To convert a Unix timestamp to a DateTime object in PowerShell, you can use the following code snippet to perform the conversion.
$unixTimestamp = 1633024800
$dateTime = [System.DateTime]::FromFileTimeUtc(($unixTimestamp * 10000000) + 621355968000000000)
Write-Host $dateTime
Understanding Unix Timestamp
What is a Unix Timestamp?
A Unix timestamp is a way to track time, defined as the number of seconds that have elapsed since the "Unix Epoch," which is 00:00:00 UTC on 1 January 1970, not counting leap seconds. This time format is commonly used in programming and computing for its simplicity and universality.
Unix timestamps are typically represented as a single integer value, making them efficient for storage and calculations. They play a crucial role in various applications, such as logging events, scheduling tasks, and interacting with APIs that return time-sensitive data.
The Need for Conversion
In scripting and data analysis, developers and system administrators often encounter Unix timestamps. However, these timestamps need conversion to a human-readable format for meaningful interpretation. For example, if a log file generates timestamps, they must be transformed to inform users about the exact date and time of events. Such conversions can also aid in report generation and data visualization.
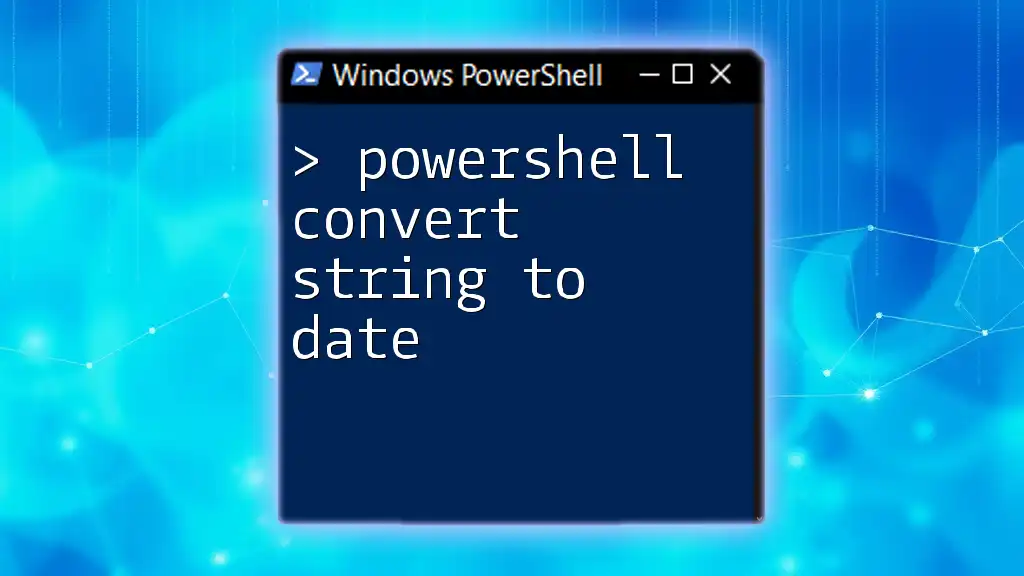
PowerShell Basics
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework from Microsoft, consisting of a command-line shell and an associated scripting language. With the ability to handle complex tasks and manipulate system configurations, PowerShell enables users to automate administrative tasks across Windows and other operating systems.
Key PowerShell Concepts for Beginners
Before diving into converting Unix timestamps, it's essential to grasp some key PowerShell concepts:
-
Cmdlets: These are built-in PowerShell functions that perform a specific action. They follow a verb-noun format, such as `Get-Process`.
-
Objects: PowerShell deals primarily with objects, allowing users to leverage .NET framework capabilities seamlessly.
-
Pipelines: PowerShell allows for piping data between cmdlets, enhancing data manipulation and processing capabilities.
