The PowerShell MSI product code is a unique identifier used to differentiate software installations, enabling you to manage and manipulate Windows Installer packages effectively.
Here’s a code snippet to retrieve the MSI product code of an installed application:
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'YourAppName'" | Select-Object -Property IdentifyingNumber
Understanding MSI Product Codes
What is an MSI Product Code?
An MSI Product Code is a unique identifier for a software application that is installed via the Microsoft Installer (MSI). It is represented as a GUID (Globally Unique Identifier) and plays a crucial role in managing installations and uninstallation processes. When an application is installed using an MSI package, the installer registers this product code in the system, allowing administrators to easily manage and reference that application.
Importance of Product Codes
Product codes are essential for several reasons:
- Automated Deployments: In enterprise environments where software is deployed automatically, having product codes simplifies the process by ensuring specific versions of applications are referenced correctly.
- Troubleshooting: When issues arise with installed software, knowing the product code can help in troubleshooting or reinstallation without manually searching for applications.
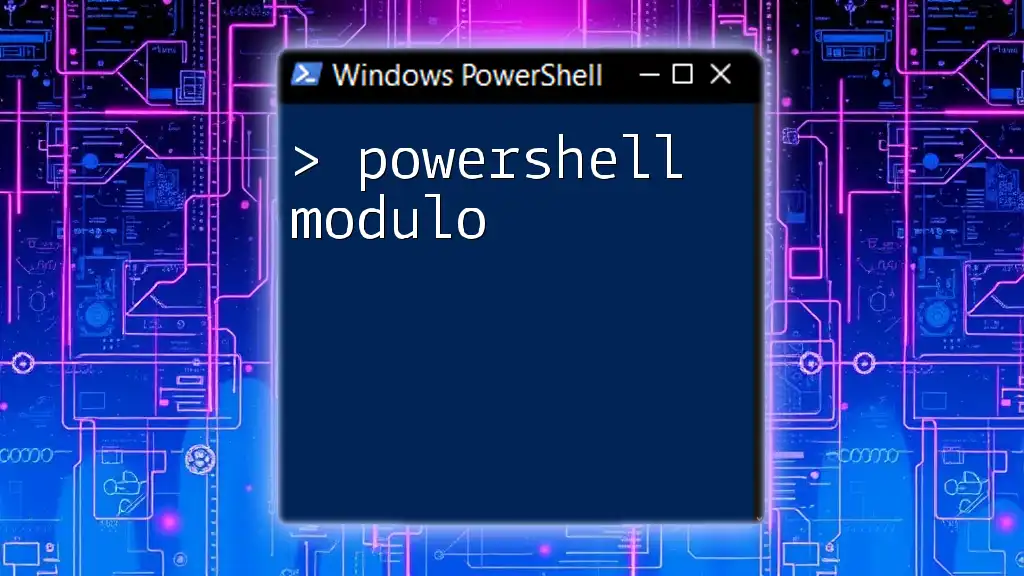
Finding the MSI Product Code
Using PowerShell to Retrieve Product Codes
PowerShell is a powerful tool that allows administrators to interact with the Windows environment effortlessly. One of the simplest methods to retrieve installed software and their product codes is to utilize the `Get-WmiObject` cmdlet.
Example: Retrieving Installed Software and Their Product Codes
To get a list of software installed on your machine along with their product codes, you can run the following command:
Get-WmiObject -Query "SELECT * FROM Win32_Product" | Select-Object Name, IdentifyingNumber
In this command, `Win32_Product` is a WMI class that represents all the products installed via Windows Installer. The output lists all installed applications with their corresponding product codes (IdentifyingNumber).
Filtering Installed Applications
In situations where you want the product code for a specific application, filtering is crucial.
Example: Find a Specific Application's Product Code
Here's how you can find the product code for a specific application:
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'YourApplicationName'" | Select-Object IdentifyingNumber
By replacing `YourApplicationName` with the actual name of the application, you can easily retrieve its product code. This targeted approach saves time and keeps information organized.
