To require a user to change their password at the next logon using PowerShell, you can use the following command:
Set-LocalUser -Name "USERNAME" -PasswordNeverExpires $false -ChangePasswordAtLogon $true
Replace `"USERNAME"` with the actual username of the account you want to modify.
Understanding Password Policies
Importance of Password Management
Password management is a crucial aspect of any organization’s security strategy. Requiring users to change their passwords regularly helps mitigate risks associated with breaches and unauthorized access. When a user is prompted to change their password, it serves as a safeguard against potential insider threats and ensures that outdated credentials do not leave sensitive systems vulnerable.
Windows Password Policies
Windows environments come with built-in password policies aimed at maximizing security. These policies determine how often users must change their passwords, the complexity required, and whether old passwords can be reused. Adjusting these settings according to organizational needs can greatly enhance security measures.
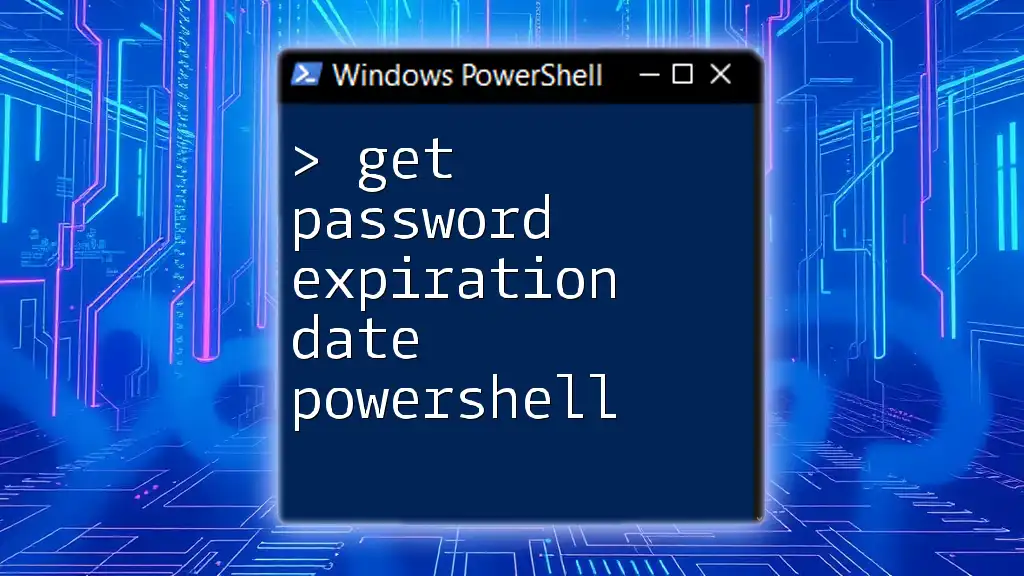
PowerShell Basics
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language designed for system administration. It enables IT professionals to automate tasks and manage configurations, making it an indispensable tool for Windows administrators.
Key Components of PowerShell
In PowerShell, tasks are executed using cmdlets—lightweight commands built into the shell. These cmdlets follow a verb-noun format, making them easy to understand. For example, `Get-Process` retrieves the list of currently running processes, and `Set-ADUser` modifies Active Directory user accounts.