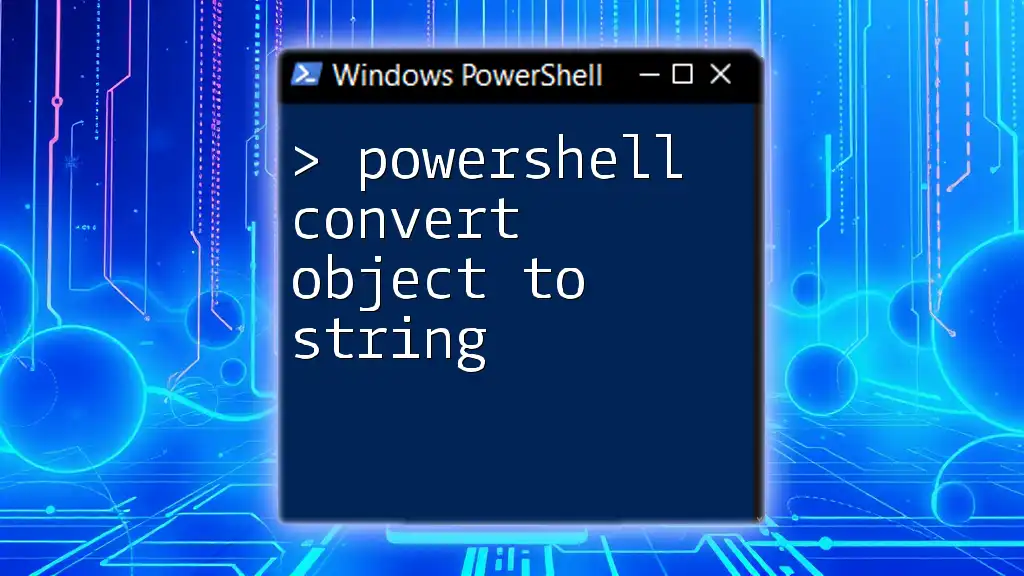
Converting Unix Timestamp to DateTime in PowerShell
Using Get-Date in PowerShell
One of the simplest ways to convert a Unix timestamp to a DateTime object in PowerShell is by using the `Get-Date` cmdlet. Below is a basic example:
# Convert Unix timestamp to DateTime
$timestamp = 1633036800
$dateTime = Get-Date -UnixTimeSeconds $timestamp
$dateTime
In the example above:
- $timestamp: We initialize this variable with a Unix timestamp value (`1633036800`).
- Get-Date: This cmdlet is then used with the `-UnixTimeSeconds` parameter to convert the Unix timestamp to a DateTime object.
- Output: Running this code will return a DateTime value that we can read and understand, such as `10/1/2021 12:00:00 AM`.
Step-by-Step Breakdown of the Code
Explanation of Variables
The `$timestamp` variable represents the Unix timestamp in seconds. This integer value is crucial for the conversion process.
Using Get-Date
The `Get-Date` cmdlet is powerful in PowerShell. The `-UnixTimeSeconds` parameter tells PowerShell to interpret the provided number as a Unix timestamp, facilitating a straightforward conversion to a DateTime format.
Output
The output from the function provides a DateTime object, which can then be used directly in further scripts, displayed to users, or logged.
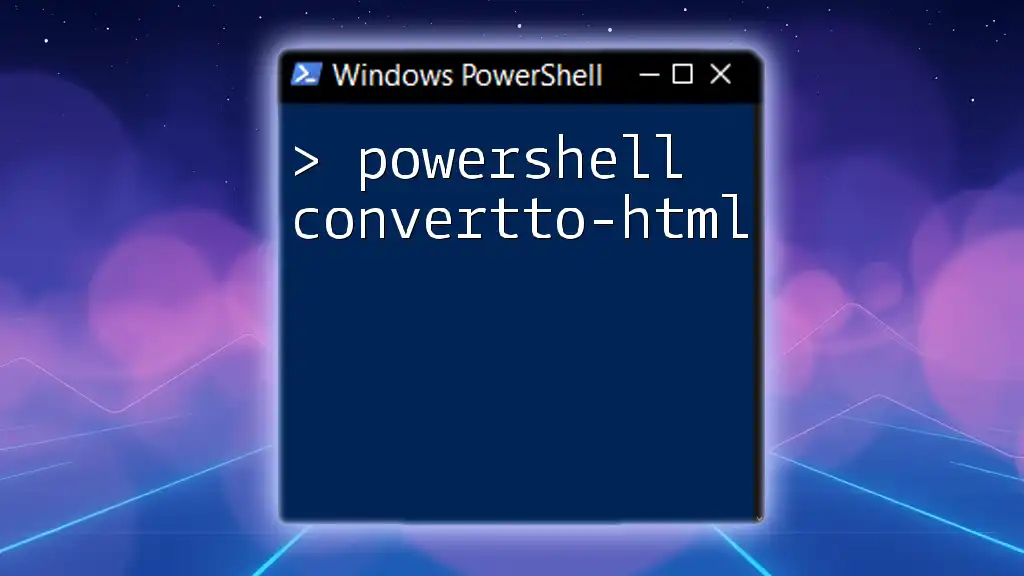
Advanced Conversion Techniques
Handling Different Time Zones
Time zones play a significant role when converting timestamps, as they can lead to discrepancies in the output. When working with different time zones, you may want to adjust the DateTime object accordingly. Here’s an example of handling time zones:
$timestamp = 1633036800
$dateTime = [System.DateTime]::FromFileTime([long]$timestamp*10000000)
$dateTime.ToLocalTime()
In this code:
- FromFileTime: This method is used to convert the Unix timestamp, but it first requires the timestamp to be multiplied by `10000000` because it's expected in .NET's file time format.
