In PowerShell, the "less than or equal to" operator (`-le`) is used to compare two values, returning `True` if the left operand is less than or equal to the right operand.
$number1 = 5
$number2 = 10
$result = $number1 -le $number2
Write-Host $result # This will output 'True'
What is the 'Less Than or Equal To' Operator?
The less than or equal to operator in PowerShell is defined by `-le`. This operator allows you to compare two values and return a boolean `True` or `False`, depending on whether the left operand is less than or equal to the right operand. This operator plays a crucial role in decision-making processes within your PowerShell scripts.
Syntax of Using `-le`
The basic syntax of the `-le` operator is:
$result = $a -le $b
In this statement, `$result` will be `True` if `$a` is less than or equal to `$b`; otherwise, it will be `False`.
Key Differences from Other Comparison Operators
The `-le` operator is one of several comparison operators in PowerShell. Other related operators include `-lt` (less than), `-ge` (greater than or equal to), `-gt` (greater than), and `-eq` (equal to). Understanding the differences between these operators can significantly enhance your ability to write effective scripts and conditional statements.
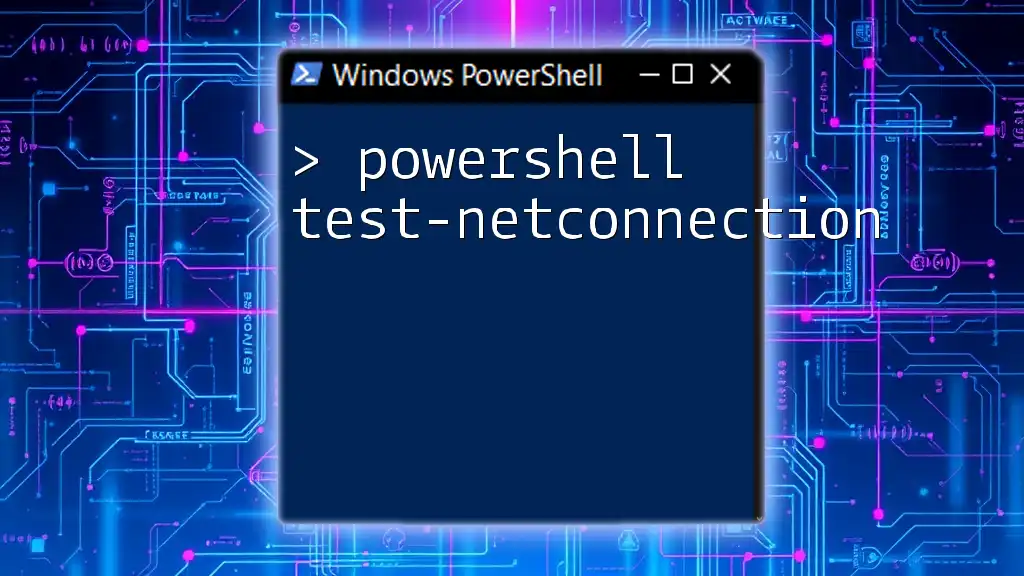
How to Use the 'Less Than or Equal To' Operator in PowerShell
Syntax and Basic Examples
Example 1: Basic Numeric Comparison
One of the simplest applications of the `-le` operator is in numeric comparisons. Consider the following code:
$x = 5
$y = 10
$result = $x -le $y
Write-Output $result # Outputs: True
In this example, `$x` is indeed less than `$y`, so the output will be `True`. This demonstrates how straightforward it is to use the `-le` operator for direct numerical comparisons.
Example 2: Comparing Strings
You can also use the `-le` operator for string comparisons. PowerShell allows string comparison based on their lexicographical order:
$str1 = "Apple"
$str2 = "Banana"
$result = $str1 -le $str2
Write-Output $result # Outputs: True
In this case, "Apple" comes before "Banana" in the dictionary; thus, the output is `True`. It’s important to note that string comparisons are case-insensitive by default in PowerShell.
Combining Multiple Conditions
The `-le` operator can be combined with other conditions using logical operators such as `-and` and `-or`. This enables you to craft more complex conditional statements.
Example: Using `-le` in an If Statement
$a = 3
$b = 5
if ($a -le $b) { Write-Output "$a is less than or equal to $b" }
Here, since `$a` is indeed less than `$b`, the output will confirm that the statement holds true.
