In PowerShell, the `Wrap-LongLines` function helps format long command output by ensuring it wraps neatly within the console window for better readability. Here's a simple code snippet demonstrating how to use it:
Write-Host 'This is a very long line that will automatically wrap around to the next line based on the console width.'
Understanding PowerShell Output
What is PowerShell Output?
In PowerShell, output refers to the data produced by commands. This output can take various forms, including text output, object representations, or formatted tables. By default, PowerShell presents data in a way that aims to balance detail and readability, but sometimes, this can lead to overly long lines, making the information harder to digest.
Why Line Length Matters
When lines of output are excessively long, they can become difficult to read and parse. This is particularly significant in scripts and command outputs where understanding the content quickly can optimize workflow. By ensuring that lines are appropriately wrapped, users facilitate better reading, quicker comprehension, and easier navigation through complex outputs.
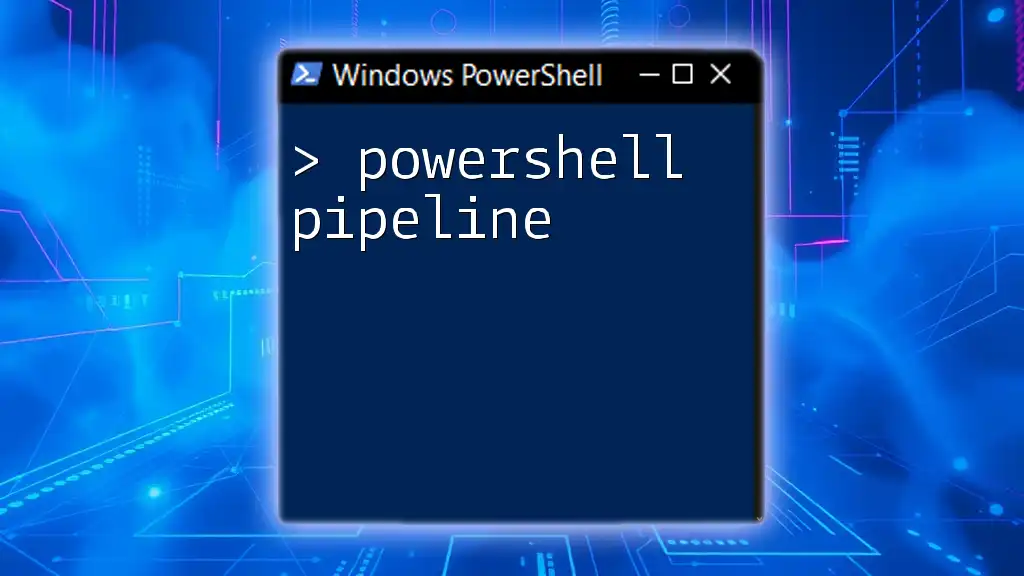
The Power of Line Wrapping
What is Line Wrapping in PowerShell?
Line wrapping in PowerShell refers to the automatic continuation of text onto the next line when it exceeds a set length. Rather than allowing long lines to spill off the screen, which can lead to confusion, line wrapping helps maintain readability by breaking up the information into more digestible segments.
Benefits of Line Wrapping
Using line wrapping offers several advantages:
- Improving readability: Wrapped lines ensure that users can view all relevant information without needing to scroll horizontally.
- Making scripts more maintainable: When scripts contain fewer long lines, they become easier to debug and modify.
- Enhancing user experience: Users can quickly grasp the information presented without being overwhelmed by extensive lines of text.
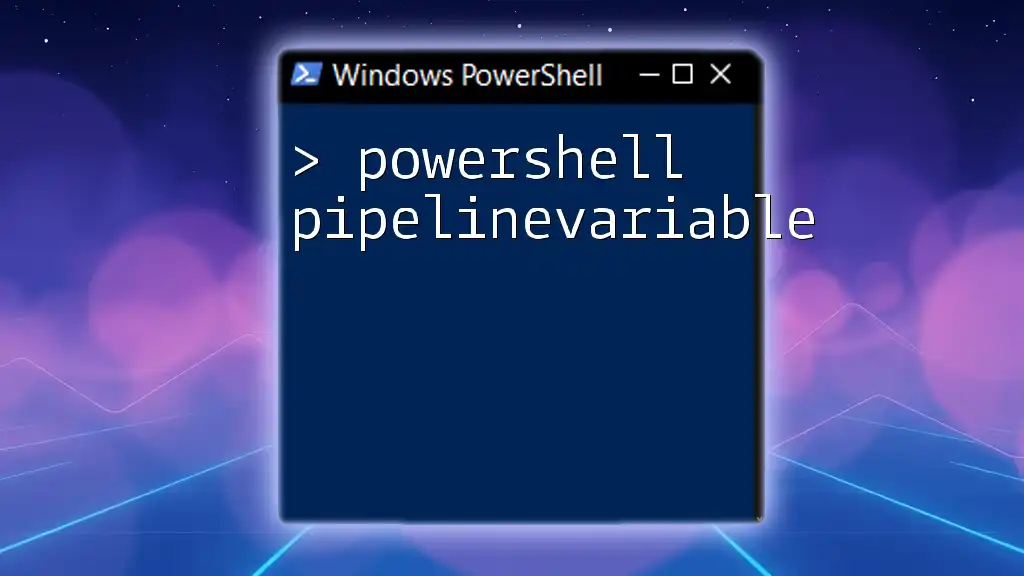
Using the `Format-Table` Cmdlet
Introduction to `Format-Table`
The `Format-Table` cmdlet is a powerful tool in PowerShell, used to format the output of commands into tables. It helps you present data in an organized manner. The `-Wrap` parameter specifically instructs PowerShell to wrap long lines within the table cells.