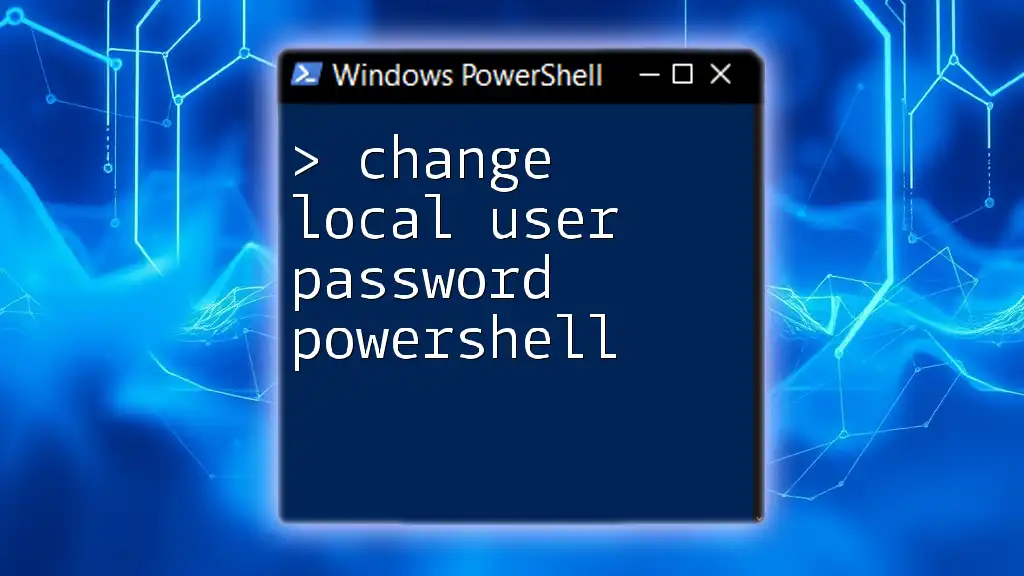
Setting a User to Change Password at Next Logon
Prerequisites and Permissions
Before you can require a user to change their password at the next logon, ensure you have administrative access. Without proper permissions, you’ll be unable to execute password management tasks effectively in a Windows environment.
Cmdlet for Changing Password Requirement
Using `Set-ADUser` Cmdlet
The primary cmdlet used for managing passwords in Active Directory is `Set-ADUser`. To require a user to change their password at the next logon, the command can be structured as follows:
Set-ADUser -Identity "username" -ChangePasswordAtLogon $true
In this command, `-Identity` specifies the username of the account being modified, while `-ChangePasswordAtLogon $true` activates the password change requirement.
Example Command
Here’s a practical example of how to apply this command:
Set-ADUser -Identity "john.doe" -ChangePasswordAtLogon $true
In this case, the user “john.doe” will be forced to change his password upon his next login, enhancing security protocols within your organization.
Using `Set-LocalUser` Cmdlet (for Local Users)
For non-domain environments, PowerShell also provides the `Set-LocalUser` cmdlet. This is useful for managing local user accounts, especially on stand-alone systems:
Set-LocalUser -Name "localusername" -ChangePasswordAtLogon $true
This command serves the same purpose; it prompts the local user to update their credentials at the next logon, ensuring compliance with your password security policies.
Code Snippet: Local User Example
Set-LocalUser -Name "alice" -ChangePasswordAtLogon $true
In this example, the user “alice” will be required to change her password at next sign-in.
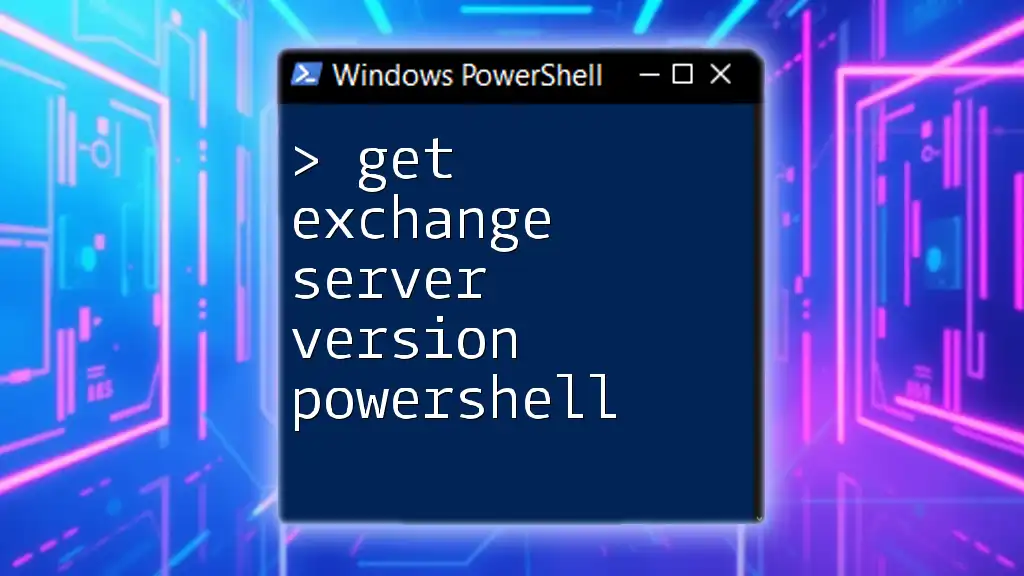
Verifying Password Change Requirement
Check User Settings
After modifying a user’s account settings, it's essential to verify that the requirement was indeed set. You can use the `Get-ADUser` cmdlet to confirm the user settings:
Get-ADUser -Identity "username" -Properties PasswordLastSet, PasswordNeverExpires
This command retrieves key properties associated with the user account, providing information about the last time the password was set and whether the password is configured to never expire.
