You can install software using PowerShell by utilizing the `Install-Package` cmdlet from the PackageManagement module, allowing for streamlined software installation directly from the command line. Here's a code snippet demonstrating how to install a package:
Install-Package -Name 'packageName' -Source 'sourceName' -Force
Replace `packageName` with the name of the software you wish to install and `sourceName` with the appropriate source for the package.
Benefits of Installing Software with PowerShell
Streamlined Processes
Using PowerShell to install software offers a significant advantage in streamlining processes. The ability to write scripts allows you to automate repetitive tasks related to software installation. This automation minimizes the potential for human error, ensuring that installations are executed consistently and accurately. When you write a PowerShell script, you can define all the steps needed for installation in a clear, repeatable manner, making it easier to install software multiple times or on numerous machines without manual intervention.
Remote Management
PowerShell shines in its ability to manage multiple systems from a single console. This capability is particularly beneficial for IT administrators and DevOps professionals who need to manage software installations across a network. With PowerShell, you can run commands remotely, allowing you to install software on client machines without having to physically touch each one. This capability saves time and resources, especially in large organizations where efficiency is key.
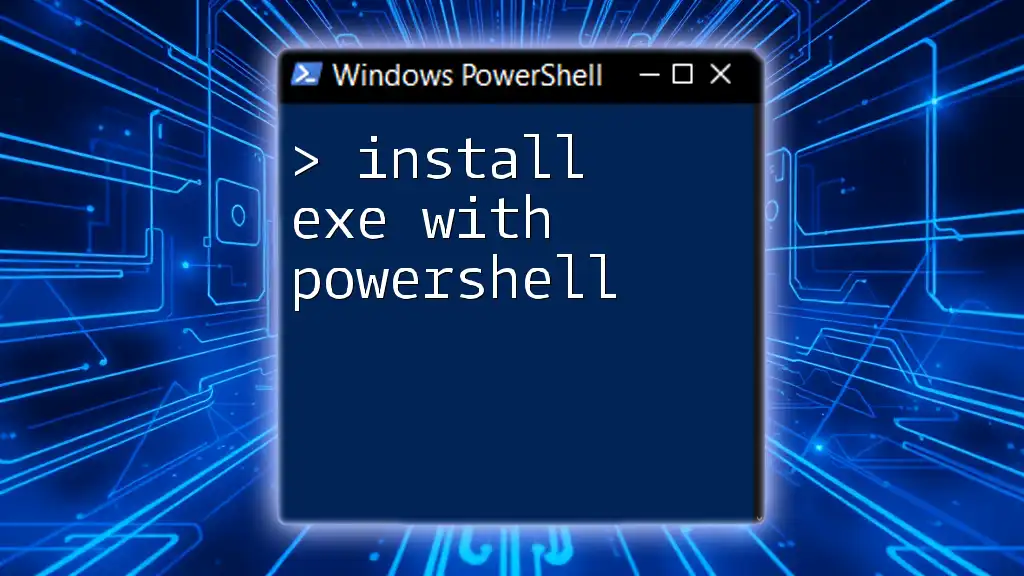
Prerequisites for Installing Software with PowerShell
PowerShell Version
Before diving into software installation, it’s important to verify that you are using an appropriate version of PowerShell. Different versions have varying features and capabilities, and some might not support certain commands or packages. You can check your PowerShell version by running the following command:
$PSVersionTable.PSVersion
Administrative Privileges
Most software installations require administrative access. It’s crucial to run PowerShell with elevated permissions to avoid permission-related errors. You can easily run PowerShell as an administrator by right-clicking the PowerShell icon and selecting "Run as administrator." This will ensure you have the necessary permissions to install software effectively.
