In PowerShell, you can retrieve the properties of an object using the `Get-Member` cmdlet, which lists all the members (properties and methods) associated with the object.
Here’s a code snippet to demonstrate this:
$object = Get-Process -Name "notepad"
$object | Get-Member
Understanding PowerShell Objects
What is a PowerShell Object?
In PowerShell, everything is treated as an object. An object is an instance of a class that contains both data and methods. Understanding objects is crucial for effective scripting, as they come with properties that hold valuable information. Common types of objects include strings, arrays, files, processes, and services, each with its unique set of properties.
The Role of Object Properties
Properties are characteristics of an object that provide details about its state or identity. For example, a file object can have properties such as `Name`, `Size`, and `CreationTime`. Understanding the difference between methods (functions associated with the object) and properties is essential for navigating and manipulating these objects effectively.
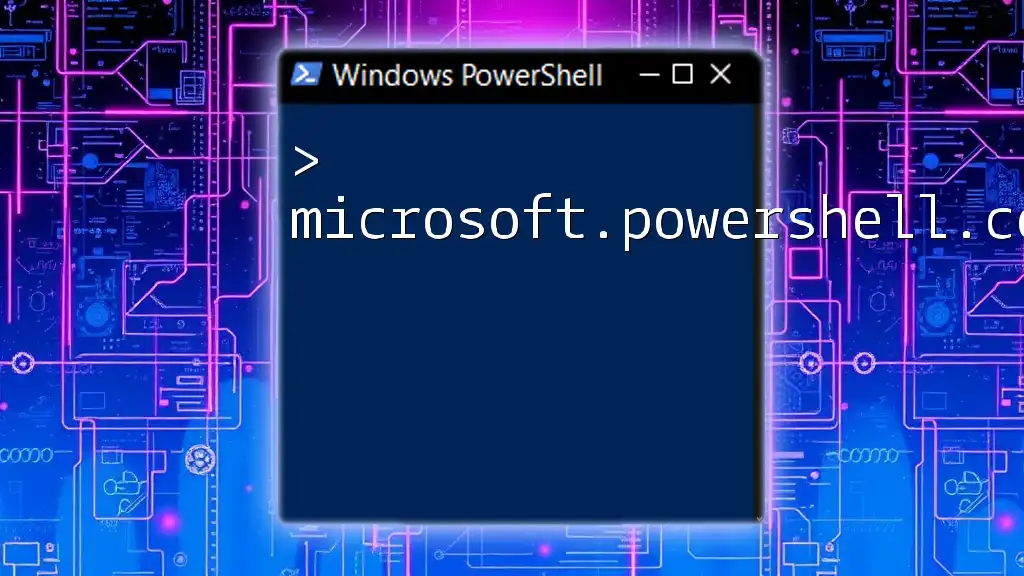
How to Get Object Properties in PowerShell
PowerShell Get Properties of Object Command
To access the properties of an object in PowerShell, one of the fundamental commands you will use is `Get-Member`. This cmdlet displays the properties and methods of PowerShell objects, allowing you to see what data is available for manipulation.
Example:
Get-Process | Get-Member
This command retrieves a list of all processes running on your system and outputs their associated properties and methods. The output makes it easy to spot relevant properties such as `Id`, `Name`, and `CPU`.
Using `Get-Item` to View Object Properties
When working with file system objects, the `Get-Item` cmdlet is invaluable. This cmdlet retrieves the specified item (like files or folders) and allows you to explore its properties.
Example:
Get-Item -Path "C:\example.txt" | Get-Member
This command returns the properties and methods of the file located at `C:\example.txt`. Understanding these properties enables you to manipulate the file effectively.
