Sure! Here's a concise explanation along with a code snippet for your post:
A PowerShell script to copy files from a source to a destination is a simple yet powerful command that allows users to duplicate files efficiently with just one line of code.
Copy-Item -Path "C:\Source\*" -Destination "D:\Destination\" -Recurse
Understanding the Basics of File Management in PowerShell
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language developed by Microsoft. Built on the .NET Framework, it is designed to automate the administration of the Windows operating system and its applications. With its ability to interact with various system components and manage tasks, PowerShell has become an essential tool for system administrators and IT professionals.
The Importance of File Copy Scripts
File copying is a fundamental task in many IT workflows, whether for backups, file migrations, or organizing files across directories. Using scripts to automate these processes offers several advantages over manual copying. Automation increases efficiency, reduces the likelihood of human error, and allows for reproducibility in handling file operations. By learning how to write an effective PowerShell script to copy files from source to destination, you can streamline your file management tasks considerably.
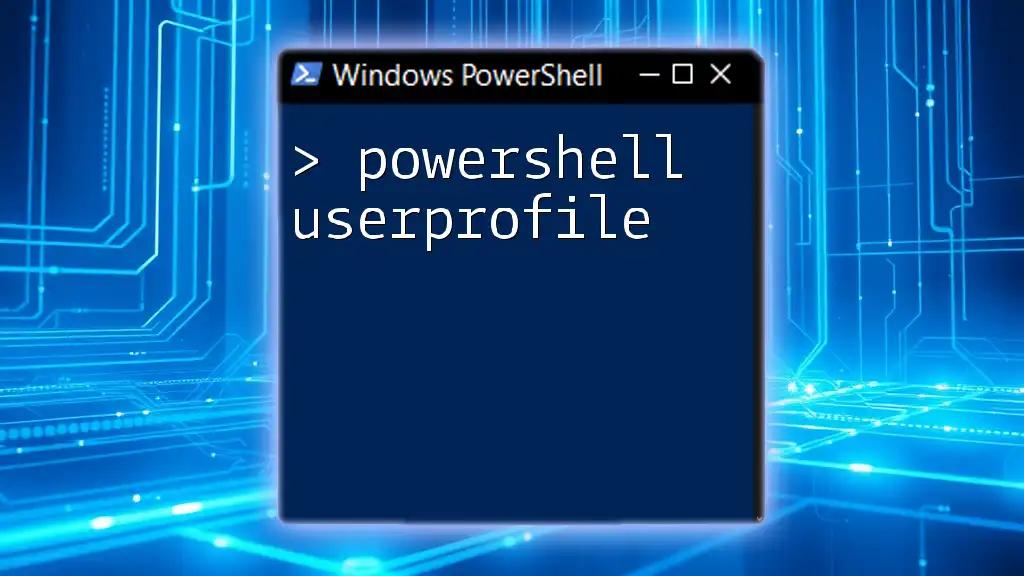
The `Copy-Item` Cmdlet
Overview of `Copy-Item`
The `Copy-Item` cmdlet in PowerShell is explicitly designed for copying files and directories. Understanding its syntax is crucial for writing robust scripts. The basic syntax of `Copy-Item` is as follows:
Copy-Item -Path <string> -Destination <string> [-Recurse] [-Force] [-Verbose]
This command allows you to specify the source items you want to copy and where you want to send them.
Common Parameters Used with `Copy-Item`
-Path
The -Path parameter indicates the source files or directories you wish to copy. For instance, to copy all files from a specific source directory, you can use:
Copy-Item -Path "C:\Source\*" -Destination "C:\Destination\"
This command specifies that every file in the `C:\Source\` directory should be copied to `C:\Destination\`.
-Destination
The -Destination parameter determines where the copied files will be saved. For example, if you want to copy a specific file:
Copy-Item -Path "C:\Source\File.txt" -Destination "C:\Backup\File.txt"
This command copies `File.txt` from the source to the backup directory.
