PowerShell can run scripts or commands in the background using the `Start-Job` cmdlet, allowing for concurrent execution without blocking the main console.
Start-Job -ScriptBlock { Write-Host 'Hello, World!' }
Understanding Background Processes
What is a Background Process?
A background process is a program or command that runs behind the scenes while the user continues to interact with other commands and applications. Unlike foreground processes, which require user input and have exclusive control over the console, background processes allow users to continue working without interruption. This is particularly useful for long-running tasks, batch processing, or operations that consume considerable resources.
The main benefit of executing commands in the background is that it helps maintain user productivity by freeing up the console for other tasks. For instance, you could initiate a resource-intensive operation while simultaneously checking system status or executing additional commands.
Common Use Cases for Background Execution
Running PowerShell commands in the background serves various purposes, including:
- Long-running scripts: Automating tasks that take time, such as data processing or system checks.
- Batch processing: Executing multiple scripts or commands at once without needing user input.
- Scheduled tasks: Running scripts at predefined times or intervals without manual initiation.
- Resource-intensive operations: Performing tasks that may slow down the system if executed in the foreground.
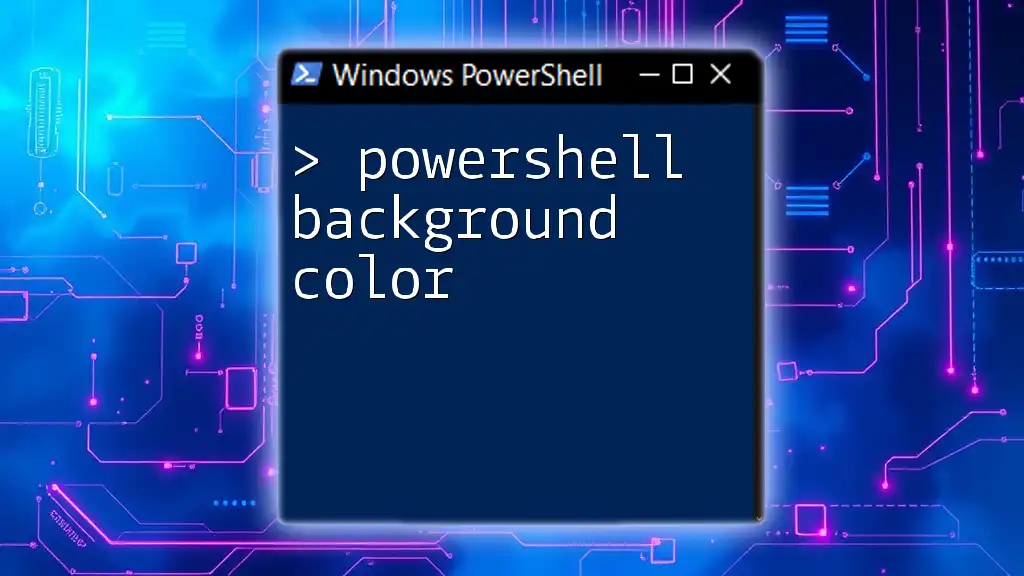
PowerShell Methods for Running in the Background
Using Start-Job Cmdlet
The `Start-Job` cmdlet is one of the primary tools for launching background jobs in PowerShell. This cmdlet creates a new job that runs the specified command or script block.
Syntax and Parameters
The syntax is straightforward. Here’s an example:
Start-Job -ScriptBlock { Get-Process }
This command starts a job that retrieves the list of running processes without locking up the PowerShell console.
How to Retrieve Results
Once you have started a job, you can retrieve its results using `Get-Job` and `Receive-Job`. Here’s how:
$job = Start-Job -ScriptBlock { Get-Process }
Get-Job
Receive-Job -Id $job.Id
In this example, `$job` holds the job object, which allows you to check its status with `Get-Job`. When you call `Receive-Job`, you can get the output from the background job.
Using the Invoke-Command Cmdlet
The `Invoke-Command` cmdlet allows for both local and remote execution of commands. When used with the `-AsJob` parameter, it enables running tasks in the background effectively.
Syntax and Parameters
An example of local execution in the background would be:
Invoke-Command -ScriptBlock { Get-Service } -AsJob
Collecting Results
Just as with `Start-Job`, you can retrieve results from background processes initiated by `Invoke-Command`.
$job = Invoke-Command -ScriptBlock { Get-Process } -AsJob
Receive-Job -Job $job
In this case, the command retrieves the list of processes, and you can access the results just like any other background job.
