To ping a range of IP addresses in PowerShell, you can use a simple loop that iterates over the desired range and sends a ping request to each address. Here's a code snippet to accomplish this:
$ipRange = 1..254; foreach ($i in $ipRange) { Test-Connection -ComputerName "192.168.1.$i" -Count 1 -ErrorAction SilentlyContinue }
Understanding the `Test-Connection` Cmdlet
What is `Test-Connection`?
The `Test-Connection` cmdlet is a built-in PowerShell command that serves as an alternative to the traditional ping command. It allows you to send ICMP echo request packets to one or more network hosts and receive echo response packets in return. This cmdlet is critical for network diagnostics, enabling users to check the reachability of hosts and measure round-trip times for packets.
Benefits of Using `Test-Connection`
Using `Test-Connection` comes with several benefits over the conventional ping command:
- Robustness: It’s designed to be more flexible, offering options for more detailed interactions with network devices.
- Scripting Ability: Being part of PowerShell, it can easily be integrated into scripts to automate network testing, making it invaluable for network administrators and IT professionals.
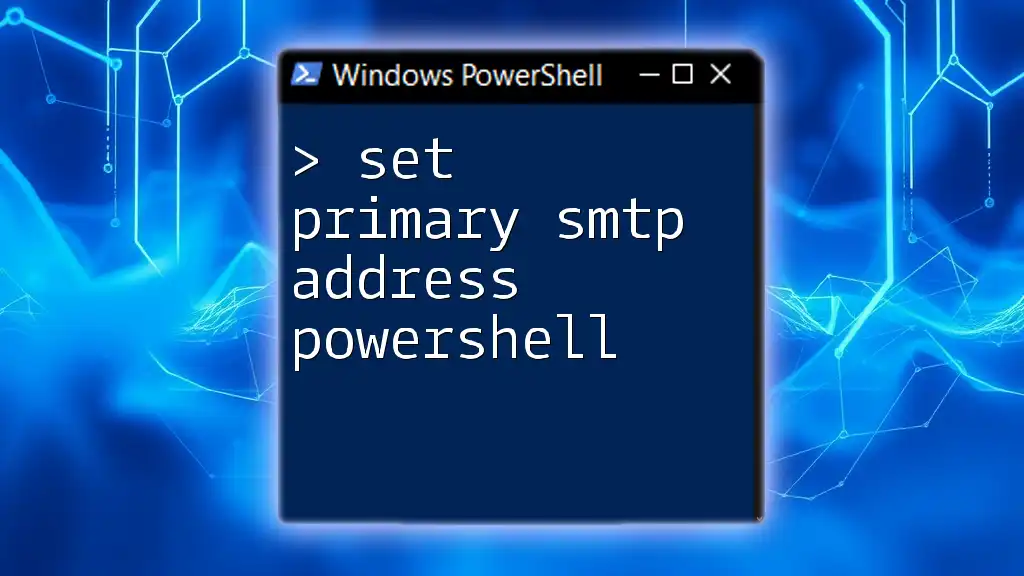
Preparing to Ping a Range of IP Addresses
What is a Range of IP Addresses?
An IP address range refers to a sequence of consecutive IP addresses, often defined for a subnet. For instance, you might have a range such as `192.168.1.1` to `192.168.1.255`. When diagnosing network connectivity, pinging a range of IPs allows you to efficiently ascertain which devices are online and responsive.
Setting Up PowerShell
Before you start using PowerShell, ensure it’s set up correctly on your Windows machine. To open PowerShell, you can search for it in the Start Menu. If you plan to run scripts that you or others create, you may need to enable script execution by typing the following command:
Set-ExecutionPolicy RemoteSigned
This command allows you to run your own scripts while still retaining security by requiring scripts downloaded from the internet to be signed by a trusted publisher.