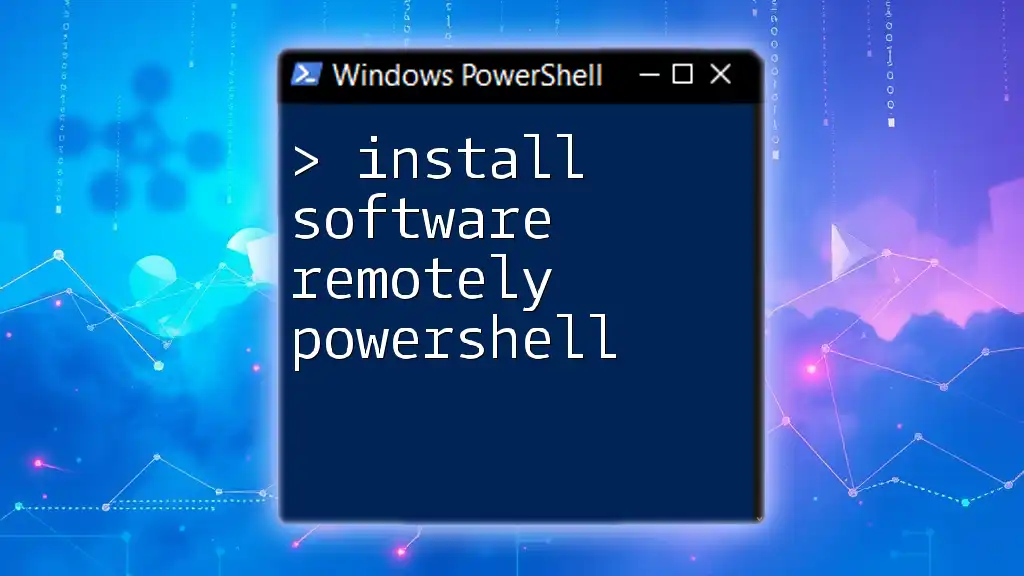
Methods to Install Software Using PowerShell
Using Windows Package Manager (winget)
Introduction to winget
The Windows Package Manager, also known as winget, is a powerful tool that simplifies the process of installing software on Windows systems. It enables you to automate the installation and updating of applications directly from the command line.
Installation of winget
If you don’t already have winget installed on your system, you can set it up quickly using the following command:
Add-AppxPackage -Path "C:\Path\To\Microsoft.DesktopAppInstaller_XXXXX.appxbundle"
Make sure to replace `C:\Path\To\Microsoft.DesktopAppInstaller_XXXXX.appxbundle` with the actual path where the installer is located.
Finding and Installing Software
Once winget is installed, you can easily search for available software. For example, to install Mozilla Firefox, you can execute:
winget install Mozilla.Firefox
This command retrieves the latest version of Mozilla Firefox and initiates the installation process.
Updating Software
Keeping your software up to date is essential. With winget, updating installed software is straightforward. Use the command:
winget upgrade Mozilla.Firefox
This will check for updates to Firefox and install them if available.
Using Chocolatey
Overview of Chocolatey
Chocolatey is another popular package manager for Windows that integrates seamlessly with PowerShell. It provides a simple way to install and manage software packages, streamlining the installation process greatly.
Installation of Chocolatey
To use Chocolatey, you must first install it. You can do this by running the following command in an elevated PowerShell session:
Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.SecurityProtocolType]::Tls12; iex ((New-Object System.Net.WebClient).DownloadString('https://chocolatey.org/install.ps1'))
This command downloads and runs the Chocolatey installation script, setting the execution policy temporarily to allow the script to run.
Installing Software with Chocolatey
After installing Chocolatey, you can install software packages easily. For example, to install Google Chrome, simply run:
choco install googlechrome
This command downloads and installs the latest version of Google Chrome automatically, simplifying the process compared to manual installation.
List Installed Packages
To see which packages you have installed via Chocolatey, use:
choco list --local-only
This command will display a list of installed packages, allowing you to manage your software effectively.