-Recurse
The -Recurse parameter is particularly useful when dealing with directories. It allows you to include all files and subdirectories in the copy operation. For example:
Copy-Item -Path "C:\Source\" -Destination "C:\Backup\" -Recurse
This command ensures that everything in `C:\Source\` is copied to `C:\Backup\`, maintaining the folder structure.
-Force
The -Force parameter is used to overwrite existing files in the destination without prompting the user. This is essential in automated scripts where you want to ensure the copy happens without interruptions. For example:
Copy-Item -Path "C:\Source\Example.txt" -Destination "C:\Destination\Example.txt" -Force
This command will overwrite `Example.txt` if it already exists in the destination.
-Verbose
The -Verbose parameter provides feedback during the copy operation, which can be invaluable for debugging and ensuring the script runs as expected. Here’s how to implement it:
Copy-Item -Path "C:\Source\" -Destination "C:\Destination\" -Verbose
Using this command will display detailed information about each file being copied.
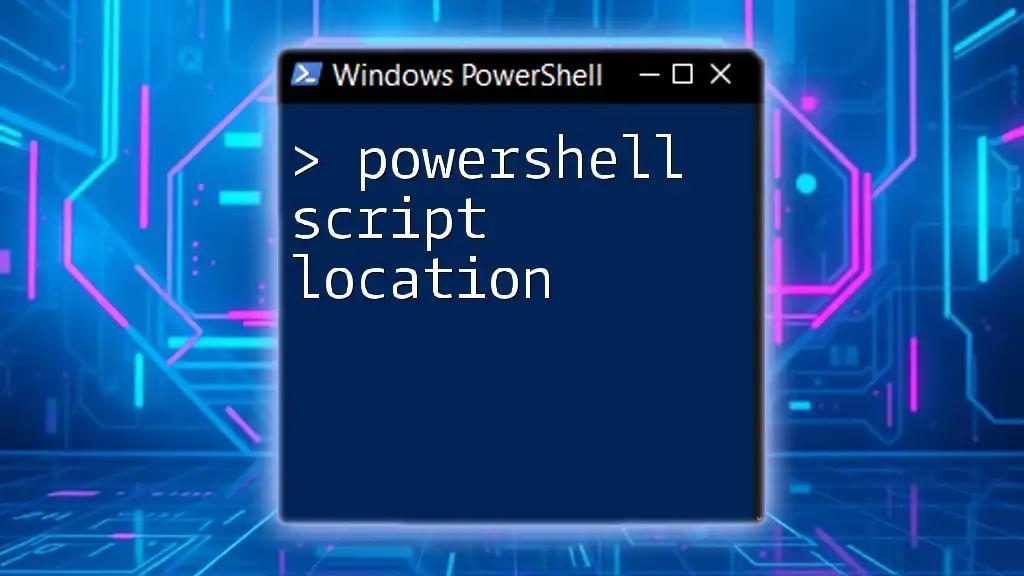
Writing a Simple PowerShell Script to Copy Files
Creating a Script File
To create a PowerShell script, you can use any text editor to write your command and save it with a `.ps1` extension. Here’s a simple example of a script that copies files:
$source = "C:\Source\"
$destination = "C:\Destination\"
Copy-Item -Path $source -Destination $destination -Recurse -Verbose
Above, the script defines a source and destination path, then performs the copy operation while providing verbose output to track the process.
