To retrieve the current logged-on user from a remote computer using PowerShell, you can utilize the `Get-WmiObject` cmdlet as demonstrated in the following code snippet:
Get-WmiObject -Class Win32_ComputerSystem -ComputerName "RemoteComputerName" | Select-Object -ExpandProperty UserName
Make sure to replace `"RemoteComputerName"` with the actual name of the remote computer you wish to query.
Understanding PowerShell Remote Management
What is PowerShell Remoting?
PowerShell Remoting is a feature that allows users to run commands on remote computers. It is an essential tool for system administrators and IT professionals who need to manage multiple machines from a single point of control. This capability is especially useful for performing tasks like querying system information, updating software, or even troubleshooting issues without needing to physically access each machine.
Prerequisites for PowerShell Remoting
Before using PowerShell remoting, certain configurations must be in place:
-
Enabling PowerShell Remoting: Ensure that the remote computer has PowerShell Remoting enabled. This can typically be done by running the following command on the target machine:
Enable-PSRemoting -Force
-
Configuring Windows Firewall: Make sure that the Windows Firewall on the remote computer is configured to allow PowerShell remoting. The enabling command automatically adjusts the firewall settings as needed.
-
Requirements for Remote Connections: Users must have the right permissions to connect to the remote machine, and ideally, it's beneficial to use credentials that are valid on the target computer.
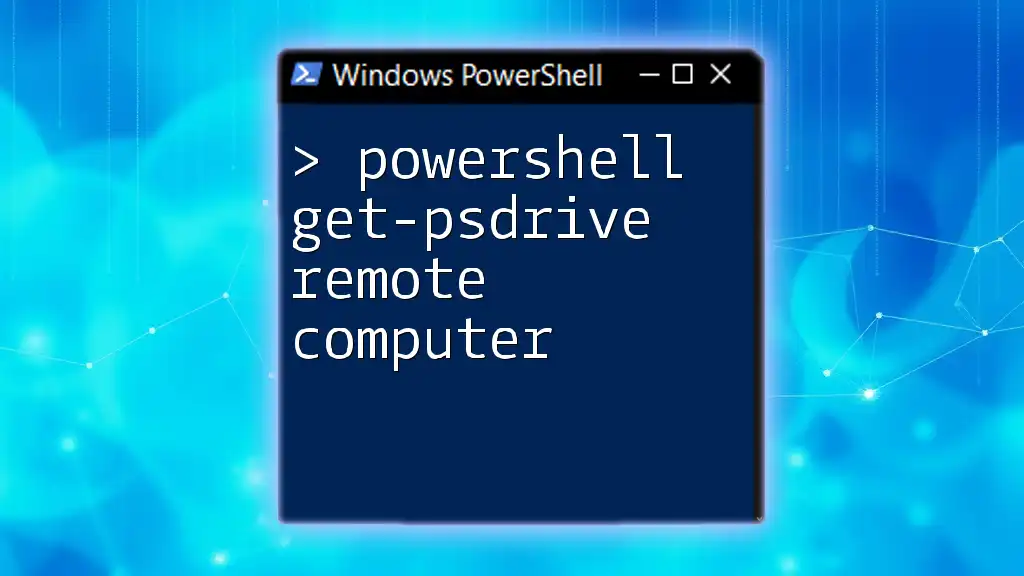
Using PowerShell to Get the Current Logged On User
Overview of Common Cmdlets
To retrieve the current logged-on user on a remote computer, several cmdlets can be utilized, including:
-
Get-WmiObject: This cmdlet is traditional and has been used for a long time to interact with WMI (Windows Management Instrumentation).
-
Get-CimInstance: A more modern replacement for `Get-WmiObject`, which uses WS-Man protocol and supports a broader range of functionalities, particularly in remote scenarios.
-
Invoke-Command: This cmdlet is instrumental when you want to run code on multiple remote machines seamlessly.
Basic PowerShell Command Structure
Each cmdlet works with a consistent command structure, typically following this format:
<CmdletName> -Parameters
Understanding the syntax and available parameters is crucial for crafting effective commands.
