SQL Server and PowerShell can be combined to automate database management tasks efficiently, allowing users to execute queries and manage server configurations seamlessly.
# Example of retrieving a list of SQL Server databases
$server = "localhost" # Replace with your SQL Server instance
Invoke-Sqlcmd -ServerInstance $server -Query "SELECT Name FROM sys.Databases"
Getting Started with PowerShell for SQL Server
Prerequisites for Using PowerShell with SQL Server
Before diving into the world of SQL Server and PowerShell, it's essential to set up your environment correctly. First, ensure that you have the following software installed:
- SQL Server Management Studio (SSMS): This tool not only helps to manage your databases conveniently, but also facilitates the integration of PowerShell commands.
- SQL Server PowerShell Module: The `SqlServer` module allows you to run various PowerShell commands specifically tailored for SQL Server.
It's also important to have a basic understanding of both PowerShell commands and SQL Server concepts. Familiarity with these concepts will significantly ease your learning curve.
Installing the SQL Server PowerShell Module
To utilize PowerShell with SQL Server effectively, you need to install the SQL Server module. You can do this easily using the following command in your PowerShell console:
Install-Module -Name SqlServer
This command will download and install the latest version of the SQL Server module from the PowerShell Gallery. Always ensure you’re running PowerShell as an administrator to avoid permissions issues during installation.
Connecting to SQL Server using PowerShell
Once the SQL Server module is installed, the next step is to establish a connection to a SQL Server instance. Here’s how to do it:
$SqlServer = "YourServerName"
$Database = "YourDatabaseName"
$ConnectionString = "Server=$SqlServer;Database=$Database;Integrated Security=True;"
$SqlConnection = New-Object System.Data.SqlClient.SqlConnection($ConnectionString)
$SqlConnection.Open()
By defining the server and database names in your connection string, you establish a live connection to SQL Server. If there are any connection issues, PowerShell will throw an error message, which may relate to incorrect server names, user credentials, or network connectivity.
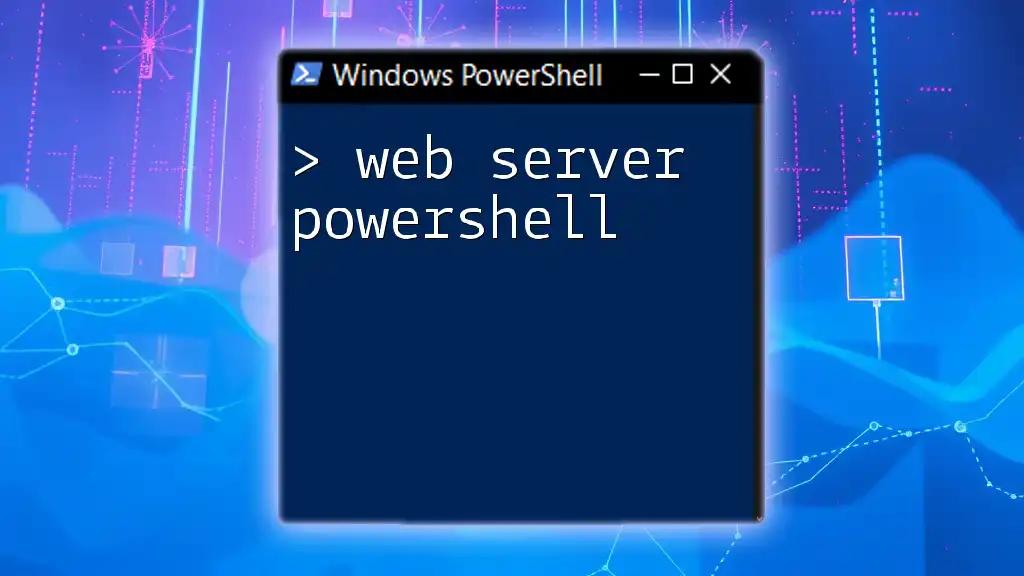
Common PowerShell Commands for SQL Server
Retrieving Database Information
PowerShell makes it easy to fetch information about your databases using the `Get-SqlDatabase` command. This command retrieves a collection of database objects from the specified SQL Server instance. For instance:
Get-SqlDatabase -ServerInstance "YourServerInstance"
The output from this command provides essential details such as database names, status, recovery model, and more, which is invaluable for managing your databases.
Managing SQL Server Jobs
SQL Server Agent jobs are an essential feature for automating tasks within SQL Server. With PowerShell, you can easily create, start, stop, and monitor these jobs.
