You can easily retrieve the current day of the week in PowerShell by using the `Get-Date` cmdlet with the `-Format` parameter. Here's the code snippet:
(Get-Date).ToString('dddd')
Understanding Days of the Week in PowerShell
The Concept of Days of the Week
When working with PowerShell, understanding the concept of days of the week is fundamental. Each day carries significance in various domains, such as scheduling tasks, generating reports, and processing logs.
Recognizing the day of the week allows you to implement logic based on specific days, such as executing a backup script every Sunday or sending notifications every Monday. Whether you're automating routine tasks or developing complex applications, knowing how to retrieve the day of the week is an essential skill.
The System.DateTime Object
PowerShell leverages the .NET framework, and one of its pivotal components is the `DateTime` object. This object encapsulates information about dates and times, providing a comprehensive mechanism for date manipulation.
Key methods and properties of the `DateTime` object include:
- `DayOfWeek`: This property returns the day of the week as an enumerated value.
- `AddDays()`: Allows you to add or subtract days from a specific date.
- `ToString()`: Converts the `DateTime` object into a string representation, allowing for customized date formatting.
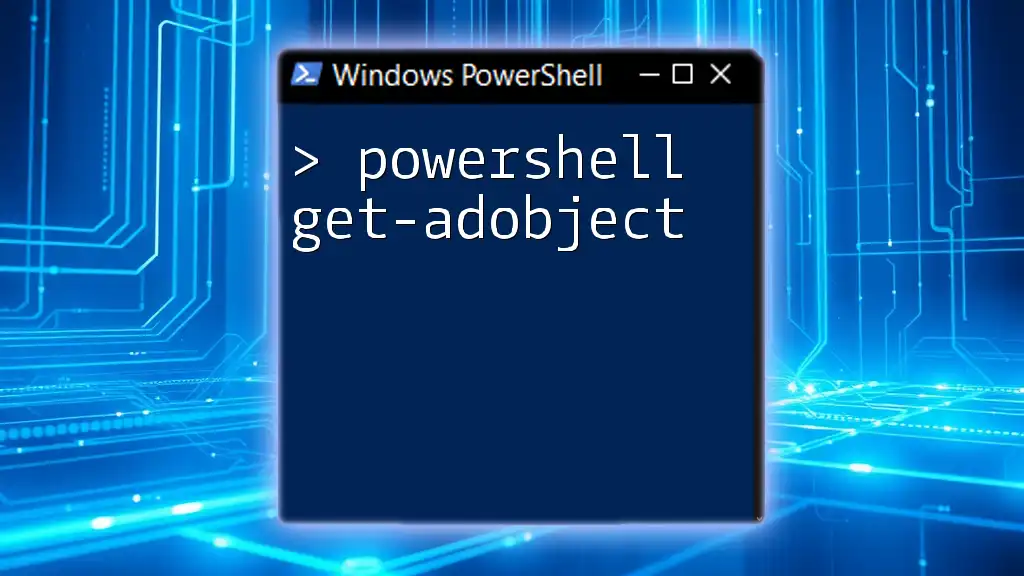
Basic Commands to Get Day of the Week
Using the Get-Date Cmdlet
The `Get-Date` cmdlet is one of the most straightforward methods for retrieving the current date and time in PowerShell. It's an essential tool for fetching date-related information quickly.
Getting the Current Day of the Week
If you want to get the current day of the week, you can use the following command:
Get-Date -Format "dddd"
In this command:
- The `-Format "dddd"` parameter instructs PowerShell to return the full name of the day (e.g., "Monday").
This method allows users to quickly ascertain the current day of the week while scripting or managing tasks.
Getting the Day of the Week for Specific Dates
To check the day of the week for a specific date, you can modify the `Get-Date` cmdlet as follows:
Get-Date -Date "2023-10-01" -Format "dddd"
In this usage:
- The `-Date "2023-10-01"` part provides a specific date, and the command will return the full name of the day corresponding to that date.
By easily adjusting the date string, you can fetch day information for any desired date.
