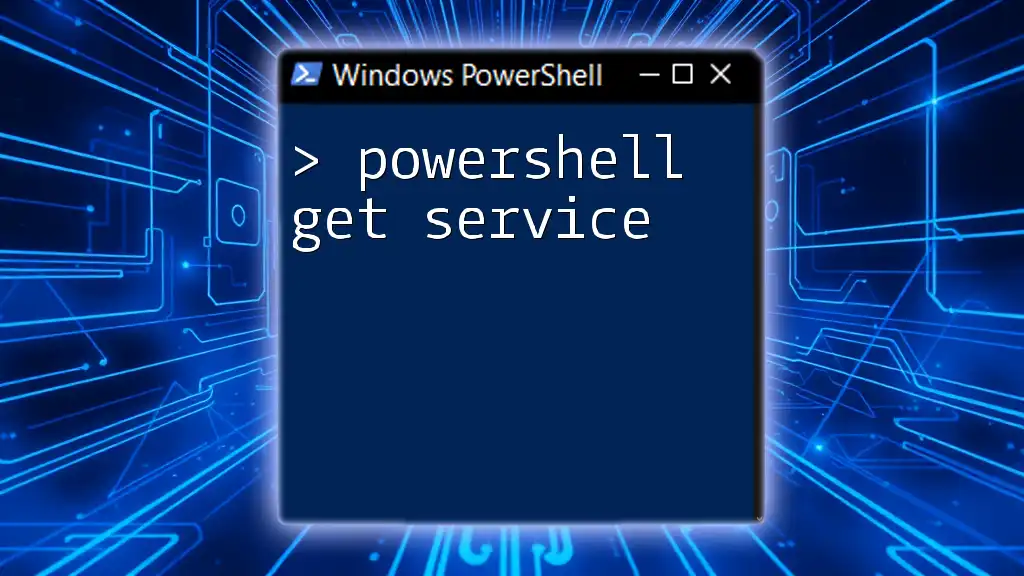
Conclusion
Monitoring your disk space with PowerShell is not just a technical task but an essential practice for maintaining system integrity. By leveraging the `Get-CimInstance` and `Get-PSDrive` cmdlets, along with calculated properties, you can gain valuable insights into your storage health. Automating the process ensures that you remain informed, enabling you to take the necessary actions to keep your system running smoothly.
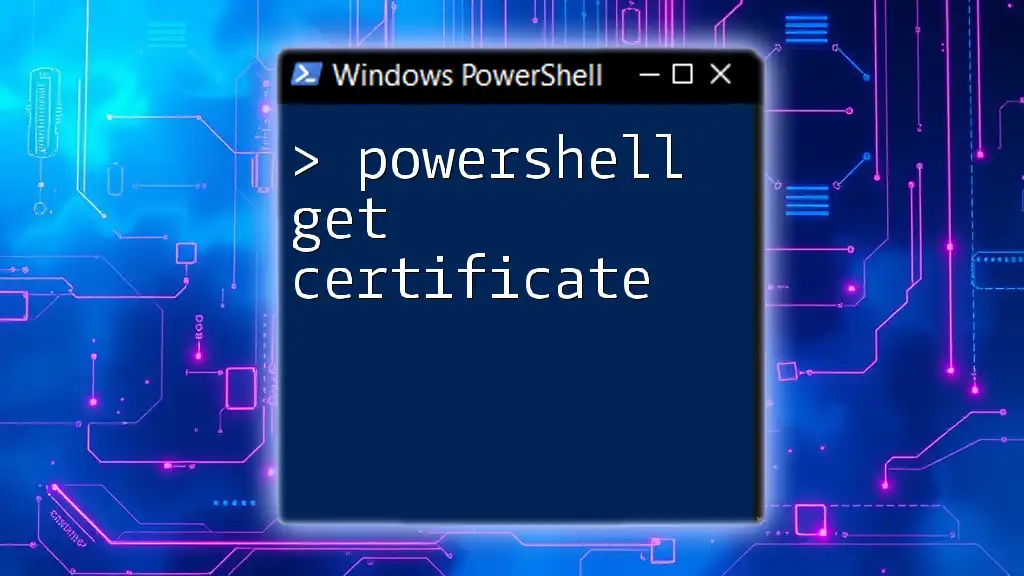
Additional Resources
For further study, consult the official Microsoft documentation on PowerShell commands and explore additional resources on disk management best practices. By enhancing your skills in PowerShell disk space management, you can contribute to a more efficient and productive computing environment.