Accessing your Dropbox for Business directory from PowerShell can be achieved using the Dropbox API along with a simple PowerShell script to list files and folders. Here’s a quick example:
# Load required modules and authenticate to Dropbox API
$AccessToken = "your_access_token_here"
$Uri = "https://api.dropboxapi.com/2/files/list_folder"
$Body = @{
path = ""
} | ConvertTo-Json
# Make the API call to list files in your Dropbox Business directory
$response = Invoke-RestMethod -Uri $Uri -Method Post -Headers @{Authorization = "Bearer $AccessToken"; "Content-Type" = "application/json"} -Body $Body
$response.entries | ForEach-Object { Write-Host $_.name }
Replace `"your_access_token_here"` with your actual Dropbox API access token to execute the script successfully.
Understanding Dropbox for Business API
Overview of the API
The Dropbox for Business API allows developers to integrate and interact with Dropbox's business features seamlessly. Unlike the personal Dropbox API, it provides additional capabilities to manage team workspaces, manage users, and access team files programmatically. For PowerShell users, leveraging this API opens up a realm of possibilities for automating tasks and integrating Dropbox functionality into existing workflows.
Prerequisites for API Access
Before diving into accessing your Dropbox for Business directory from PowerShell, it’s crucial to set up the necessary prerequisites.
-
Creating a Dropbox for Business account: If you haven't already, sign up for a Dropbox for Business account. This is essential as personal accounts do not have access to business features via the API.
-
Generating an API key:
- Navigate to the Dropbox App Console.
- Create a new app and select the appropriate permissions based on your needs.
- Once created, generate an access token. This token will be your key to accessing the API and must be kept secure.
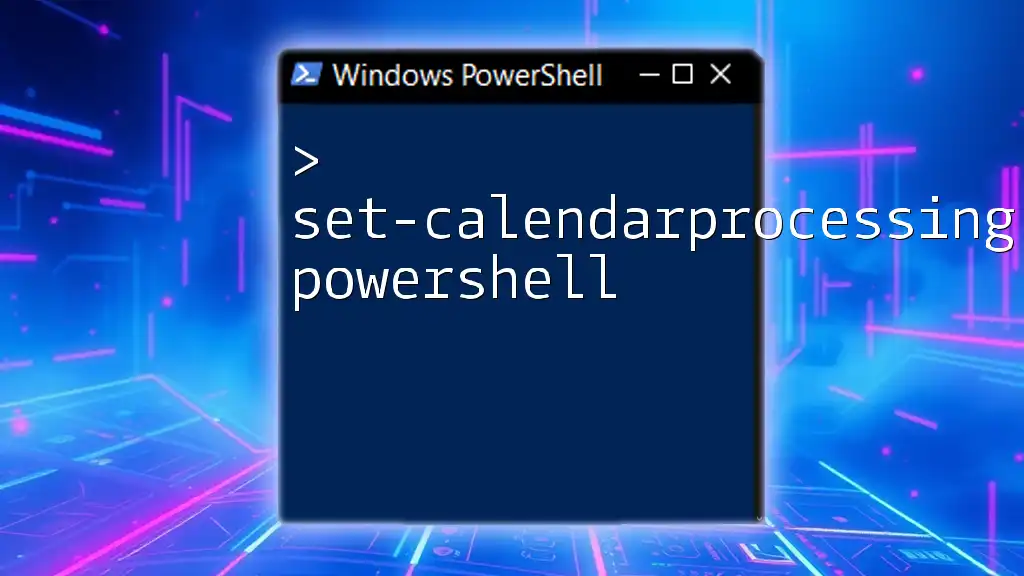
PowerShell Basics
Installation and Setup
The first step in using PowerShell to access Dropbox for Business dir is ensuring you have PowerShell installed. PowerShell comes pre-installed on Windows systems, while macOS and Linux might require installation of the latest version.
You will also need to familiarize yourself with necessary modules. The Invoke-RestMethod cmdlet is particularly important, as it will be used to make API calls from PowerShell.
Basic PowerShell Commands
Familiarize yourself with some foundational PowerShell commands that will come in handy. Understanding how to manipulate API requests will ease your PowerShell scripting.
-
`Get-Command`: Lists all the available commands.
-
`Get-Help`: Provides help documentation for specific commands to deepen your understanding.