Using Scheduled Tasks
Scheduled tasks provide a robust way to run PowerShell scripts in the background without manual intervention continuously. They can be created using PowerShell cmdlets.
Creating a Scheduled Task
Below is an example of how to set up a scheduled task that runs a PowerShell script at startup:
$action = New-ScheduledTaskAction -Execute 'PowerShell.exe' -Argument '-File C:\Path\To\YourScript.ps1'
Register-ScheduledTask -Action $action -Trigger (New-ScheduledTaskTrigger -AtStartup) -TaskName "MyBackgroundTask"
This command prepares an action that runs a PowerShell script upon system startup. Now, the task runs at startup without any user action required.
Benefits of Scheduled Tasks
Creating scheduled tasks through PowerShell provides multiple benefits:
- Automation: Scheduled tasks can be set to run at specific times or triggered by system events.
- Robustness: Tasks can run even when no user is logged in, making them suitable for server environments.
- Logging: Tasks can log their output, allowing for easier troubleshooting and performance tracking.
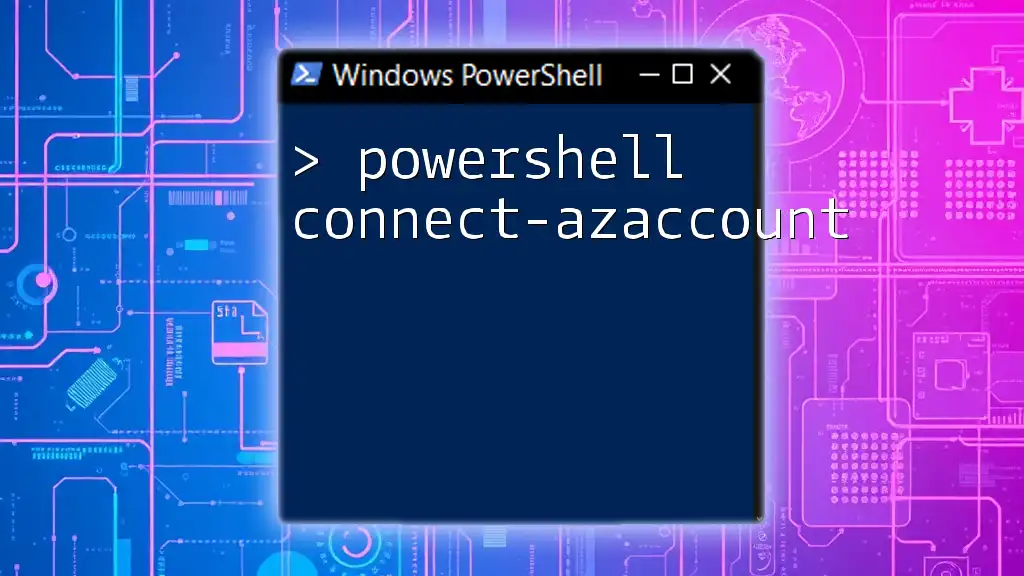
Monitoring and Managing Background Jobs
Checking Job Status
To effectively manage background jobs, monitoring their status is essential. `Get-Job` lists active jobs, while providing information on their state.
Get-Job | Where-Object { $_.State -eq 'Running' }
This command filters the jobs and displays only those currently running, making it easier to manage ongoing processes.
Stopping and Removing Jobs
If you need to stop or remove jobs, PowerShell provides the respective commands:
To stop a job, use:
Stop-Job -Id $job.Id
This command forcibly terminates the specified job.
To remove a completed or stopped job from memory, employ:
Remove-Job -Id $job.Id
Doing this keeps your job list clean and manageable.
