To read data from a CSV file in PowerShell, you can use the `Import-Csv` cmdlet, which allows you to easily import the contents of the file into a PowerShell object for further manipulation.
$data = Import-Csv -Path 'C:\path\to\your\file.csv'
Understanding CSV Files
What is a CSV File?
A CSV (Comma-Separated Values) file is a simple text format used to store tabular data, such as spreadsheets or databases. Each line of the file corresponds to a data record, and each record consists of fields, separated by commas. This format is widely adopted due to its simplicity and easy-to-read structure.
Advantages of Using CSV Files
Using CSV files comes with several benefits:
- Simplicity: CSV files are easy to create and edit, even in basic text editors.
- Compatibility: They can be used across various platforms and applications, making data exchange between systems seamless.
- Human-readable: CSV files maintain a straightforward format that can be easily understood by humans.
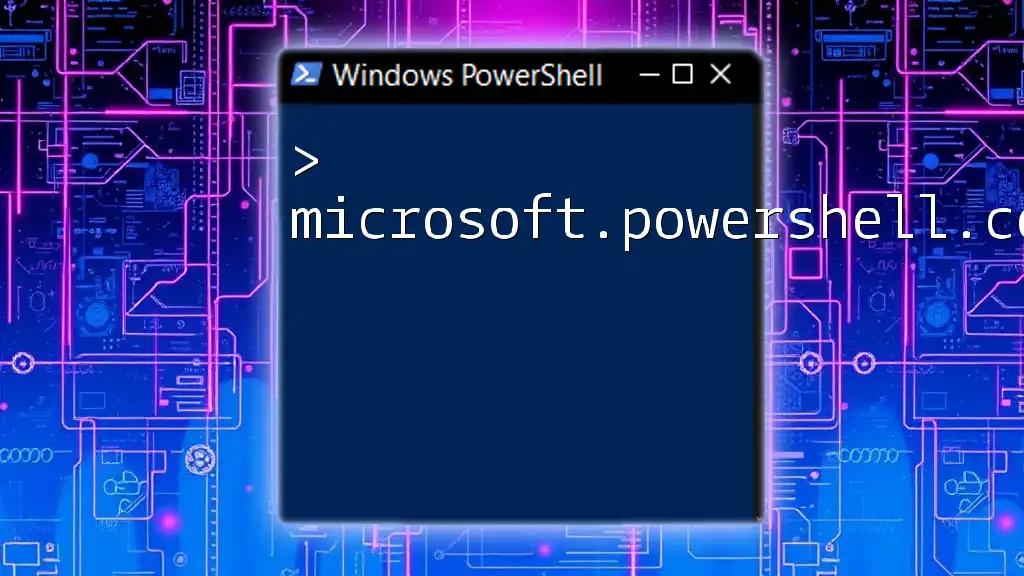
Getting Started with PowerShell
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language developed by Microsoft. It offers a variety of features tailored for administration and automation tasks, allowing users to interact with the file system, manage system settings, and manipulate data, making it particularly effective for handling CSV files.
Preparing Your Environment
To get started, ensure you have PowerShell installed on your machine. Most Windows systems come with it pre-installed. You may want to familiarize yourself with the PowerShell Integrated Scripting Environment (ISE) for ease of coding.
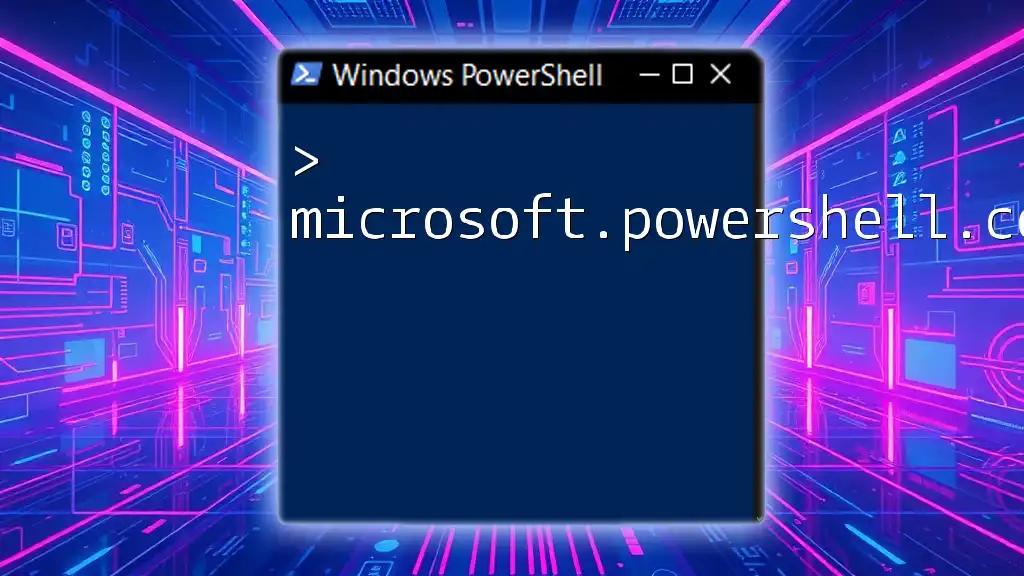
The Basics of Reading CSV Files in PowerShell
Importing CSV Data
To read from a CSV file in PowerShell, you primarily use the `Import-Csv` command. This command imports the contents of a CSV file into a PowerShell object, making it easy to access and manipulate the data.