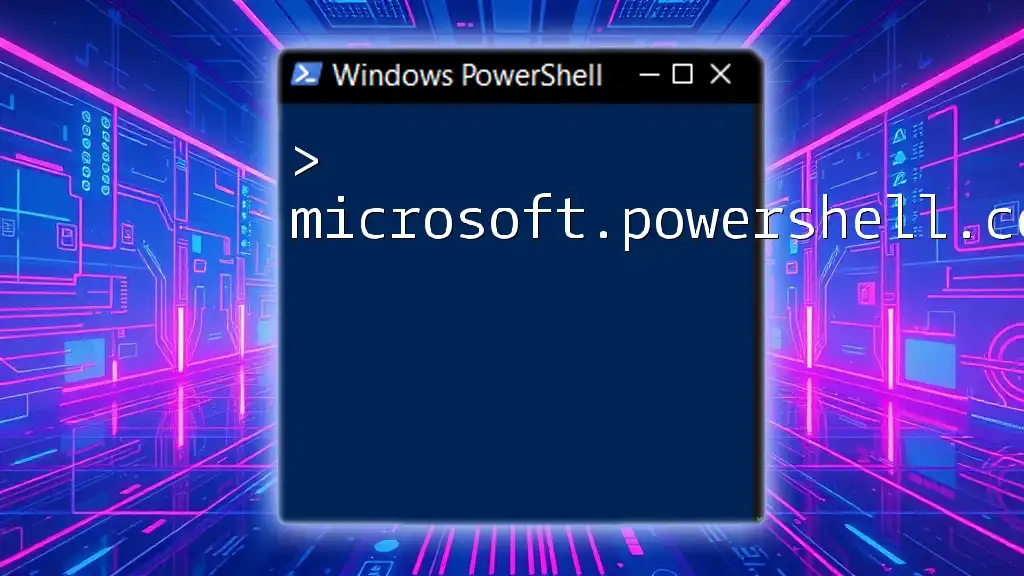
Viewing Object Properties with `Select-Object`
Filtering Properties
To improve readability and focus on specific data points, you can use the `Select-Object` cmdlet. This cmdlet allows you to filter the properties of an object to display only the information you need.
Example:
Get-Service | Select-Object DisplayName, Status
In this command, you retrieve a list of all services on the system, showing only the `DisplayName` and `Status` properties. This method helps to declutter the output, displaying only relevant information.
Customizing Output
You can further customize the output of `Select-Object` by creating calculated properties. This feature allows you to add your transformed data into the output based on existing properties.
Example:
Get-Process | Select-Object @{Name="ProcessName"; Expression={$_.Name}}, @{Name="MemoryUsage"; Expression={($_.WorkingSet/1MB) -as [int]}}
In this example, we are displaying the `ProcessName` and a calculated `MemoryUsage`. By converting the `WorkingSet` property from bytes to megabytes, we provide a more human-readable format of memory usage.
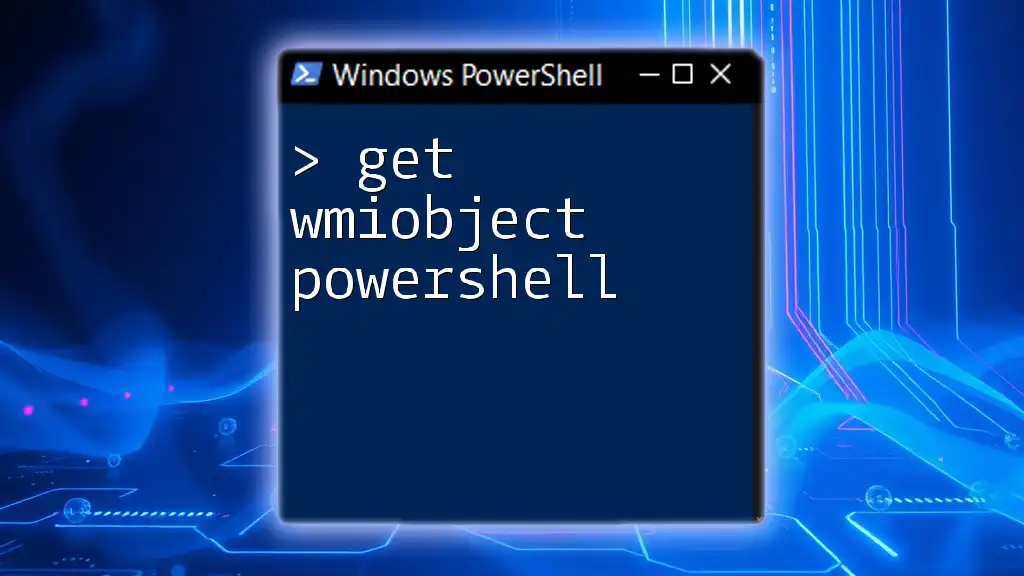
Getting Property Values from PowerShell Objects
Using the Dot Notation
To access specific property values directly, you can use the dot notation. This allows you to retrieve a single property from an object without additional filtering.
