To access Azure PowerShell, you first need to install the Azure PowerShell module and then log in using your Azure account credentials; here's a simple command to get you started:
Install-Module -Name Az -AllowClobber -Scope CurrentUser
Connect-AzAccount
What is Azure PowerShell?
Azure PowerShell is a set of modules that lets you manage Azure resources directly from PowerShell. Unlike traditional PowerShell, which commands local and remote systems, Azure PowerShell is specifically designed for managing Azure services. This differentiation allows you to automate and streamline tasks across your Azure environment effectively.
Benefits of using Azure PowerShell include:
- Efficiency: Automate repetitive tasks, reducing manual errors.
- Simplicity: Simplified command structure allows quick execution of complex actions.
- Integration: Seamlessly works with other Azure tools and services, making resource management straightforward.
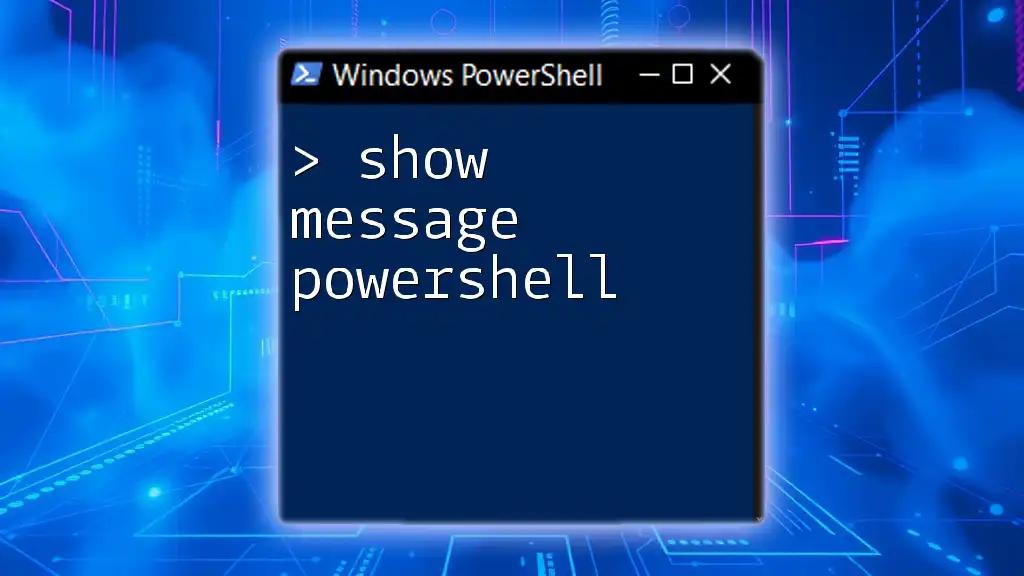
Prerequisites for Accessing Azure PowerShell
System Requirements
To access Azure PowerShell, ensure your system meets the following requirements:
- Operating System: Windows, macOS, or Linux.
- PowerShell Version: At least PowerShell 5.1 is required to run Azure PowerShell commands. You can check your version with:
$PSVersionTable.PSVersion
Azure Account Setup
You will need an Azure account to interact with Azure services. If you don't have one, follow these steps to create an account:
- Visit the [Azure sign-up page](https://azure.microsoft.com/en-us/free/).
- Click on 'Start free' and follow the instructions.
- Provide your email and billing information (though you won't be charged during the trial).