To create a SQL Server job, you would typically use the following script:
$Job = New-SqlAgentJob -ServerInstance "YourServerInstance" -JobName "YourJobName"
Add-SqlAgentJobStep -Job $Job -Database "YourDatabaseName" -Command "Your SQL Command Here"
To start a job, execute the command below:
Start-SqlAgentJob -Name "YourJobName" -ServerInstance "YourServerInstance"
Monitoring your SQL Server jobs is just as easy. Using `Get-SqlAgentJob` will allow you to see the current status of your jobs.
Executing SQL Queries
PowerShell also simplifies running SQL queries against your database. If you need to execute a SQL command, you can do so with the following script:
$SqlCommand = $SqlConnection.CreateCommand()
$SqlCommand.CommandText = "SELECT * FROM YourTable"
$SqlReader = $SqlCommand.ExecuteReader()
This code sets up a command to select all rows from a specified table. The returned data can then be processed for reporting, logging, or even display purposes.
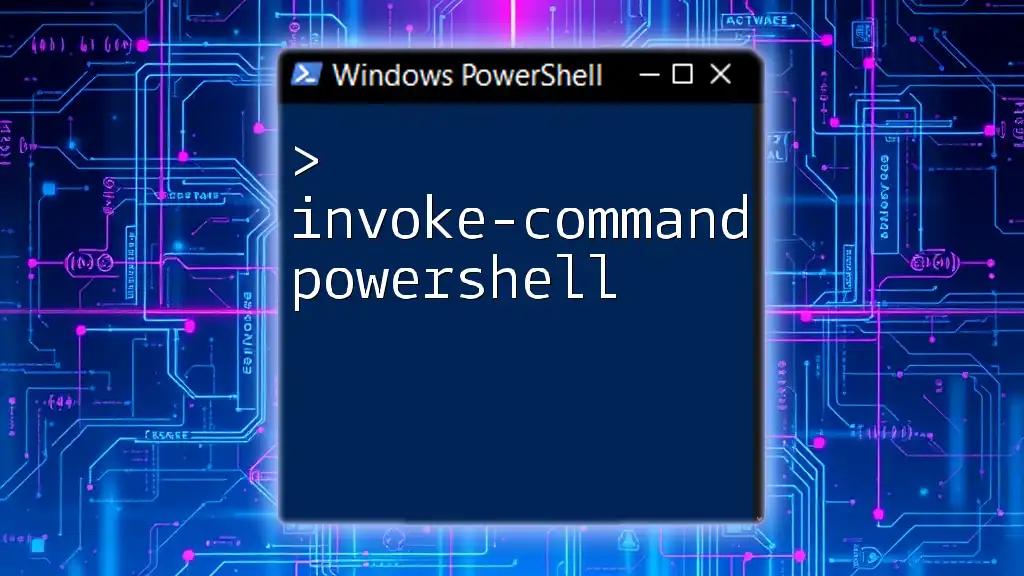
Automating SQL Server Maintenance Tasks
Backing Up a Database
One of the critical tasks in database administration is the backup process. Automating this task with PowerShell eliminates the risk of human error and ensures regular backups. The following command will back up your database:
Backup-SqlDatabase -ServerInstance "YourServerInstance" -Database "YourDatabase" -BackupDirectory "C:\Backups\" -Overwrite
Be sure to adjust the backup directory to a location that is appropriate for your environment.
Restoring a Database
In the unfortunate event of data loss, knowing how to programmatically restore a database is crucial. The following command restores a database from a previously created backup:
Restore-SqlDatabase -ServerInstance "YourServerInstance" -Database "YourDatabase" -BackupFile "C:\Backups\YourBackupFile.bak"
This command brings your database back to its last known good state swiftly and efficiently.