Using PowerShell Scripts
Creating a Simple Script for Software Installation
Using scripts for software installation can further enhance automation. Here’s an example of a basic PowerShell script for installing software:
# InstallSoftware.ps1
param (
[string]$SoftwareName
)
Start-Process "winget" -ArgumentList "install $SoftwareName" -Wait
In this script, the `param` block allows you to input the name of the software you wish to install. The `Start-Process` command runs the installation command, while the `-Wait` parameter ensures that execution waits until the installation finishes.
Scheduling Installation Scripts
By using Task Scheduler, you can automate your installation scripts to run at specific times or under certain conditions. This is particularly useful for maintaining software across multiple workstations in a network.
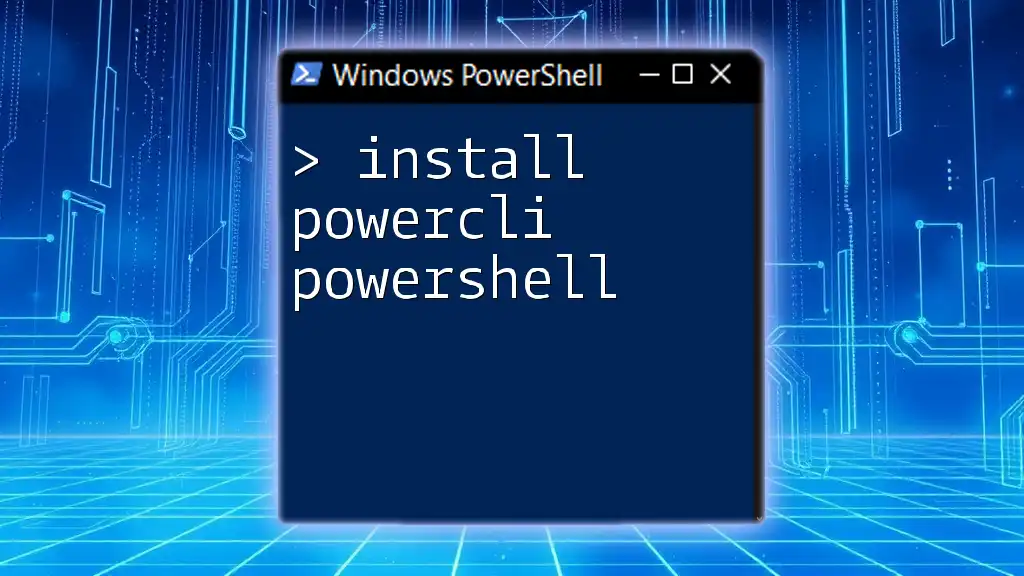
Handling Software Dependencies
Why Dependencies Matter
Software dependencies refer to packages or components that a program needs to function correctly. Tracking these dependencies is crucial; if they’re missing or misconfigured, it can lead to installation failures or runtime errors.
Utilizing PowerShell to Manage Dependencies
When installing software through Chocolatey, you can handle dependencies effectively. For example, to ensure that all dependencies for a package are installed, you can use:
choco install [package] --params "/Dependency"
This ensures that any required dependencies are included during the installation process, streamlining the setup.
