In PowerShell, you can use the `-gt` (greater than) operator to compare two values and execute a command based on that condition, as illustrated in the code snippet below:
if (5 -gt 3) { Write-Host '5 is greater than 3' }
Understanding PowerShell Conditional Statements
What Are Conditional Statements?
Conditional statements are fundamental constructs in programming that allow you to execute different blocks of code based on specific conditions. They enable you to make decisions in your scripts, which is invaluable when automating system tasks or managing data.
Why Use If Statements?
The if statement is one of the most powerful tools in a programmer's toolbox. It enables you to evaluate conditions and execute code based on whether those conditions are true or false. Real-world applications abound; for instance, you might use if statements to verify user input, check security permissions, or dynamically adjust system configurations based on environmental variables.
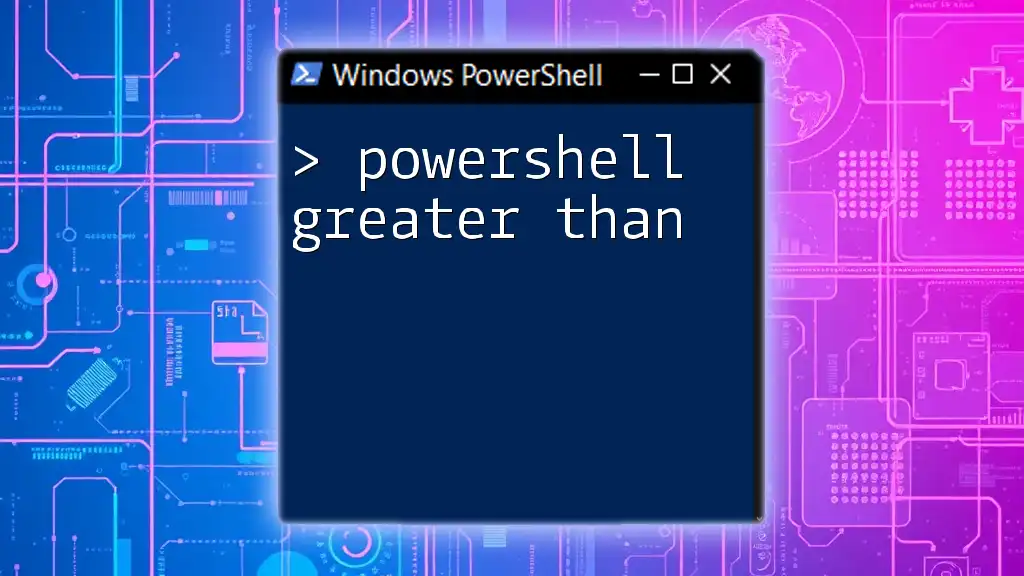
The Greater Than Operator in PowerShell
What Does "Greater Than" Mean?
In PowerShell, the greater than operator is denoted as `-gt`. It is used to compare two values, returning true if the left operand is greater than the right operand. Understanding how to utilize this operator effectively is essential for performing comparisons in your scripts.
PowerShell Comparisons: Understanding Operators
PowerShell provides a suite of comparison operators that allow you to evaluate conditions. Here’s a quick overview:
- `-lt`: Less than
- `-le`: Less than or equal to
- `-gt`: Greater than
- `-ge`: Greater than or equal to
- `-eq`: Equal to
- `-ne`: Not equal to
These operators form the backbone of decision-making in PowerShell scripts.
Syntax of the If Statement with Greater Than
The basic syntax for an if statement using the greater than operator is quite straightforward. Here’s how you structure it:
if (<condition>) {
<code to execute if condition is true>
}
For example, you might write:
if ($value -gt $threshold) {
"Value is greater than threshold"
}
Here, if the condition `$value -gt $threshold` evaluates to true, PowerShell will execute the code block inside the curly braces.
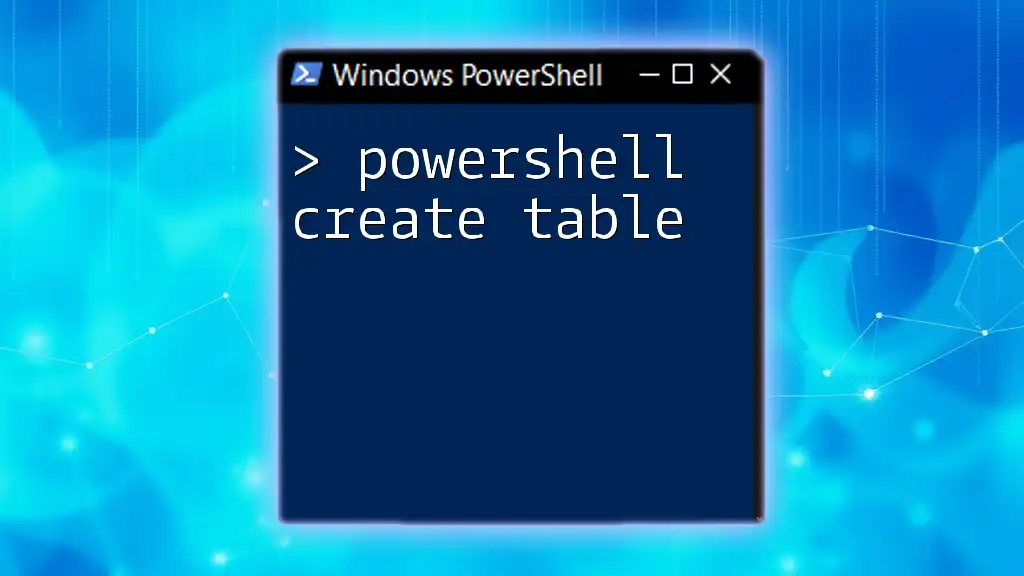
Practical Examples of If Greater Than
Simple Single Condition Example
Let’s consider a basic scenario where you compare two numbers. This will illustrate the usage of the greater than operator clearly.