Example Verification Command
To fully verify if the password change requirement is set, you can run:
Get-ADUser -Identity "john.doe" -Properties ChangePasswordAtLogon
This command will return details confirming whether “john.doe” needs to change his password during the next logon.
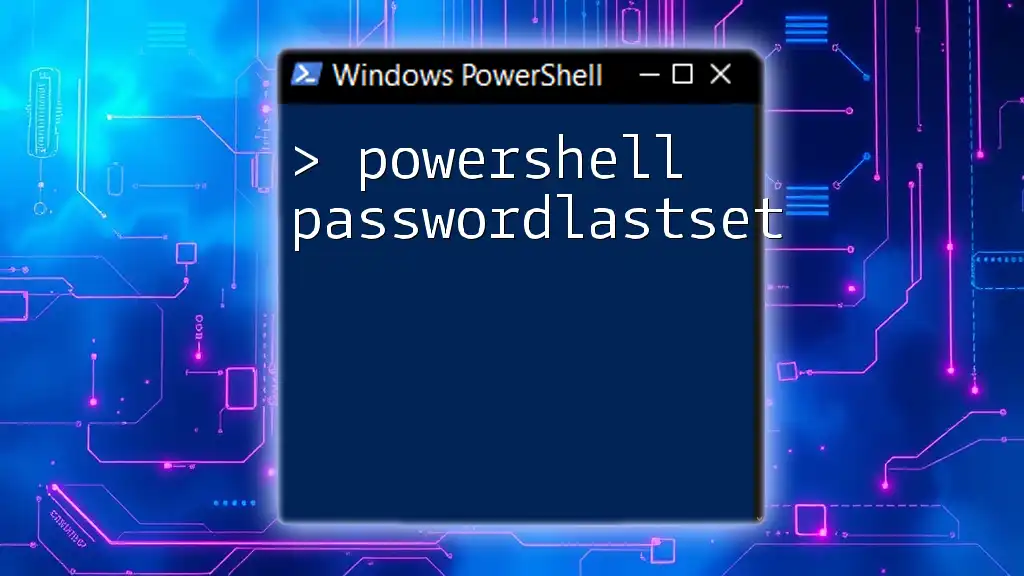
Handling Common Issues
Troubleshooting Tips
While executing PowerShell commands, users may encounter errors. Common issues include insufficient permissions or incorrect cmdlet syntax. Always ensure that you’re running PowerShell with administrative privileges and double-check your commands for typos.
Best Practices for Administrative Actions
Document any changes made to user account settings for auditing purposes. Keeping a log not only aids in troubleshooting but also aligns with change management policies within an organization.
