In PowerShell, a yes/no prompt can be created using the `Read-Host` cmdlet to capture user input, allowing for conditional actions based on the response.
Here’s a simple code snippet to demonstrate how to implement a yes/no prompt in PowerShell:
$answer = Read-Host 'Do you want to continue? (Y/N)'
if ($answer -eq 'Y' -or $answer -eq 'y') {
Write-Host 'Continuing...'
} else {
Write-Host 'Process cancelled.'
}
Understanding the Yes/No Prompt
What is a Yes/No Prompt?
A Yes/No prompt is an interactive tool used in PowerShell scripts that allows users to make decisions based on simple binary input—typically "Yes" or "No." These prompts are essential when a script requires user confirmation before continuing with an operation, ensuring that actions are taken deliberately and thoughtfully. They serve as a safety mechanism that helps prevent errors and unintended consequences, especially in critical operations like deleting files or altering system configurations.
Why Use Yes/No Prompts?
Using Yes/No prompts enhances the script's interactivity, making it easier for users to engage with the script. It provides them with control over processes, allowing them to review their choices before proceeding. This is especially important in scripting environments where mistakes can lead to significant problems or data loss.
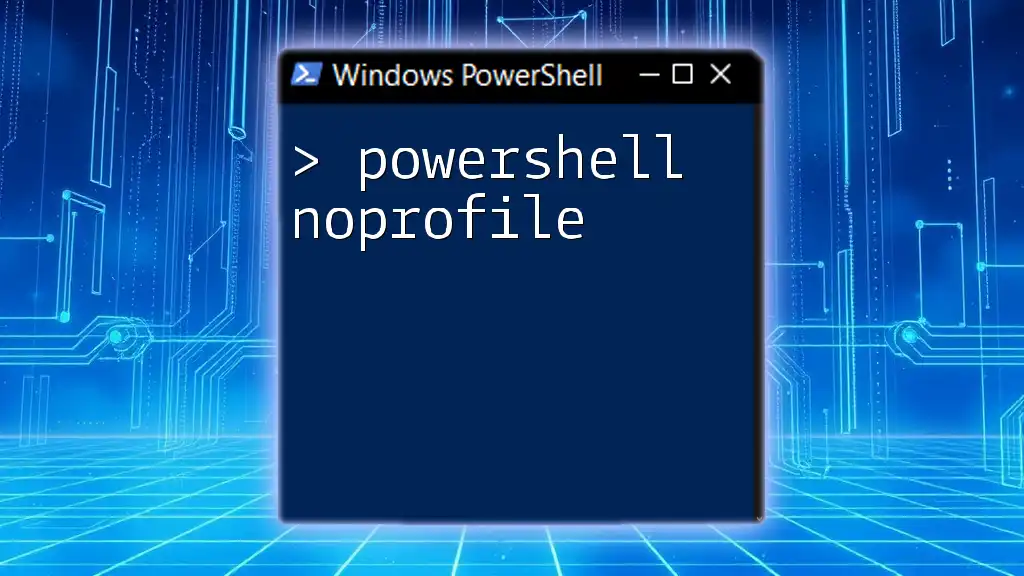
Setting Up a Yes/No Prompt in PowerShell
Basic Syntax
To create a Yes/No prompt in PowerShell, you can use the `Read-Host` cmdlet, which reads user input from the console. The syntax is straightforward:
$response = Read-Host "Do you want to continue? (Y/N)"
This command displays a message in the console asking the user if they want to continue, and it captures the input in the `$response` variable.
Handling User Input
It's a good practice to standardize the user input, especially since users may enter input in various formats, such as uppercase or lowercase. To ensure that the script processes input consistently, you can convert the response to lowercase:
$response = Read-Host "Do you want to continue? (Y/N)"
$response = $response.ToLower()
This simple conversion allows you to handle user input without worrying about case sensitivity.