$a = 10
$b = 5
if ($a -gt $b) {
"a is greater than b"
}
In this example, since `$a` is indeed greater than `$b`, PowerShell outputs: “a is greater than b.”
Advanced Example Using Variables
You may also encounter instances where you want to check a variable against a predefined threshold. Here’s a typical use case:
$score = 75
if ($score -gt 50) {
"You have passed"
}
In this case, because `$score` is greater than 50, the script will return the message: “You have passed.”
Real-World Application Example
PowerShell can also be very useful when working with files. Let’s check if a file's size exceeds a certain limit.
$file = Get-Item "C:\path\to\file.txt"
$maxSize = 1MB # 1 Megabyte
if ($file.Length -gt $maxSize) {
"File size exceeds maximum allowed size."
}
In this example, the script retrieves the file and compares its length to the defined `$maxSize`. If it exceeds 1 MB, it outputs a warning message.
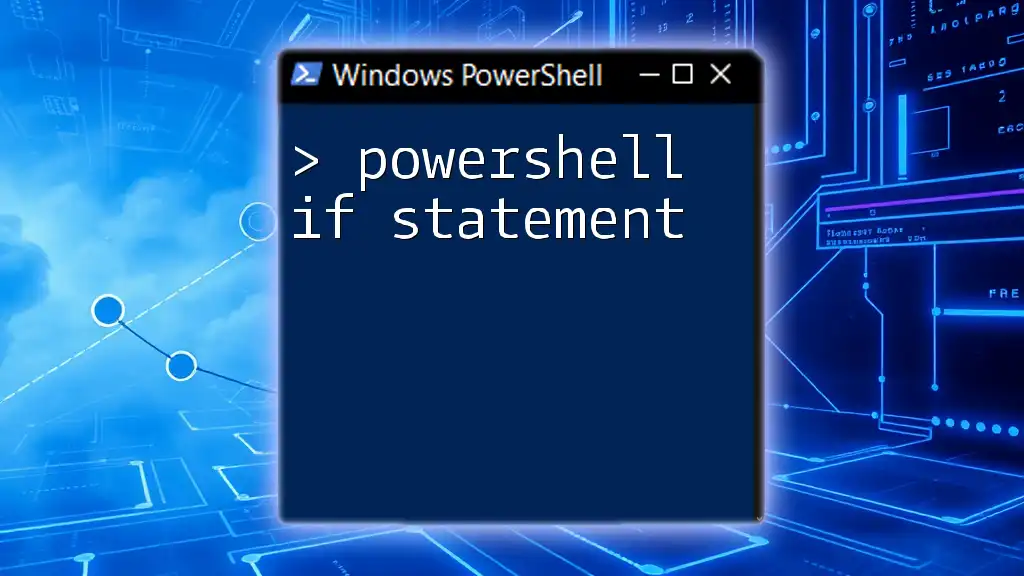
Combining Greater Than with Other Comparison Operators
Using Greater Than with Less Than
You can create more complex conditions by combining the greater than operator with others, like less than. Here’s an example:
$number = 15
if ($number -gt 10 -and $number -lt 20) {
"Number is between 10 and 20."
}
In this case, the condition checks that `$number` is somehow within a specified range. Here, it would return “Number is between 10 and 20.”
Nested If Statements
You may require more intricate logic, which can be accomplished with nested if statements. This is useful when multiple conditions must be checked.
$value1 = 30
$value2 = 20
if ($value1 -gt 25) {
if ($value2 -lt 25) {
"Value1 is greater than 25 and Value2 is less than 25."
}
}
This structure allows for a clear evaluation of multiple conditions, providing your script the flexibility it needs to adapt to different scenarios.