- ToLocalTime: Converts UTC time to local time, depending on your system's configuration.
Converting an Array of Timestamps
Often, you may encounter multiple timestamps that require conversion. Instead of converting them one by one, you can leverage the `ForEach-Object` cmdlet to handle an array. For instance:
$timestamps = @(1633036800, 1672537600, 1609459200)
$dateTimes = $timestamps | ForEach-Object { Get-Date -UnixTimeSeconds $_ }
$dateTimes
In this snippet:
- Array of Timestamps: We create an array containing multiple Unix timestamps.
- ForEach-Object: This cmdlet processes each timestamp individually and converts it to DateTime.
Handling Invalid Timestamps
When working with data, you might come across invalid timestamps. Implementing validation can prevent errors. Here’s an example:
function Convert-UnixTime {
param (
[int64]$timestamp
)
if ($timestamp -lt 0) {
throw "Invalid Unix Timestamp"
}
return Get-Date -UnixTimeSeconds $timestamp
}
In this function:
- Validation Check: The function checks if the timestamp is less than zero. If it is, an exception is raised.
- Conversion: If the timestamp is valid, it proceeds to convert it.
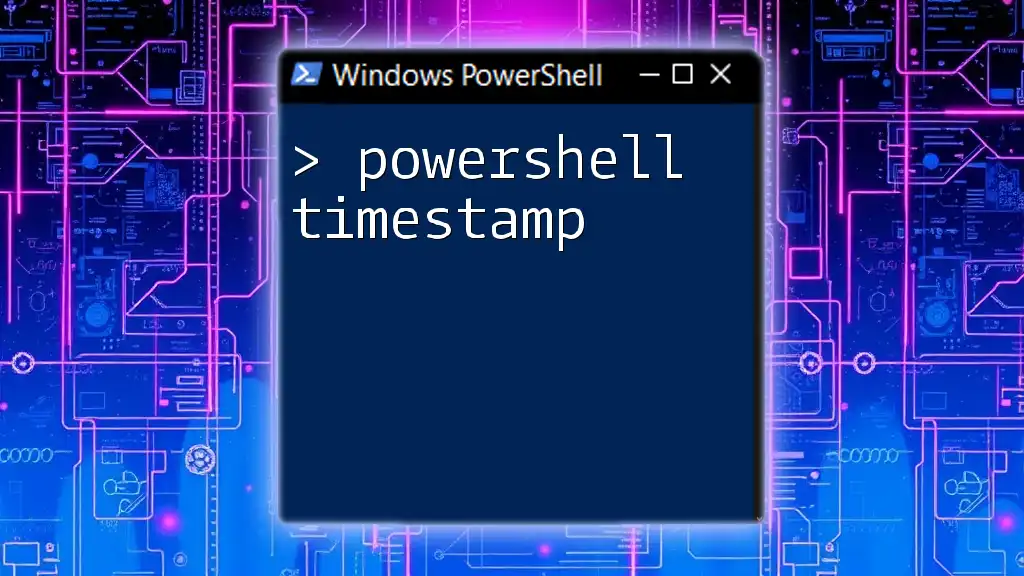
Practical Applications of Conversion
Use Cases in Scripting
The conversion of Unix timestamps is prevalent in script automation, particularly when generating reports or scheduling tasks. For instance, if a system regularly logs events using Unix timestamps, converting these timestamps allows admins to generate readable logs that inform actions and decisions.
Data Analysis with PowerShell
In data analysis, transforming Unix timestamps aids in preparing datasets for insights. Once converted, timestamps can be merged with other DateTime data types, enriching the datasets, and allowing for comprehensive analysis, trend identification, and decision-making.
