To retrieve information about a Group Managed Service Account (gMSA) in PowerShell, you can use the `Get-ADServiceAccount` cmdlet. Here's a code snippet to do just that:
Get-ADServiceAccount -Identity "YourGMSAName"
Replace `"YourGMSAName"` with the actual name of the gMSA you want to query.
Understanding GMSA
What is a Group Managed Service Account?
A Group Managed Service Account (GMSA) is a special type of managed service account designed for use in scenarios where multiple servers or services need to share the same identity for authentication. Unlike traditional service accounts, GMSAs provide enhanced security and management features, including automatic password management and simplified service principal name (SPN) management.
The main advantages of using GMSA include:
- Automatic password management: Passwords are automatically updated and securely managed, reducing the risk of password-related vulnerabilities.
- Simplified deployment: GMSAs can be used across multiple systems, making deployment and management easier than traditional accounts.
- Enhanced security: By using managed service accounts, you eliminate the need for hard-coded credentials in applications and services.
How GMSA Works
GMSAs operate by allowing Active Directory to manage the password for the service account. Instead of manually managing passwords or using prior service account implementations, you can leverage the inherent security capabilities of GMSA. When an application requires authentication, the GMSA retrieves its credentials automatically, ensuring consistent and secure access.

Setting Up the Environment
Requirements for Using GMSA
To effectively utilize GMSA accounts, you need:
- Active Directory Domain Services (AD DS): Ensure you have a functioning Active Directory environment.
- Windows Server: GMSAs require specific components available in Windows Server 2012 and later.
- Permissions: You'll need the right permissions to create, manage, and query GMSA accounts in your domain.
Prerequisite PowerShell Modules
Before you begin using PowerShell to manage GMSA accounts, make sure you have the required PowerShell module installed. The Active Directory Module is essential for utilizing the `Get-ADServiceAccount` cmdlet. You can check if the module is available with the following command:
Get-Module -ListAvailable ActiveDirectory
If it's not installed, you'll need to install the Remote Server Administration Tools (RSAT) that include the Active Directory module.
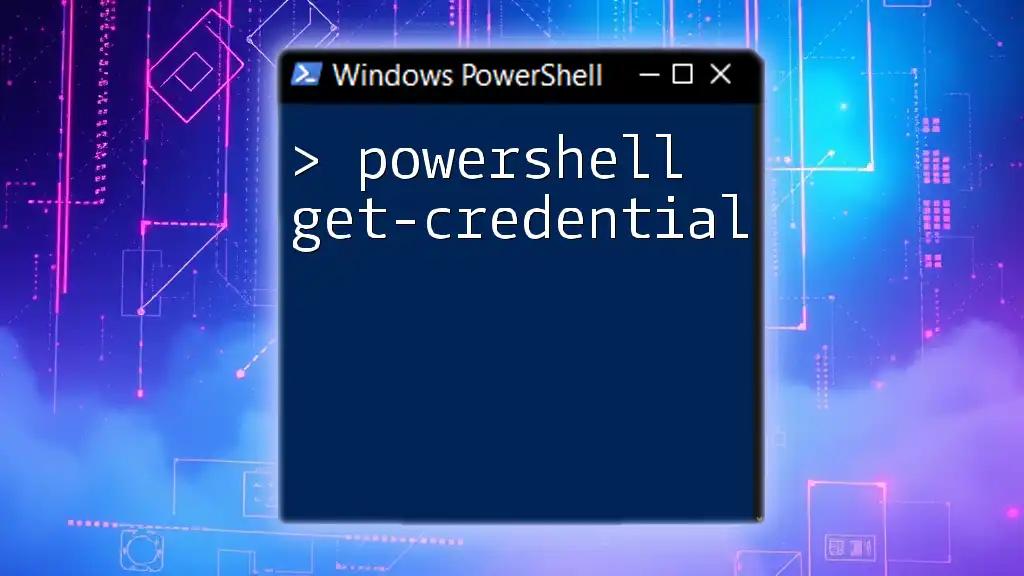
Using Get-ADServiceAccount
Syntax of Get-ADServiceAccount
The `Get-ADServiceAccount` cmdlet is the primary tool for retrieving information about GMSAs. Its basic syntax is as follows:
Get-ADServiceAccount -Identity <GMSA_Name>
Common Parameters
-
-Identity: This parameter allows you to specify the name of the GMSA you want to retrieve. It’s a required parameter.
-
-Filter: With this parameter, you can search for GMSAs based on specific criteria. This is particularly useful if you want to retrieve multiple accounts that meet certain conditions.
-
-Properties: This parameter extends the information retrieved by specifying additional properties to include in the output, such as `ServicePrincipalNames` or `ManagedPassword`.
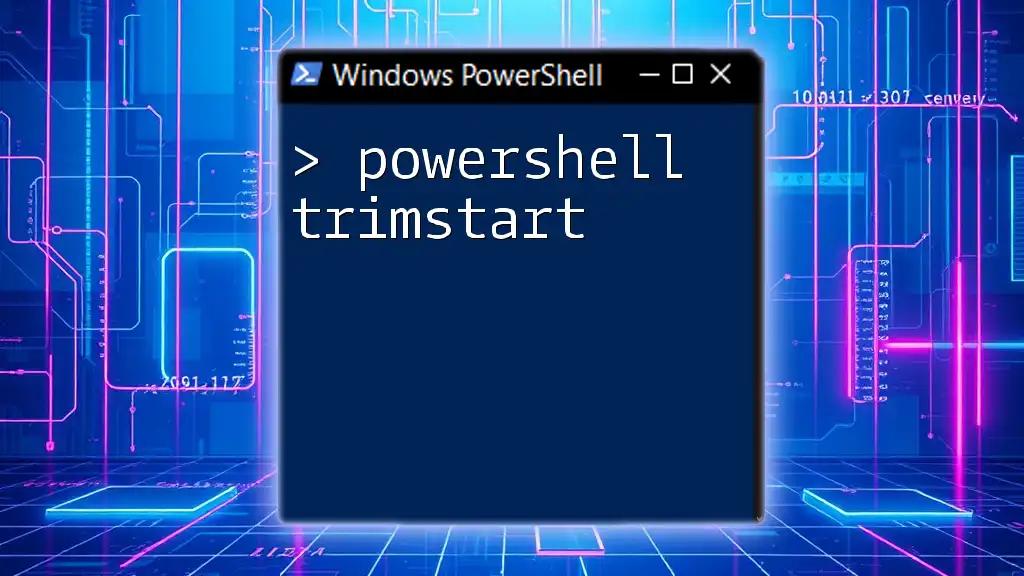
Examples of Using Get-ADServiceAccount
Retrieving a Specific GMSA
To retrieve information about a specific GMSA account, use the following command, replacing `gmsaAccount1` with your GMSA name:
Get-ADServiceAccount -Identity "gmsaAccount1"
The output will display various fields, including:
- Name: The name of the GMSA.
- SamAccountName: The security account name.
- Description: Any description associated with that GMSA account.
Listing All GMSA Accounts
If you want to list all GMSA accounts in your Active Directory, you can utilize the `-Filter` parameter without specifying an identity:
Get-ADServiceAccount -Filter {ServiceAccount -eq $true}
This command retrieves all service accounts marked as GMSAs, giving you a comprehensive overview of available accounts.
Retrieving Specific Properties
You may want to retrieve additional details about a GMSA account. Using the `-Properties` parameter enables you to specify which properties to retrieve. Here’s an example command:
Get-ADServiceAccount -Identity "gmsaAccount1" -Properties ServicePrincipalNames, ManagedPassword
This command will display the specified properties alongside standard information, allowing you to evaluate the account's configurations better.