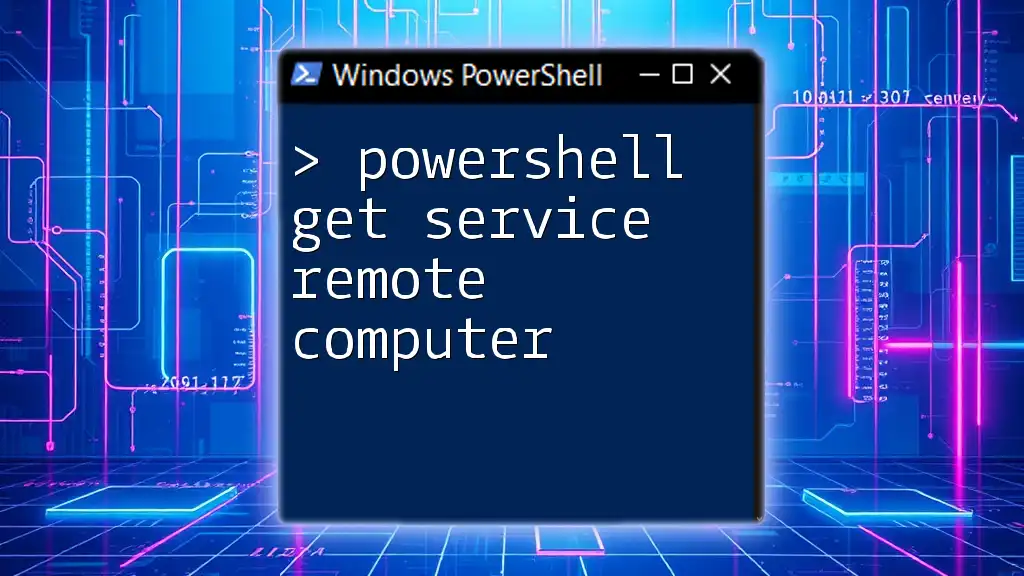
Retrieving the Current Logged On User
Using Get-WmiObject
The `Get-WmiObject` cmdlet can be employed to fetch the current logged-on user information as follows:
$computerName = "RemotePC"
Get-WmiObject -Class Win32_ComputerSystem -ComputerName $computerName | Select-Object -Property UserName
In this command, Win32_ComputerSystem is the WMI class that holds several properties about the machine, including the currently logged-in username. The output will show the UserName property, which contains the name of the user logged in.
Key Point: If you encounter permission issues when running this command, ensure that the credentials used have proper access rights on the remote computer.
Using Get-CimInstance
For a more modern approach, you might prefer to use `Get-CimInstance` instead of `Get-WmiObject`. Here's how you can do it:
$computerName = "RemotePC"
Get-CimInstance -ClassName Win32_ComputerSystem -ComputerName $computerName | Select-Object -Property UserName
This command serves the same purpose as the previous one but utilizes the more efficient CIM framework. It's particularly useful in cases where you need to manage multiple computers or require better performance.
Using Invoke-Command for Querying Multiple Computers
If your goal is to retrieve the current logged-on users from multiple machines simultaneously, `Invoke-Command` will come in handy:
$computerNames = @("RemotePC1", "RemotePC2")
Invoke-Command -ComputerName $computerNames -ScriptBlock { Get-WmiObject -Class Win32_ComputerSystem | Select-Object -Property UserName }
In this example, you can specify an array of computer names. The `-ScriptBlock` parameter allows you to define the commands to run on each remote computer, providing results in a consolidated manner.