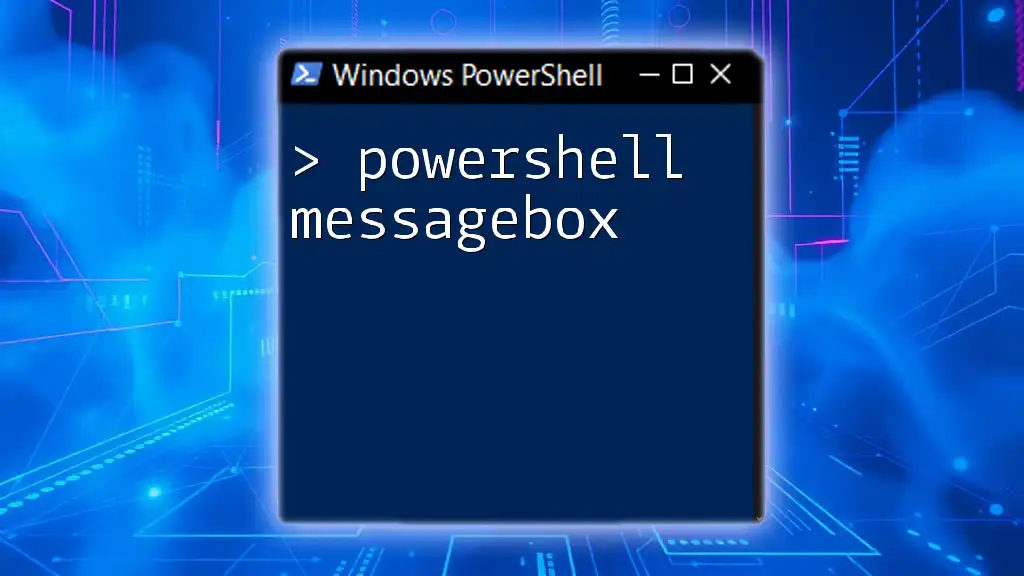
Other Related Comparison Operators in PowerShell
Greater Than or Equal To: The `-ge` Operator
The `-ge` operator serves the opposite function of `-le`. It checks if the left operand is greater than or equal to the right operand.
Example of `-ge` in Action
$a = 10
$b = 5
$result = $a -ge $b
Write-Output $result # Outputs: True
In this case, `$a` is greater than `$b`, which results in a `True` output.
Less Than: The `-lt` Operator
Conversely, the `-lt` (less than) operator is essential for checks where you want to determine if one value is strictly smaller than another. Understanding how these operators work in tandem can help simplify complex scripts.
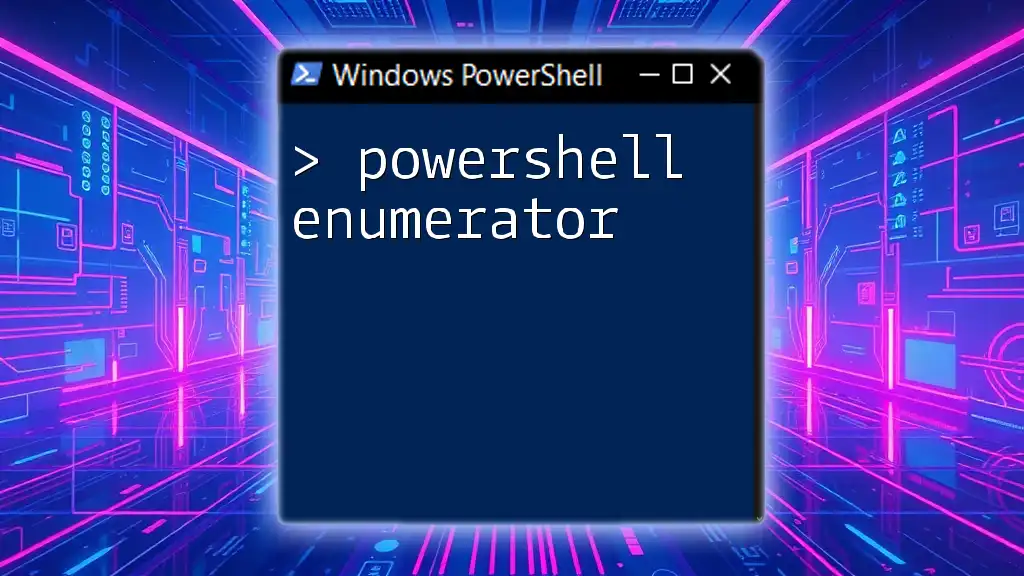
Real-World Scenarios for 'Less Than or Equal To'
Filtering Data
In practical applications, the `-le` operator is invaluable for filtering data. For instance, assume you have an array of numbers, and you want to extract those that are less than or equal to five:
$numbers = 1..10
$filtered = $numbers | Where-Object { $_ -le 5 }
Write-Output $filtered # Outputs: 1 2 3 4 5
In this example, the `Where-Object` cmdlet uses the `-le` operator to filter the numbers efficiently.