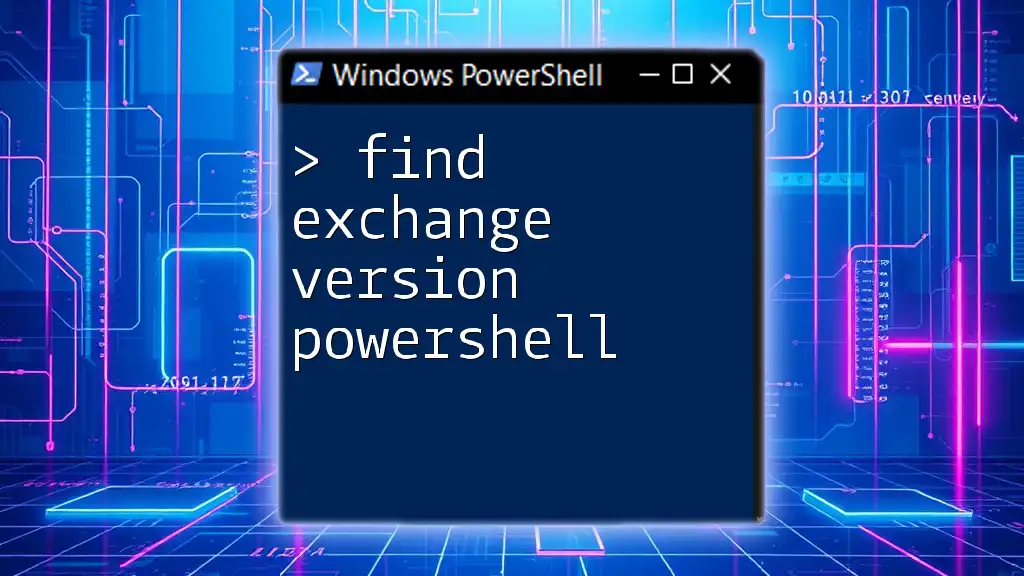
Pinging a Simple Range of IP Addresses
Constructing the Basic Script
To ping a range of IP addresses in PowerShell, you can build a straightforward script that iterates through the desired range. Here is an example that demonstrates how to accomplish this:
$startIP = [System.Net.IPAddress]::Parse("192.168.1.1")
$endIP = [System.Net.IPAddress]::Parse("192.168.1.254")
$startBytes = $startIP.GetAddressBytes()
$endBytes = $endIP.GetAddressBytes()
for ($i = $startBytes[3]; $i -le $endBytes[3]; $i++) {
$ip = "$($startBytes[0]).$($startBytes[1]).$($startBytes[2]).$i"
Test-Connection -ComputerName $ip -Count 2 -ErrorAction SilentlyContinue |
Select-Object Address, ResponseTime, StatusCode |
Format-Table -AutoSize
}
Explanation of the Code
Let’s break down the script:
-
IP Address Setup: The `$startIP` and `$endIP` variables define the range of IP addresses. The `Parse` method converts string representations of the IP addresses into objects that PowerShell can manipulate.
-
Extracting Bytes: The `GetAddressBytes()` method retrieves the individual octets of the IP addresses, making it easier to work with the last octet (which is commonly sequentially assigned in local networks).
-
Iterating Through the Range: The `for` loop iterates through the last octet, assembling the full IP address for each iteration (e.g., 192.168.1.1, 192.168.1.2, etc.).
-
Executing the Ping: The `Test-Connection` cmdlet is invoked for each IP address, sending out two echo requests. The results are formatted into a readable table displaying the address, response time, and status code.
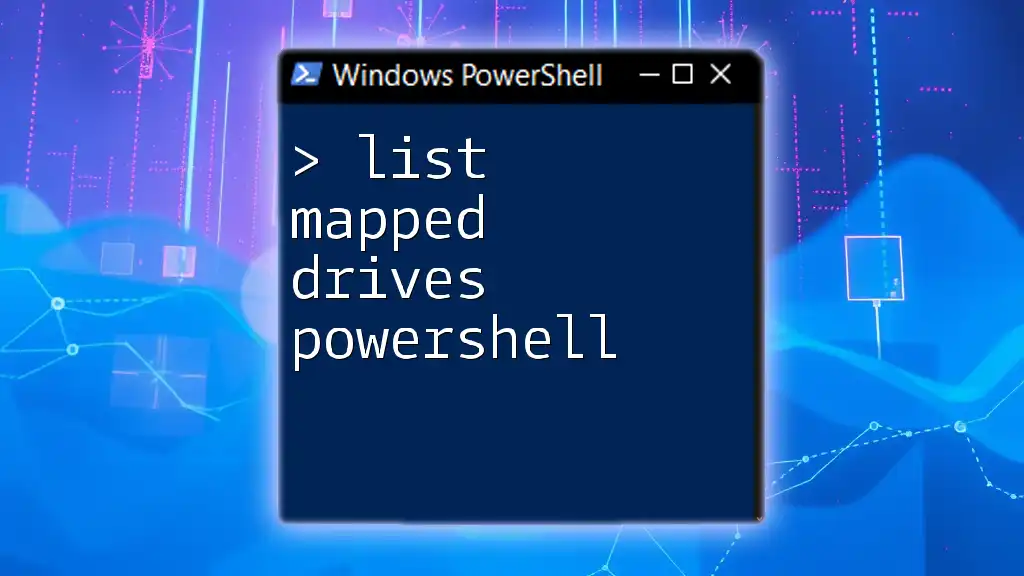
Advanced Pinging Techniques
Adding Error Handling
In real network environments, issues like timeouts or unreachable hosts are common. Handling these errors gracefully increases the robustness of your script. Here’s how you can incorporate error handling:
if (-not (Test-Connection -ComputerName $ip -Count 1 -ErrorAction SilentlyContinue)) {
Write-Host "Host $ip is unreachable." -ForegroundColor Red
}
In this additional snippet, we check if the host is reachable before attempting to collect response time, allowing you to output a clear message for hosts that are unresponsive.