Example:
$process = Get-Process -Name "explorer"
$process.Id
Here, we retrieve the `Id` property of the `explorer` process. Understanding dot notation is crucial as it lets you pinpoint and manipulate individual properties easily.
The `Where-Object` Method
The `Where-Object` cmdlet enables you to filter objects based on specific conditions, accessing properties dynamically.
Example:
Get-Service | Where-Object { $_.Status -eq 'Running' }
This command retrieves all services that are currently running. The `$_` symbol refers to the current object in the pipeline, allowing you to access its properties directly within the filtering expression.
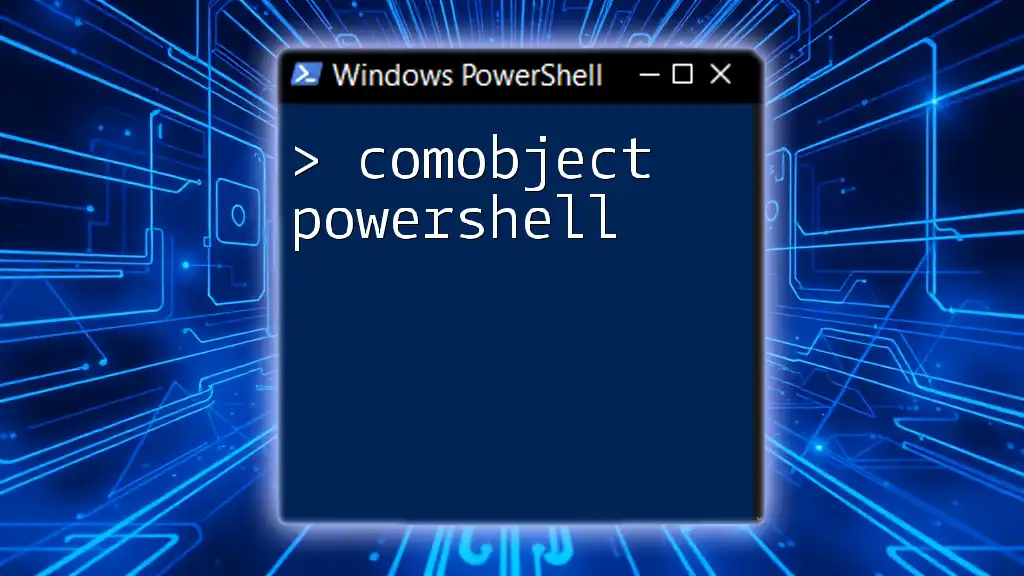
Advanced Techniques
Exploring Object Properties with `Get-Property`
For users of PowerShell v7 and above, the `Get-Property` feature offers enhanced ways to explore the properties of objects.
Example:
[System.IO.FileInfo] | Get-Property
This showcases how to dynamically access the available properties of the `FileInfo` class, providing insights into file-specific attributes.
Importing Custom Objects
Creating your custom objects in PowerShell allows you to define properties that are relevant to your scripting tasks.
Example:
$customObject = New-Object PSObject -Property @{
Name = "Sample"
Value = 123
}
$customObject | Get-Member
In this example, we define a custom object with properties `Name` and `Value`. The `Get-Member` command will show you the properties of this object, demonstrating the flexibility of PowerShell.
