In PowerShell, you can create a multiple line comment using the `<# #>` syntax, allowing you to include detailed explanations or notes without affecting the execution of your script.
<#
This is a multiple line comment in PowerShell.
It can span multiple lines without interfering with the code.
#>
Write-Host 'Hello, World!'
Understanding Comments in PowerShell
What are Comments?
Comments are annotations in source code that are ignored during execution. They are essential for providing context, explaining complex logic, and improving the overall readability of scripts. In PowerShell, comments are a vital tool for both individual developers and teams to document their thought processes and coding decisions.
Types of Comments in PowerShell
In PowerShell, there are primarily two types of comments:
-
Single-line comments begin with a `#` symbol. Anything following this symbol on the same line will not be executed.
-
Multiple-line comments are encapsulated within `<#` and `#>`. This syntax allows developers to write notes spanning multiple lines, making it perfect for providing detailed explanations or documenting larger blocks of code.
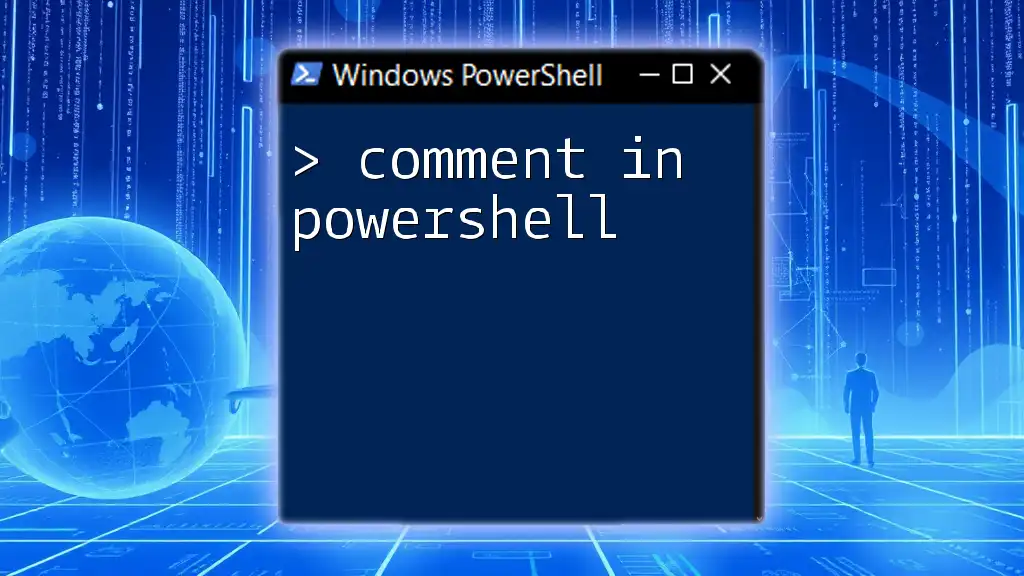
Syntax for Multiple Line Comments in PowerShell
Using `<# #>` for Multiple Line Comments
The syntax for creating multiple line comments is straightforward. Here’s how you can use it:
<#
This is a multiple line comment.
It can span across several lines.
#>
This format allows you to create comments that explain sections of code in detail, making your scripts more understandable for others (or yourself in the future).
Best Practices for Writing Multiple Line Comments
When adding multiple line comments, adhere to a few best practices to enhance readability and maintainability:
-
Keep comments focused: Ensure that each comment block pertains specifically to the associated code it explains. This helps ease navigation through complex scripts.
-
Use clear language: Stick to simple and direct language. Avoid jargon that may confuse readers who are not familiar with specifics.
-
Avoid verbosity: While it is essential to be descriptive, lengthy comments can detract from the overall flow of your code. Aim for conciseness without sacrificing clarity.