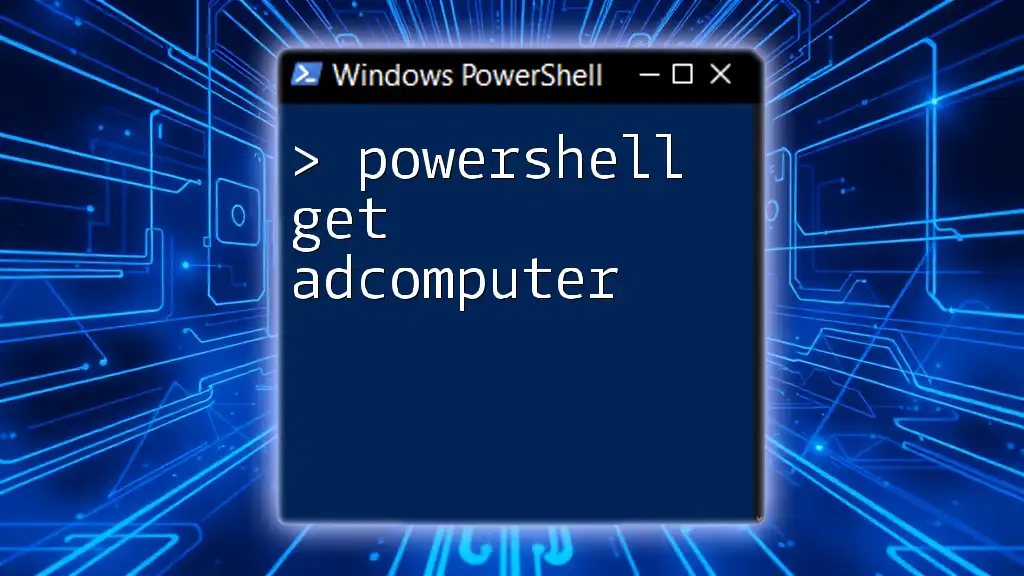
Advanced Techniques to Retrieve Day of the Week
Using Calculated Properties
For more advanced manipulations, PowerShell allows the creation of custom objects using calculated properties. This approach lets you derive new information from existing data.
For example, if you want to find out what the next day will be based on the current date, you could use:
(Get-Date).AddDays(1) | Select-Object @{Name='NextDay'; Expression={($_.DayOfWeek)}}
In this command:
- The `AddDays(1)` method calculates tomorrow’s date, and `Select-Object` creates a custom property named "NextDay" that displays the day of the week.
Working with Arrays for Multiple Dates
When processing multiple dates, PowerShell allows for efficient handling through arrays. Here’s how you could retrieve the day of the week for a list of dates:
$dates = @("2023-10-01", "2023-10-07", "2023-10-14")
$dates | ForEach-Object { Get-Date -Date $_ -Format "dddd" }
In this example:
- An array called `$dates` holds several specified dates.
- The `ForEach-Object` cmdlet iterates through the array, applying the `Get-Date` command to each date, returning the day of the week for each one consistently.
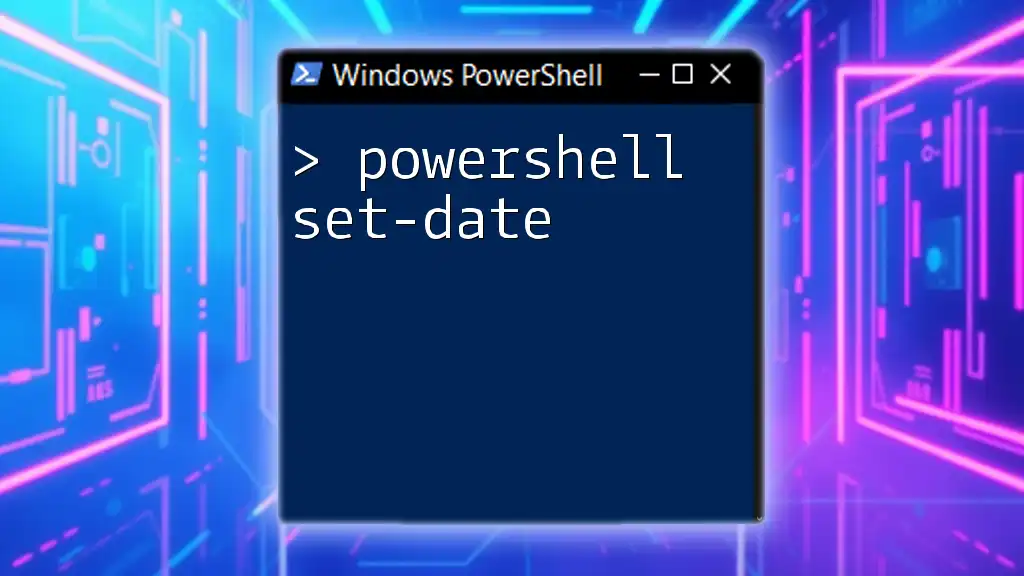
Formatting Options and Customization
Custom Format Strings
PowerShell provides various formatting options for outputting dates. Using custom format strings allows users to tailor the output to their needs.
For instance, you could retrieve the current date in a custom format:
Get-Date -Format "MMMM d, yyyy"
In this command, "MMMM d, yyyy" yields an output like "October 1, 2023," providing complete flexibility in how dates are presented.
Localization and Time Zones
When working in a global environment, handling localization ensures your applications respect regional settings. PowerShell can efficiently retrieve dates while considering different languages or cultures.
To ensure you capture the correct day names in the localized context:
- Utilize the `CultureInfo` class in .NET to set the appropriate culture when formatting dates.