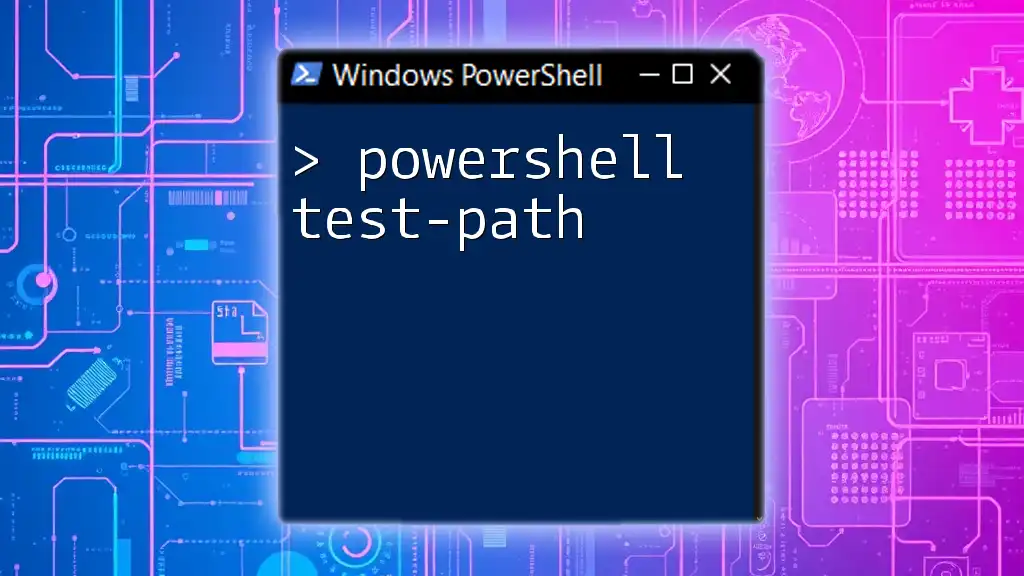
Implementing Yes/No Logic in Scripts
Basic If-Else Structure
The core functionality of a Yes/No prompt comes from its ability to control the flow of operations based on user input. You can utilize a basic `if-else` structure to determine the action to take based on the user's response:
if ($response -eq 'y') {
Write-Host "Continuing with the operation..."
} elseif ($response -eq 'n') {
Write-Host "Operation cancelled."
} else {
Write-Host "Invalid input. Please enter Y or N."
}
In this example, if the user types "Y", the script continues the operation. If they type "N", it cancels. Any other input prompts an error message, guiding the user back to valid options.
Using Switch Statement (Advanced)
For more complex prompts where multiple responses might need to be handled, a `switch` statement can be a more elegant solution. It allows for cleaner code and easier management of various cases:
switch ($response) {
'y' { Write-Host "Continuing with the operation..." }
'n' { Write-Host "Operation cancelled." }
default { Write-Host "Invalid input. Please enter Y or N." }
}
This approach neatly categorizes input handling and can be expanded to include more options if needed in the future.
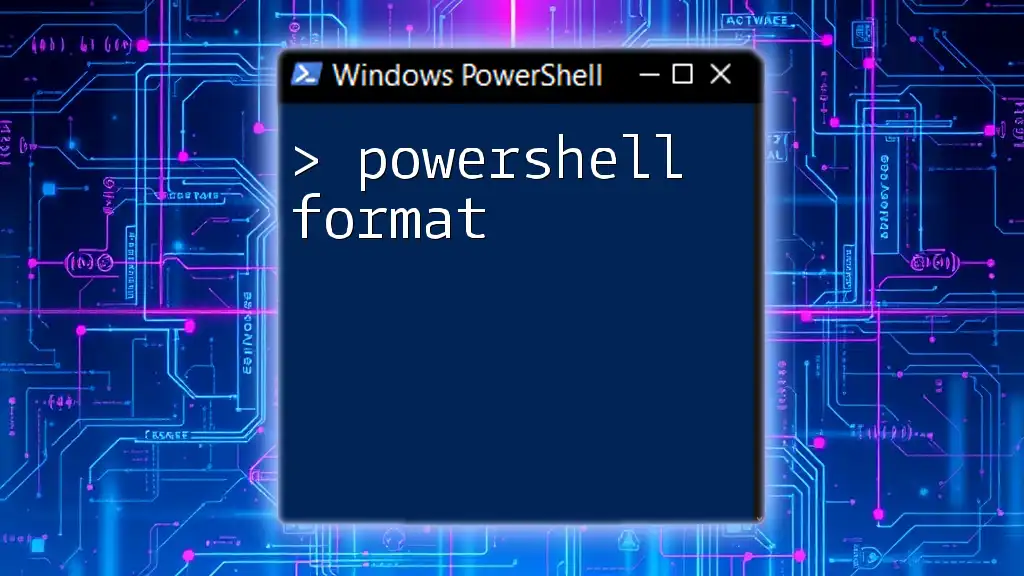
Enhancing User Experience
Custom Messages
To make your scripts more user-friendly, consider personalizing prompts and messages based on the context in which they are being executed. Clear and specific questions can significantly improve user experience by ensuring that the user understands what they are agreeing to.