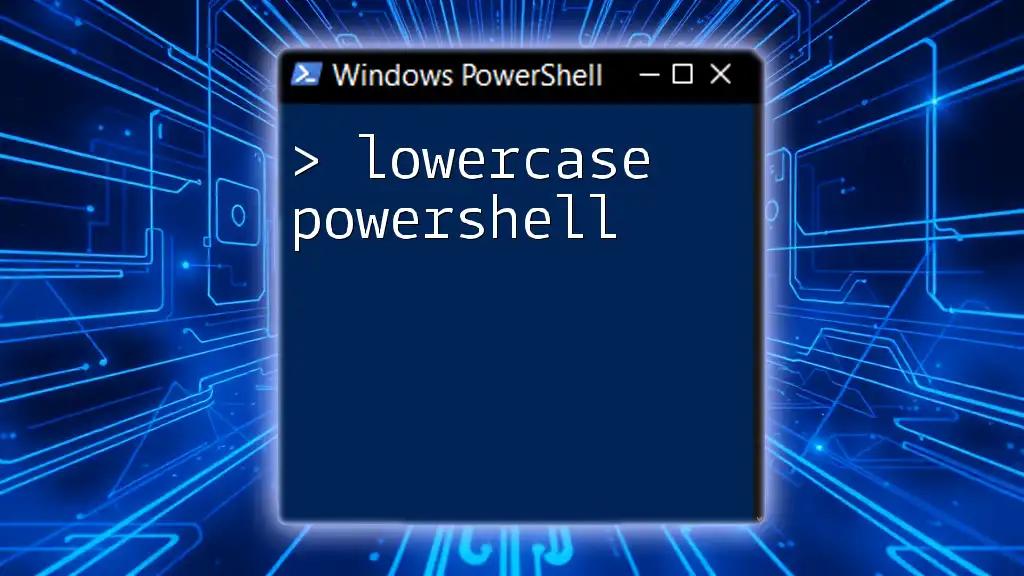
Advanced PowerShell Management Techniques for SQL Server
Using PowerShell with SQL Server Agent
SQL Server Agent is a powerful tool for scheduling and executing jobs in SQL Server. If you want to create alerts and operators, PowerShell allows for seamless integrations. For instance, you could set up alerts to notify you whenever a job fails.
Monitoring SQL Server Performance
To ensure SQL Server operates optimally, you must monitor its performance regularly. PowerShell can retrieve various performance counters to keep you informed about your SQL Server environment:
Get-Counter -Counter "\SQLServer:Buffer Manager\Buffer cache hit ratio"
This command provides insight into how effectively your server is utilizing its buffer cache, allowing you to adjust performance settings accordingly.
PowerShell Scripting Best Practices
When working with PowerShell, adherence to best practices for scripting is essential for maintaining scripts effectively. This includes writing clear, reusable code and implementing robust error handling and logging mechanisms. Here’s a snippet demonstrating a simple error-handling technique:
try {
# Your code execution here
} catch {
Write-Host "An error occurred: $_"
}
Implementing such practices not only aids in debugging but also makes your scripts more maintainable in the long run.
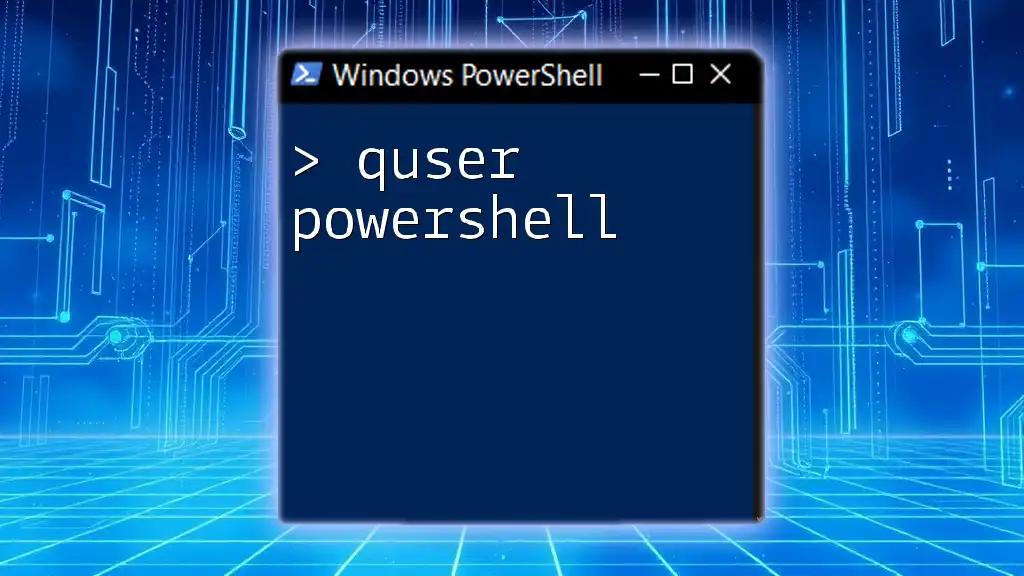
Conclusion
The integration of SQL Server and PowerShell opens up a world of possibilities for efficient database management. Whether automating routine tasks, fetching data, or monitoring performance, PowerShell commands empower database administrators to streamline their work processes.
As you continue your journey into PowerShell, remember to practice regularly and explore the vast array of commands available. For those wanting to delve deeper into these subjects, consider enrolling in structured courses to enhance your skills further.