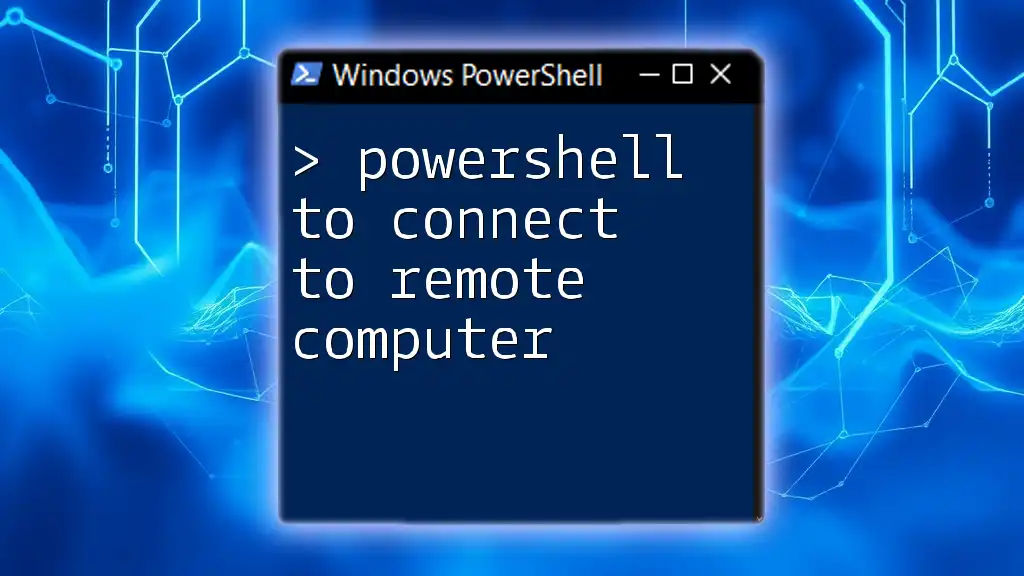
Additional Considerations for Remote User Retrieval
Handling Errors and Exceptions
When working with remote commands, errors and exceptions can arise, especially related to connectivity or permissions. Using `try-catch` blocks will help manage these issues gracefully. Here’s an example:
try {
Get-WmiObject -Class Win32_ComputerSystem -ComputerName "RemotePC"
}
catch {
Write-Output "Error: $_"
}
In this snippet, if there’s an error (e.g., the remote computer is unreachable), the catch block will execute, displaying a message with the error description.
Security Best Practices
Maintaining security is paramount when using PowerShell remoting. Here are some best practices:
-
Use Secure Credentials: Always use the `Get-Credential` cmdlet to securely input usernames and passwords instead of hardcoding them in scripts.
-
Limit Remote Access: Only enable PowerShell remoting on computers that require it, and limit the accessible commands a user can execute remotely.
-
Use HTTPS for Communication: If feasible, configure PowerShell remoting to use HTTPS to encrypt the communication channel, thus ensuring sensitive data remains protected.
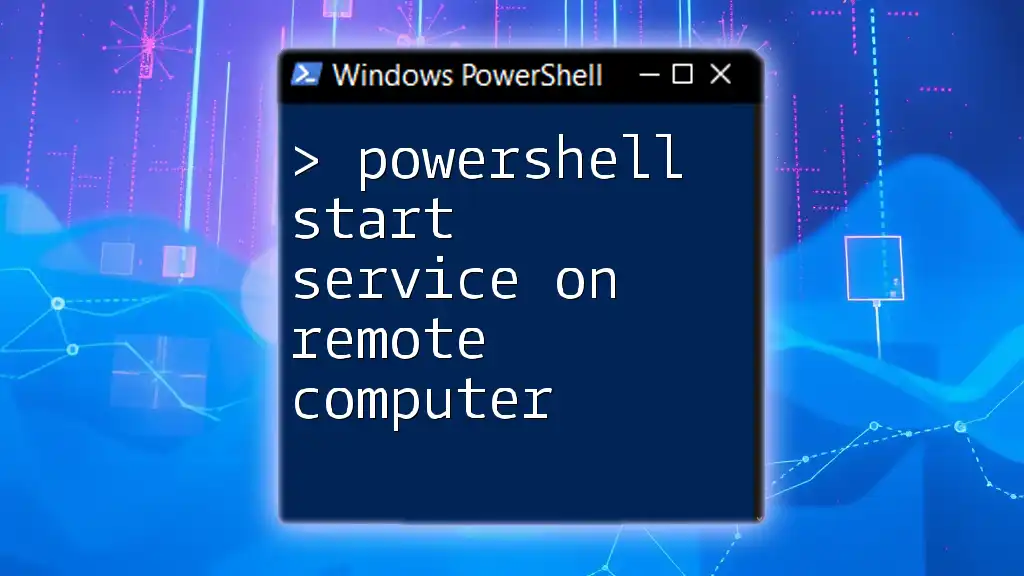
Conclusion
In this comprehensive guide, we've explored how to retrieve the current logged-on user on a remote computer using PowerShell. We've introduced multiple methods and cmdlets that serve different use cases. Remember that each approach has its strengths, so choose the one that best suits your scenario.
PowerShell provides powerful tools for system management, and with practice, you will become proficient in effectively utilizing these commands.
