Jenkins, an open-source automation server, can leverage PowerShell scripts to streamline build and deployment processes in Windows environments.
# Example of triggering a PowerShell script in Jenkins
$PipelineScript = @"
node {
stage('Run PowerShell Script') {
powershell 'Write-Host \"Hello, World!\"'
}
}
"@
write-host $PipelineScript
What is Jenkins?
Jenkins is an open-source automation server designed to facilitate Continuous Integration (CI) and Continuous Deployment (CD). It allows developers to automatically build, test, and deploy their applications, streamlining software development processes and exponentially increasing efficiency. Some key features of Jenkins include:
- Extensibility: Jenkins supports hundreds of plugins that can accommodate a variety of tools and services, allowing seamless integration into your development workflow.
- Distributed Builds: Jenkins can distribute the workload of building and testing projects across multiple machines, ensuring faster execution and efficient resource use.
- Pipeline as Code: Through Jenkins' pipeline capabilities, complex workflows can be defined as code, providing transparency and easy version control.
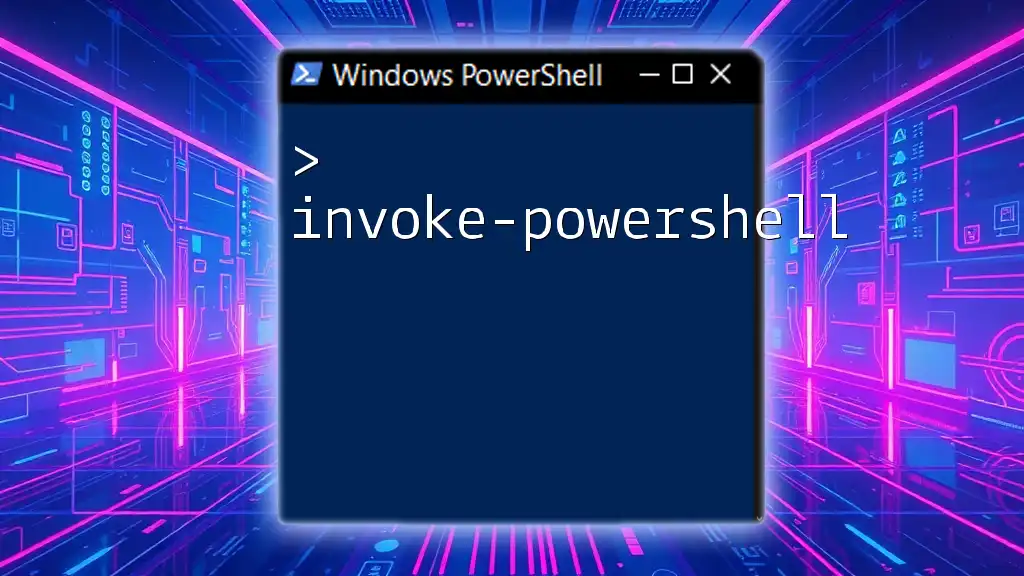
What is PowerShell?
PowerShell is a task automation framework that consists of a command-line shell and a scripting language built on the .NET Framework. Designed primarily for system administration, PowerShell is highly versatile, allowing users to automate repetitive tasks and manage system configurations effectively. Its importance lies in its ability to:
- Automate Administrative Tasks: PowerShell can execute scripts to perform system operations, facilitating everything from file management to network configurations.
- Interact Seamlessly with System Components: With built-in cmdlets, users can interact directly with Windows systems, APIs, and even Azure services.