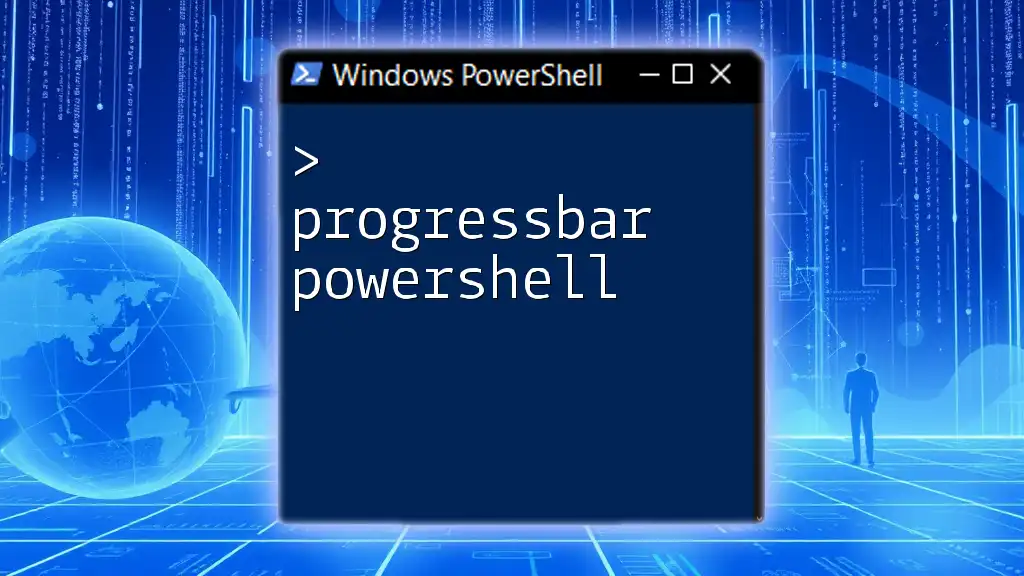
Troubleshooting Object Property Issues
Common Issues When Accessing Object Properties
When accessing properties, users often encounter typical mistakes such as trying to access non-existent properties or making typos. Ensure that you carefully read the output from `Get-Member` and confirm property names.
Utilizing Help and Documentation
PowerShell offers robust help documentation for cmdlets. Utilizing `Get-Help` can clarify how different cmdlets function.
Example:
Get-Help Get-Member -Full
This command fetches comprehensive documentation on the `Get-Member` cmdlet, helping you understand its usage and options.
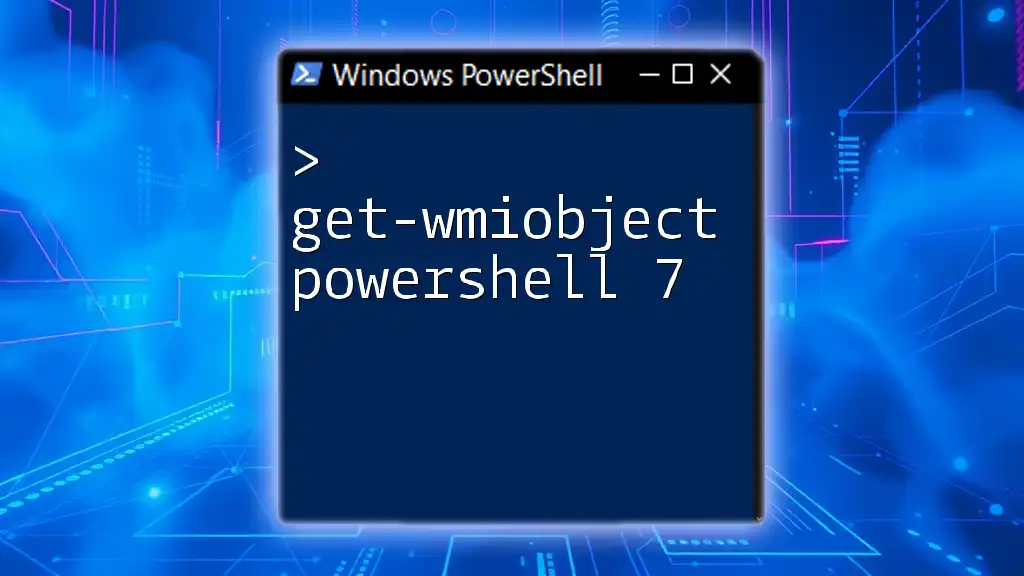
Conclusion
Mastering how to get properties of objects in PowerShell is fundamental for efficient scripting and automation. By exploring commands like `Get-Member`, `Select-Object`, and utilizing custom objects, you can manipulate data effortlessly. Keep practicing these techniques and leverage PowerShell's extensive capabilities to enhance your scripting skills. Don’t hesitate to explore additional resources and engage with the community to deepen your understanding and proficiency in PowerShell scripting.