In PowerShell, you can calculate the time difference between two dates by subtracting the earlier date from the later date, resulting in a TimeSpan object that represents the duration between them.
$startTime = Get-Date '2023-10-01 10:00'
$endTime = Get-Date '2023-10-01 15:30'
$timeDifference = $endTime - $startTime
Write-Host "The time difference is: $timeDifference"
Understanding DateTime Objects
What is a DateTime Object?
In PowerShell, a DateTime object is a fundamental data type that represents an instant in time, typically expressed as a date and time. Understanding these objects is crucial since you will frequently deal with time-based data in scripting and automation tasks. Unlike basic data types like strings and integers, DateTime objects provide methods and properties that allow for complex calculations and manipulations.
Creating DateTime Objects
Creating DateTime objects is straightforward, and you often do it using the `Get-Date` cmdlet. For instance, if you want to create two date objects for New Year’s Day and the end of the year, you would write:
$date1 = Get-Date "2023-01-01"
$date2 = Get-Date "2023-12-31"
You can also create DateTime objects using different formats. For example:
$date3 = [datetime]::ParseExact("31/12/2023", "dd/MM/yyyy", $null)
This versatility allows you to accommodate various date formats based on your needs.
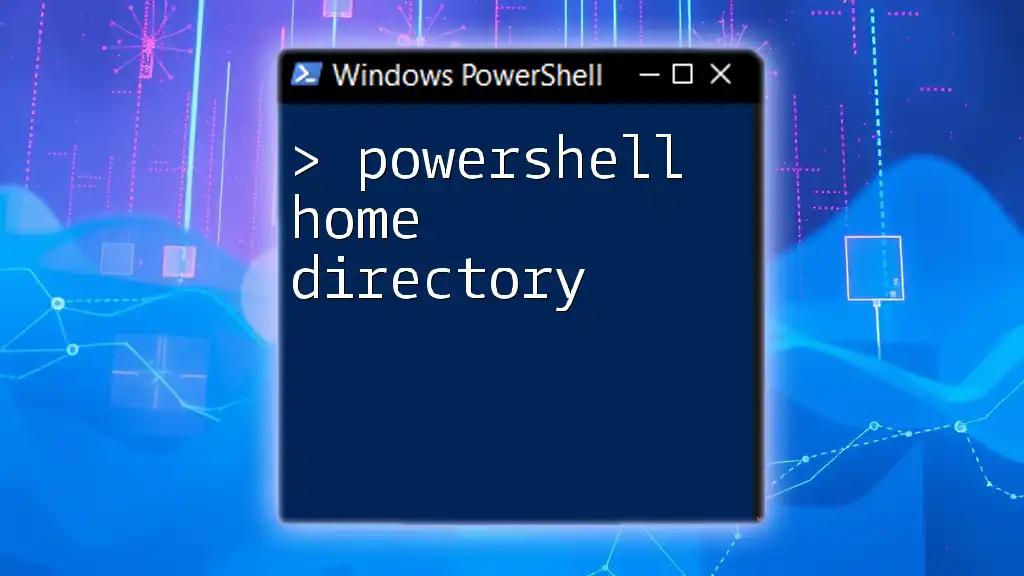
Calculating Time Differences
Using Subtraction to Find Differences
One of the simplest ways to calculate the time difference in PowerShell is to subtract one DateTime object from another. This operation returns a TimeSpan object representing the duration between the two dates. Here’s how it’s done:
$difference = $date2 - $date1
Write-Host "The difference is: $difference"
The output will display the total difference, showcasing days, hours, minutes, and seconds.
Understanding the Output
The result of this subtraction is a TimeSpan object. This object contains various properties that allow you to interpret the difference. Here’s an example of how to access these properties:
Write-Host "Days: $($difference.Days) Hours: $($difference.Hours) Minutes: $($difference.Minutes)"
In this case, you can break down the difference into exactly how many days, hours, and minutes are between the two dates.
Formatting Time Differences
Using .Total* Properties
PowerShell provides several properties to capture the total amount of time in different units. If you required the total seconds or total minutes, you can easily retrieve these as follows:
$totalSeconds = $difference.TotalSeconds
Write-Host "Total seconds: $totalSeconds"
These properties are particularly useful for calculations where precise timing is essential.
Example Scenarios
Finding the Age of a Person
To illustrate how time differences are useful, let’s say you want to calculate someone's age. This can be done by comparing a birth date to the current date.