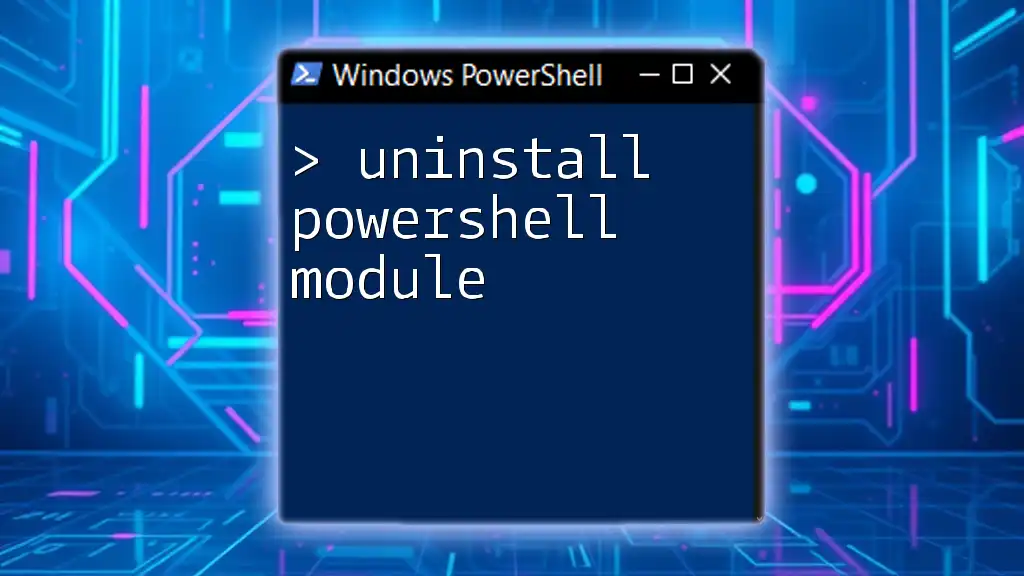
Why Use PowerShell with Jenkins?
Integrating PowerShell with Jenkins enhances the build and deployment processes significantly. By utilizing PowerShell scripts within Jenkins, you can:
- Streamline Operations: PowerShell commands can automate numerous steps in the build process, from setup configurations to deployment tasks.
- Increase Consistency and Reliability: Using scripts ensures that processes are executed the same way every time, reducing the likelihood of human error during deployments.
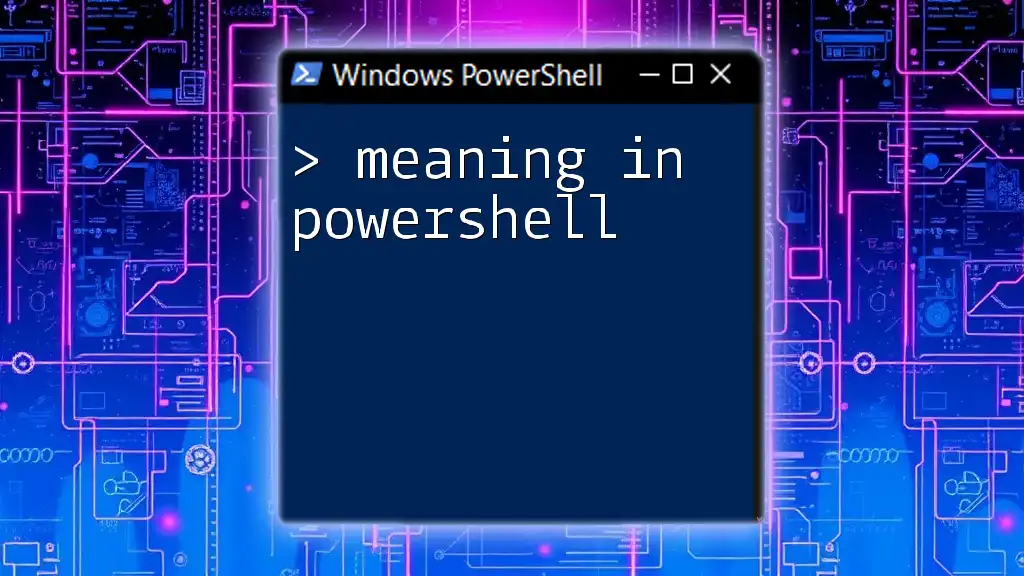
Setting Up Jenkins for PowerShell Integration
Installing Jenkins
To start utilizing Jenkins and PowerShell, you'll first need to install Jenkins. Here’s a quick overview of the steps:
- Download the Installer: Visit the [official Jenkins website](https://www.jenkins.io/download/) to download the installer for Windows.
- Run the Installer: Follow the prompts to complete the installation. Jenkins will usually run on default port 8080.
- Access Jenkins: Open a web browser and navigate to `http://localhost:8080` to access the Jenkins dashboard.
Installing PowerShell Plugin in Jenkins
Once Jenkins is installed, you can enhance its functionality by installing the PowerShell plugin.