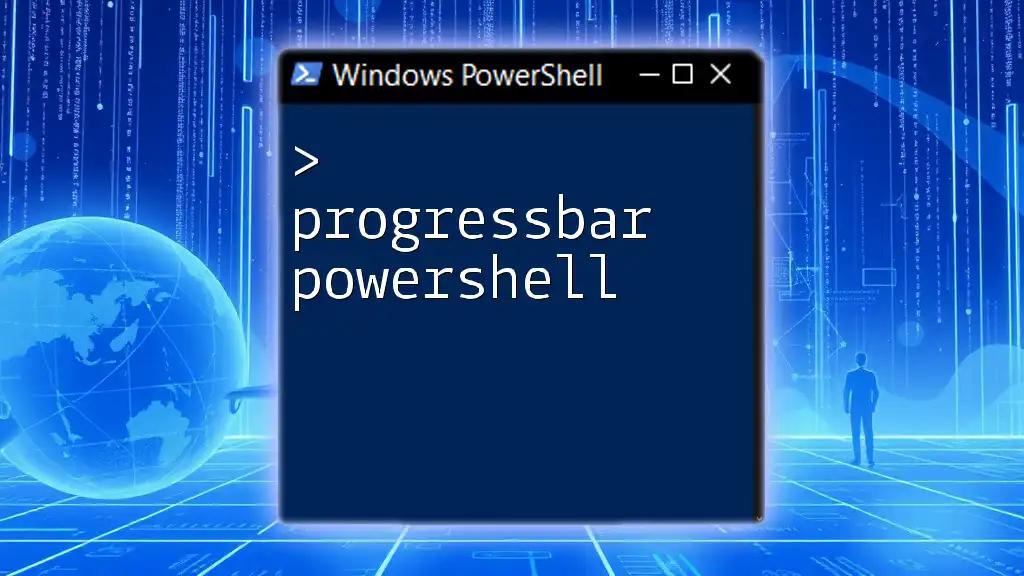
Installing Azure PowerShell
Installation Methods
To install Azure PowerShell, you can use two primary methods:
1. Installing via PowerShell Gallery
Using the PowerShell Gallery is the recommended way of installing Azure PowerShell. Open your PowerShell console and run the following command:
Install-Module -Name Az -AllowClobber -Scope CurrentUser
This command downloads and installs the necessary modules from the PowerShell Gallery.
2. Alternative Installation via MSI Package
For users who prefer a graphical installation, Microsoft offers an MSI package. You can download it from the official [Azure PowerShell GitHub page](https://github.com/Azure/azure-powershell). Simply follow the installation wizard.
Verifying the Installation
To confirm that Azure PowerShell has been installed successfully, execute:
Get-Module -ListAvailable -Name Az
If installed correctly, this command will list the Az module along with its version information.
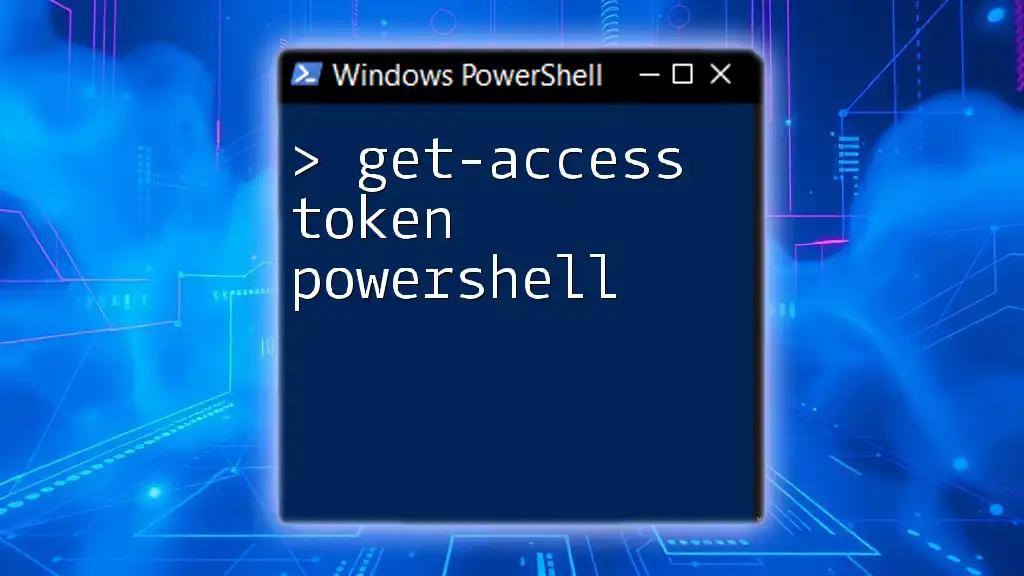
Accessing Azure PowerShell
Starting Azure PowerShell
Windows users can start Azure PowerShell from the Start menu. For macOS and Linux users, you can use your terminal. The commands and features remain consistent across platforms, allowing flexibility in environments.