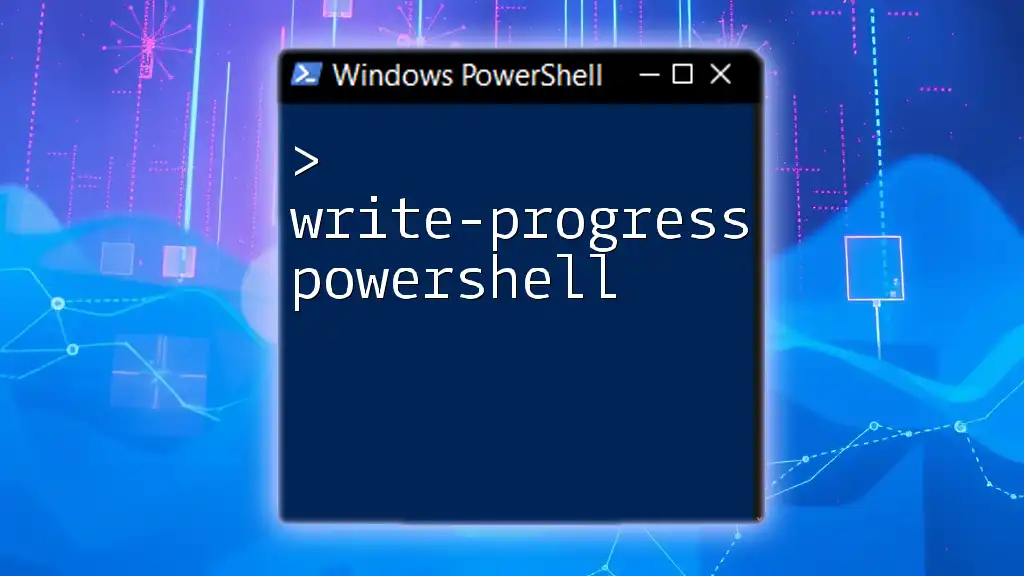
Authenticating to the Dropbox API
Using OAuth 2.0
Authentication is a critical step in ensuring secure access to your Dropbox data. Dropbox adopts OAuth 2.0 for secure access. Understanding this authentication method is paramount.
-
What is OAuth 2.0?: OAuth 2.0 is a protocol that grants a third-party application limited access to an HTTP service, without revealing user credentials.
-
Steps to authenticate using OAuth 2.0 with PowerShell:
- First, acquire your client ID and client secret from the Dropbox App Console.
- Construct an authorization URL, and navigate there to grant permission.
Example Code Snippet
For authentication, here’s a basic script to guide you through obtaining an access token:
# Sample code to authenticate and obtain an access token
$clientID = "YOUR_CLIENT_ID"
$clientSecret = "YOUR_CLIENT_SECRET"
$redirectURI = "YOUR_REDIRECT_URI"
# Construct the URL to request authorization
$uri = "https://www.dropbox.com/oauth2/authorize?client_id=$clientID&response_type=code&redirect_uri=$redirectURI"
# This URL will need to be visited by the user for manual authorization.
Start-Process $uri
Storing Access Tokens
Storing access tokens can be critical for maintaining seamless interactions with the API. Consider utilizing Windows Credential Manager or environment variables for secure storage. This prevents hardcoding sensitive information directly into your scripts.
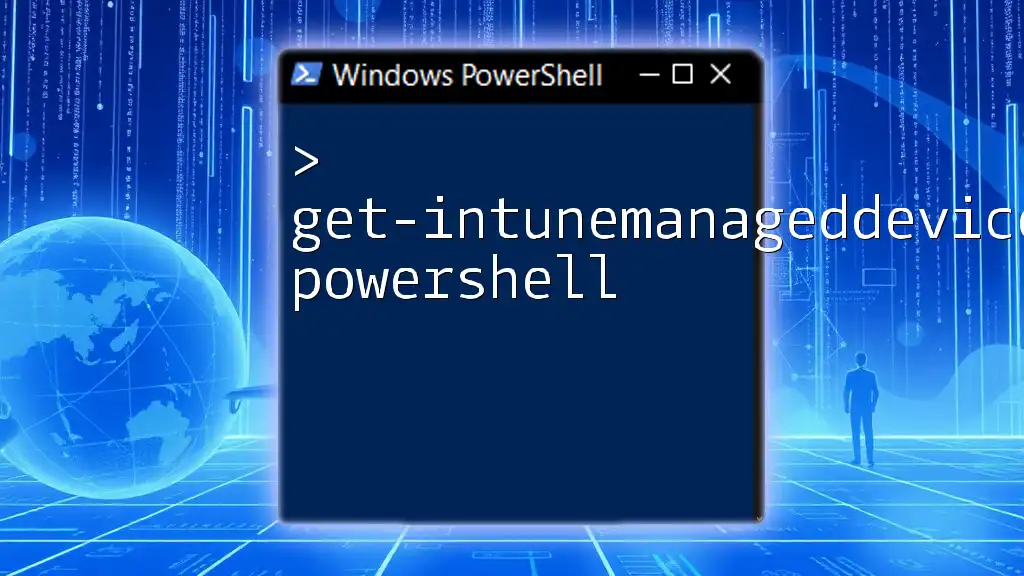
Accessing Dropbox for Business Directory
Making API Calls with PowerShell
API calls are the primary way to interact with your Dropbox data. Before making calls, familiarize yourself with the vital Dropbox API endpoints that will aid in accessing directories:
- List Folder: Retrieve files and folders from a specified path.