Running Your PowerShell Script
To execute your script, open PowerShell and navigate to the directory containing your script. You can run it by typing the script name preceded by a dot-slash like so:
.\YourScriptName.ps1
If you encounter issues related to execution policies, you may need to allow scripts to run by adjusting the policy settings. You can do this with the following command, which requires administrative privileges:
Set-ExecutionPolicy RemoteSigned
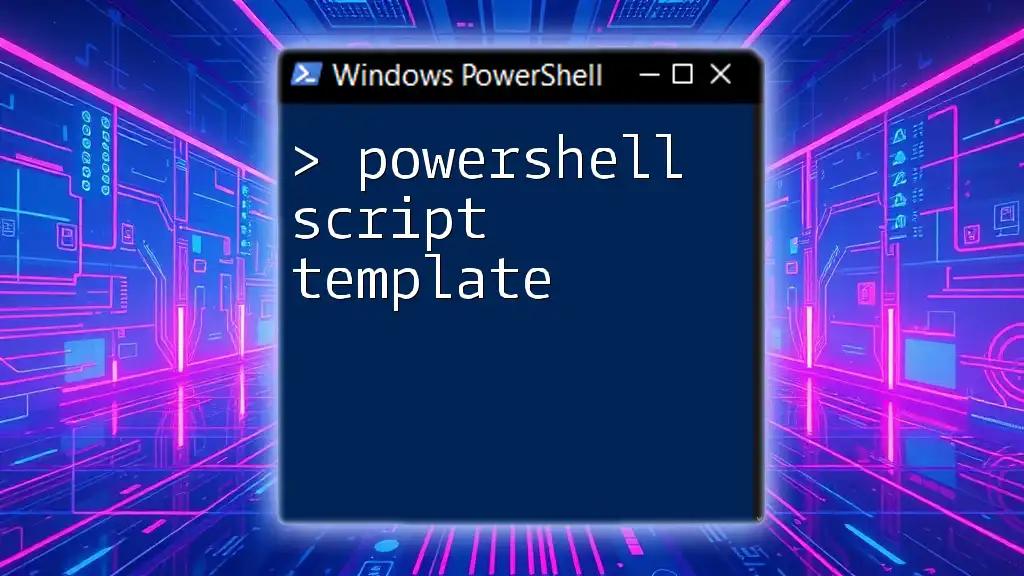
Advanced File Copy Techniques
Copying Files Based on Filters
Using Wildcards
PowerShell supports the use of wildcards, making it easier to specify which files to copy based on patterns. For example, to copy only text files, you can use:
Copy-Item -Path "C:\Source\*.txt" -Destination "C:\Destination\" -Recurse
This command instructs PowerShell to copy only files with the `.txt` extension.
Copying with Error Handling
Adding error handling in your script can make it more resilient to issues that may arise, such as missing source files or inaccessible directories. You can incorporate `try/catch` blocks as shown below:
try {
Copy-Item -Path "C:\Source\*" -Destination "C:\Destination\" -ErrorAction Stop
} catch {
Write-Host "Error: $_"
}
This structure will catch any errors during the copy process and display a message indicating what went wrong.
Logging Copy Operations
Logging can help you keep track of copy operations and verify their success. To do so, you can redirect the output to a log file as follows:
$logFile = "C:\log.txt"
Copy-Item -Path "C:\Source\" -Destination "C:\Destination\" -Recurse -Verbose | Out-File -FilePath $logFile
This command copies files and logs detailed operations into `log.txt`, allowing for easy review later.