Connecting to Your Azure Account
To manage Azure resources, you need to authenticate. Use the following command to log in:
Connect-AzAccount
This will open a dialog prompting you for your Azure credentials. If your account has multi-factor authentication enabled, you'll need to complete that verification as well.
Selecting Your Azure Subscription
Once logged in, Azure PowerShell will default to your primary subscription. To view all subscriptions linked to your account, execute:
Get-AzSubscription
To select a specific subscription to work within your session, use:
Select-AzSubscription -SubscriptionId "<YourSubscriptionId>"
Replace `<YourSubscriptionId>` with the ID of your desired subscription. This ensures that all subsequent commands apply to the correct Azure resources.
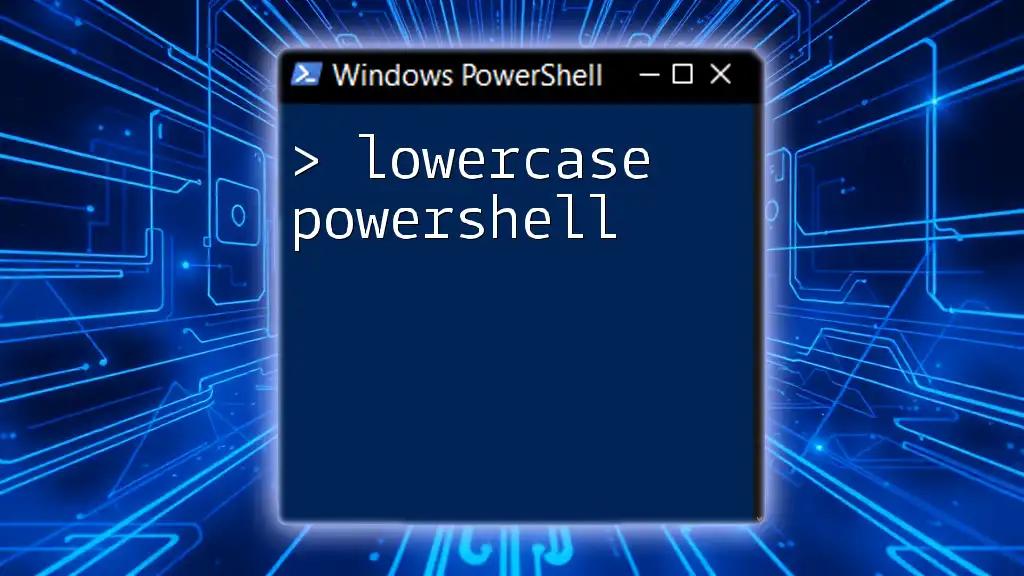
Basic Commands for Azure PowerShell
Understanding Cmdlets
In Azure PowerShell, commands are composed using cmdlets, which follow the verb-noun structure. The verb indicates the action, while the noun identifies the resource type. For example, `Get-AzResourceGroup` retrieves information about resource groups in your Azure account.
Commonly Used Cmdlets
Here are some fundamental cmdlets that you will frequently use:
To list all Azure resource groups:
Get-AzResourceGroup
This command helps you understand the organization of your resources.
To create a new resource group:
New-AzResourceGroup -Name "MyResourceGroup" -Location "EastUS"
This command establishes a new resource group, which is essential for organizing resources by region.
