The `-ceq` operator in PowerShell is a case-sensitive equality comparison, used to determine if two values are identical while respecting their case.
# Example of using -ceq
if ('Hello' -ceq 'hello') {
Write-Host 'They are equal.'
} else {
Write-Host 'They are not equal.'
}
Understanding the -ceq Operator
What is -ceq?
The `-ceq` operator in PowerShell stands for "case-sensitive equality." It is a comparison operator used to evaluate whether two values are equal, taking into account the letter cases of each character. Unlike its case-insensitive counterpart `-eq`, the `-ceq` operator matters if the strings being compared have different cases.
Why Use -ceq?
Using the `-ceq` operator is essential in scenarios where case sensitivity plays a critical role. For instance, when validating usernames or passwords in a secure environment, it is vital to ensure that the exact cases are matched. Failing to do so may pose security risks or lead to input errors that can be costly or time-consuming to troubleshoot.
When deciding whether to use `-ceq` or `-eq`, consider:
- Strict Equality: Use `-ceq` when dealing with cases where letter casing is significant, such as programming identifiers or environment variables.
- Common Application Scenarios: Situations such as checking configurations or user credentials are perfect candidates for `-ceq`.

Syntax and Usage
Basic Syntax
The general syntax for using the `-ceq` operator is straightforward:
<value1> -ceq <value2>
This structure allows for the direct comparison of two values, returning `$true` if they are the same (case-sensitive) or `$false` otherwise.
Code Examples
Example 1: Basic Case-Sensitive Comparison
Here’s a simple comparison demonstrating how `-ceq` differentiates between different cases in strings:
$Value1 = "Hello"
$Value2 = "hello"
$result = $Value1 -ceq $Value2
Write-Output $result # Output: False
In this example, the output is `False` because the cases of the letters in `Value1` and `Value2` do not match.
Example 2: Case-Sensitive Comparison in Strings
To highlight how `-ceq` works effectively, consider this example:
$Value1 = "PowerShell"
$Value2 = "PowerShell"
$result = $Value1 -ceq $Value2
Write-Output $result # Output: True
Here, the two strings are identical in both case and content, resulting in a `True` output.
Common Mistakes
One common mistake when using `-ceq` is failing to recognize the importance of data types and cases. For example, comparing an integer with a string using `-ceq` will not yield the expected results. Understanding the types being used is crucial to avoid unexpected issues.

Real World Scenarios for Using -ceq
Comparing User Input
A typical use case for `-ceq` is in validating credentials. Consider the following scenario:
$inputUser = Read-Host "Enter your username"
$actualUser = "Admin"
if ($inputUser -ceq $actualUser) {
Write-Output "Access granted"
} else {
Write-Output "Access denied"
}
In this script, if the user enters `admin` instead of `Admin`, access will be denied due to the case difference, showcasing the importance of case-sensitive checks in security protocols.
Filtering Data in Arrays
Another practical application of `-ceq` is when filtering data in arrays. Consider this example:
$array = @("apple", "Orange", "banana")
$filteredArray = $array | Where-Object { $_ -ceq "apple" }
Write-Output $filteredArray # Output: apple
In this instance, the script filters the array to find only exact matches, ensuring that any variations in case do not yield false positives or unexpected results.