- Access a Plugin Manager: From the Jenkins dashboard, click on “Manage Jenkins” and then “Manage Plugins.”
- Search for PowerShell: Under the Available tab, search for “PowerShell” and check the box.
- Install the Plugin: Click the “Install without restart” button to add the PowerShell plugin, enabling the use of PowerShell scripts in your Jenkins jobs.
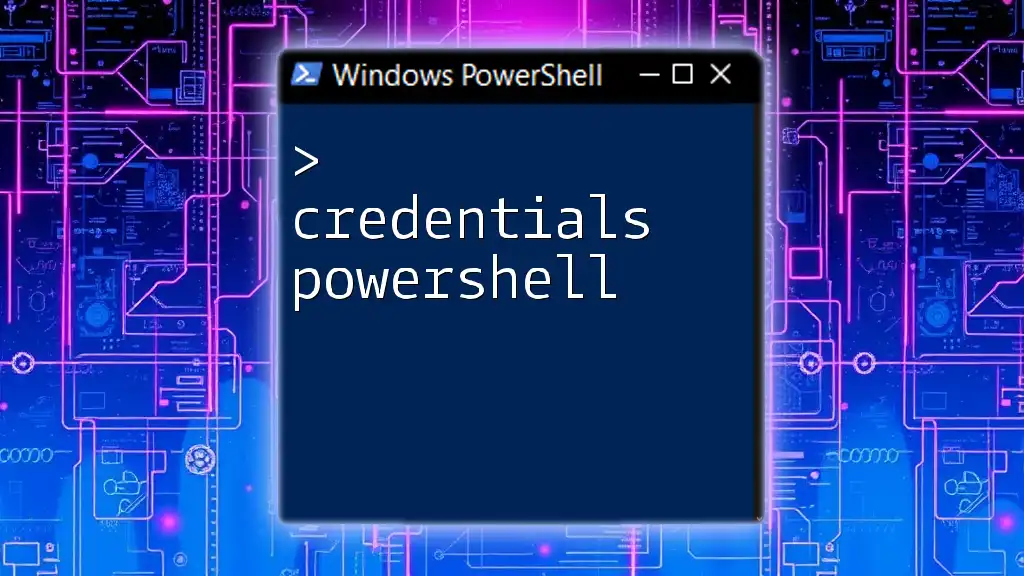
Creating Your First PowerShell Job in Jenkins
Job Configuration Basics
To create your first Jenkins job that leverages PowerShell, follow these steps:
- From the Jenkins dashboard, click on “New Item” and enter a name for your job.
- Select the “Freestyle project” option and click “OK.”
Adding PowerShell Build Steps
Now that your basic job is configured, the next step is to add a PowerShell build step.
- Scroll to Build Section: In the job configuration page, scroll down to the “Build” section.
- Add a Build Step: Click on “Add build step” and select “Windows PowerShell.”
- Enter Your Script: In the script input area, provide a simple PowerShell command, such as:
Write-Host "Hello, Jenkins from PowerShell!"
Saving and Running Your Job
After configuring your build steps, save your job settings by clicking on “Save.” To execute your job, click on “Build Now” and monitor the output in the “Build History” section. You can check the console output to verify that your PowerShell script ran successfully.