Outputting Results to a CSV File
Documenting the results is essential for analyzing network conditions over time. You can easily output your results to a CSV file:
$result = @()
for ($i = $startBytes[3]; $i -le $endBytes[3]; $i++) {
$ip = "$($startBytes[0]).$($startBytes[1]).$($startBytes[2]).$i"
$ping = Test-Connection -ComputerName $ip -Count 2 -ErrorAction SilentlyContinue
if ($ping) {
$result += [PSCustomObject]@{ IPAddress = $ping.Address; ResponseTime = $ping.ResponseTime; Status = 'Online' }
} else {
$result += [PSCustomObject]@{ IPAddress = $ip; ResponseTime = 'N/A'; Status = 'Offline' }
}
}
$result | Export-Csv -Path "PingResults.csv" -NoTypeInformation
Scheduling the Script
For continuous network monitoring, consider automating the process. Windows Task Scheduler allows you to run your PowerShell scripts at defined intervals. This is particularly useful if you need ongoing diagnostics without manual intervention.
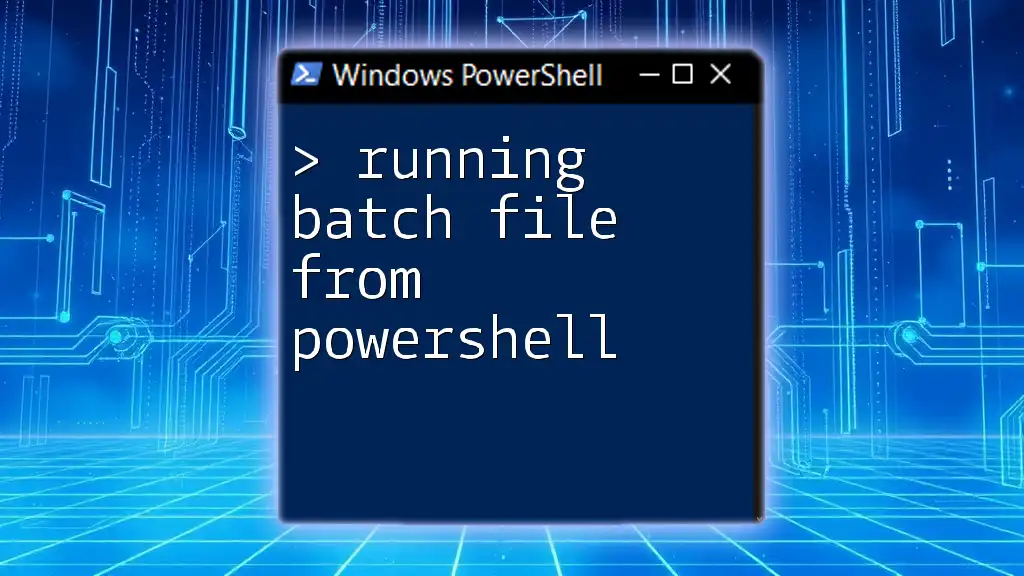
Troubleshooting Common Issues
Identifying Issues with Network Connectivity
Understanding network connectivity is crucial. If you receive a response from `Test-Connection`, it indicates the target is reachable; alternatively, timeouts or lost packets suggest connectivity problems. Use this information to drill down into specific issues with your network configuration.
Script Not Executing as Expected
If your script fails to run correctly, check for common issues such as incorrect permissions, syntax errors, or script execution policies. Always verify your PowerShell version is up to date, as syntax and cmdlet behavior can change between versions.