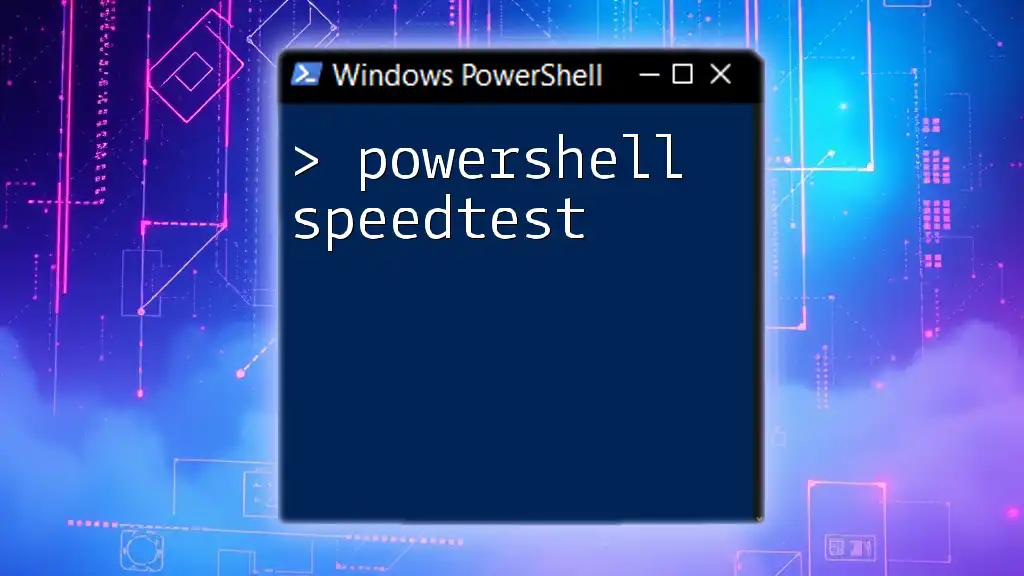
Working with MSI Product Codes
Installing Applications Using Product Codes
To install applications using MSI Product Codes, you will typically use the `msiexec` command. This command-line utility is designed to manage installation and uninstallation processes.
Example: Installing an Application
To install an application using its product code, you can utilize the following PowerShell command:
Start-Process msiexec.exe -ArgumentList '/i {ProductCode} /qn'
In this command:
- `/i` indicates that you want to install the application.
- `{ProductCode}` should be replaced with the actual product code you wish to install.
- `/qn` runs the installation quietly without user interaction, making it perfect for automated scripts.
Uninstalling Applications Using Product Codes
Just as you can install applications by referencing their product codes, you can also uninstall them.
Example: Uninstalling an Application
To uninstall an application, the command resembles the installation process:
Start-Process msiexec.exe -ArgumentList '/x {ProductCode} /qn'
In this context:
- `/x` indicates the intention to uninstall the application. Replacing `{ProductCode}` with the correct identifier will ensure the right application is removed from the system, again utilizing `/qn` for a quiet operation.
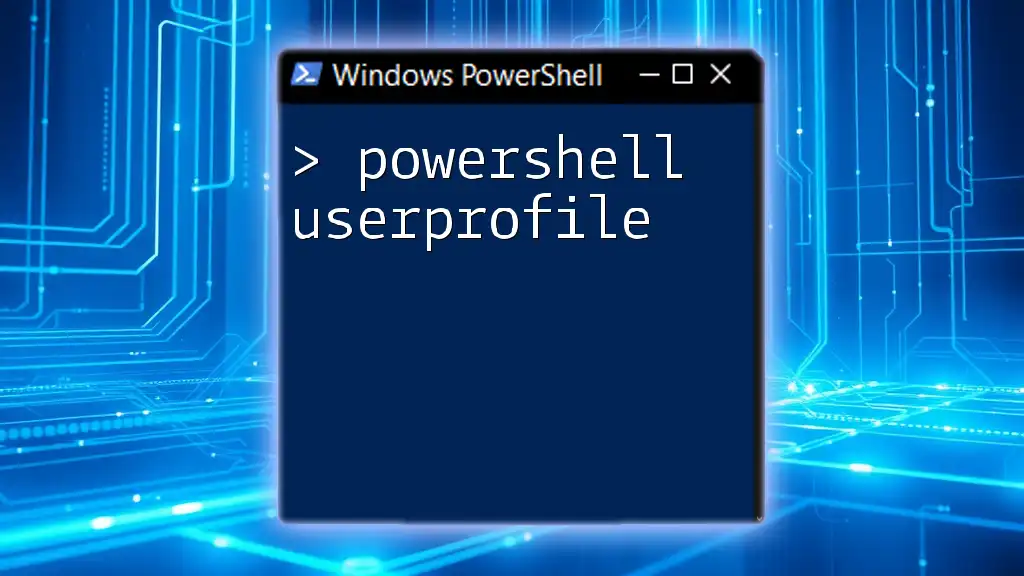
Handling Common Issues
Troubleshooting Uninstallation Problems
Sometimes, uninstallation may not go as planned. Common problems include application locks or missing permissions. Ensuring that you have administrative rights is paramount for executing these commands successfully.
Verifying Installation and Uninstallation
After performing installations or uninstallations, confirming the state of the software is crucial.
Example: Confirming Installation
You can verify if an application is currently installed by executing:
Get-WmiObject -Query "SELECT * FROM Win32_Product WHERE Name = 'YourApplicationName'"
This command checks if the specified application still exists in the installed products list, providing valuable confirmation.