$response = Read-Host "Would you like to execute the backup? (Y/N)"
In this example, the prompt directly references the action for clarity.
Looping Until Valid Input
Sometimes users might input incorrect options. To ensure the script only proceeds with valid input, implement a loop that repeatedly asks for input until it receives a proper answer:
do {
$response = Read-Host "Do you want to proceed with the operation? (Y/N)"
$response = $response.ToLower()
} while ($response -ne 'y' -and $response -ne 'n')
This method prevents the script from progressing until valid input is received, enhancing reliability and control.
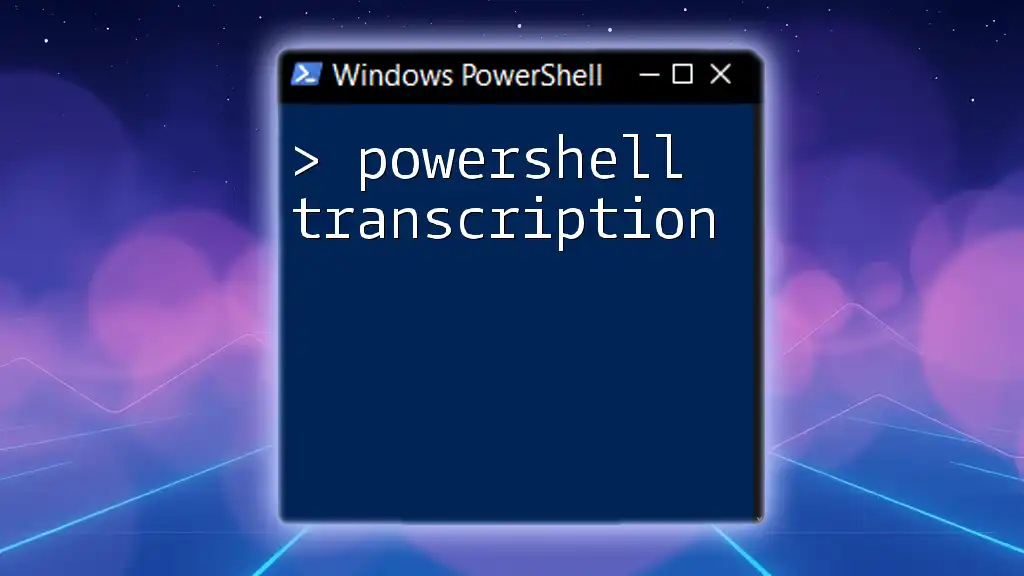
Best Practices for Yes/No Prompts
Clear Instructions
Always provide clear instructions in your prompts. Ambiguous questions can lead to confusion, resulting in incorrect input or decisions that may affect operations negatively.
Error Handling
Implement robust error handling to guide users when their input is invalid. This ensures that even if the user strays from expected inputs, they have a path back to successful interaction.
Testing Your Scripts
Before deploying scripts with prompts to production environments, thoroughly test them for expected behaviors. This testing ensures that every possible user input—including unexpected ones—is handled gracefully.
