The error "requested registry access is not allowed" in PowerShell typically occurs when you do not have the necessary permissions to access a specific registry key.
# Granting permissions to a registry key
$acl = Get-Acl 'HKLM:\Software\YourKey'
$rule = New-Object System.Security.AccessControl.RegistryAccessRule('Username','ReadKey','Allow')
$acl.SetAccessRule($rule)
Set-Acl 'HKLM:\Software\YourKey' $acl
Understanding the Registry
What is the Windows Registry?
The Windows Registry is a hierarchical database that stores configuration settings and options on Microsoft Windows operating systems. It contains information, settings, and options for both the operating system itself and installed applications, making it critical for system performance and behavior.
The structure of the registry consists of keys and values. Keys are akin to folders that store specific configurations, while values represent actual settings or data associated with those keys.
Importance of Registry Management
Managing the registry correctly is vital for several reasons:
- System Configuration: The registry holds essential settings that dictate how Windows and applications behave.
- Troubleshooting: Many issues can be resolved by understanding and modifying the registry settings associated with hardware and software.
- Optimization: Proper management of the registry can lead to better system performance.
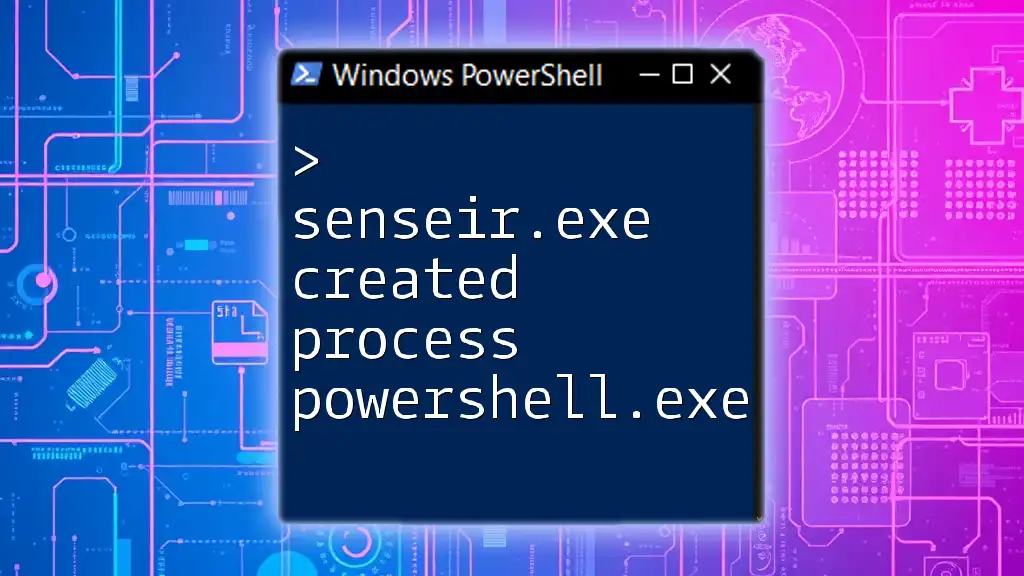
Common Reasons for "Requested Registry Access is Not Allowed"
User Permissions
One of the most common culprits behind the "requested registry access is not allowed powershell" error is user permissions. Windows employs a permissions system to restrict access to the registry, ensuring that unauthorized users cannot make changes that might affect system stability or security. If you're not logged in with an account that has sufficient privileges, you may encounter this error when attempting to modify registry values.
Registry Key Permissions
Beyond user permissions, each registry key itself has security settings that determine who can access or modify it. These security settings include:
- Full Control: Allows complete access to the key.
- Read: Allows viewing the key and its values.
- Write: Allows modifying the values of the key.
Getting a handle on registry key security settings is crucial in resolving access issues.
UAC (User Account Control)
User Account Control (UAC) is a security feature in Windows that helps prevent unauthorized changes to the system. If a PowerShell script tries to modify a registry key without elevated permissions, UAC will block the action. As a result, you may see the "requested registry access is not allowed" message. Understanding how UAC interacts with your PowerShell scripts is essential for smooth registry management.