Example of Wrapping Lines with `Format-Table`
To see the power of the `Format-Table` cmdlet in action, consider the following command:
Get-Process | Format-Table -Wrap -AutoSize
This command retrieves a list of currently running processes and formats the output as a table, automatically adjusting the column widths and wrapping long entries. With the `-Wrap` flag, the table header and row contents will adjust dynamically, ensuring that even lengthy process names fit gracefully within the confines of the table format.
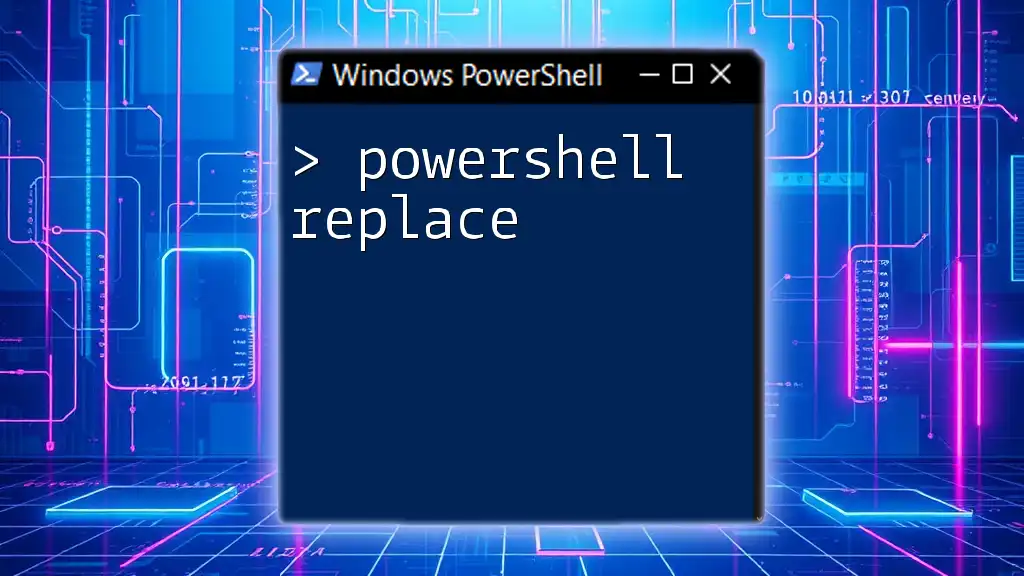
Adjusting Line Width with `Out-Default`
What is `Out-Default`?
The `Out-Default` cmdlet in PowerShell outputs command results to the default display output for your environment. Adjusting how it displays output can significantly improve your workflow, especially when dealing with larger data sets.
Example of Adjusting Output Width
To modify how wide the output can be, you can set the width parameter globally:
$PSDefaultParameterValues['Out-Default:Width'] = 100
This command tells PowerShell to limit the output width to 100 characters for all commands that use `Out-Default`. Adjusting the width allows for better organization of results, ensuring they will wrap appropriately within the set limits, enhancing both readability and presentation.
