In PowerShell, you can comment out a line of code using the `#` symbol, which allows you to include notes or disable code execution without affecting performance.
Here’s a code snippet demonstrating how to comment out a line:
# This is a comment that will not be executed
Write-Host 'Hello, World!'
What Does "Comment Out" Mean in PowerShell?
In PowerShell, to “comment out” means to annotate or disable code temporarily. Comments provide valuable insights to anyone reading the code later—including yourself. They clarify the purpose of the code, explain complex logic, and mark areas that require further attention. While executing a script, any text following a comment symbol is ignored, allowing you to focus on specific parts without affecting functionality.

Types of Comments in PowerShell
Single-Line Comments
In PowerShell, single-line comments begin with the `#` symbol. Everything that follows `#` on that line will not be executed.
Example:
# This is a single-line comment
Write-Host "Hello, World!" # This prints Hello, World!
This format is ideal for brief annotations or notes. It can clarify what a line of code is doing, making it easier for others (and for you in the future) to understand the rationale behind the code decisions.
Multi-Line (Block) Comments
When you need to comment out multiple lines at once, PowerShell offers a block comment syntax using the `<# ... #>` notation. This allows you to encapsulate a series of lines within the block comment symbols.
Example:
<#
This is a multi-line comment
that spans multiple lines.
#>
Write-Host "This will run"
Block comments are particularly useful when you are temporarily removing code for testing or debugging purposes. They enable you to silence entire sections without needing to prefix each line.

Commenting Out Code Blocks
Why Comment Out Code?
Developers often comment out code as a temporary measure—perhaps while debugging or when they want to analyze the script without executing every line. This practice is essential for maintaining flexibility while developing, allowing for quick rollbacks without permanent changes to your codebase.
How to Comment Out Code Blocks in PowerShell
To comment out a block of code, you can encapsulate it within block comments.
Example:
<#
Write-Host "This code is commented out"
Write-Host "So it won't run"
#>
This approach allows you to keep the code for future reference or analysis, without executing it in your current workflow.
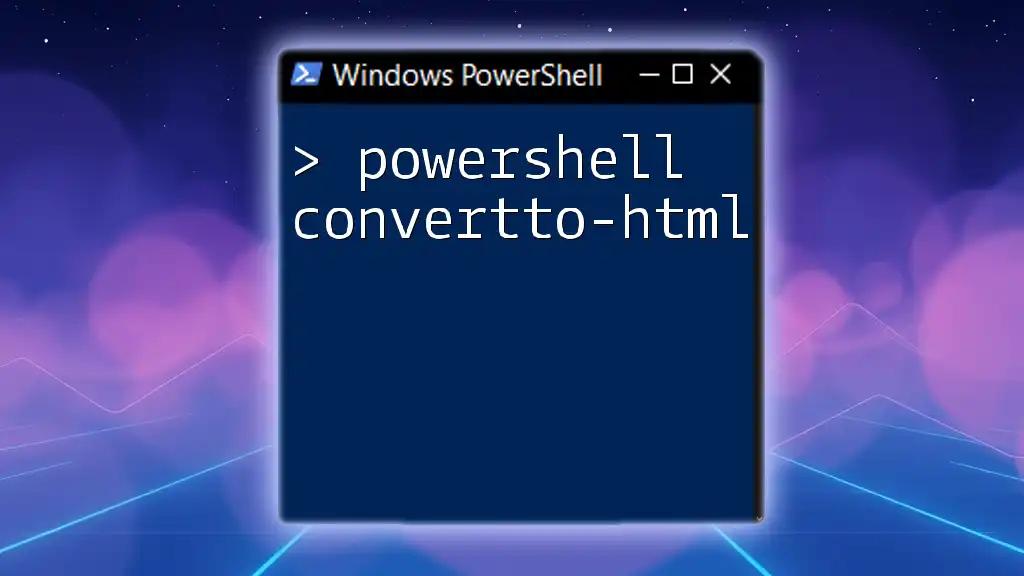
Best Practices for Commenting in PowerShell
Keep Comments Relevant and Clear
Effective comments are relevant and succinct, enhancing the readability of your scripts. Writing clear and concise comments ensures that they serve their purpose without overwhelming the reader.