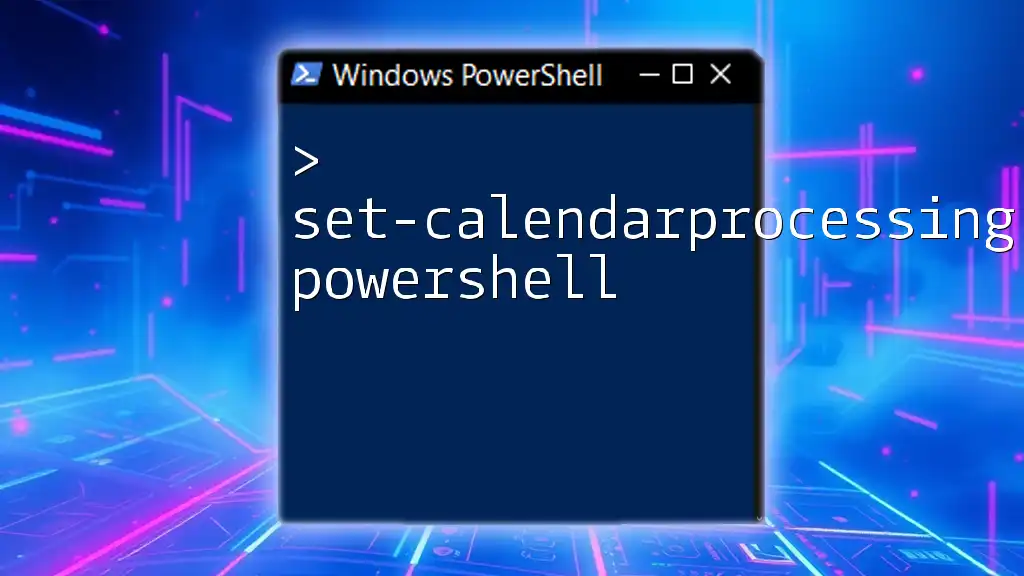
Troubleshooting the Error
Identifying the Problem
Before attempting to fix the error, it is crucial to identify which specific registry key is causing the problem. You can easily check permissions using the `Get-Acl` cmdlet.
Example Command to Check Registry Permissions
Get-Acl -Path "HKLM:\Software\YourKey" | Format-List
This command retrieves the access control list (ACL) for the specified registry key, allowing you to examine the current permissions.
Granting Access to the Registry Key
Once you identify the key and confirm that permissions are the issue, you can change the permissions using PowerShell.
Change Registry Key Permissions
$acl = Get-Acl "HKLM:\Software\YourKey"
$rule = New-Object System.Security.AccessControl.RegistryAccessRule("Everyone", "Read", "Allow")
$acl.SetAccessRule($rule)
Set-Acl -Path "HKLM:\Software\YourKey" -AclObject $acl
In the example above, we retrieve the ACL for the specified key, create a new access rule allowing read permissions to everyone, and then set the new ACL. Adjust the permissions as necessary based on your security needs.
Running PowerShell as Administrator
To avoid encountering the requested registry access issues, it’s imperative to run PowerShell as an administrator. This provides you with elevated permissions required to make changes to the registry:
- Right-click on the PowerShell icon in the Start menu.
- Select Run as administrator.
- You’ll need to confirm that you want to allow the app to make changes.
With elevated privileges, you should have no trouble making the necessary modifications to the registry.