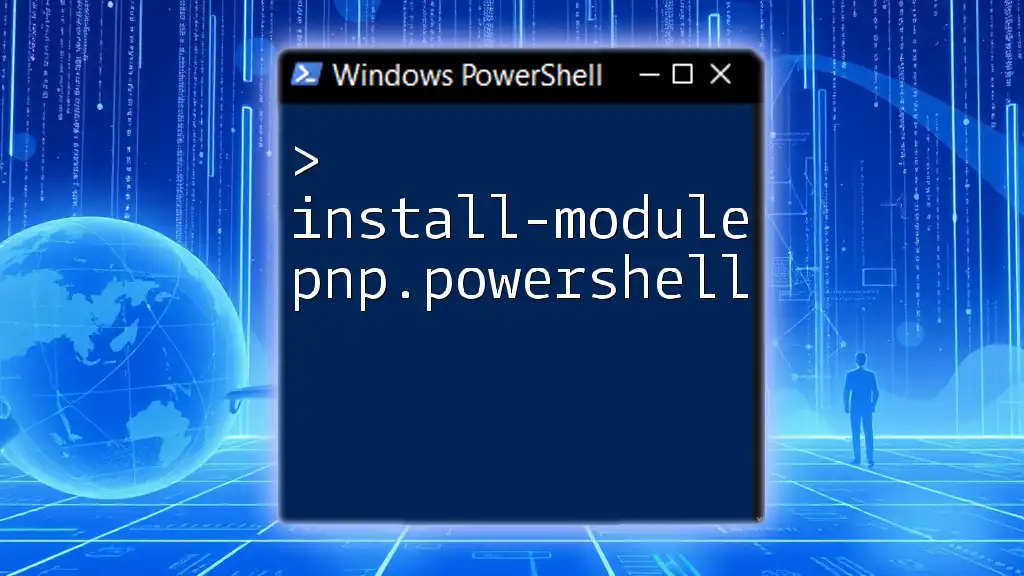
Troubleshooting Common Installation Errors
Permission Issues
If you encounter permission-related issues during installation, it’s typically due to the lack of administrative rights. Always ensure you are running PowerShell as an administrator to avoid these errors.
Command Not Found Errors
Mistyped commands or missing packages can result in "command not found" errors. Double-check your command syntax and ensure that the package you are attempting to install is available in your chosen package manager.
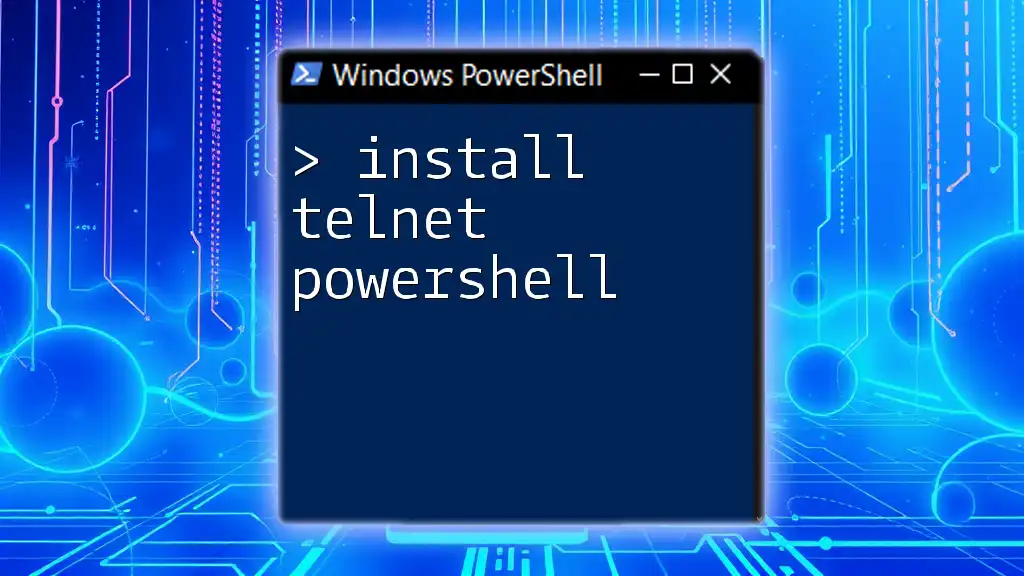
Conclusion
Installing software with PowerShell provides an efficient, effective, and powerful way to manage applications on Windows systems. By leveraging tools like winget and Chocolatey, along with the capabilities of PowerShell scripts, you can automate installations, troubleshoot issues, and streamline your software management processes.