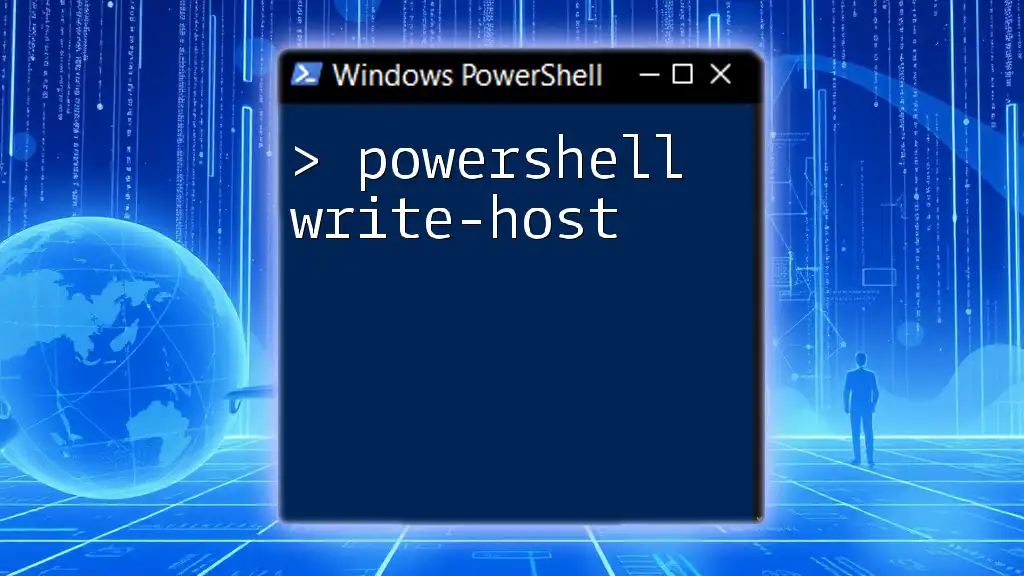
PowerShell ISE and Visual Studio Code Settings
Utilizing PowerShell ISE
The PowerShell Integrated Scripting Environment (ISE) provides an interactive environment for running PowerShell commands. Within ISE, you can adjust your line wrapping settings through the preferences, ensuring that long lines of code or output do not make the interface cluttered. This helps maintain a clean coding environment and improves your coding experience.
Visual Studio Code Configuration
Visual Studio Code (VS Code) has become a highly favored editor for PowerShell scripting. To enable line wrapping here, navigate to File -> Preferences -> Settings, and search for "word wrap." Set it to `on` or `bounded`, based on your preference.
Example settings may include options to wrap at the editor's width, accordingly keeping your code neat and aligned. Such configurations help maintain focus and organization, especially as script complexity grows.
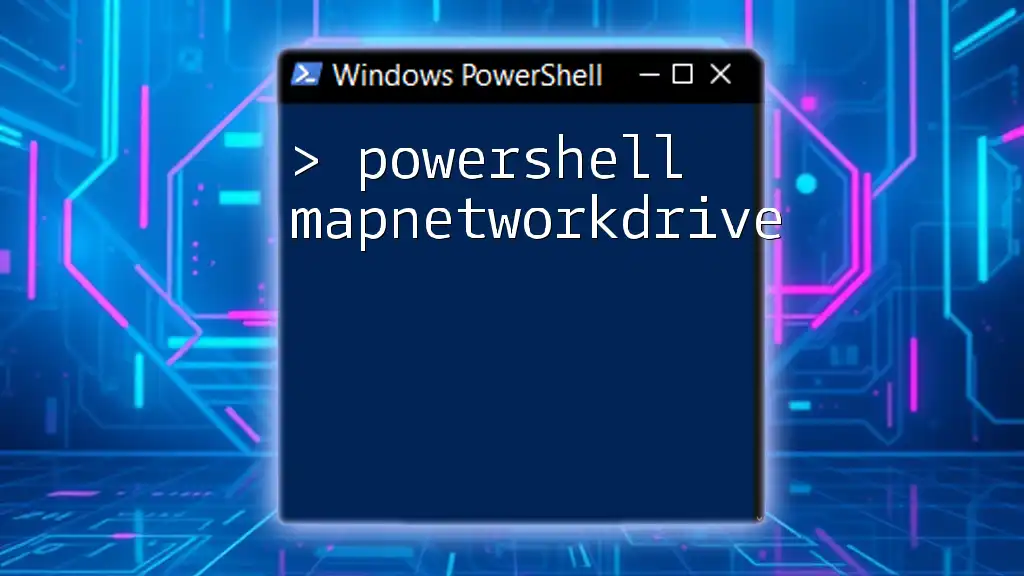
Conclusion
In summary, becoming comfortable with how to powerfully utilize line wrapping in PowerShell significantly improves both the readability of your output and the maintainability of your scripts. It's an essential skill for any PowerShell user looking to enhance their efficiency and usability. By mastering commands like `Format-Table`, leveraging the `Out-Default` settings, and appropriately configuring your scripting environment in ISE or VS Code, you can ensure that your PowerShell experience is both productive and enjoyable. Practice these techniques, and you will find your workspace transformed for the better.