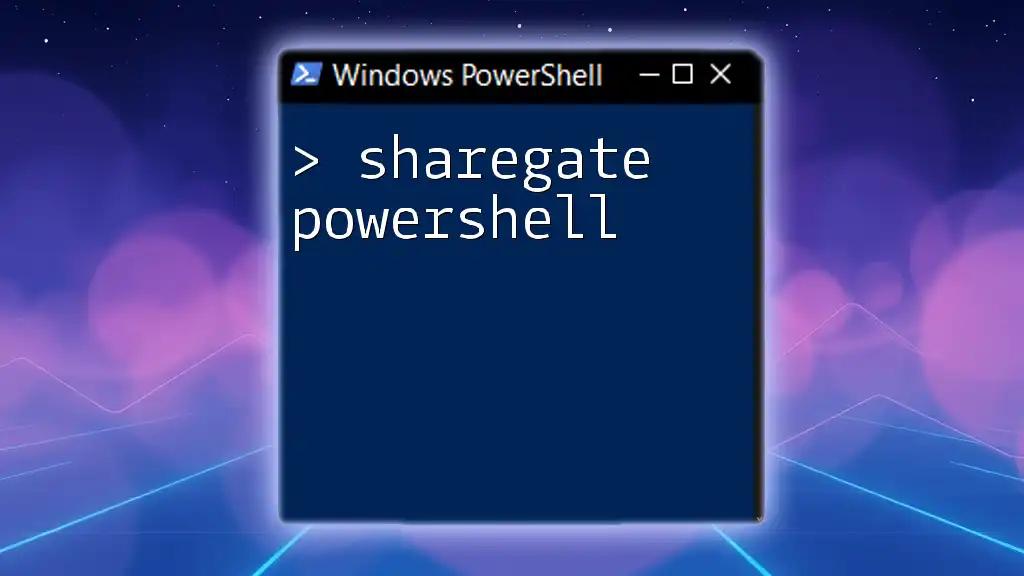
Troubleshooting Common Issues
Authentication Problems
Sometimes, issues may arise during the authentication process. Common errors include:
- Invalid Credentials: Double-check your Azure username and password.
- Account Lockout: Ensure your account isn’t locked out due to multiple failed login attempts.
If issues persist, consider revoking any existing sessions with:
Disconnect-AzAccount
Module Conflicts
It's possible to run into conflicts between modules if you have multiple versions installed. To resolve issues, ensure that you are using the latest module by checking for updates with:
Update-Module -Name Az
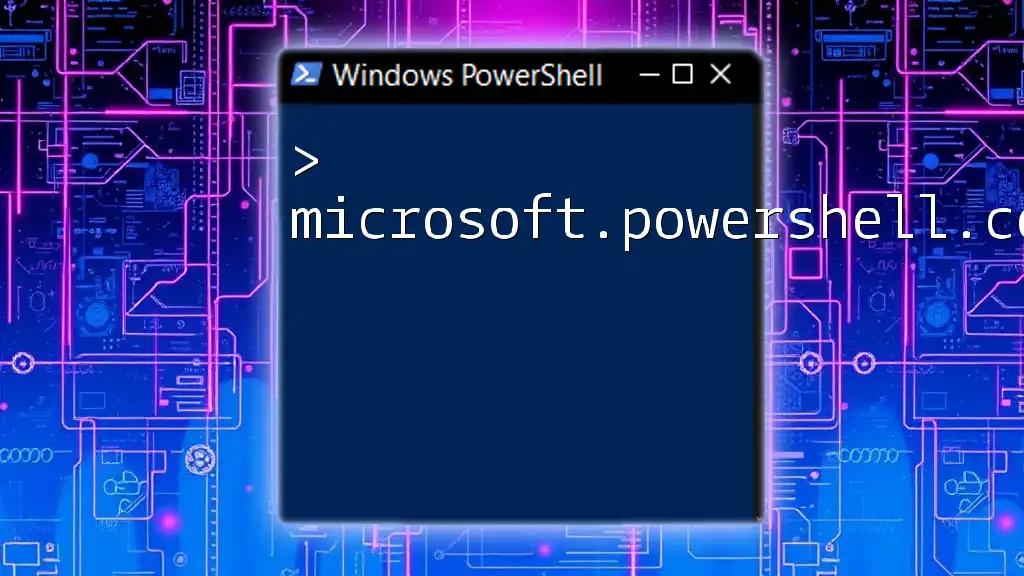
Best Practices for Using Azure PowerShell
Scripting and Automation
Using scripts can significantly enhance your productivity. When writing Azure PowerShell scripts, make sure to:
- Group related commands together.
- Use proper variable names for clarity.
- Add comments to explain complex logic for future reference.
Staying Updated
Regularly update your Azure PowerShell modules to benefit from new features and fixes. Update your modules frequently using:
Update-Module -Name Az
This command fetches the latest version from the PowerShell Gallery, ensuring you have the best functionality available.