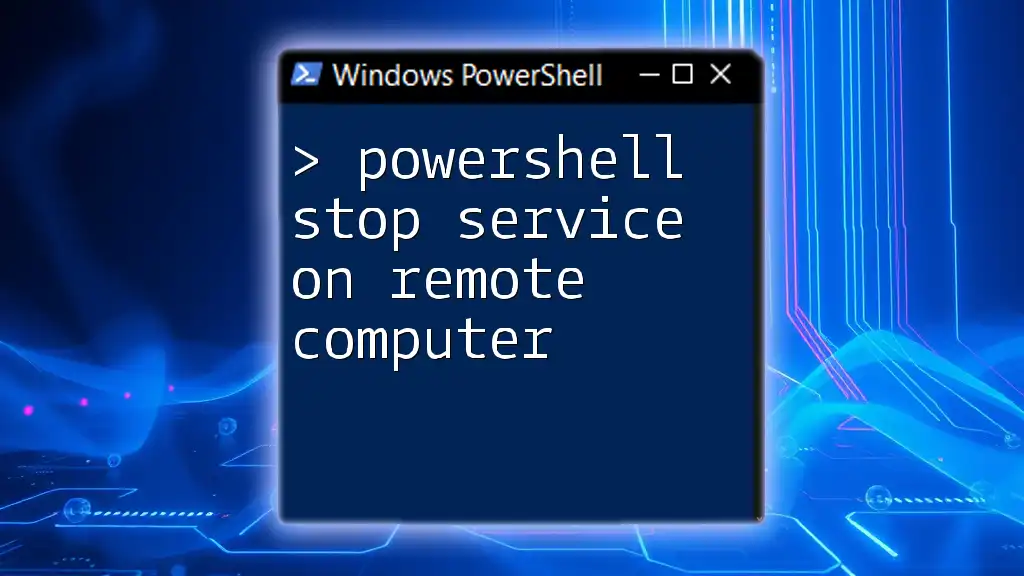
FAQs
What if the remote computer is turned off?
If the remote computer is turned off, you will receive an error indicating that the computer cannot be reached. This is a natural response, as the command needs the target machine to be operational to retrieve information.
Can I access users logged onto a Linux machine?
PowerShell is predominantly used within Windows environments. For Linux systems, different commands or protocols (such as SSH) should be employed to access user information.
Is there a GUI alternative available?
While PowerShell is a command-line tool, several third-party applications provide a GUI for managing remote systems. Tools like Remote Desktop Manager or Microsoft Server Manager allow for user management without needing to resort to command line tools.
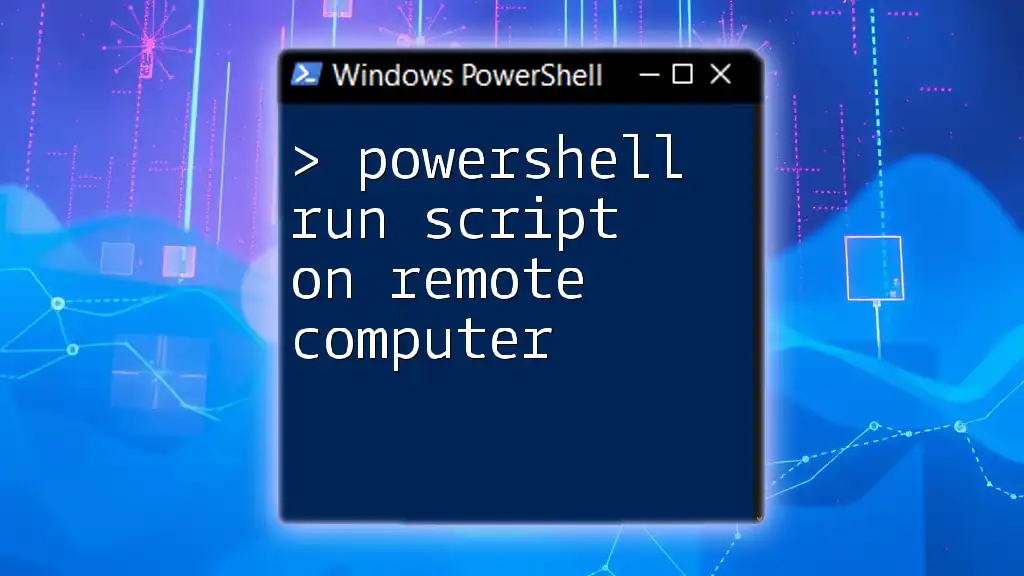
Additional Resources
For more in-depth learning, consider exploring official Microsoft documentation, joining PowerShell communities online, or taking specialized courses tailored to enhance your PowerShell skills.