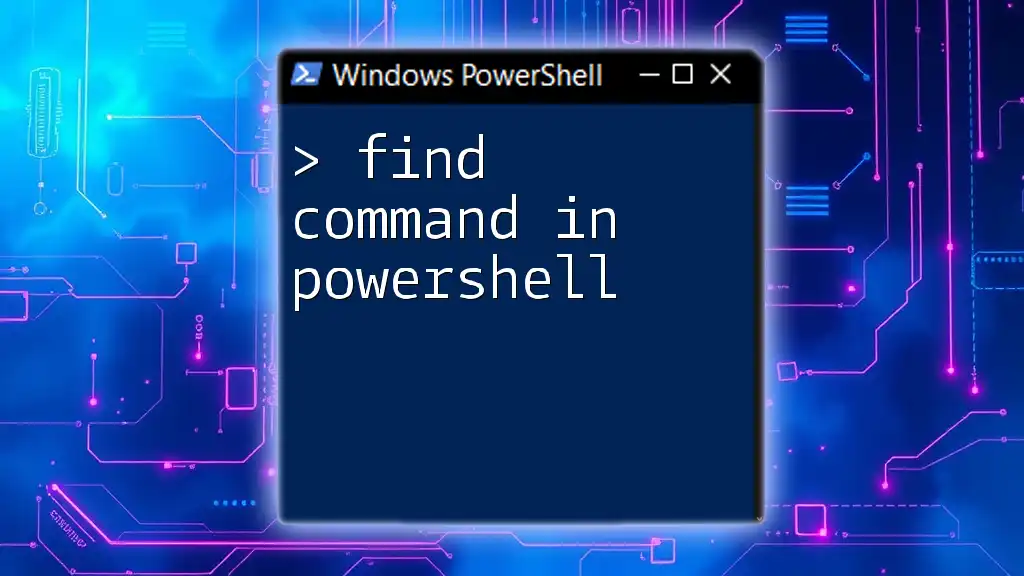
When to Use Multiple Line Comments
Scenarios for Implementation
Multiple line comments are particularly useful in several scenarios:
-
Documenting complex logic: If a code segment involves intricate calculations or conditional statements, providing a thorough explanation can help others (and future you) understand the reasoning behind it.
-
Providing detailed explanations: Large functions or scripts that perform multiple tasks benefit from comments that break down each section explicitly.
-
Outlining script usage: For scripts that require specific input or execute particular actions, documenting these details can save time and prevent misuse.
Examples of When Not to Use Multi-Line Comments
While multiple line comments are beneficial, they are not always necessary. Consider the following:
-
In straightforward code blocks that clearly convey their purpose, it may be more appropriate to use single-line comments or, in some cases, none at all.
-
Scenarios where inline comments suffice may result in a cleaner and more maintainable script if used judiciously.
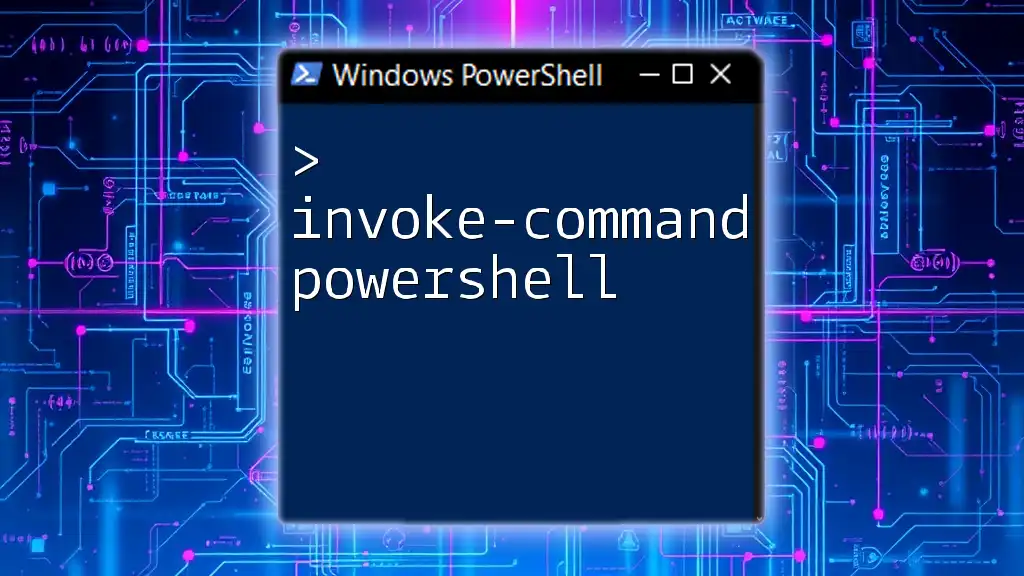
Common Misconceptions About Multiple Line Comments in PowerShell
Misconception #1: They Slow Down Script Execution
An often-held belief is that comments can adversely affect performance. However, it’s crucial to clarify that comments are ignored during execution. Therefore, using them liberally will not slow down your scripts.