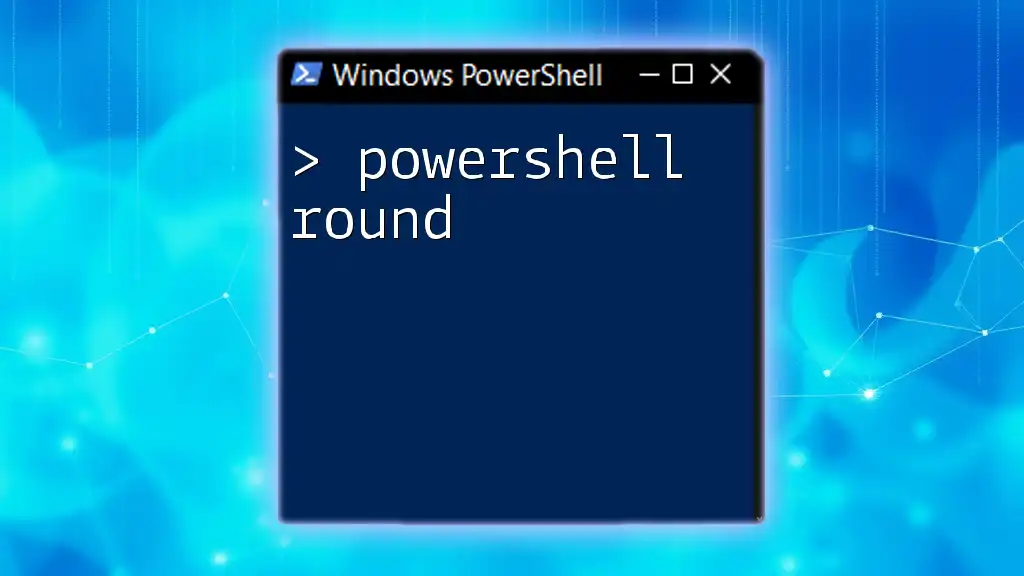
Best Practices When Running PowerShell in Background
Error Handling
Effective error handling is crucial for background jobs. Logging any errors that arise allows for troubleshooting and enhances script reliability. Implementing try/catch blocks is one way to catch and log errors.
Performance Considerations
Background jobs can consume system resources. Optimize your scripts to reduce load. Examples include:
- Limiting the number of concurrent jobs.
- Efficiently structuring scripts to prevent bottlenecks.
Security Aspects
Always follow security best practices. Run jobs with the least privilege required, utilizing secure strings and appropriate permissions to protect sensitive information.
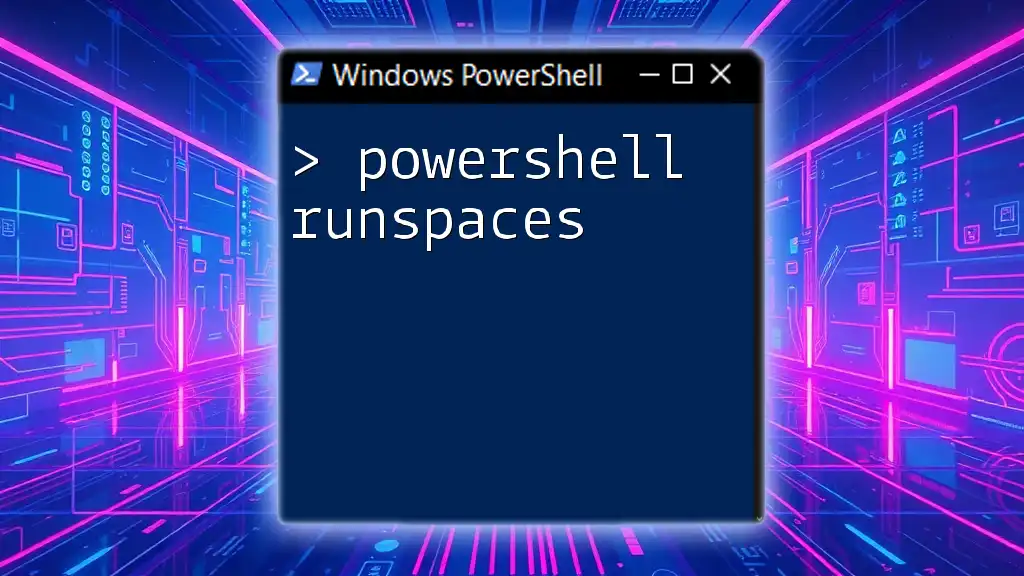
Troubleshooting Common Issues
Common Errors and Solutions
You may encounter issues such as jobs failing to run or producing no output. Typical solutions involve thorough checking of your script syntax and ensuring permissions are correctly set.
Debugging Background Scripts
Debugging background scripts can be challenging. Use techniques like logging errors to a file to capture significant events during execution. For instance:
$job = Start-Job -ScriptBlock { Get-Process; throw "Error!" }
Receive-Job -Job $job -Wait -ErrorAction SilentlyContinue | Out-File -FilePath "C:\Path\To\Log.txt"
This command captures errors in a log file, allowing you to review issues after job completion.