Additionally, PowerShell supports working with time zones, allowing you to retrieve dates considering different regions. This can be accomplished via the `TimeZoneInfo` class.
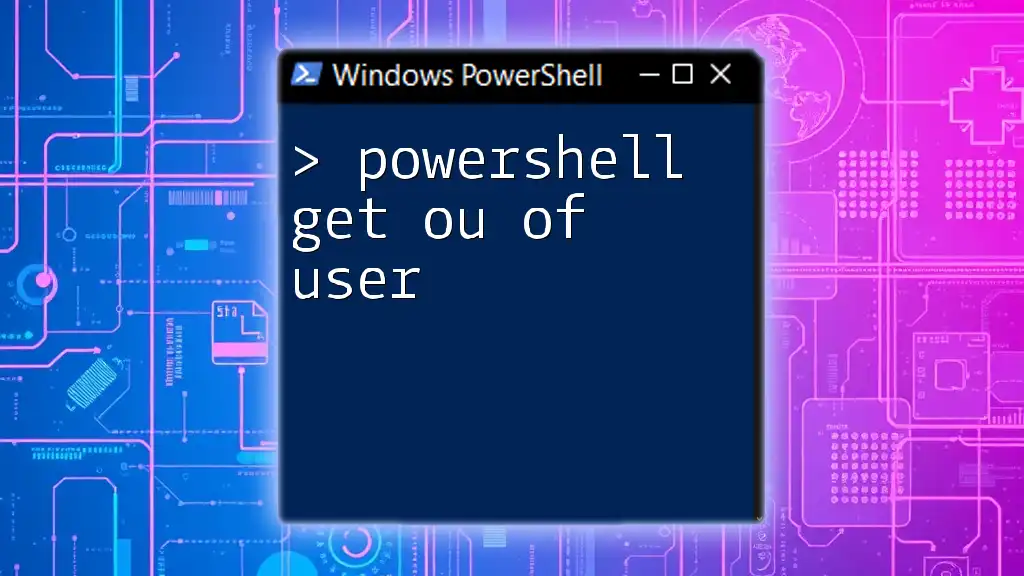
Error Handling and Best Practices
Common Issues with Date Handling
Like any scripting language, working with dates in PowerShell can lead to common pitfalls. One especially frequent issue occurs when entering an invalid date format, leading to errors.
To prevent these issues, it’s best to validate date inputs rigorously. Consider using the following approach:
$dateInput = "2023-10-01"
if (-not [datetime]::TryParse($dateInput, [ref]$null)) {
Write-Host "Invalid date format."
}
This script uses the `TryParse` method to assure that only valid date inputs proceed through your logic.
Best Practices
Best practices when working with PowerShell for date manipulation include:
- Always validate your date inputs.
- Use `try-catch` blocks to handle unexpected errors gracefully.
- Document your scripts to clarify the purpose of each date-related command.
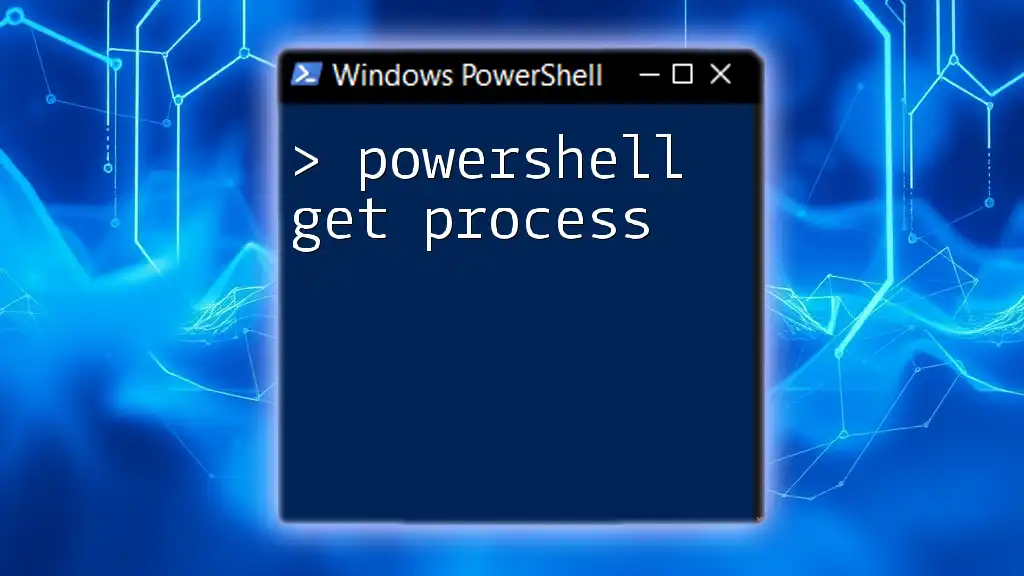
Conclusion
In this guide, we delved into the techniques of using PowerShell to get the day of the week, starting from the fundamental commands to advanced techniques. PowerShell's capabilities transform date handling into a powerful ally for automation and scripting tasks.