Imagine someone else reading your code—not every detail needs explaining, but providing context is key. Ask yourself: Will this comment add value? If so, write it clearly.
Use Comments to Document Functionality
Comments should serve as documentation for functions, modules, and scripts. Adding comments that describe what a function does, its parameters, and return values can greatly assist anyone using your code in the future.
Example:
# This function calculates the sum of two numbers
function Add-Numbers {
param (
[int]$a,
[int]$b
)
return $a + $b
}
In this example, the comment serves to inform the user about the purpose and functionality of the `Add-Numbers` function, making it easier to understand its use.
Avoid Over-Commenting
While comments can be incredibly helpful, it’s essential to strike a balance. Excessive comments can clutter your code and obscure the logic instead of clarifying it. Aim for straightforward code that speaks for itself. Use comments to clarify specific points, not to state the obvious.
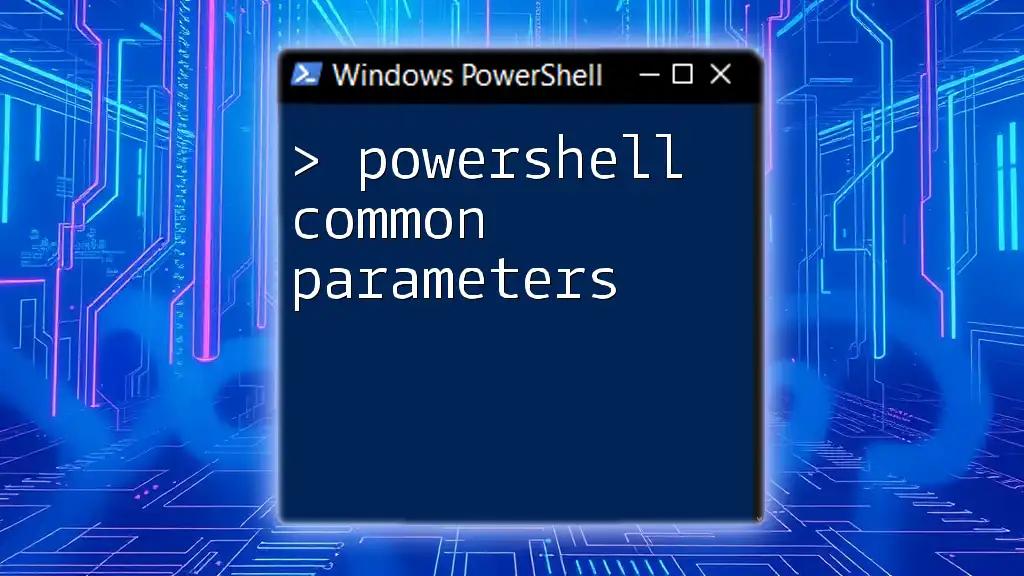
Common Mistakes to Avoid When Commenting
Misplacing Comments
One common error is placing comments too far from the code they describe. Ensure that comments are close to the related code. This proximity helps maintain context and prevents confusion for anyone reading the script later.
Commenting Out Essential Code Unintentionally
Another pitfall is inadvertently commenting out essential parts of your script. Be cautious when using the block comment syntax `<# ... #>`. It's easy to forget where you’ve placed block comments, leading to significant areas of code being disabled until you notice.

Conclusion
Understanding how to use the `powershell comment out` functionality effectively can profoundly impact the maintainability and clarity of your scripts. By skillfully incorporating comments, you enhance not only your coding practices but also collaborate more effectively within a team. Adopting good commenting habits means your scripts will be easier to read and maintain, ultimately making you a better developer.