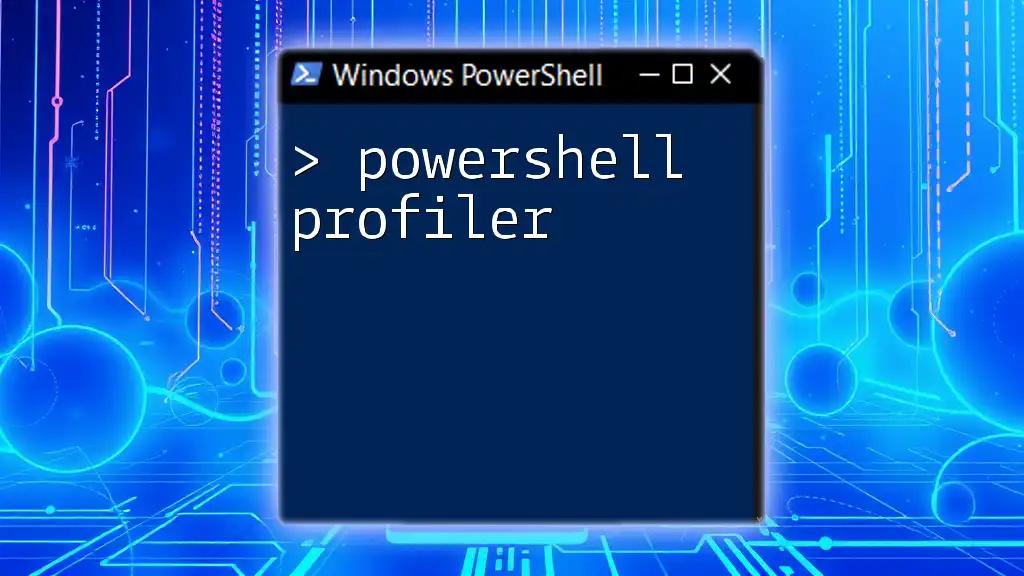
Conclusion
In this article, we've thoroughly explored how to create a PowerShell script to copy files from source to destination. The `Copy-Item` cmdlet, along with its associated parameters, offers great flexibility and power to automate file management tasks effectively. We encourage you to experiment with various parameters and scenarios to discover all the capabilities PowerShell can offer. Don't hesitate to share your own scripts and ideas, and continue exploring the vast world of PowerShell automation!
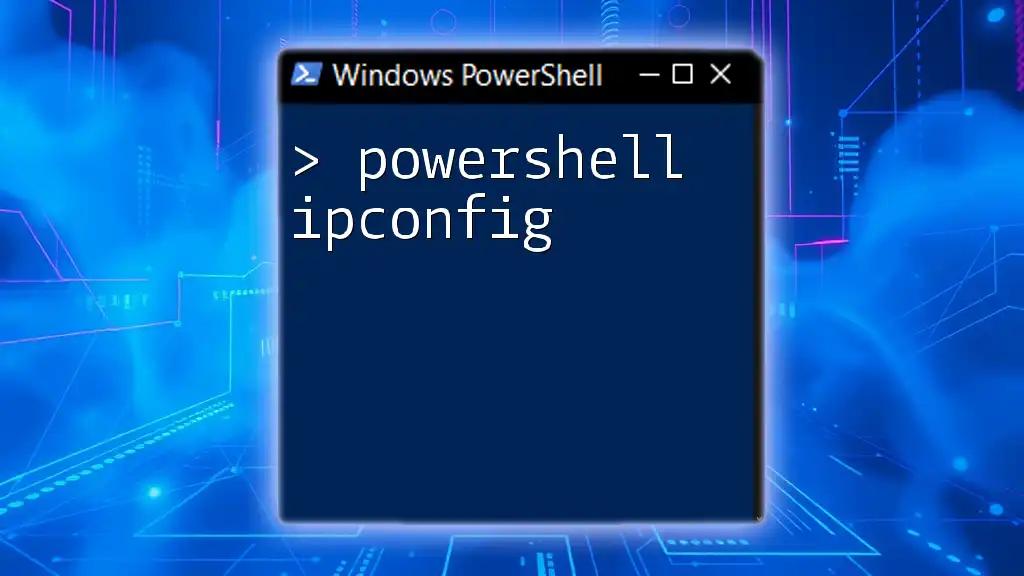
Additional Resources
Recommended Reading
For further information, consider checking the official Microsoft documentation for PowerShell, which provides comprehensive details about cmdlets, syntax, and best practices. Additionally, many online courses can deepen your understanding of scripting and automation.
Example Scripts Repository
For practical examples, look for repositories dedicated to PowerShell scripts where you can find ready-to-use commands related to file management and share your own creations with the community.