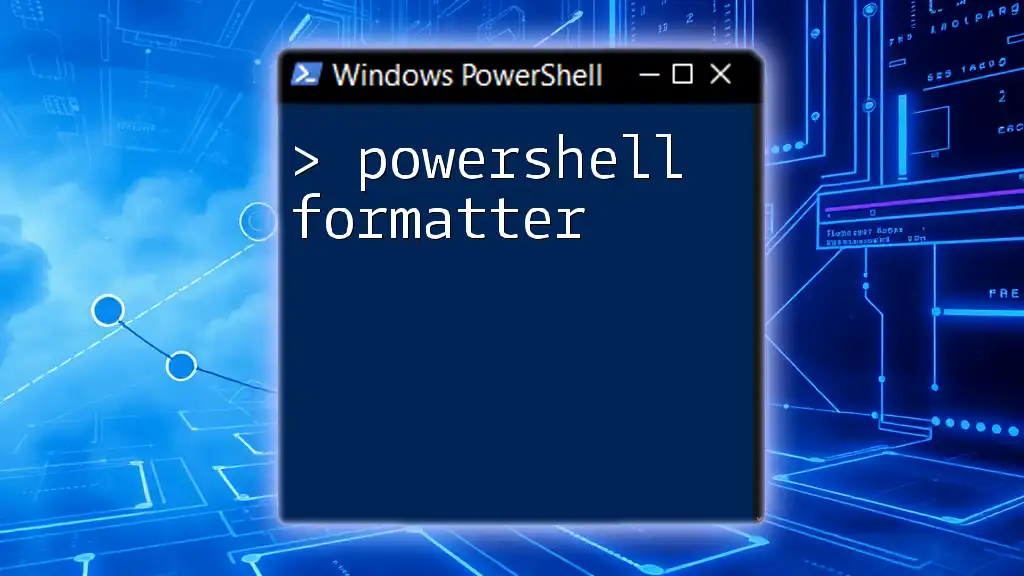
Real-World Applications of Yes/No Prompts
Automation Scripts
Automation scripts often require user confirmation before executing potentially destructive actions. For instance, if a script deletes temporary files, it’s wise to prompt the user for consent first to prevent accidental data loss.
User-Interactive Tools
In a broader context, PowerShell Yes/No prompts are invaluable in creating user-interactive tools that require user input throughout their execution. This interactivity enhances the tool's usability by making it clear when user attention is needed.
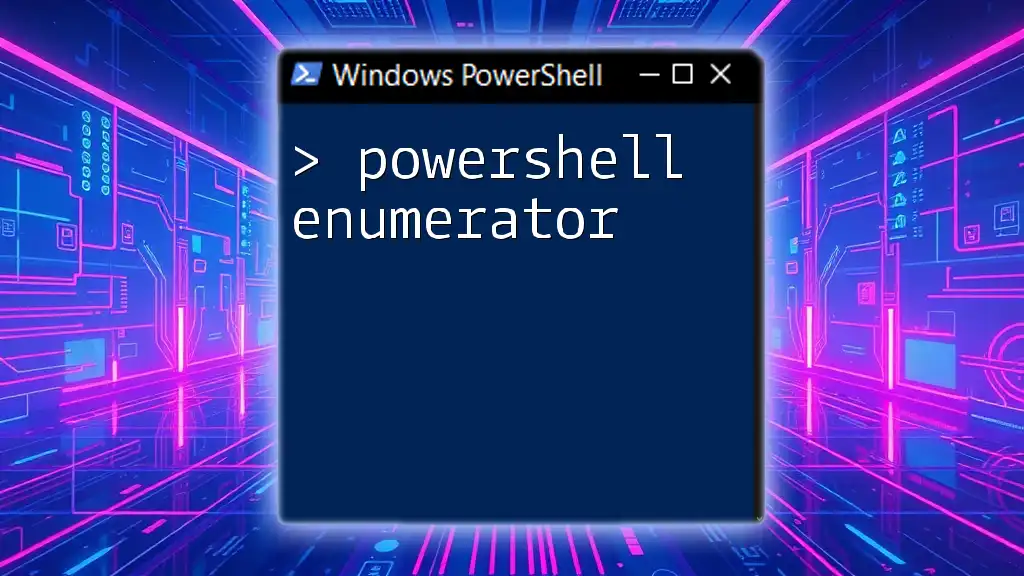
Conclusion
Implementing a PowerShell Yes/No prompt is a straightforward yet powerful way to enhance your scripting capabilities. By engaging users and inviting them to make conscious decisions, you can create scripts that are not only functional but also user-friendly and reliable. Incorporating prompts elevates the user experience and safeguards critical operations from unintended consequences.