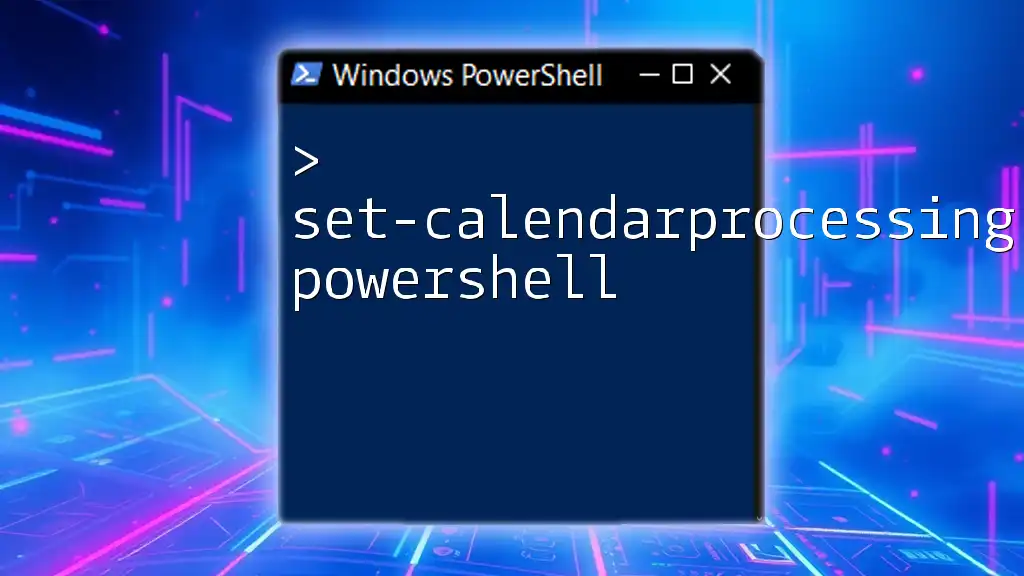
Conclusion
This guide has covered the essential steps to set a user to change their password at the next logon using PowerShell effectively. By implementing these practices, you can enhance your organization’s security posture. Regularly requiring password changes is a critical aspect of maintaining secure user accounts and protecting sensitive information.
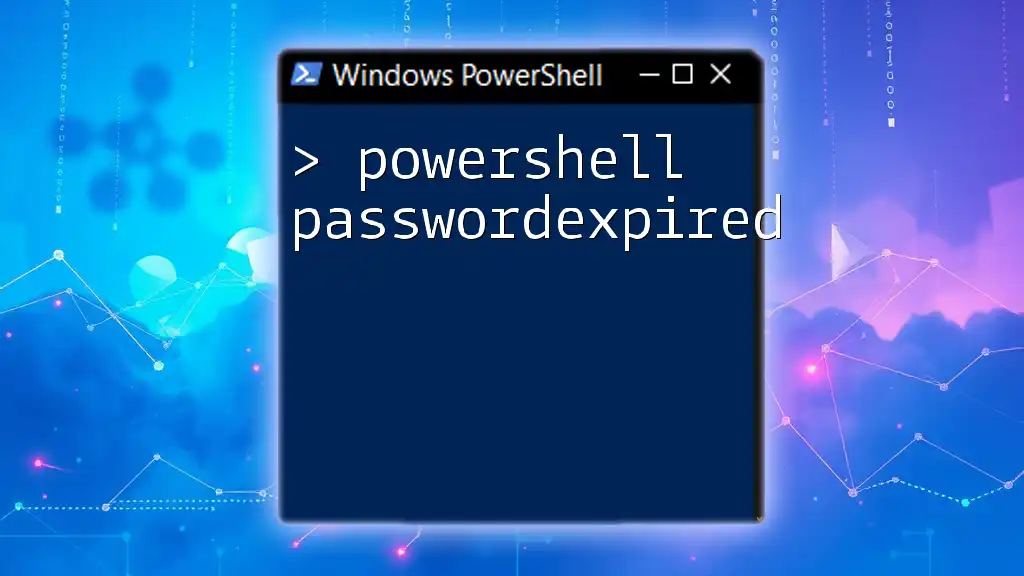
Additional Resources
For further reading and deeper understanding, consult the official Microsoft PowerShell documentation and consider enrolling in reputable PowerShell online courses. By expanding your knowledge, you can become an effective PowerShell user and better manage your IT environment.