$birthDate = Get-Date "1990-06-15"
$age = (Get-Date -Format "yyyy") - $birthDate.Year
Write-Host "Age: $age years"
This straightforward calculation provides the age in years at a given time, assuming the day and month have already passed in the current year.
Time Between Events
You might also want to calculate the time between two specific events. For instance, to find the time between two meetings scheduled on the same day:
$event1 = Get-Date "2023-10-15 10:00AM"
$event2 = Get-Date "2023-10-15 12:30PM"
$timeBetween = $event2 - $event1
Write-Host "Time between events: $timeBetween"
This simple operation can help you manage schedules efficiently.

Advanced Time Manipulations
Adding and Subtracting Time
Another powerful feature of DateTime objects is the ability to add or subtract time. This is especially useful for future planning or historical analysis. For instance, to calculate a date 30 days from a specific event:
$futureDate = $date1.AddDays(30)
Write-Host "Future date: $futureDate"
Comparing Dates
When working with multiple dates, you often need to know if one date is earlier or later than another. This can be done using comparison operators:
if ($date1 -gt $date2) {
Write-Host "$date1 is greater than $date2"
} else {
Write-Host "$date1 is not greater than $date2"
}
This conditional statement helps you make decisions based on date comparisons in your scripts.
Working with Time Zones
Understanding Time Zone Differences
Dealing with different time zones adds complexity but can be effectively managed in PowerShell. For instance, converting UTC time to a specific time zone can be accomplished with:
$utcTime = [datetime]::UtcNow
$localTime = [System.TimeZoneInfo]::ConvertTimeBySystemTimeZoneId($utcTime, "Pacific Standard Time")
Write-Host "Local Time: $localTime"
This conversion ensures your scripts account for local times when needed.

Best Practices
Handling Edge Cases
When working with dates and times, be aware of edge cases like leap years and daylight saving time. For instance, to check if a year is a leap year:
$leapYear = [datetime]::IsLeapYear(2024)
Write-Host "Is 2024 a leap year? $leapYear"
Such checks can be crucial in ensuring the accuracy of your time-based calculations.
Performance Considerations
When processing large datasets involving DateTime calculations, performance optimization becomes important. Instead of calculating DateTime differences on individual items, consider batching your processes to increase efficiency.
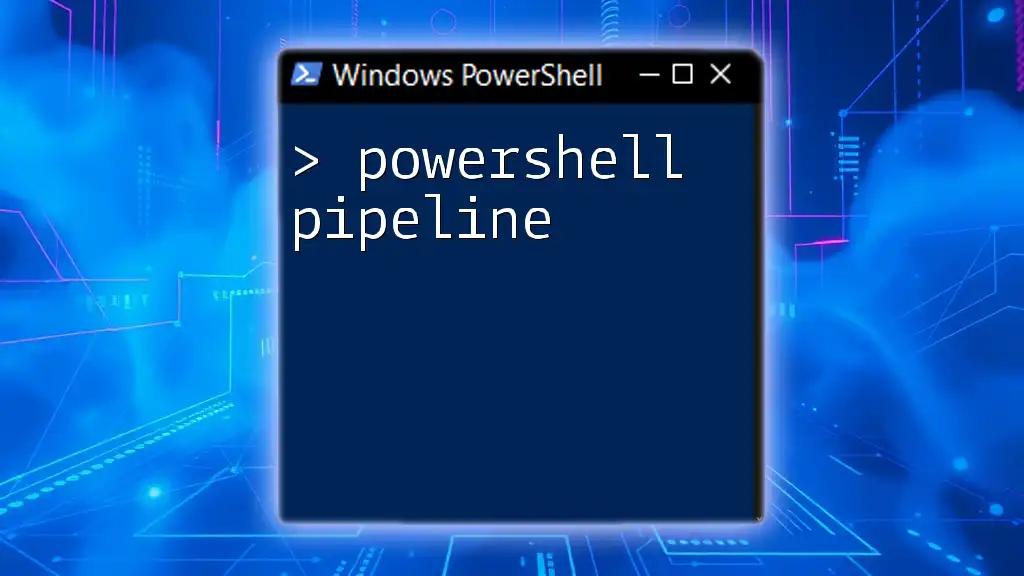
Conclusion
A solid understanding of PowerShell time differences is invaluable for scripting and automation tasks. By mastering DateTime objects, performing calculations, and accounting for time zones, you greatly enhance your ability to manage and manipulate date and time data effectively. Be sure to practice these concepts with real-world scenarios as you continue to explore more advanced topics in PowerShell.