Example Code Snippet:
$data = Import-Csv -Path "C:\path\to\yourfile.csv"
This command assigns the imported data to the `$data` variable, which you can reference throughout your PowerShell script.
Displaying Imported Data
Once you've imported the data, it’s often useful to view it in a structured format. You can achieve this by using the `Format-Table` command.
Example Code Snippet:
$data | Format-Table
This will display the contents of `$data` in a neat table format, allowing you to easily inspect the information.
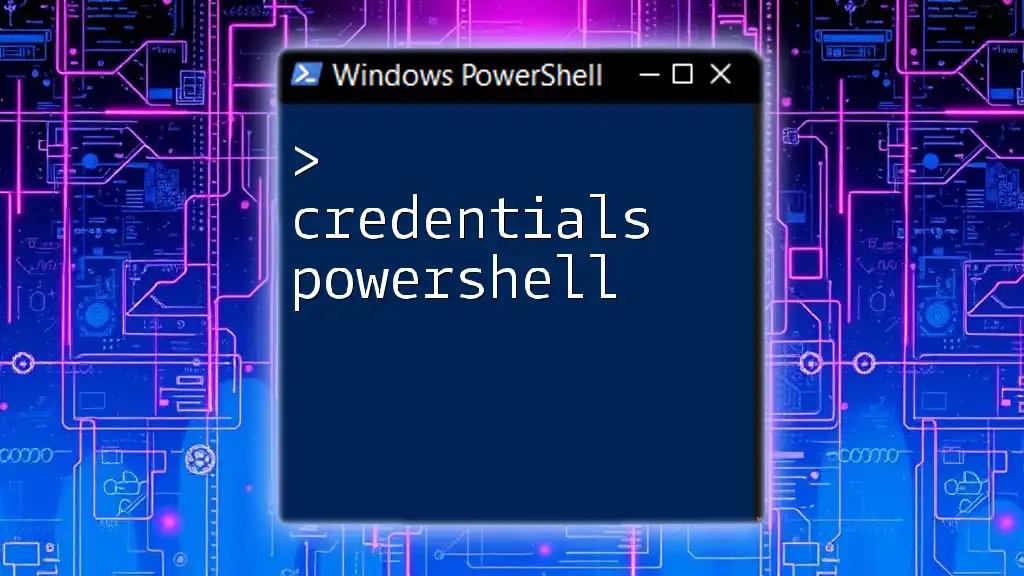
Exploring CSV Data
Accessing Specific Columns
Accessing individual columns from your imported data is straightforward. You can do this by referencing the column name directly from the object.
Example Code Snippet:
$data.ColumnName
This line will return all the values from the column labeled "ColumnName". It’s important to note that if your column names contain spaces, you should wrap the name in quotes, like so:
$data."Column Name With Spaces"
Filtering Data
Filtering rows based on specific criteria is quite powerful in PowerShell. You can use the `Where-Object` cmdlet to filter results.
Example Code Snippet:
$filteredData = $data | Where-Object { $_.ColumnName -eq "value" }
In this snippet, you replace "ColumnName" with the actual column name and "value" with the criteria you want to filter for. The `$_` symbol represents the current object in the pipeline.
Sorting Data
Sorting your data can help you find trends or organize information. To sort CSV data, use the `Sort-Object` cmdlet.
Example Code Snippet:
$sortedData = $data | Sort-Object ColumnName
This command sorts the dataset based on the values in "ColumnName." You can include the `-Descending` parameter if you prefer a reverse order of sorting.