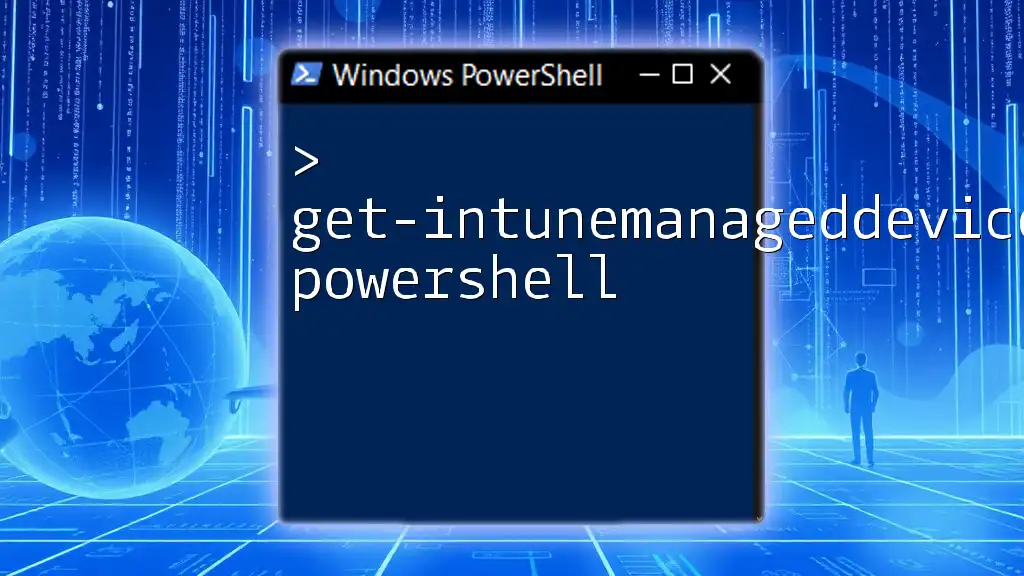
Preventing Future Access Issues
Best Practices for Registry Management
To prevent running into registry access issues in the future, consider the following best practices:
- Back Up the Registry: Always create a backup before making changes. This allows you to restore previous settings if something goes wrong.
- Limit Access: Only grant permissions to users who truly need them and avoid using broad permissions like Everyone unless absolutely necessary.
- Document Changes: Keep a record of any changes made to the registry for future reference.
Using Group Policy for Permissions
For organizations or users managing multiple systems, using Group Policy is an efficient way to enforce consistent settings across all computers. By configuring Group Policy, administrators can set specific permissions for registry keys, ensuring that users have the access they need without compromising security. This can be particularly useful for controlling user access to sensitive keys within the registry.
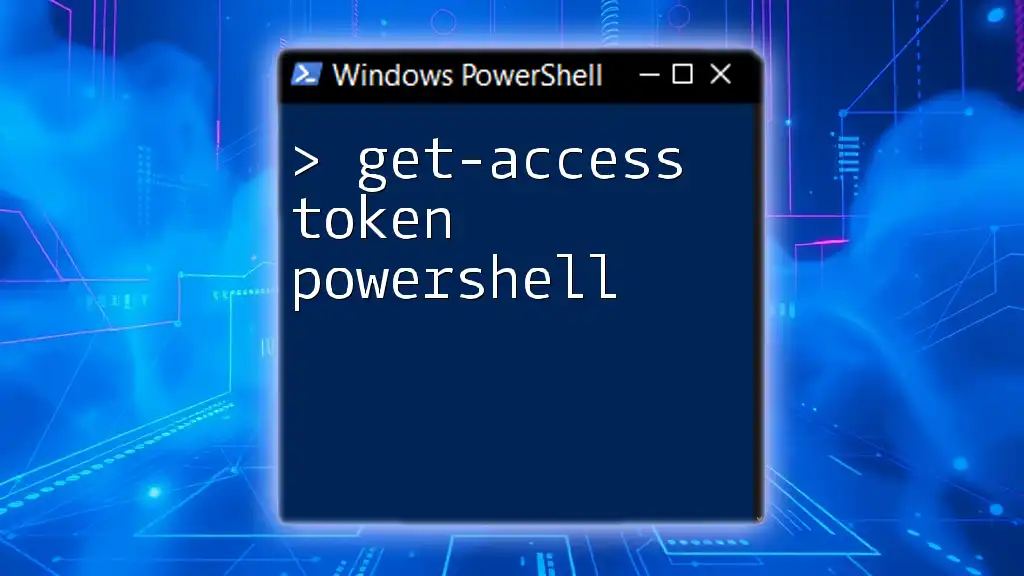
Conclusion
Understanding and resolving the "requested registry access is not allowed powershell" error requires a grasp of Windows registry permissions and user roles. By following the guidance outlined in this article—identifying issues, granting necessary permissions, and adopting best practices—you can effectively manage the registry using PowerShell while minimizing future access problems.