Misconception #2: All Comments Should Be Detailed
There is a common misunderstanding that all comments must be exhaustive. In reality, finding a balance between brevity and thoroughness is key. For instance, sometimes a simple note is more effective than an overly detailed explanation. Here’s an example of an unnecessarily verbose comment:
<#
This block of code is here to demonstrate the function for adding
two integers. The function will take two parameters and return
the sum of those parameters. This is a basic operation.
#>
Instead, a more concise comment could serve just as well:
<#
Adds two integers.
#>
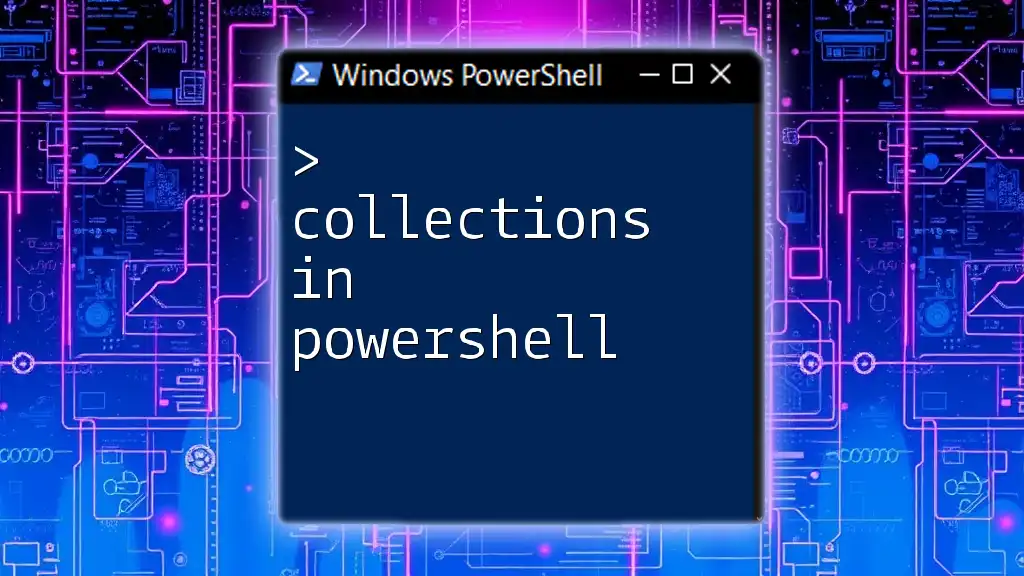
Advanced Techniques with Multiple Line Comments
Nesting Multiple Line Comments
It’s worth noting that nesting multiple line comments can lead to confusion and complications. PowerShell does not support nested multi-line comments, which could result in syntax errors. For example:
<#
This is an outer comment.
<#
This is a nested comment.
#>
#>
The above code creates ambiguity as it's uncertain where the outer comment ends.
Using Multiple Line Comments for Debugging Purposes
Multiple line comments can be incredibly helpful for temporarily disabling code during testing or debugging. You can quickly comment out blocks of code using this method. Here’s an example:
<#
# This block of code is temporarily disabled for testing.
Write-Host "This line will not execute."
#>
By wrapping code in multiple line comments, you can easily isolate sections of your script without deleting any code, which is particularly useful when you want to test various features selectively.