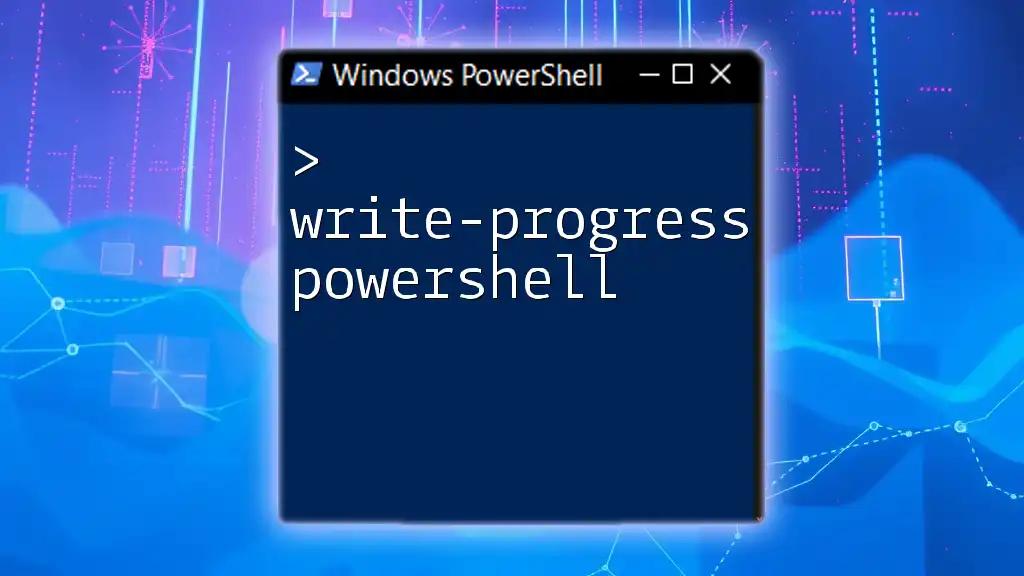
Advanced Techniques for Working with CSV Files
Modifying CSV Data
Adding New Columns
Sometimes, you may need to add new columns to your dataset. This can easily be done using the `Add-Member` cmdlet.
Example Code Snippet:
$data | ForEach-Object { $_ | Add-Member -MemberType NoteProperty -Name NewColumn -Value "DefaultValue" }
This snippet creates a new column called NewColumn for each row, initialized with the DefaultValue.
Removing Unneeded Columns
Just as important as adding new columns is the ability to remove unnecessary ones. You can selectively exclude columns using the `Select-Object` cmdlet.
Example Code Snippet:
$data | Select-Object -Property * -ExcludeProperty UnwantedColumn
This allows you to specify which columns to exclude while retaining others.
Exporting Data Back to CSV
Once you've manipulated your data, you might want to save it back to a CSV file. The `Export-Csv` cmdlet is used for this purpose.
Example Code Snippet:
$data | Export-Csv -Path "C:\path\to\exportedfile.csv" -NoTypeInformation
The `-NoTypeInformation` flag prevents PowerShell from adding a header about the types of the objects being exported, keeping your CSV clean and focused on the data.
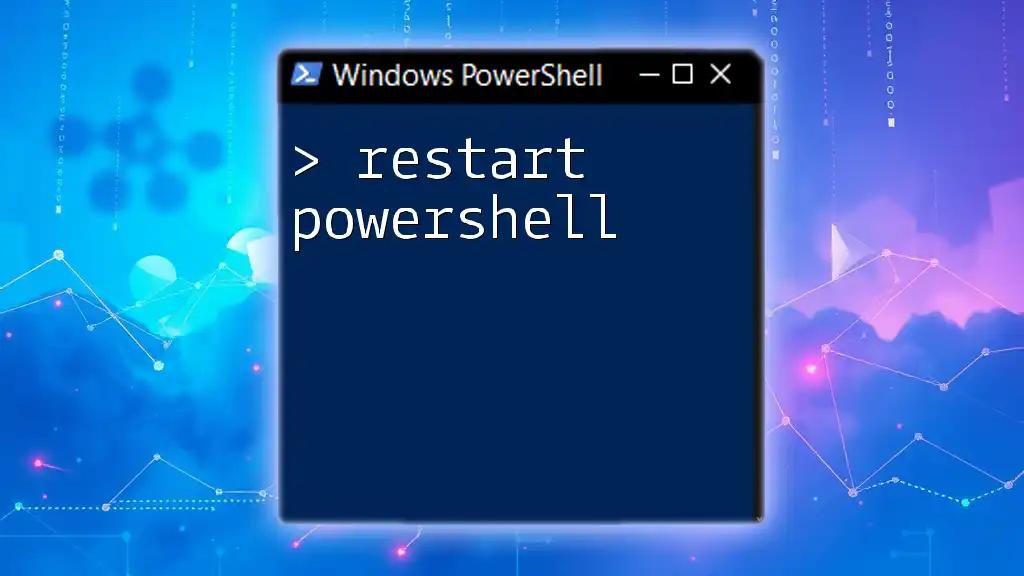
Real-World Applications of Reading CSVs in PowerShell
Common Use Cases
Reading from CSV files in PowerShell can streamline many tasks, such as automating data analysis, preparing reports, or integrating with databases and APIs. Businesses frequently utilize this method to manage bulk data efficiently.