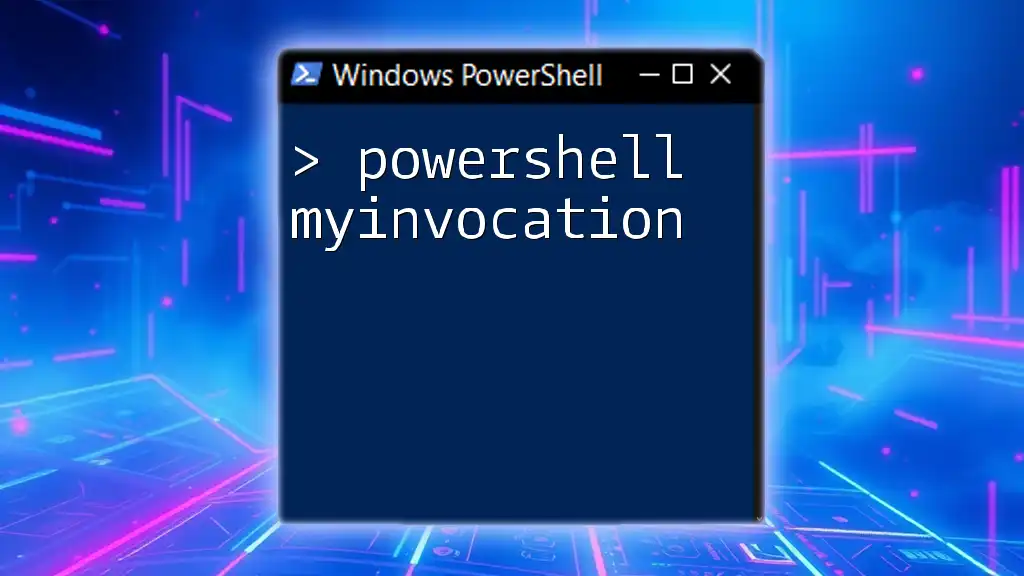
Advanced PowerShell Techniques
Using PowerShell Scripts for Bulk Operations
In a production environment, you may often need to install or uninstall multiple applications simultaneously. Leveraging PowerShell scripts allows for efficient management.
Example: Bulk Uninstallation Script
You can create a simple script for bulk uninstallation like this:
$productsToUninstall = @('{ProductCode1}', '{ProductCode2}', '{ProductCode3}')
foreach ($product in $productsToUninstall) {
Start-Process msiexec.exe -ArgumentList "/x $product /qn"
}
This script declares an array of product codes and loops through each code to uninstall the corresponding application quickly. It demonstrates how PowerShell can streamline repetitive tasks.
Event Logging and Reporting
Another best practice is to implement logging for installation and uninstallation actions. This is beneficial for auditing and troubleshooting.
Example: Logging Install/Uninstall Actions
To log installation activity, you can extend the installation command as follows:
Start-Process msiexec.exe -ArgumentList '/i {ProductCode} /qn' -PassThru | Out-File -FilePath "C:\Logs\install.log"
This command creates an installation log that can assist you later in tracking installations and diagnosing issues.
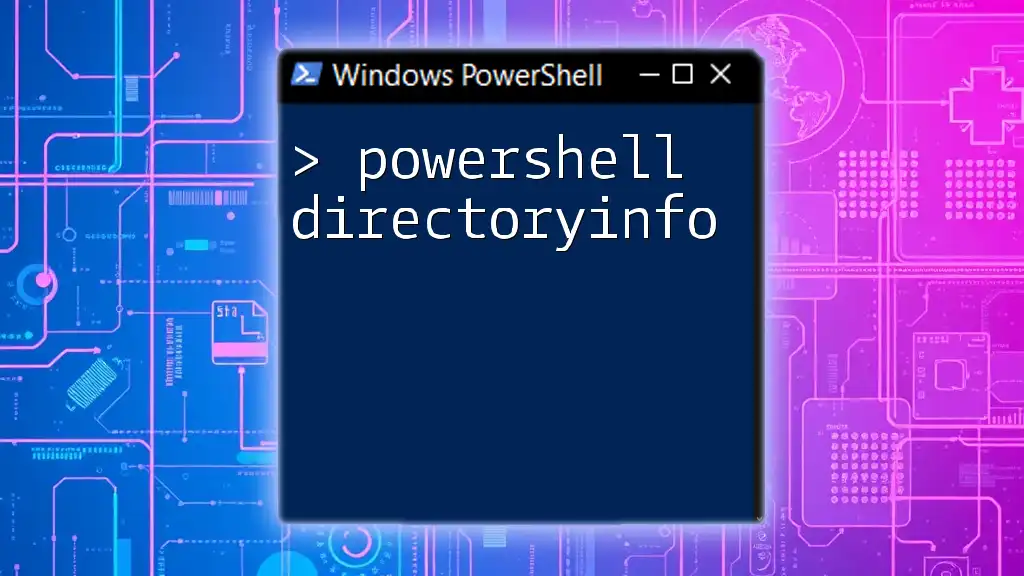
Conclusion
Understanding how to manage MSI Product Codes using PowerShell is invaluable for anyone involved in IT and systems administration. It not only streamlines software management processes but also aids in automating repetitive tasks. Embracing PowerShell allows you to enhance your technical skills and improve efficiency in managing Windows applications.
Consider exploring more about PowerShell commands and their practical applications to make your workflow more effective. You’ll find that with practice, these commands can become second nature, opening new avenues for managing and automating systems.