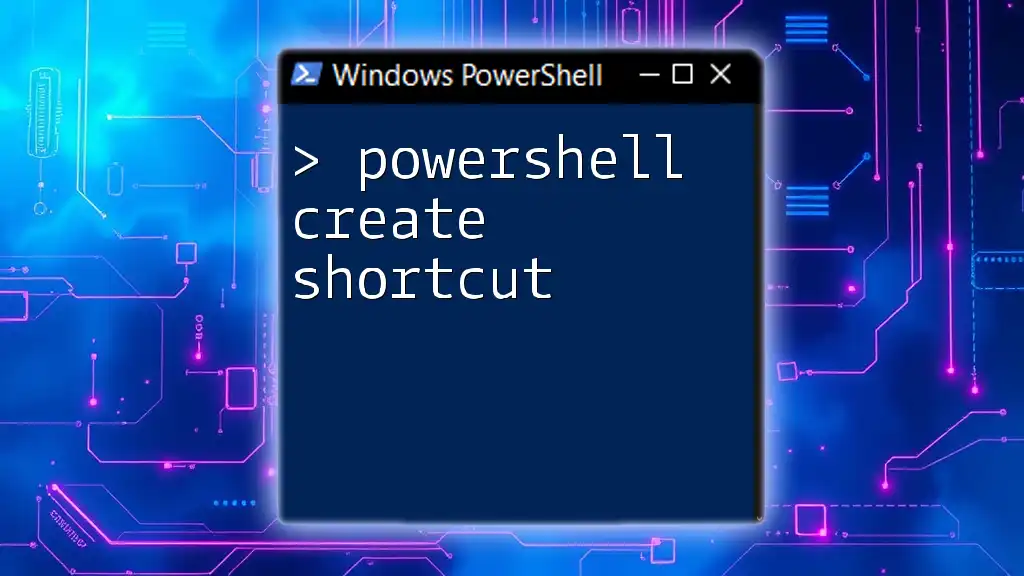
Debugging If Greater Than Conditions
Common Errors with If Statements
Even simple scripts can run into errors, especially when dealing with different data types or incorrect syntax. Common issues include using the wrong operator and neglecting to consider data types, especially when comparing numbers and strings.
Using Write-Host for Debugging
To trace the flow of your scripts and display intermediate values, you can utilize `Write-Host`. This approach is especially beneficial during debugging.
$value = 100
if ($value -gt 50) {
Write-Host "The value is greater than 50."
} else {
Write-Host "The value is 50 or less."
}
Using `Write-Host` this way enables you to see exactly what the script is evaluating at any given moment, helping to troubleshoot any issues that arise.
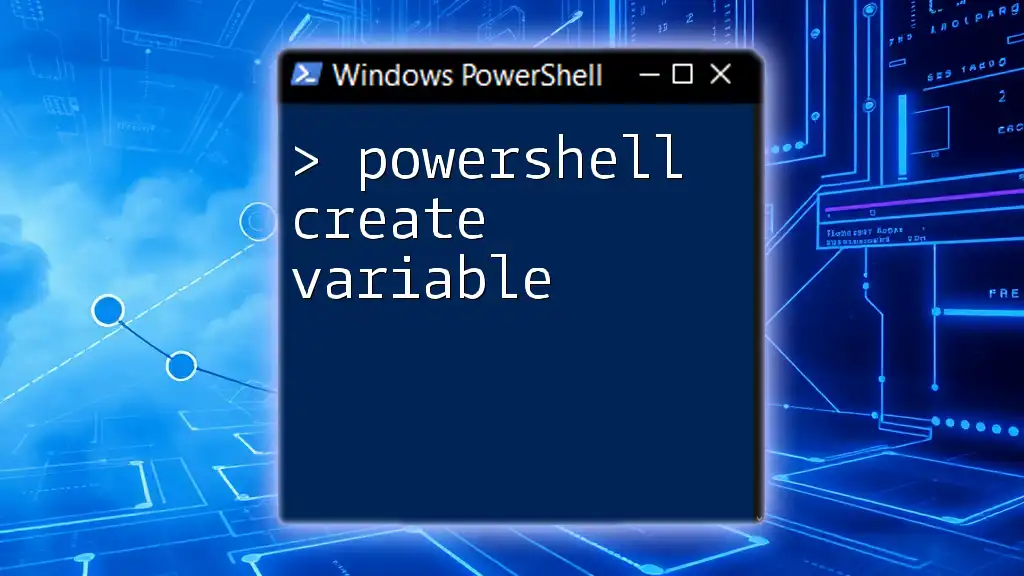
Conclusion
Mastering the concept of `PowerShell if greater than` is crucial for writing effective scripts that control execution flow based on conditional logic. It demonstrates how you can dynamically interact with your environment through comparisons, enhancing the automation capabilities of PowerShell significantly.
By practicing various scenarios and experimenting with different variables in your PowerShell scripts, you'll deepen your understanding of conditional statements and their applications. Remember, the more you practice, the more proficient you'll become at harnessing the full power of PowerShell.