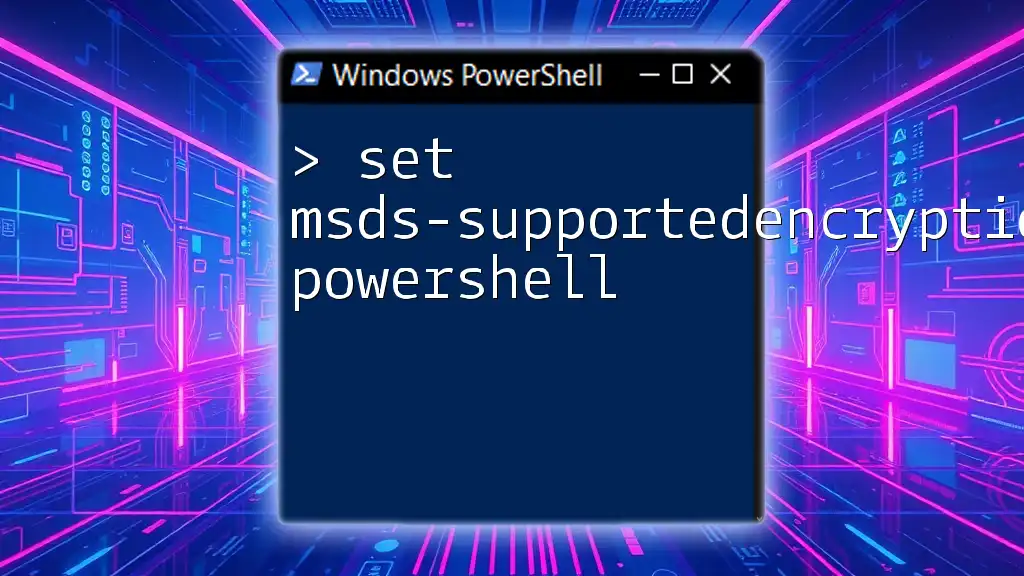
Additional Resources
For more in-depth information, consider exploring the official PowerShell documentation as well as Windows Registry management guides to enhance your understanding and proficiency.
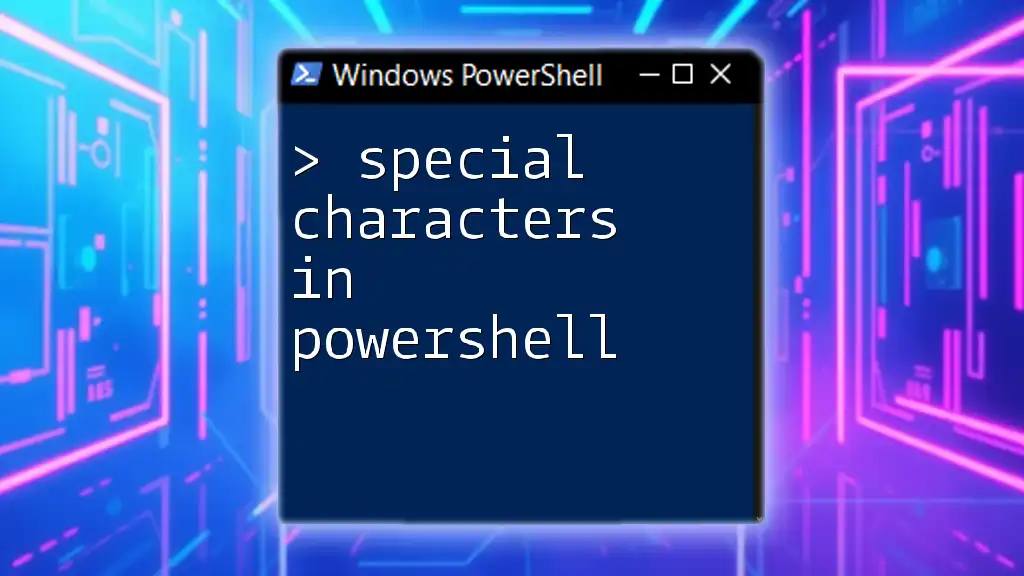
FAQs
Common Questions about Registry Access in PowerShell
-
What to do if I still encounter access issues after following the steps?
- If issues persist, ensure your user account has administrative privileges and verify that no group policies are overriding local permissions.
-
Can I change permissions for all users at once?
- Yes, you can change permissions for all users by specifying "Everyone" when creating new access rules, but be cautious with broad permissions.
-
How can I revert registry permissions after changes are made?
- You can revert permissions by restoring the original ACL from a backup or by using the `Set-Acl` cmdlet to apply previous settings.