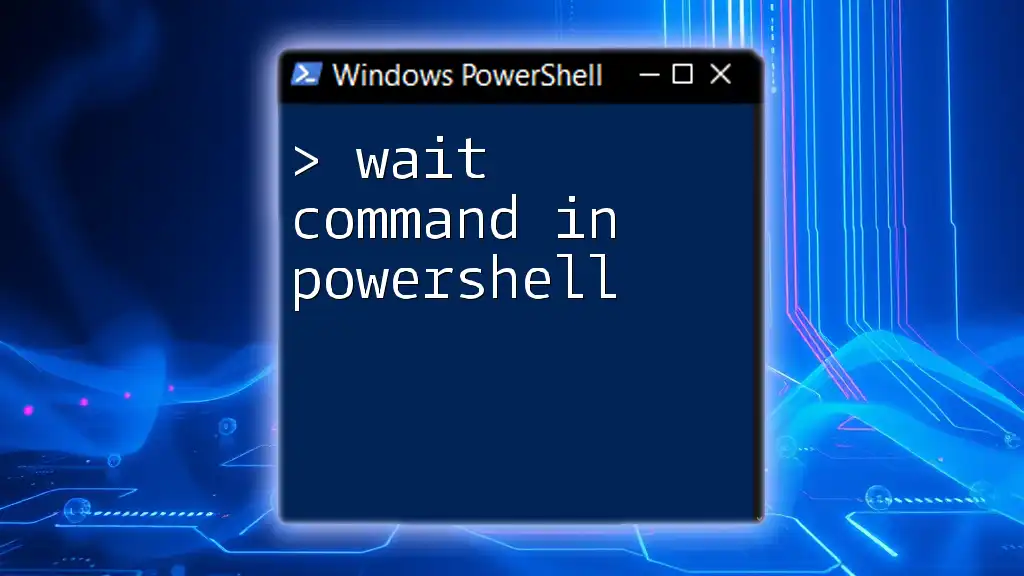
Tips and Tools for Effective Commenting
Commenting Styles to Consider
Consistency in commenting style is essential, especially when working in a team. Aligning on a style guide fosters communication among team members and reduces confusion. Consider adopting a commenting format that works well within your development environment and ensure everyone adheres to it.
Utilizing Editors with Commenting Features
Many Integrated Development Environments (IDEs) and text editors, like Visual Studio Code, offer features that enhance your commenting experience. These editors allow you to fold comments (hide them for a cleaner view) and provide shortcuts for quickly commenting and uncommenting blocks of code, making the development process more efficient.
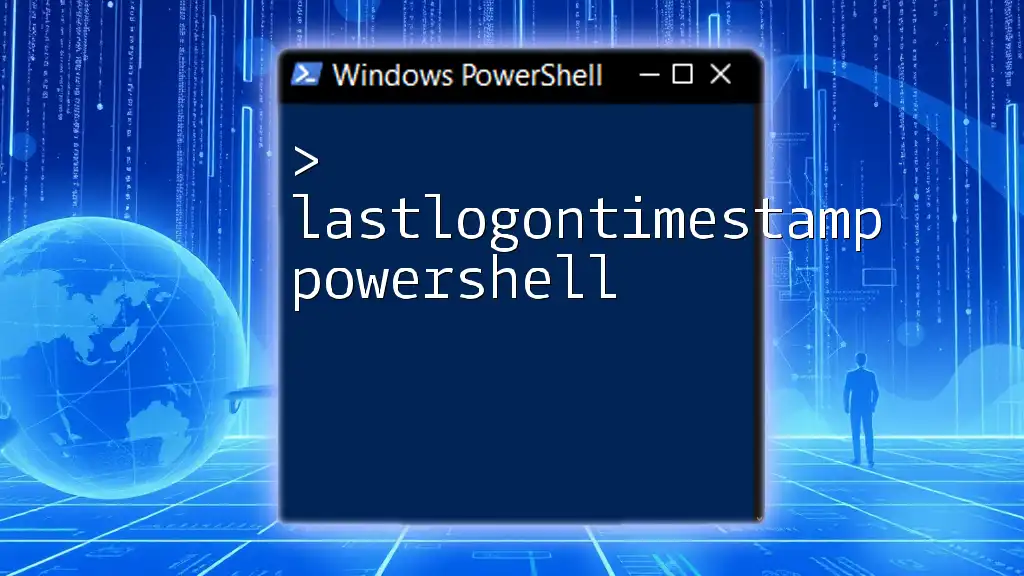
Conclusion
In summary, multiple line comments in PowerShell are a powerful tool for enhancing script readability and maintainability. By following best practices regarding syntax, usage scenarios, and misconceptions, developers can utilize comments to their full potential. Always remember that the goal of commenting is to aid understanding, so focus on clarity and conciseness when crafting your comments. As you continue to script in PowerShell, practice using multiple line comments effectively to create well-documented and easier-to-understand code.