Conditional Logic in Scripting
You can also leverage the `-le` operator to handle user input through conditional logic. For example, consider the scenario where you request a user's age and return appropriate feedback:
$age = Read-Host "Enter your age"
if ($age -le 18) {
Write-Output "You are a minor."
} else {
Write-Output "You are an adult."
}
This script aptly demonstrates how the `-le` operator can facilitate simple yet effective logic paths based on user input.
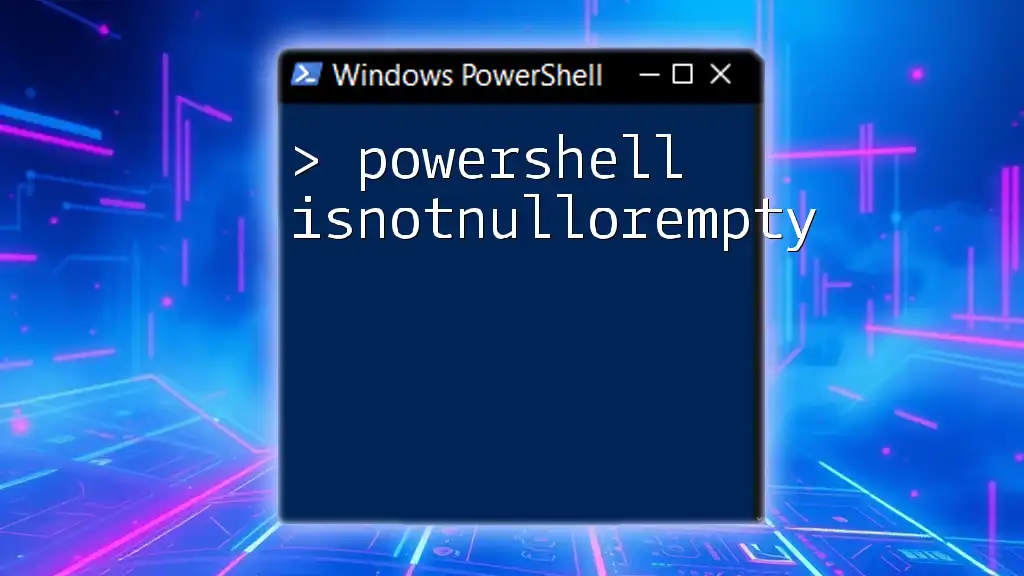
Common Mistakes When Using Comparison Operators
While utilizing the `-le` operator, there are common pitfalls to avoid:
Comparing Incompatible Types
One of the most frequent errors is attempting to compare incompatible types (e.g., strings and integers). For example:
$x = "5"
$y = 10
$result = $x -le $y # May produce unexpected results
Write-Output $result
Always ensure that the variables you are comparing are of the same type to avoid errors or misleading results.
Misunderstanding Logical Flow in Conditionals
Another common mistake is neglecting the logical flow in conditionals. Misplacing operators can easily lead to unintended consequences or logic that does not behave as expected.