For those eager to delve deeper, practice implementing these commands in real-world scenarios and explore more advanced PowerShell functionalities to enhance your scripting repertoire.
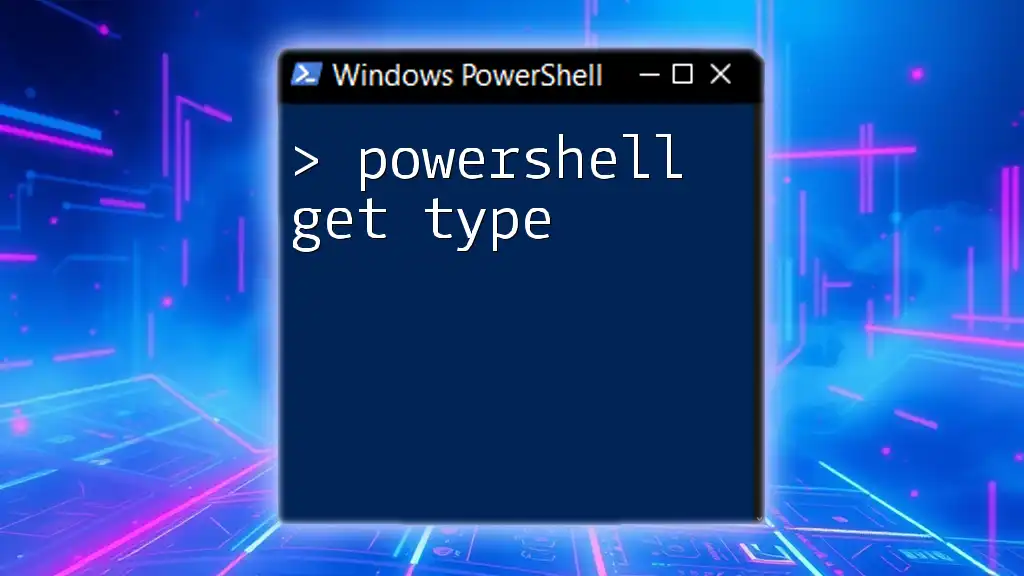
Additional Resources
For further information, refer to the [Microsoft PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) and explore community forums such as PowerShell.org for support and guidance from fellow enthusiasts.
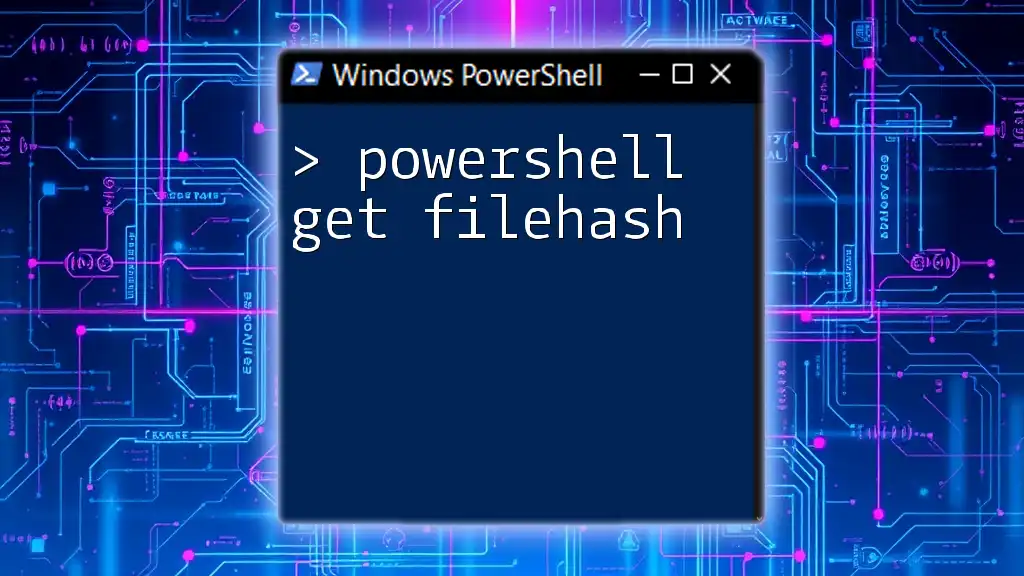
FAQs
You might encounter some common questions as you learn about managing days of the week with PowerShell. For example:
- Can I get the day of the week in different languages? Yes, utilizing the `CultureInfo` class allows you to specify different cultures for retrieving localized day names.
With this knowledge, you are well-equipped to utilize PowerShell for date manipulations, making your scripts more robust and versatile.