- Get Metadata: Obtain detailed information about a file or folder.
Example Code to List Directory Contents
Here’s a simple example of how to list files in a specific directory using PowerShell:
# Sample code to list files in a specific directory
$accessToken = "YOUR_ACCESS_TOKEN"
$uri = "https://api.dropboxapi.com/2/files/list_folder"
$response = Invoke-RestMethod -Uri $uri -Method Post -Headers @{Authorization = "Bearer $accessToken"} -Body '{"path": ""}'
$response.entries | ForEach-Object { $_.name }
In this code:
- The `Invoke-RestMethod` cmdlet is critical for making POST requests to the Dropbox API.
- The access token authenticates the request, allowing you to fetch directory contents dynamically.
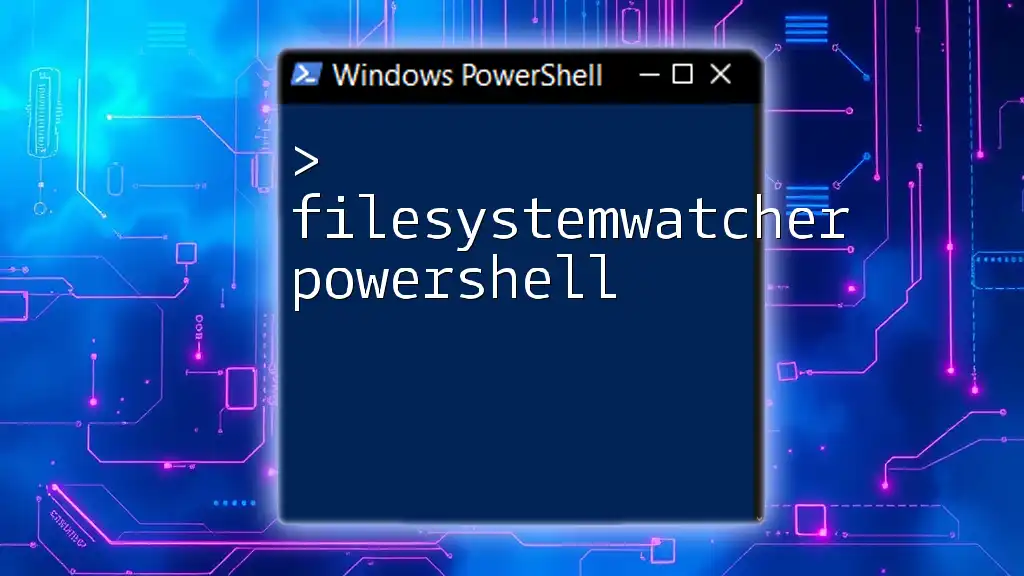
Handling Errors and Logging
Common Errors in API Calls
With any API integration, errors can happen. Errors may range from invalid access tokens to permissions issues. It’s important to anticipate these problems. Common error responses include:
- `401 Unauthorized`: Indicates issues with authentication.
- `404 Not Found`: Represents a failure in finding the requested resource.
Implementing Logging
Implementing logging within your PowerShell scripts can provide insights into API interactions and help troubleshoot issues. Here’s an example of how to log API responses:
# Example for logging API responses
Add-Content -Path "C:\Path\To\log.txt" -Value "API Response: $($response | ConvertTo-Json)"
Utilizing a log file allows you to maintain a record of requests, responses, and errors over time.