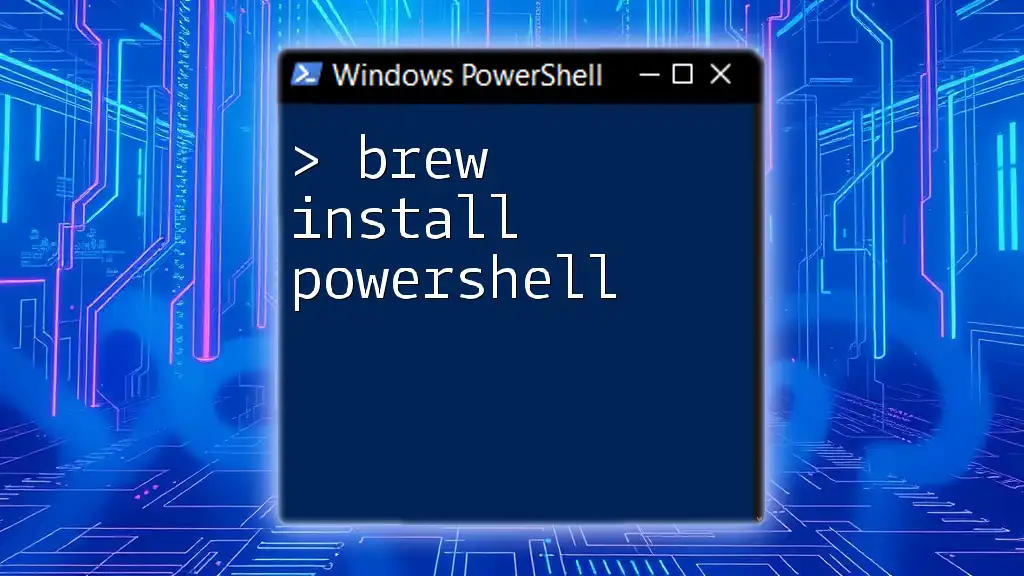
Leveraging PowerShell Scripts in Jenkins Pipelines
Understanding Jenkins Pipelines
Jenkins pipelines allow you to define your build process as code, taking automation to the next level. The two primary forms of Jenkins pipelines are Declarative and Scripted pipelines. Both forms benefit from using PowerShell scripts seamlessly.
Creating a Declarative Pipeline with PowerShell
Here’s how you can create a Jenkinsfile that uses PowerShell in a declarative pipeline:
pipeline {
agent any
stages {
stage('Run PowerShell Script') {
steps {
powershell {
script 'Write-Host "Executing PowerShell within a Jenkins pipeline!"'
}
}
}
}
}
In this example, a stage is defined that runs a PowerShell script. The flexibility of pipelines allows you to easily structure your build processes while maintaining the clarity of scripting.
Best Practices for PowerShell in Jenkins Pipelines
To make the most of using PowerShell with Jenkins, consider the following best practices:
- Organize Scripts Efficiently: Maintain a repository of reusable PowerShell scripts for common tasks. This promotes standardization and efficiency across your projects.
- Integrate Version Control: Store your Jenkinsfile and PowerShell scripts in version control systems like Git. This ensures you can track changes over time and roll back if necessary.
- Implement Robust Error Handling: Use meaningful try-catch blocks in your PowerShell scripts to manage errors effectively and ensure your pipeline can respond appropriately to failures.
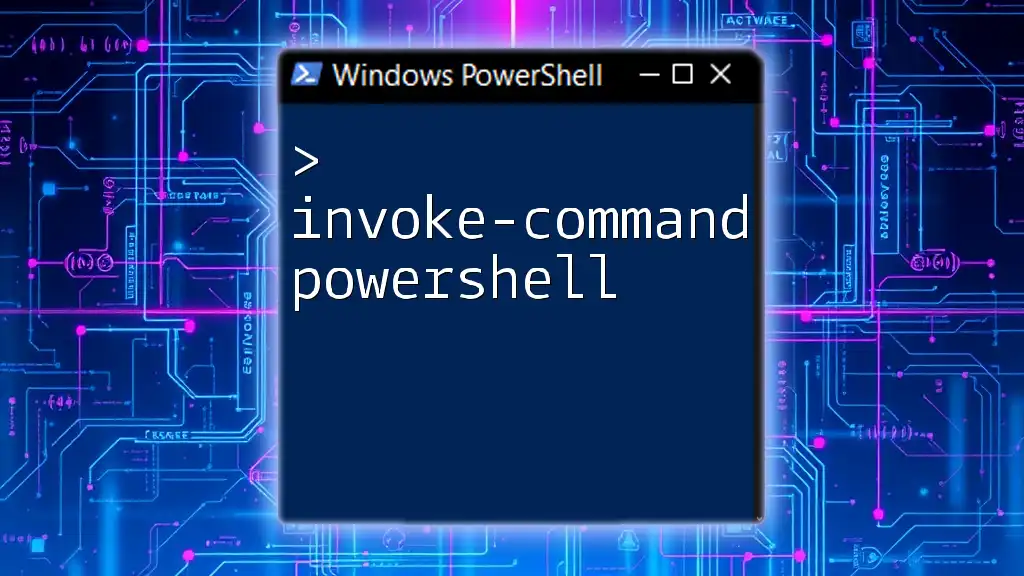
Troubleshooting Common Issues
Common Errors in PowerShell Scripts
When working with PowerShell scripts, you may encounter syntax errors or runtime issues:
- Syntax Errors: These often arise from typos or incorrect command usage. Debugging tools built into PowerShell or the `-Verbose` flag can provide detailed output to help identify these issues.