Best Practices
Using -ceq with Variables
When working with `-ceq`, always make sure variables are initialized correctly. It's also wise to check for `$null` values to prevent runtime errors during comparisons. For example:
if ($null -ceq $Value1) {
Write-Output "Value is null"
}
Combining with Other Operators
The `-ceq` operator can be combined with logical operators to create more complex conditions. For instance:
$value1 = "Test"
$value2 = "Test"
$value3 = "Check"
if ($value1 -ceq $value2 -or $value3 -ceq "Check") {
Write-Output "Conditions met"
}
This example checks if at least one of the conditions is met, demonstrating how to effectively use `-ceq` in conjunction with other logical constructs.
Performance Considerations
When using the `-ceq` operator, there are performance considerations to bear in mind. Using case-sensitive comparisons is usually more efficient in terms of execution time, particularly for large datasets or complex scripts where precision in comparison is paramount. However, it is worth weighing the trade-offs between performance and the necessity for case sensitivity in your particular application.
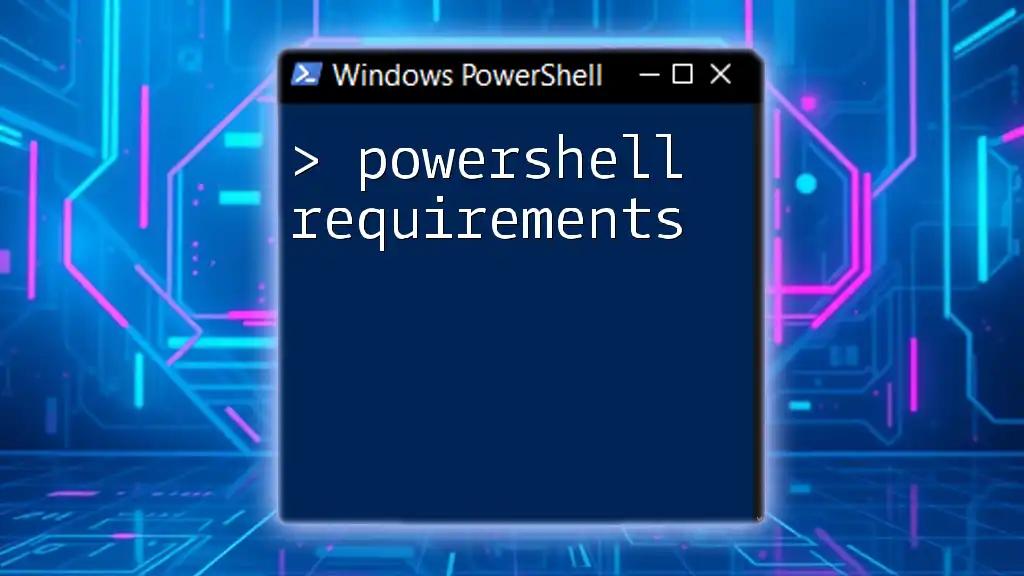
Conclusion
In summary, the `powershell -ceq` operator is a powerful tool for performing case-sensitive comparisons in PowerShell. It is vital for scenarios where letter casing is significant, particularly in areas related to security and data filtering. By understanding and implementing `-ceq` correctly, you can enhance the reliability and accuracy of your PowerShell scripts.
Encouraging continual practice with this operator will allow developers to utilize PowerShell's full capabilities, ensuring that comparisons yield the desired outcomes. The more familiar you become with `-ceq`, the more effectively you'll be able to streamline your scripting endeavors.
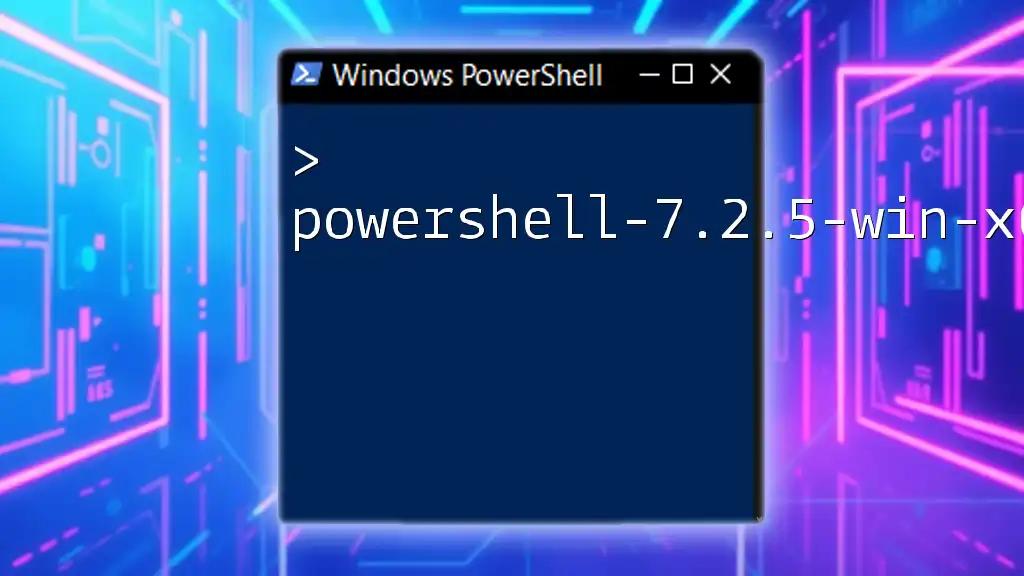
Additional Resources
For those eager to learn more, refer to the official Microsoft documentation on PowerShell operators, explore specialized PowerShell books, and join online forums to enrich your learning and discuss best practices with fellow enthusiasts.

FAQs
-
What happens if I use -ceq with different data types? Using `-ceq` with different data types, like integer and string, will result in a `False` output, as PowerShell does not coerce data types in this comparison.
-
Does -ceq work with objects? Yes, `-ceq` can be used with objects, but be mindful of the properties being compared and ensure they are of the same type and case.