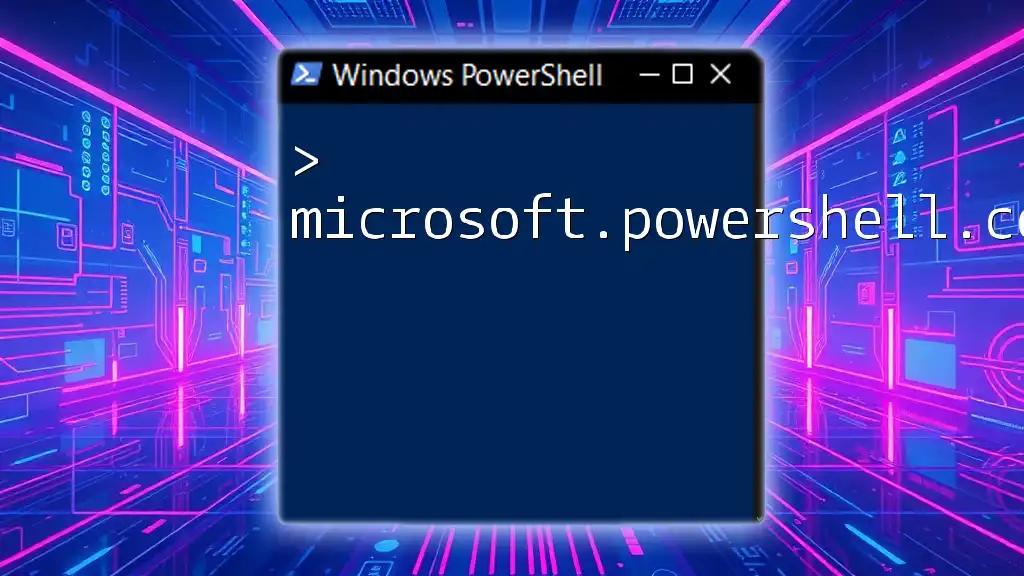
Conclusion
By following the steps outlined in this guide, you now understand how to access Azure PowerShell effectively. Mastering these concepts will empower you to manage your Azure resources with ease and efficiency.
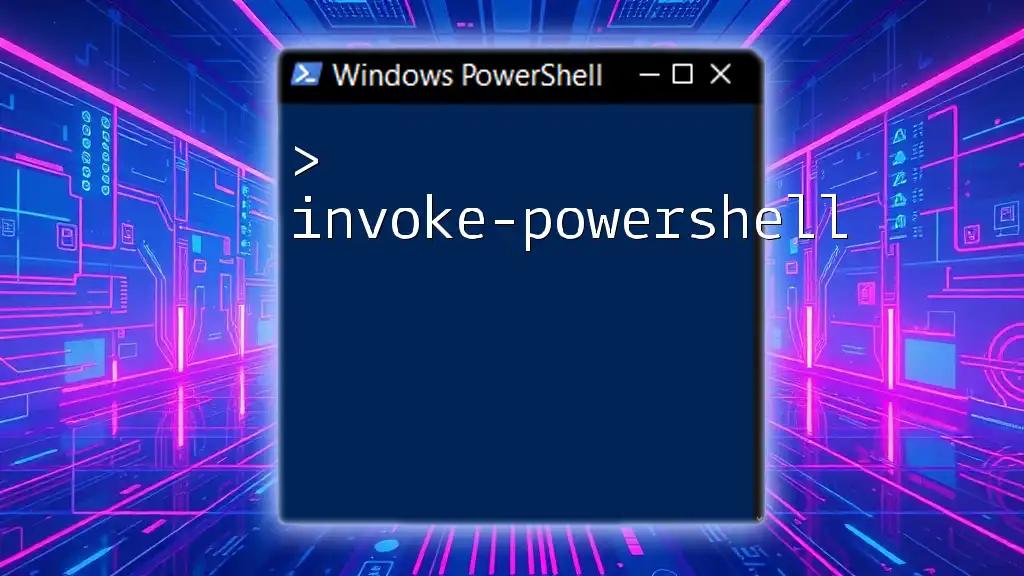
Additional Resources
For further learning, consider exploring the official Microsoft documentation. Communities and forums dedicated to Azure PowerShell can also provide additional support and insights. Dive deeply into the ecosystem, and you'll discover even more powerful ways to enhance your cloud management skills.