- Debugging Techniques: Utilize the `Write-Host` and `Write-Output` cmdlets to output intermediate results while testing scripts, which aids in locating issues.
Jenkins-Specific Challenges
When integrating Jenkins and PowerShell, you may face challenges related to permissions:
- Permissions Issues: If your scripts require administrative rights, configure Jenkins to run with sufficient privileges. You can adjust the service settings or use specific execution policies in PowerShell.
- Script Execution Policies: PowerShell scripts are affected by execution policies. Consider setting the policy to allow script execution by using:
Set-ExecutionPolicy RemoteSigned
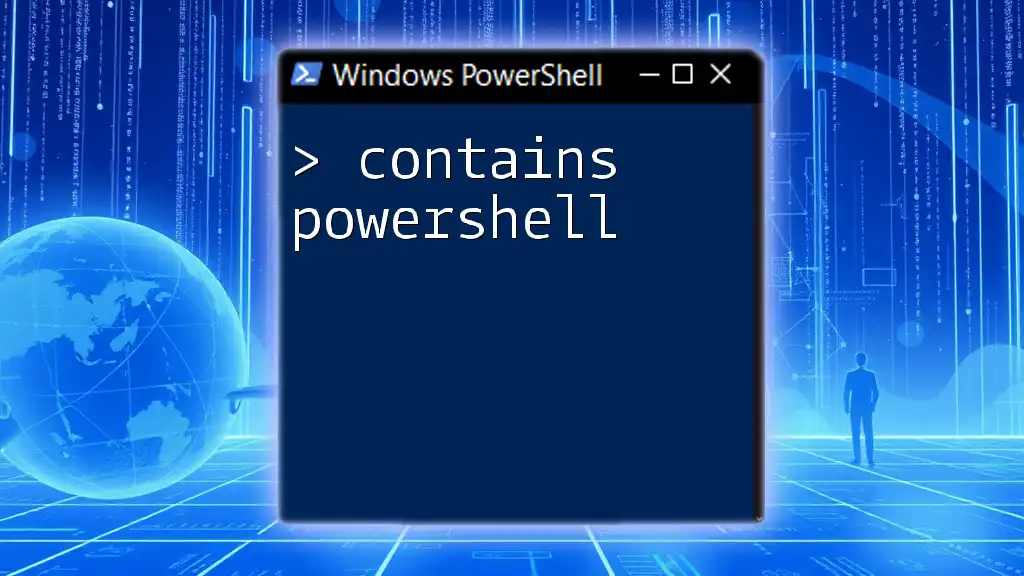
Integrating External Tools with PowerShell and Jenkins
Working with APIs
PowerShell's versatility shines when making REST API calls. Combining it with Jenkins allows you to extend your CI/CD workflows:
- Making API Calls: You can call an external API directly from a PowerShell script. Consider the following example, which retrieves data from a REST API:
$response = Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
Write-Host $response
This script retrieves JSON data from the specified API and displays the result in Jenkins.
Connecting to Databases
PowerShell scripts can also connect to databases, allowing you to automate data retrieval and manipulation. Here’s an example of how to query a SQL database:
$connectionString = "Server=yourServer;Database=yourDB;Integrated Security=true"
$query = "SELECT * FROM yourTable"
$connection = New-Object System.Data.SqlClient.SqlConnection($connectionString)
$command = New-Object System.Data.SqlClient.SqlCommand($query, $connection)
This snippet demonstrates how to establish a connection and create a command to execute SQL queries directly from your Jenkins pipeline.