Case Study Example
For instance, a company may use PowerShell to read employee data from a CSV file to generate custom reports that detail performance metrics. By employing filters and sorts, they can easily analyze employee performance over time.
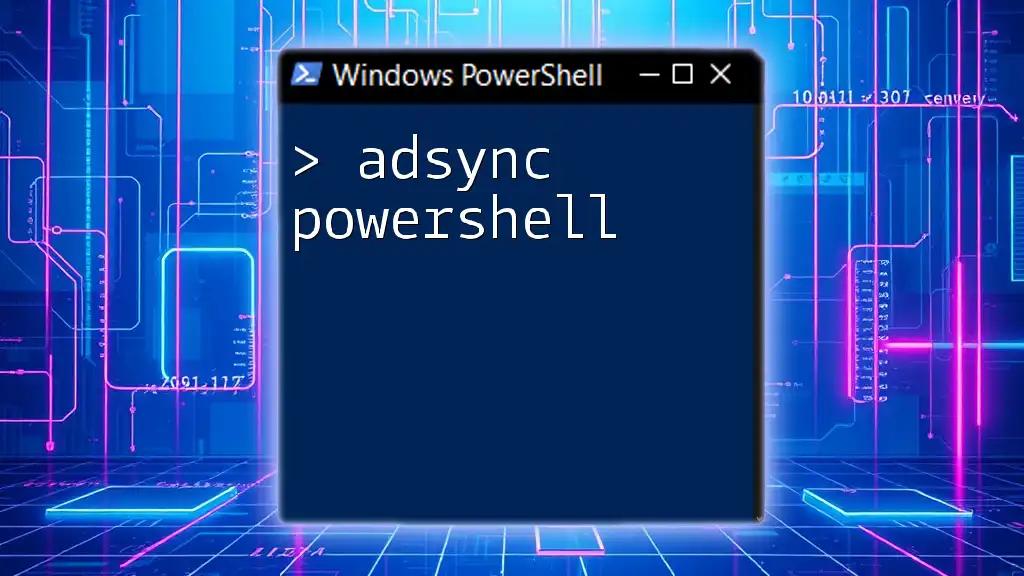
Conclusion
In this article, we've explored how to read from CSV files in PowerShell, from importing to manipulating and exporting data back. Understanding PowerShell commands and their effective application can significantly streamline data handling tasks, allowing for faster decision-making and automation.
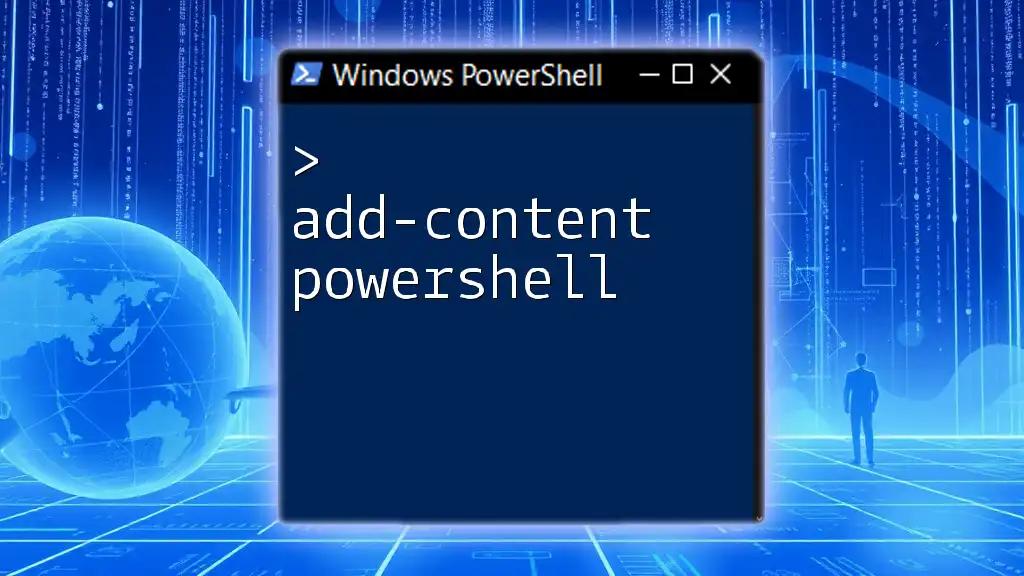
Additional Resources
For further reading and advanced topics, consider checking the official [PowerShell documentation](https://docs.microsoft.com/powershell/) or explore books that delve deeper into PowerShell scripting and data manipulation techniques.