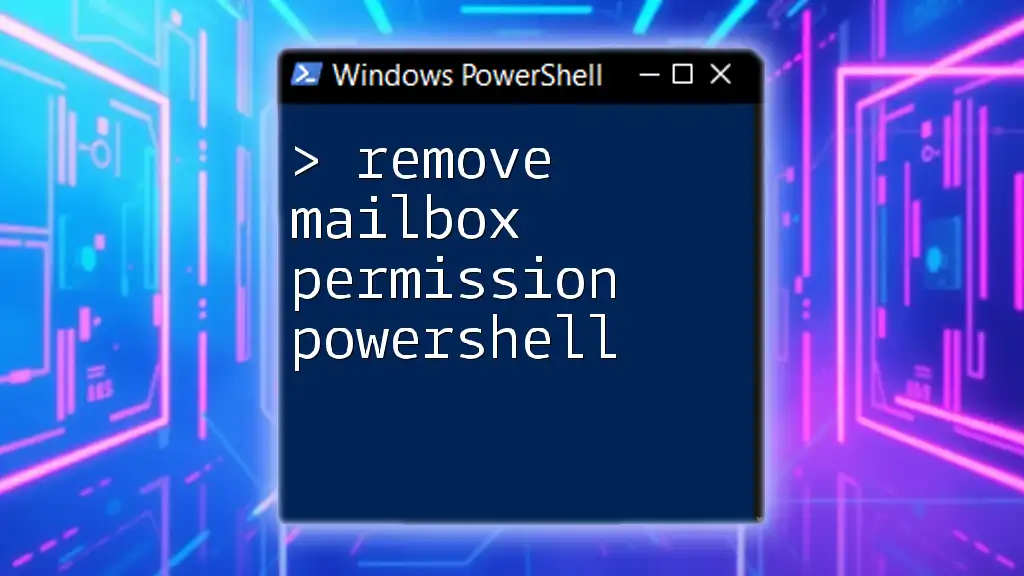
Conclusion
By following this guide, you now possess the knowledge needed to access Dropbox for Business dir from PowerShell effectively. Practicing these steps will not only enhance your PowerShell skills but also simplify your interactions with Dropbox data. Embrace the power of scripting to further streamline your workflows.
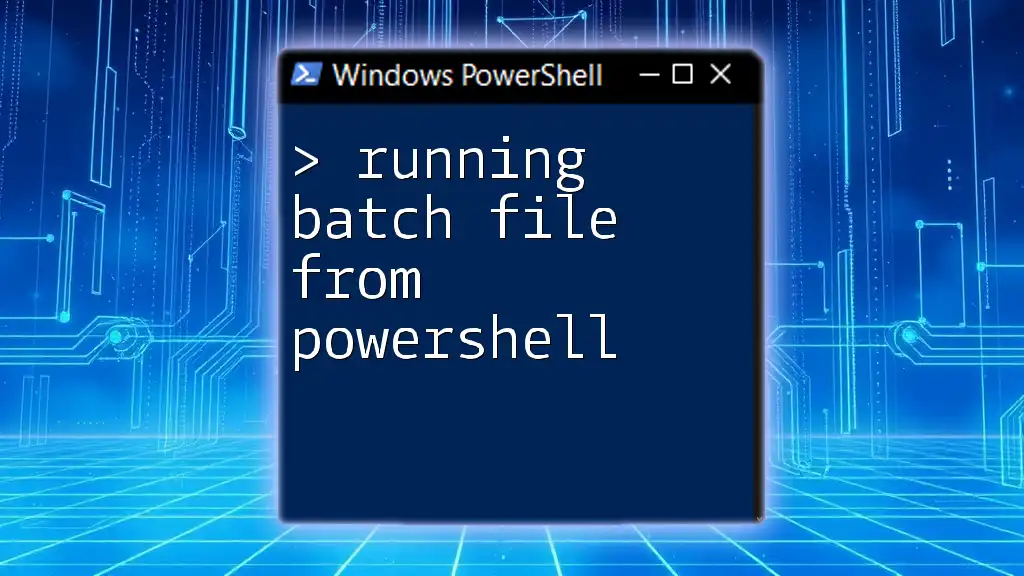
Additional Resources
- Consult the official Dropbox API documentation to explore more features.
- Consider further enhancing your PowerShell knowledge through recommended online learning resources.
- Engage with community forums for support, guidance, and tips from fellow PowerShell users.