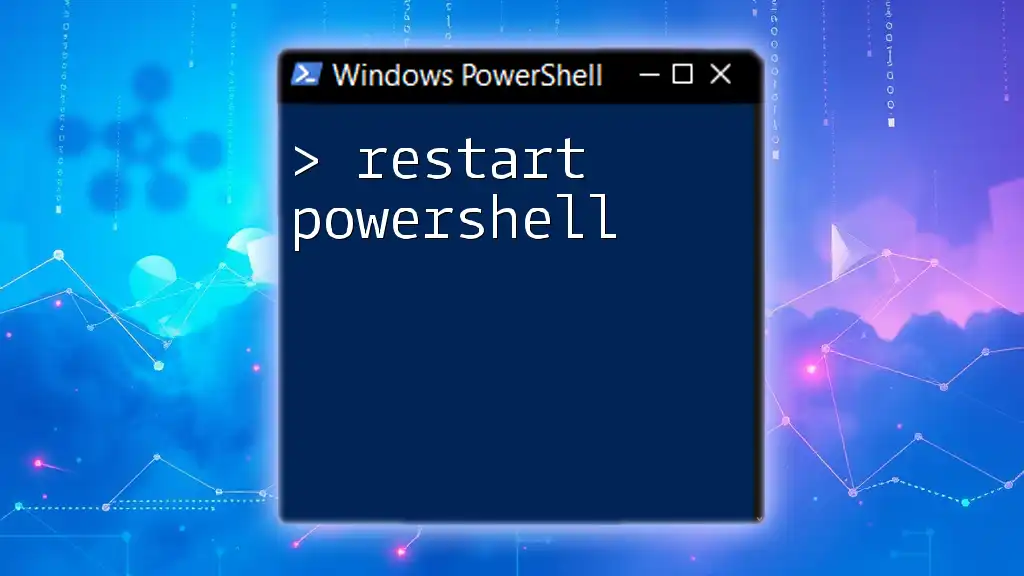
Conclusion
Using Jenkins and PowerShell together not only automates complex tasks but also enhances the reliability and efficiency of your CI/CD processes. The ability to write concise PowerShell commands and embed them into Jenkins jobs and pipelines provides an immense boost to developers and system administrators alike. The integration of these two powerful tools opens doors to automated workflows that save time and improve the overall productivity of your software development life cycle.
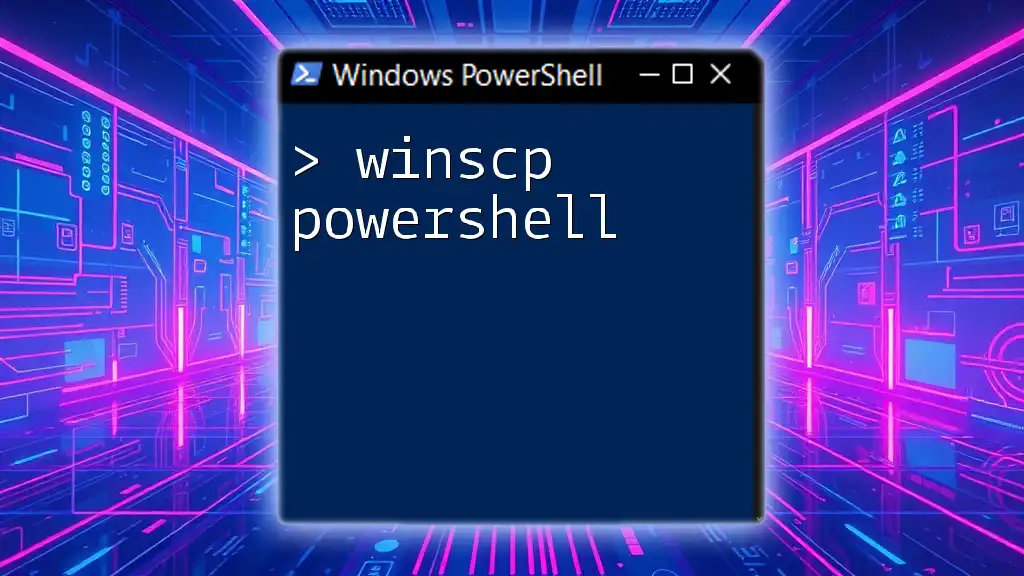
Additional Resources
For readers seeking to deepen their understanding of Jenkins and PowerShell, consider exploring:
- The [Jenkins documentation](https://www.jenkins.io/doc/)
- PowerShell scripting resources available on the [Microsoft Docs](https://docs.microsoft.com/en-us/powershell/)
- Engaging in community forums like Stack Overflow for support and shared knowledge on scripting and automation challenges.