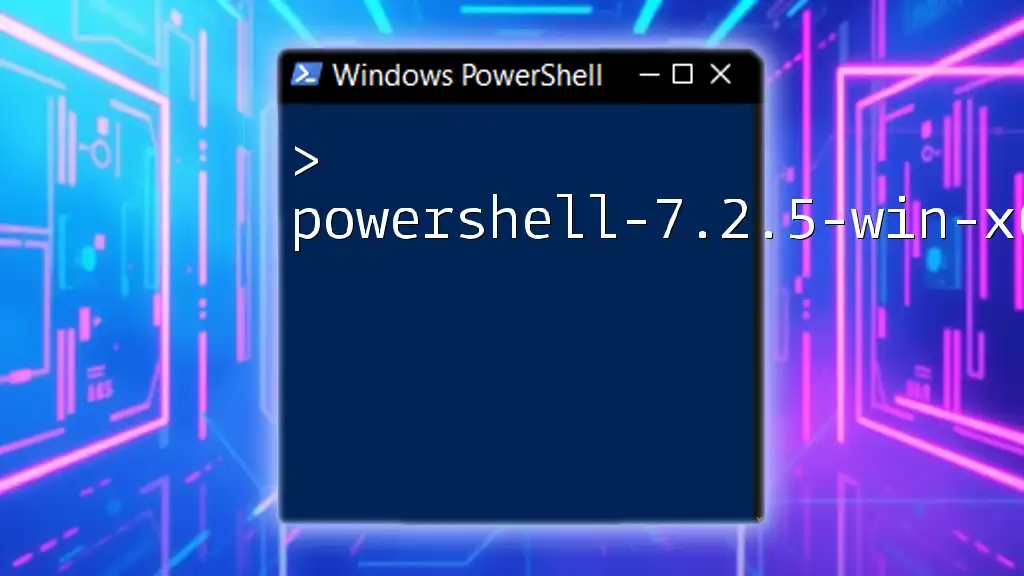
Conclusion
Understanding how to PowerShell convert Unix timestamp to DateTime is fundamental for various tasks in scripting, data analysis, and system administration. This guide has covered the core concepts behind Unix timestamps and provided practical examples to help you integrate this functionality into your workflows.
Practice with different timestamps and scenarios to enhance your skills, and don’t hesitate to reach out for assistance or clarification as you embark on your PowerShell journey!
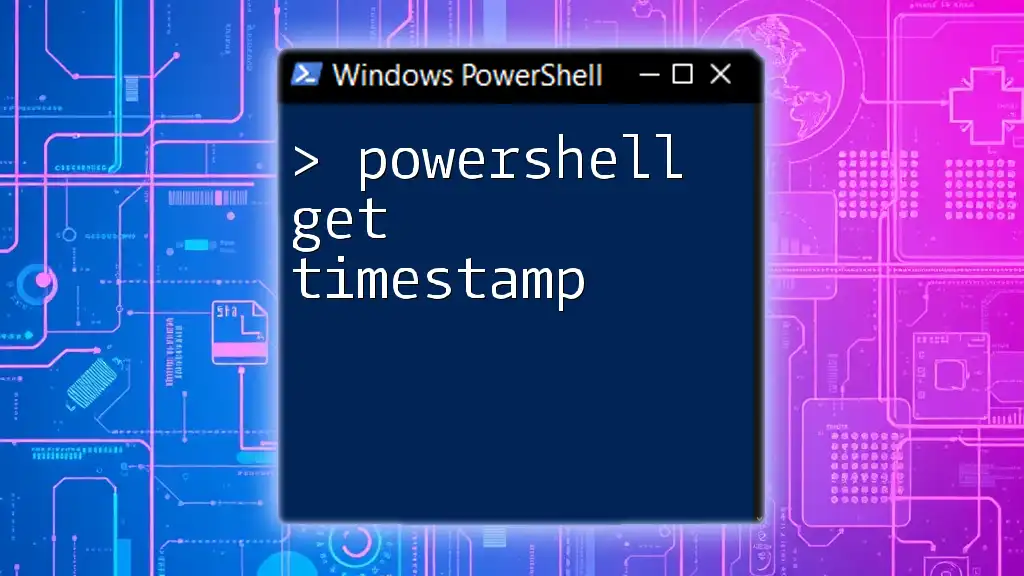
Additional Resources
For those looking to deepen their knowledge, various resources are available:
- PowerShell documentation by Microsoft
- Recommended books on PowerShell scripting
- Online courses that focus on PowerShell for system administrators
Explore these materials to continue honing your PowerShell expertise!