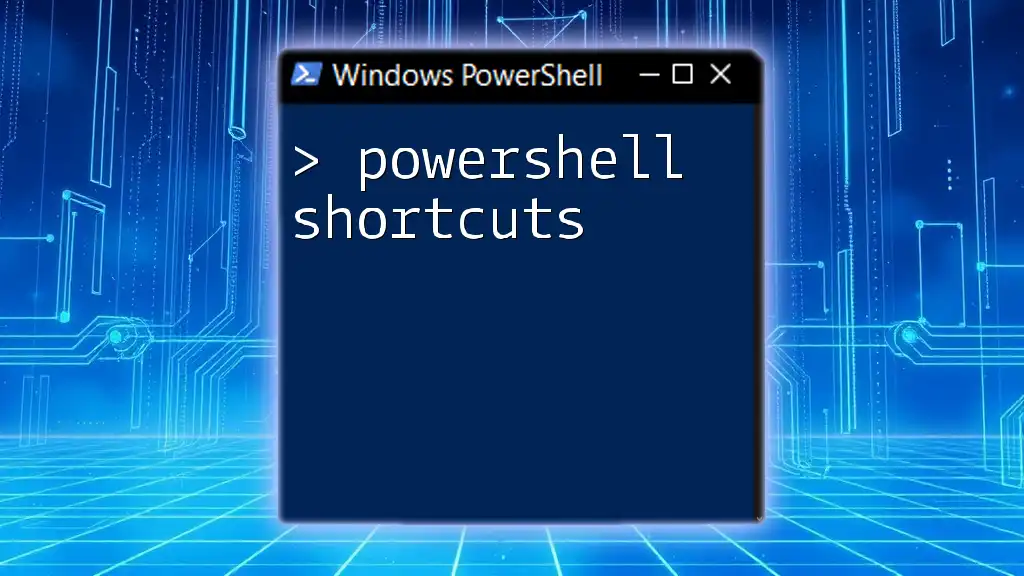
Conclusion
The PowerShell less than or equal to operator (`-le`) is a powerful tool that simplifies comparisons between values in your scripts. By mastering the use of this operator and understanding its relationship with other comparison operators, you can craft more efficient and effective PowerShell scripts. The examples and scenarios provided demonstrate the versatility and utility of the `-le` operator, making it an indispensable part of your PowerShell toolkit.
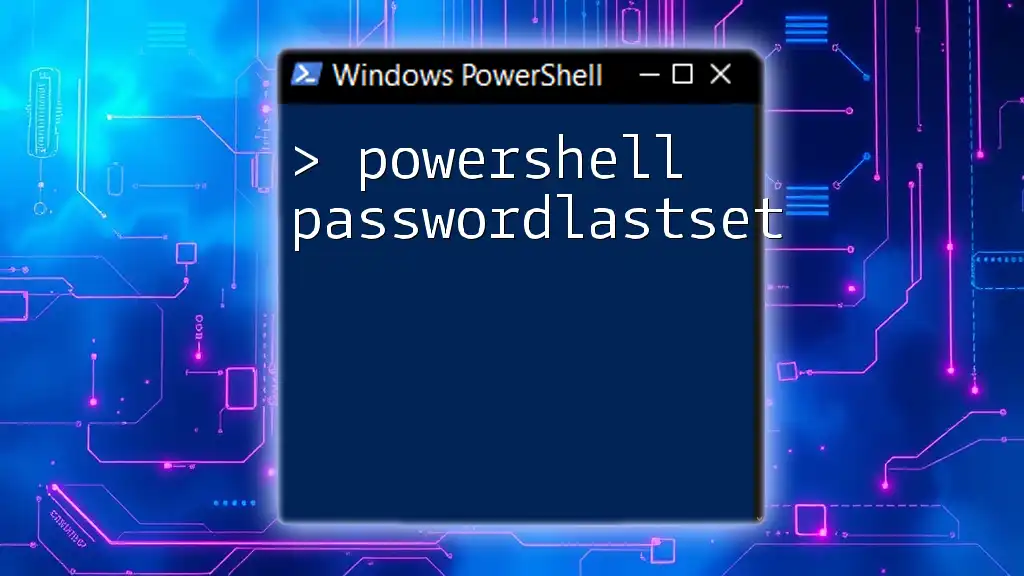
Resources for Further Learning
To deepen your understanding of PowerShell, we recommend exploring the official PowerShell documentation, engaging with community forums, and checking out online tutorials and books focused on scripting. These will offer invaluable insights and advanced techniques to enhance your PowerShell skills further.