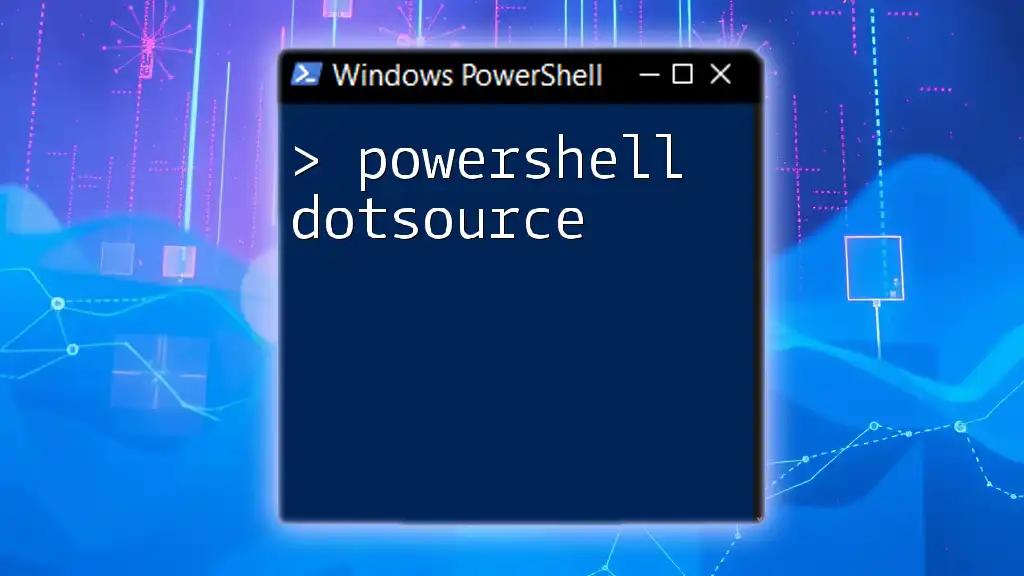
Further Reading
For those eager to expand their PowerShell skills, consider exploring the official Microsoft documentation, community forums, or online courses tailored to PowerShell scripting.
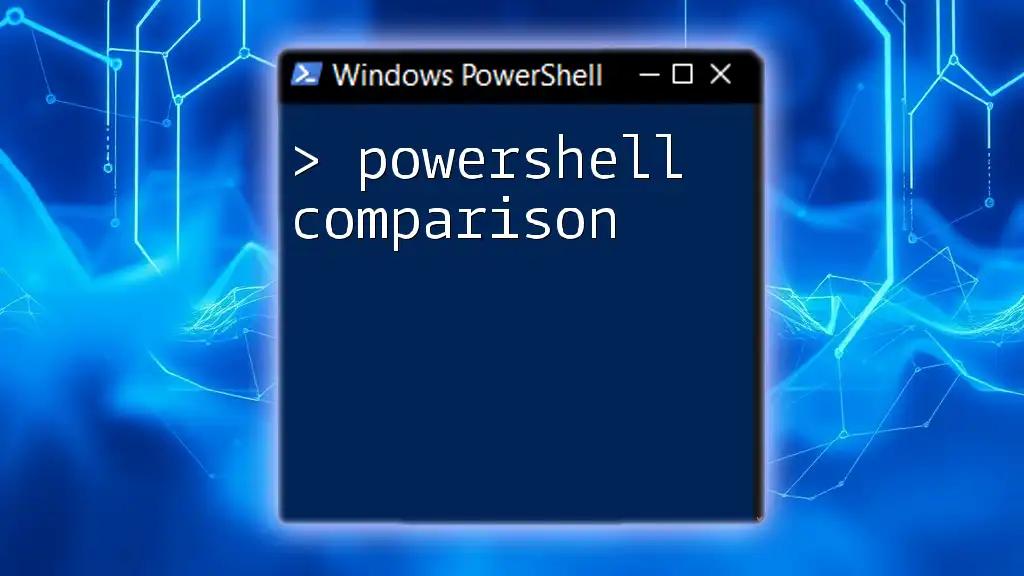
Call to Action
I invite you to share your own experiences or tips regarding commenting in PowerShell. Join our community and enroll in our training program to unlock a comprehensive understanding of PowerShell scripting and best practices!