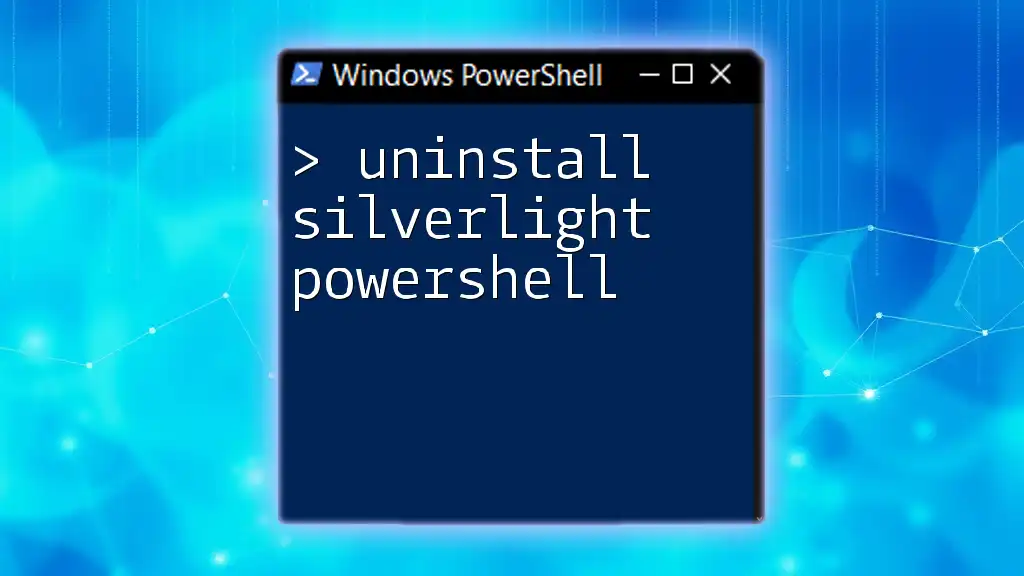
Call to Action
As you delve deeper into using PowerShell for software installation, don’t hesitate to subscribe for more detailed PowerShell tutorials. Share your own PowerShell success stories in the comments below, and let’s learn together!
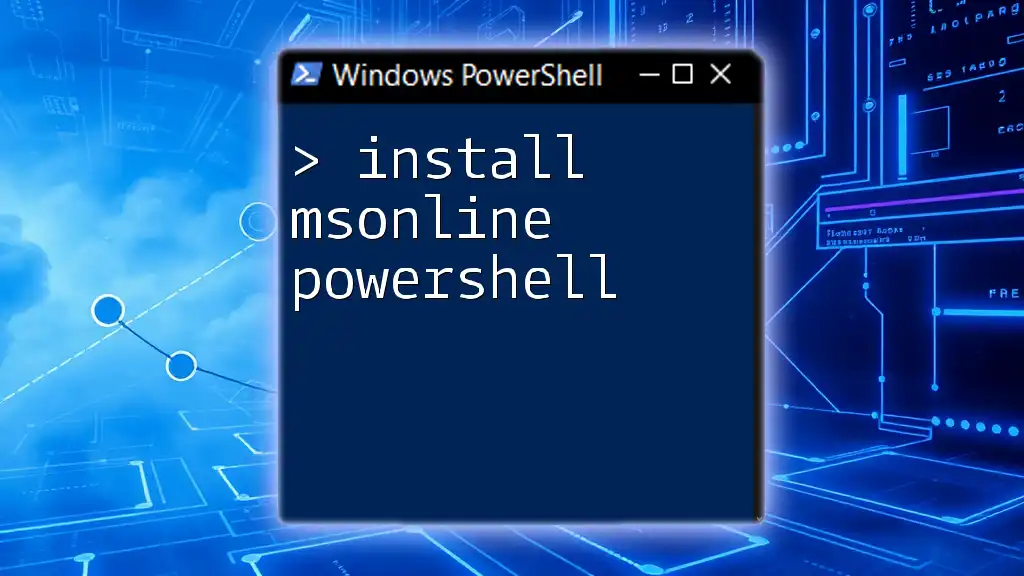
Additional Resources
For further reading, visit the official PowerShell documentation and explore tutorials on advanced PowerShell scripting techniques.