Filtering and Enhanced Queries
Using Where-Object for Custom Filters
In scenarios where you need to apply custom filters, you can pipe the output of `Get-ADServiceAccount` into the `Where-Object` cmdlet. For example, to retrieve GMSAs with certain descriptions, you can use:
Get-ADServiceAccount -Filter * | Where-Object { $_.Description -like "*web*" }
This command filters out accounts based on your described condition, presenting a focused set of results.
Combining Get-ADServiceAccount with Other Cmdlets
Combining different cmdlets can significantly enhance how you manage and analyze data. Using `Select-Object`, you can streamline the output to specific fields. For example:
Get-ADServiceAccount -Filter * | Select-Object Name, SamAccountName
This retrieves a neatly formatted output containing only names and SAM account names, simplifying the data representation.

Troubleshooting Common Issues
Common Errors When Using Get-ADServiceAccount
When using `Get-ADServiceAccount`, you may encounter various error messages. A common error is:
- "Cannot find an object with the Identity": This error usually indicates a typographical error or that the GMSA does not exist in the specified Active Directory context.
To troubleshoot, double-check the GMSA name and ensure you are querying within the correct domain.
Permissions Troubleshooting
An essential aspect of using Get-ADServiceAccount is ensuring you have the correct permissions. If you're encountering issues, verify your user roles within the Active Directory settings. Ensure that your account has the necessary permissions to read GMSA accounts.
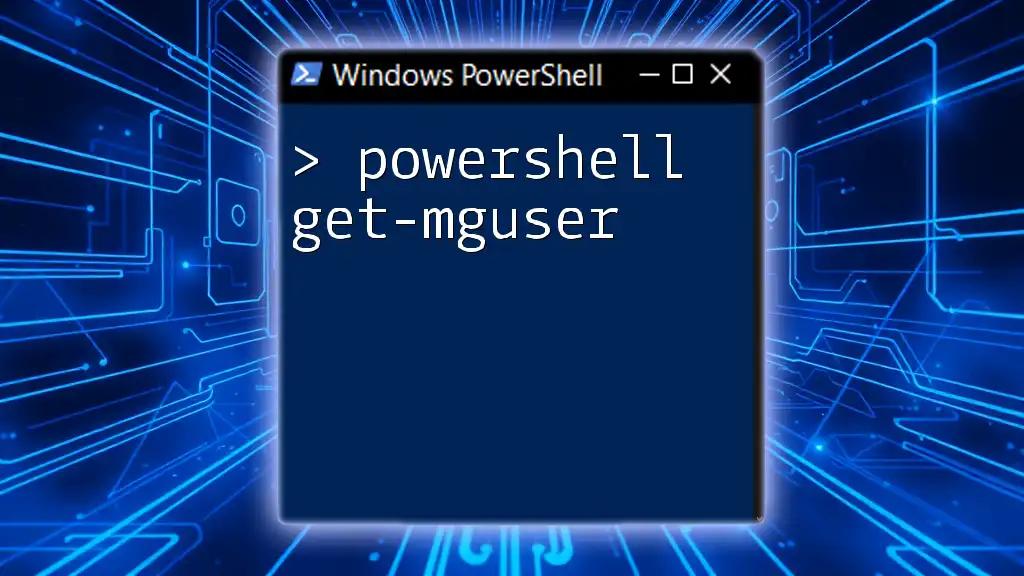
Conclusion
By leveraging the `Get-ADServiceAccount` cmdlet in PowerShell, you can efficiently manage and retrieve information about GMSA accounts in your Active Directory environment. Understanding the various parameters and how to filter results will enhance your PowerShell skills and improve your productivity in managing service accounts.
Eager to learn more about PowerShell and improve your automation capabilities? Stay tuned for more insights!