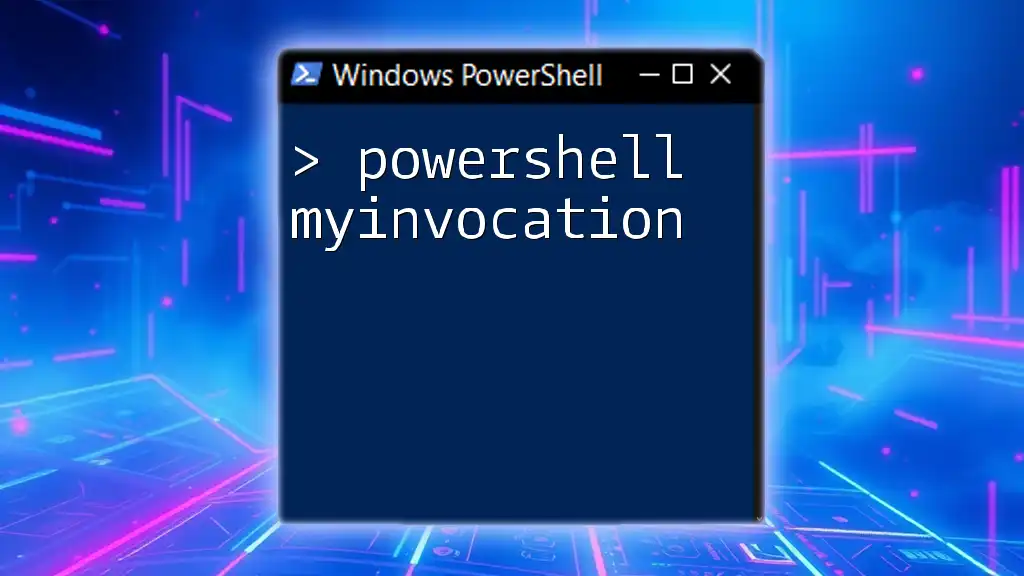
Conclusion
Understanding how to run PowerShell commands in the background is vital for efficient system management and automation. By leveraging cmdlets such as `Start-Job`, `Invoke-Command`, and scheduled tasks, you can enhance productivity while minimizing the impact on your day-to-day operations. Explore these techniques to further streamline your workflow and take full advantage of the capabilities PowerShell has to offer!
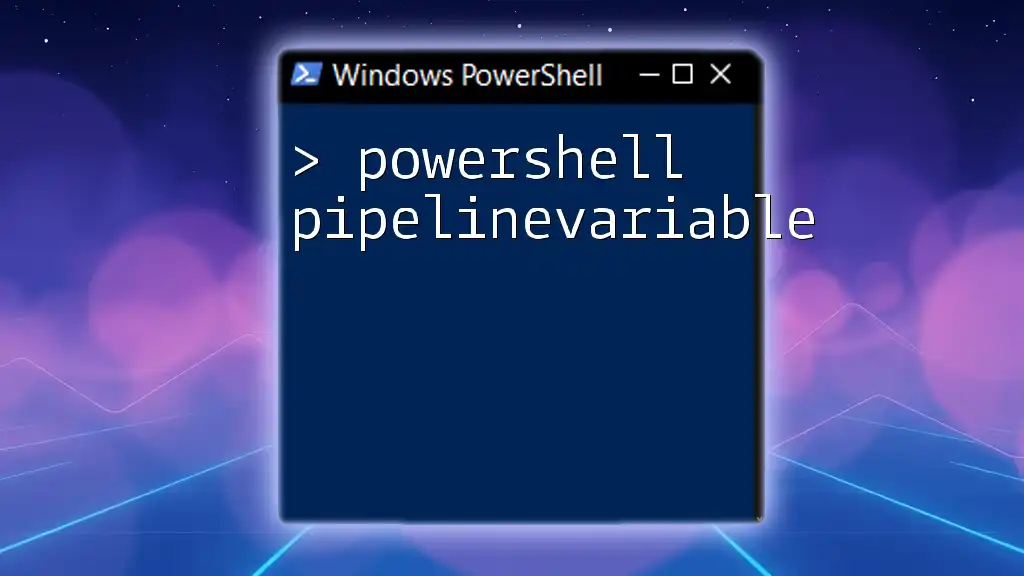
Additional Resources
For more information, refer to the official PowerShell documentation or check out recommended books and courses designed for further learning on PowerShell.