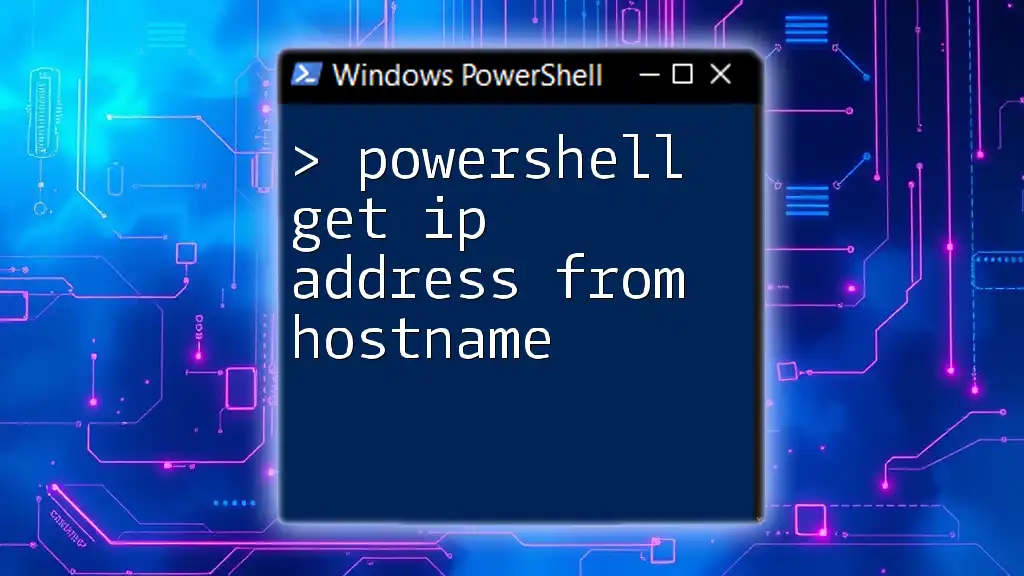
Conclusion
Utilizing PowerShell to ping a range of IP addresses is a powerful technique for network management and troubleshooting. By learning how to effectively use the `Test-Connection` cmdlet and incorporating advanced techniques like error handling and result logging, you can streamline your network diagnostics.
As you experiment and tailor these scripts to meet your needs, you’ll gain valuable insights into the health of your network environment. Feel free to share your experiences with PowerShell and let others know how you’ve benefited from its capabilities.
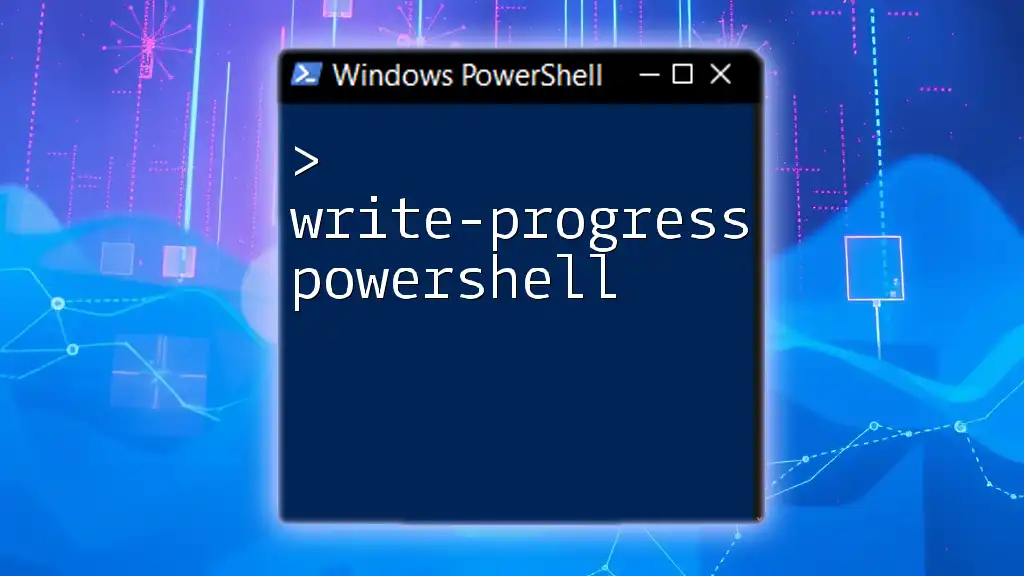
Additional Resources
For further learning, consider visiting the official Microsoft documentation on PowerShell and the `Test-Connection` cmdlet. Various books and online resources can deepen your understanding and proficiency with PowerShell, allowing you to harness its full potential in your networking tasks.